Longest Increasing Subsequence NlogN | Leetcode #300 | LIS
Summary
TLDRThis video introduces an efficient method for finding the length of the Longest Increasing Subsequence (LIS) in an array. The algorithm uses dynamic programming with a binary search technique to maintain a strictly increasing subsequence in a one-dimensional array. The key steps involve finding the appropriate position for each element using a lower bound, either appending the element or overwriting an existing one. The video explains the approach with simple code and encourages viewers to apply this efficient solution for solving LIS problems in a more optimized way.
Takeaways
- 😀 The Longest Increasing Subsequence (LIS) problem aims to find the longest subsequence in an array where the elements are strictly increasing.
- 😀 A brute-force solution to LIS involves checking every combination, which results in an inefficient O(n^2) time complexity.
- 😀 A more optimal solution can be achieved using binary search, reducing the time complexity to O(n log n).
- 😀 By iterating over the array and updating an auxiliary array, LIS can be solved efficiently.
- 😀 The key operation is finding the 'lower bound,' which is the position where an element can be inserted while maintaining the increasing order.
- 😀 If the element is larger than all current elements in the array, it is appended at the end.
- 😀 If the element is smaller than an existing element in the array, the algorithm overwrites that element with the new one to maintain the subsequence order.
- 😀 This approach efficiently maintains the LIS sequence, using binary search for optimal performance.
- 😀 The space complexity of the algorithm is proportional to the size of the original array, ensuring minimal memory usage.
- 😀 The code for this approach is relatively simple once the underlying theory is understood, and it is highly efficient for large datasets.
Q & A
What is the main goal of the algorithm discussed in the video?
-The main goal of the algorithm is to find the length of the Longest Increasing Subsequence (LIS) in an array of numbers.
What approach is used to solve the Longest Increasing Subsequence problem in this video?
-The video uses a dynamic programming approach combined with binary search to efficiently find the LIS length in O(n log n) time.
Why is binary search used in this algorithm?
-Binary search is used to find the correct position where an element should be placed in the list `L` (which represents the smallest possible ending elements of increasing subsequences). This ensures efficient placement and reduces time complexity.
How does the list `L` help in solving the LIS problem?
-The list `L` holds the smallest possible ending elements for subsequences of different lengths, ensuring that each subsequence is as small as possible while maintaining the correct order.
What happens when the element in the input array is larger than all elements in `L`?
-If the element is larger than all elements in `L`, it is appended at the end of `L`, extending the longest increasing subsequence found so far.
What happens when the element in the input array is smaller than an element in `L`?
-If the element is smaller than an element in `L`, it replaces that element, ensuring that the subsequence remains as small as possible while maintaining the increasing order.
How does the space complexity of the algorithm compare to the original input size?
-The space complexity is the same as the size of the original input array because the list `L` only stores the smallest possible ending elements of increasing subsequences, and its size is at most the length of the input array.
Why is this algorithm considered efficient?
-This algorithm is efficient because it reduces the problem from a naive O(n^2) solution (dynamic programming with nested loops) to an O(n log n) solution using binary search, making it suitable for larger input sizes.
How can we describe the general strategy for updating the list `L`?
-The general strategy involves using binary search to find the correct position for each element in `L`. If the element is larger than the current maximum, it is appended. If it fits within the existing sequence, it overwrites the appropriate element.
What would be the time complexity if binary search were not used?
-Without binary search, the algorithm would have a time complexity of O(n^2) because each element would need to be compared with all previous elements to find its position, leading to nested iterations.
