#6 Data types in Java
Summary
TLDRThis video script delves into the concept of variables and data types in Java programming. It explains the two categories of data types: primitive and non-primitive, focusing on the former. The script outlines the four main primitive data types: integers, floating-point values, characters, and Booleans. It further breaks down integers into byte, short, int, and long, detailing their storage sizes and value ranges. The video also clarifies the use of 'float' and 'double' for floating-point numbers, the importance of using single quotes for characters, and the strict true/false values for Booleans. The script aims to familiarize viewers with the nuances of variable declaration and data type usage in Java.
Takeaways
- 😀 Variables are used to store data in programming, and they are created using a data type and a value.
- 🔢 In Java, there are multiple data types available for storing data, which are categorized into primitive and non-primitive data types.
- 📏 Primitive data types are further divided into integers, floating-point values, characters, and Boolean values.
- 🔢 Integers can be of different subtypes: byte, short, int, and long, each with specific memory sizes and value ranges.
- 📉 Floating-point values include float and double, with float being limited in precision compared to double.
- 🔤 Characters in Java are stored using two bytes, supporting Unicode to represent a wide range of characters from different languages.
- 💡 Boolean data type can only have two values: true or false, and it's used for logical conditions.
- 🛠 When declaring variables, it's important to match the data type with the correct value and notation, such as using 'L' for long integers and 'F' for float values.
- ⚠️ Errors can occur if the value assigned to a variable exceeds the range supported by its data type, such as assigning a value too large for a byte.
- 💻 Understanding the size and range of each data type is crucial for efficient memory usage and accurate data representation in programming.
Q & A
What are the two categories of data types in Java?
-The two categories of data types in Java are primitive and non-primitive. The script primarily discusses primitive data types.
What does the term 'primitive' mean in the context of data types?
-Primitive, in the context of data types, means that the data type is basic and simple to use, directly supported by the Java language.
List the four main categories of primitive data types in Java.
-The four main categories of primitive data types in Java are integers, floating-point values, characters, and Boolean.
What are the subtypes of the integer data type in Java?
-The subtypes of the integer data type in Java are byte, short, int, and long.
What is the size and range of the 'int' data type in Java?
-The size of the 'int' data type in Java is 4 bytes (32 bits), and its range is from -2^31 to 2^31-1 (-2,147,483,648 to 2,147,483,647).
Why would you use the 'byte' data type in Java?
-You would use the 'byte' data type in Java to save memory when you need to store a smaller number within the range of -128 to 127.
How do you define a floating-point number in Java?
-In Java, to define a floating-point number, you can use 'float' or 'double'. By default, a number with a decimal point is considered a 'double', but you can specify 'float' by appending 'f' or 'F' to the number.
What is the difference between 'float' and 'double' in Java?
-In Java, 'float' takes 4 bytes and has a limited precision, while 'double' takes 8 bytes and provides a higher precision, which is suitable for scientific calculations.
How do you define a character variable in Java?
-To define a character variable in Java, you use the 'char' keyword, followed by the variable name and a single quote around the character, for example, `char c = 'K';`.
What are the valid values for a Boolean data type in Java?
-The valid values for a Boolean data type in Java are 'true' and 'false', with no numeric equivalents like 0 or 1.
What is the significance of the 'l' suffix when defining a long integer in Java?
-The 'l' or 'L' suffix, when used with an integer, signifies that the number is of the 'long' data type, which requires this suffix to differentiate it from other integer types.
Outlines
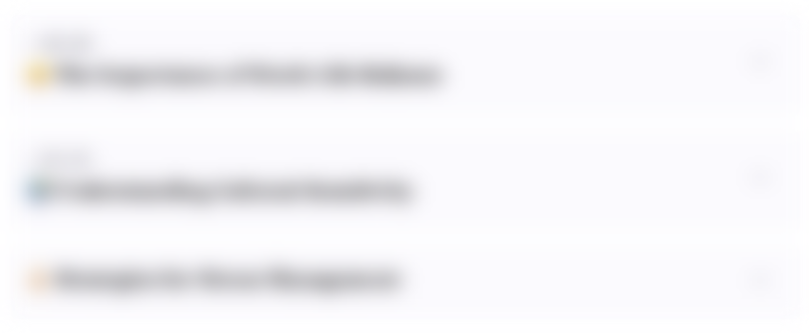
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
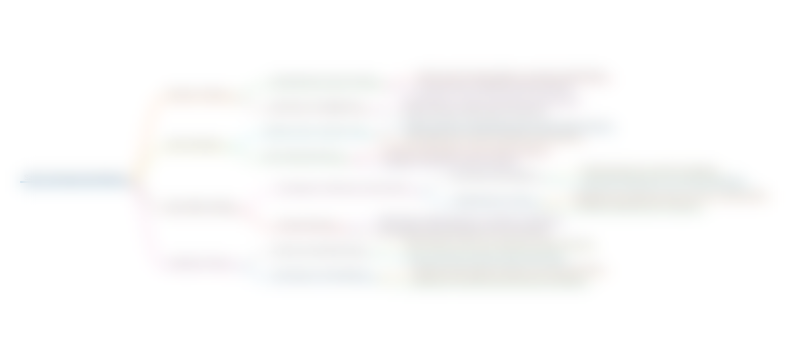
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
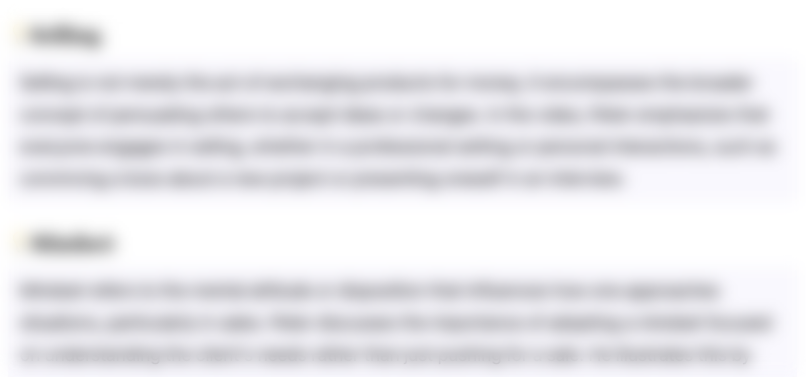
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
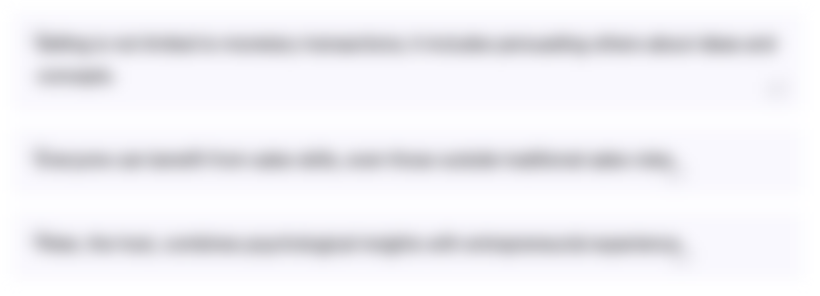
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
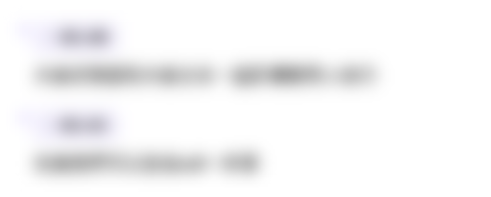
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)