Python Programming Practice: LeetCode #1 -- Two Sum
Summary
TLDRIn this Python programming tutorial, the host tackles LeetCode's problem number one, which involves finding two numbers in an array that sum up to a given target. Two solutions are presented: a brute force approach using nested loops, resulting in an O(N^2) time complexity, and a more efficient method utilizing a dictionary to reduce the time complexity to O(N). The video guides viewers through the coding process, explains the logic behind each approach, and demonstrates the significant performance improvement of the dictionary method over the brute force solution.
Takeaways
- 📝 The video is a tutorial on solving LeetCode problem number one, which involves finding two numbers in an array that sum up to a specific target.
- 🔍 The problem is described as having a difficulty level of 'easy' and assures that there is exactly one solution for each input.
- 🚫 The constraints of the problem prohibit using the same element twice and require unique indices for the solution.
- 🔢 An example is provided where the numbers at indices 0 and 1 (2 and 7) add up to the target of 9, thus the solution would return indices [0, 1].
- 🔄 The first solution approach discussed is a brute force method using a double for-loop, which results in a time complexity of O(N^2).
- ⏱ The double for-loop solution is acknowledged to be inefficient for large inputs but is used for demonstration purposes.
- 🔑 The second solution approach uses a dictionary to store numbers and their indices, allowing for a single pass through the array with a time complexity of O(N).
- 📈 The dictionary method is highlighted as more efficient, especially for larger datasets, due to its linear time complexity.
- 💡 The video demonstrates coding both solutions in Python, with a focus on clarity and understanding over optimization.
- 📊 After submitting both solutions, the video compares their performance, showing that the dictionary method is significantly faster.
- 🎓 The video concludes by encouraging viewers to continue coding and seeking out different methods to solve programming problems.
Q & A
What is the main topic of the video?
-The main topic of the video is solving LeetCode problem number one, which involves finding two numbers in an array that sum up to a specific target.
What is the difficulty level of the problem discussed in the video?
-The difficulty level of the problem is labeled as 'easy'.
What is the assumption made about the input array for this problem?
-The assumption made is that each input array will have exactly one solution where two numbers add up to the target sum.
Why is using the same element twice not a concern in this problem?