Outlines
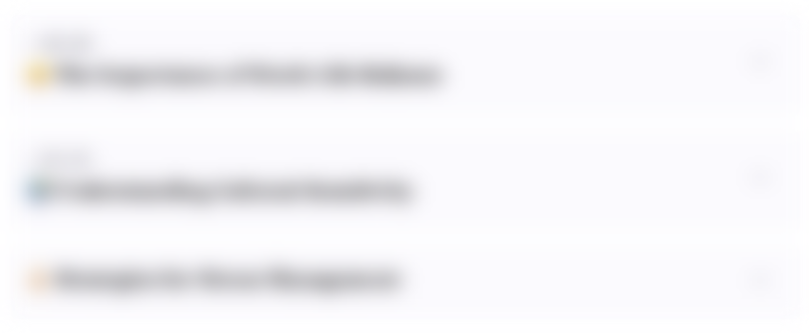
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
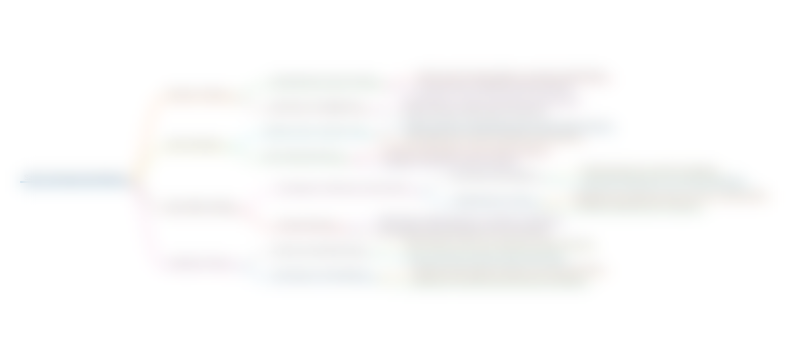
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
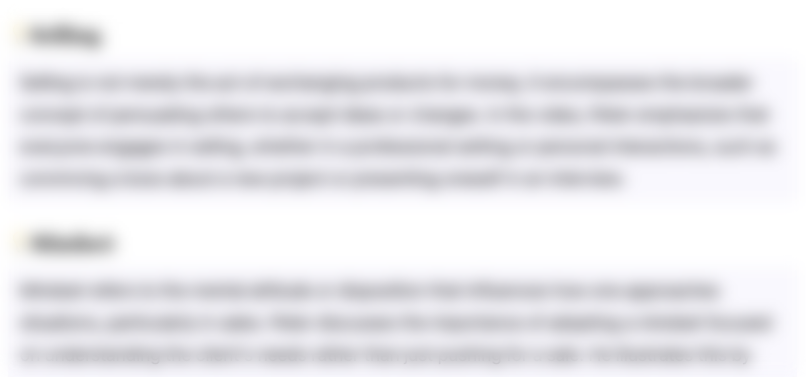
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
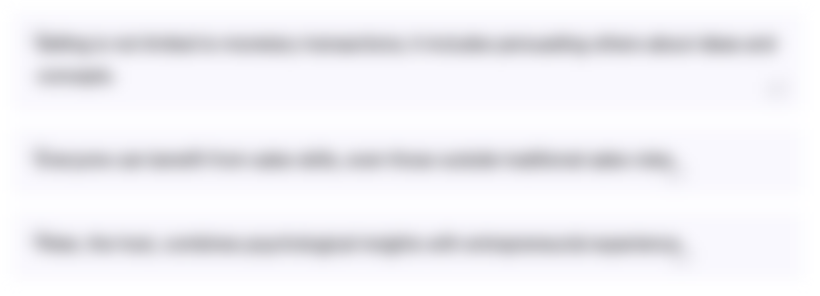
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
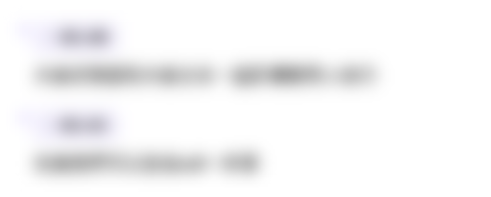
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes

5 steps to solve any Dynamic Programming problem
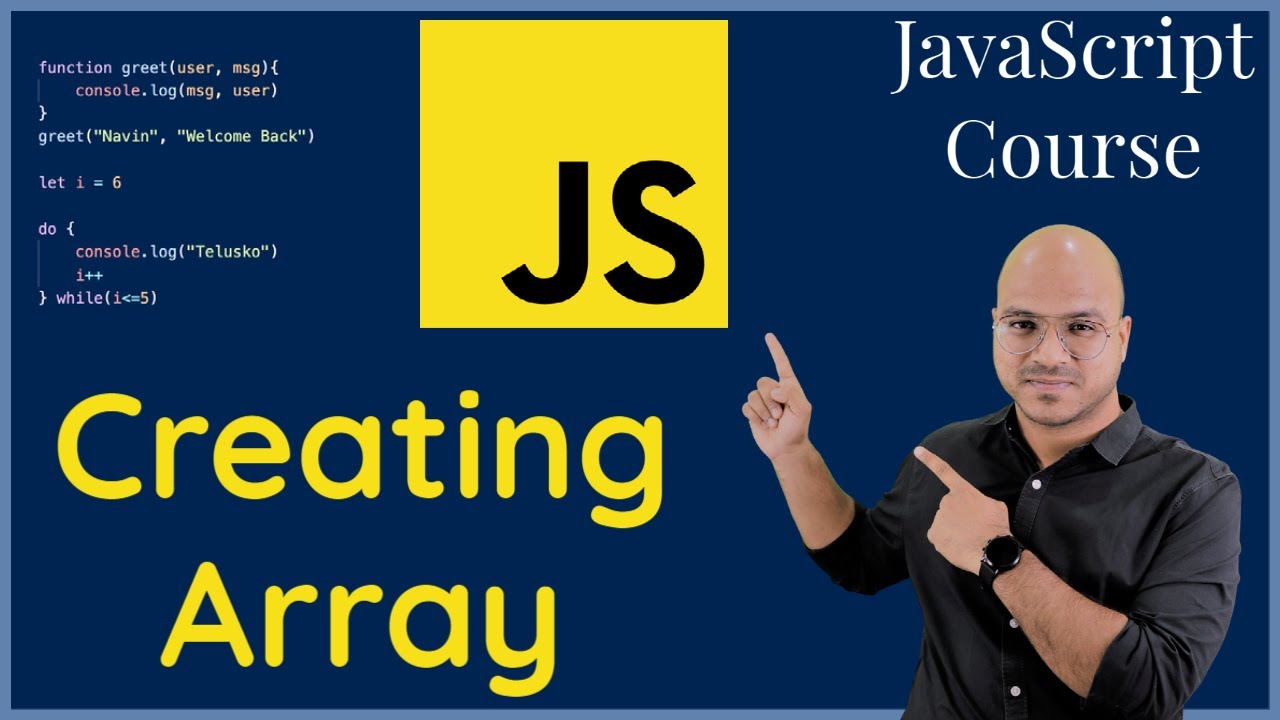
Creating Array and Fetching Elements in JavaScript

Two Sum - Leetcode 1 - HashMap - Python
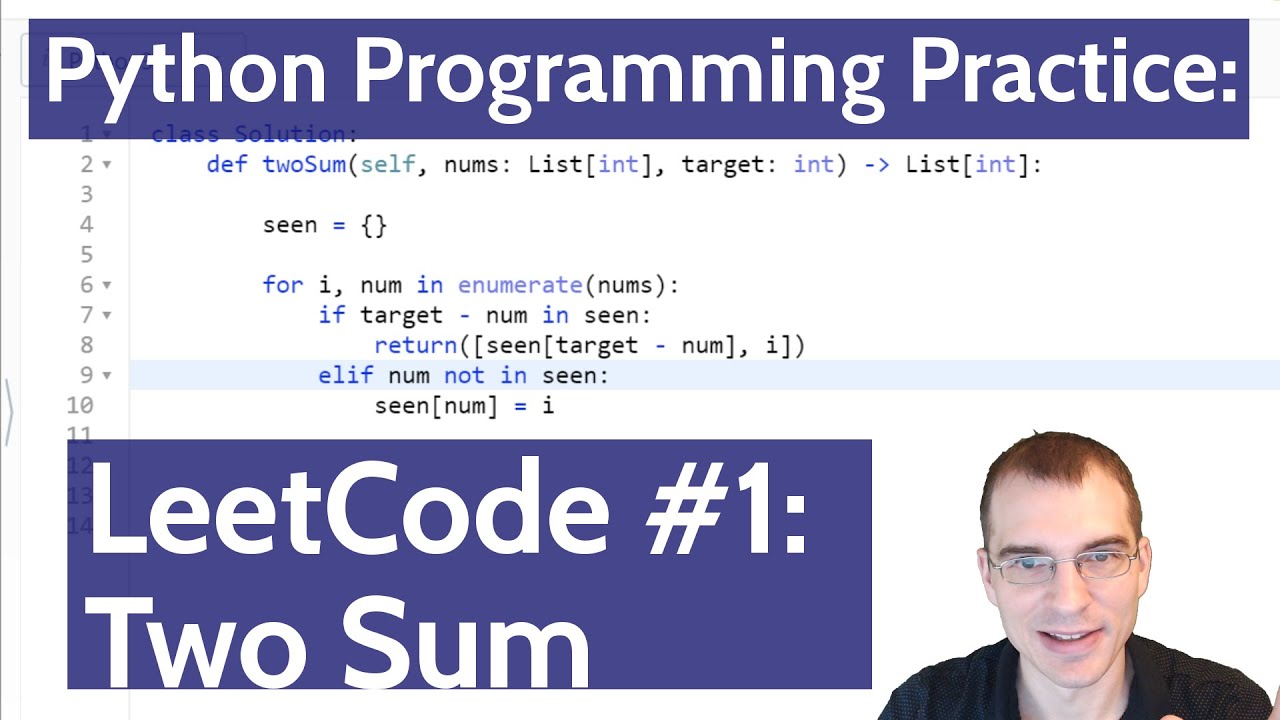
Python Programming Practice: LeetCode #1 -- Two Sum
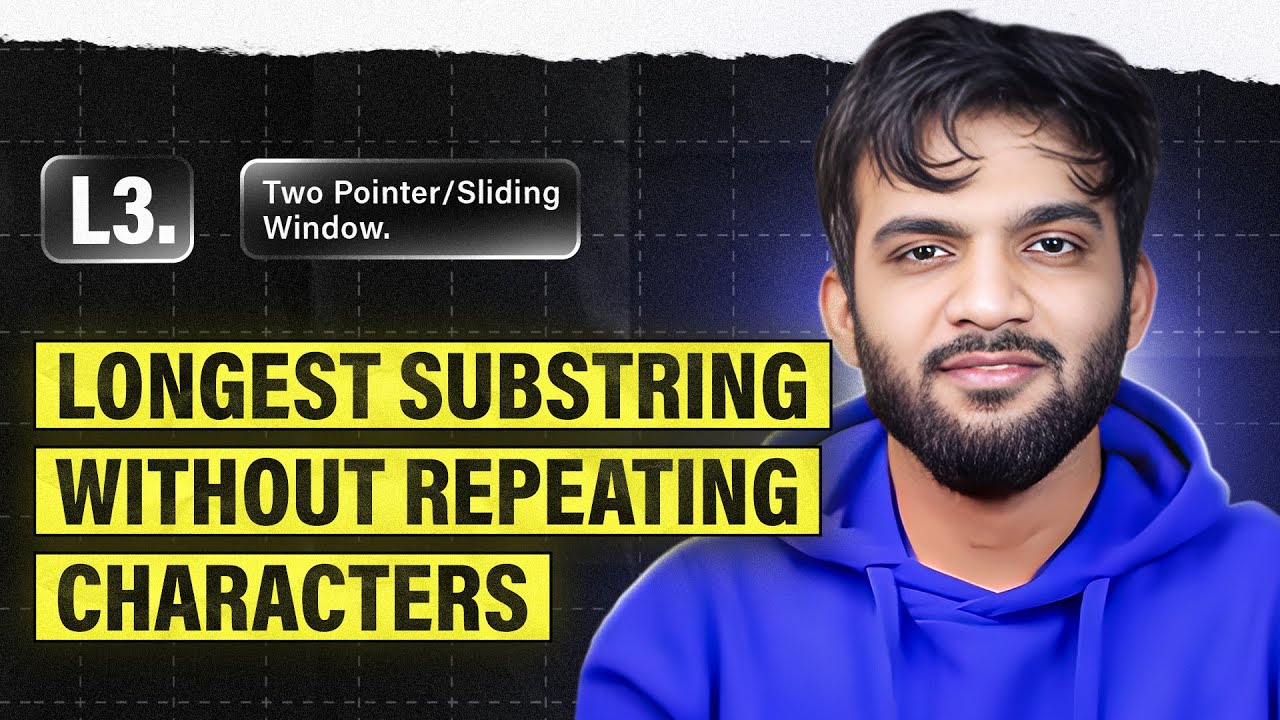
L3. Longest Substring Without Repeating Characters | 2 Pointers and Sliding Window Playlist

8.2 Searching in Arrays | Linear and Binary Search | C++ Placement Course |
5.0 / 5 (0 votes)