-Using the same element twice is not a concern because the problem statement guarantees that there will be exactly one solution without needing to use any element more than once.
What is the first solution approach discussed in the video?
-The first solution approach discussed is a brute force method using a double for loop to find all combinations of number additions in the array.
What is the time complexity of the double for loop solution?
-The time complexity of the double for loop solution is O(N^2), where N is the length of the input array.
What is the second solution approach mentioned in the video?
-The second solution approach is using a dictionary to store numbers and their indices as they are encountered, allowing for a single pass through the array.
What is the time complexity of the dictionary-based solution?
-The time complexity of the dictionary-based solution is O(N), where N is the length of the input array.
How does the dictionary-based solution improve efficiency compared to the double for loop?
-The dictionary-based solution improves efficiency by reducing the number of operations from N^2 to N, thus avoiding the need to check every possible pair of numbers in the array.
What is the trade-off when using the dictionary-based solution?
-The trade-off when using the dictionary-based solution is increased memory usage due to storing numbers and their indices in the dictionary.
What is the final verdict on the dictionary-based solution's performance in the video?
-The dictionary-based solution performed significantly faster with a runtime of 48 milliseconds and was in the 95th percentile of speed for Python 3 submissions on the website.
How does the video conclude regarding the solutions to the LeetCode problem?
-The video concludes that while there may be many ways to solve the problem, the dictionary-based solution provided a reasonably efficient method that was faster than most submissions.
Outlines
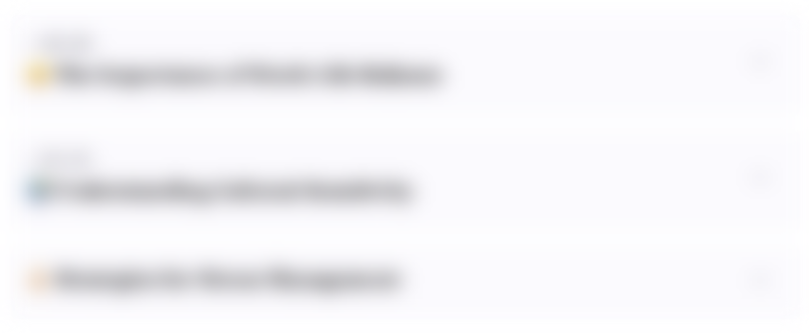
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
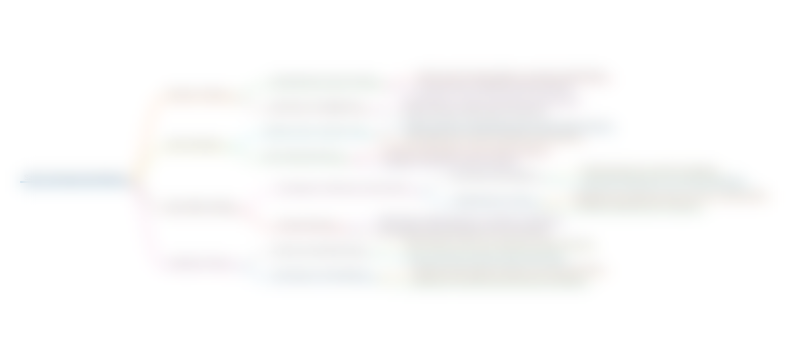
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
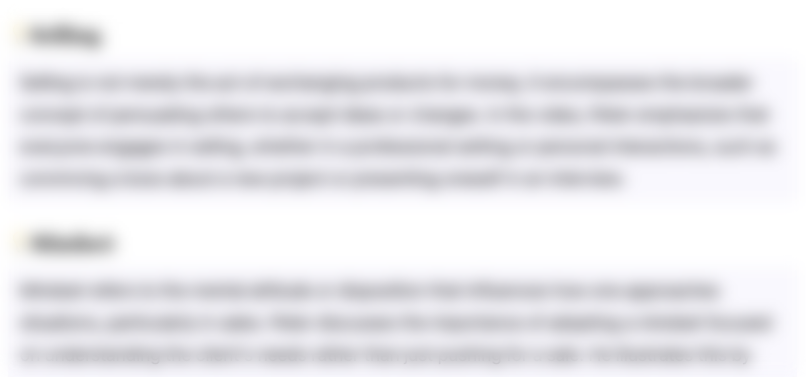
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
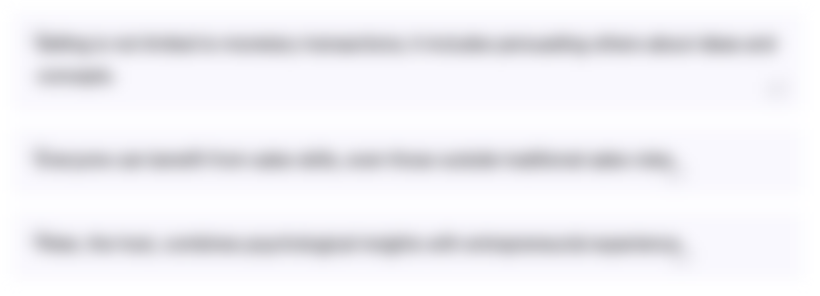
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
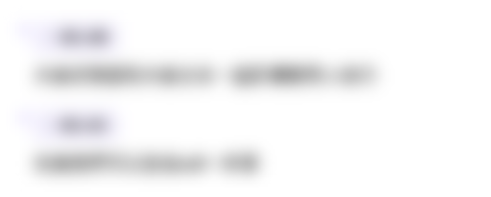
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Solving Problems Involving Quadratic Equations and Rational Algebraic Equations (Part 1)
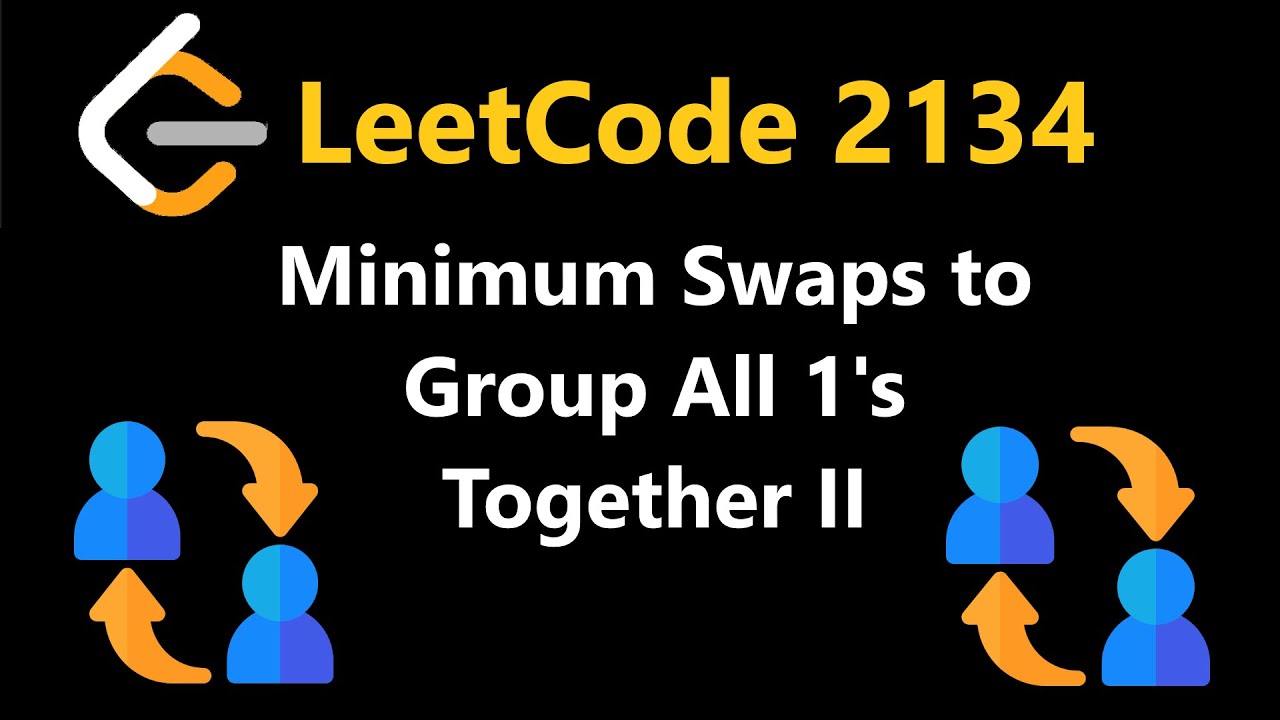
Minimum Swaps to Group All 1's Together II - Leetcode 2134 - Python

Two Sum - Leetcode 1 - HashMap - Python
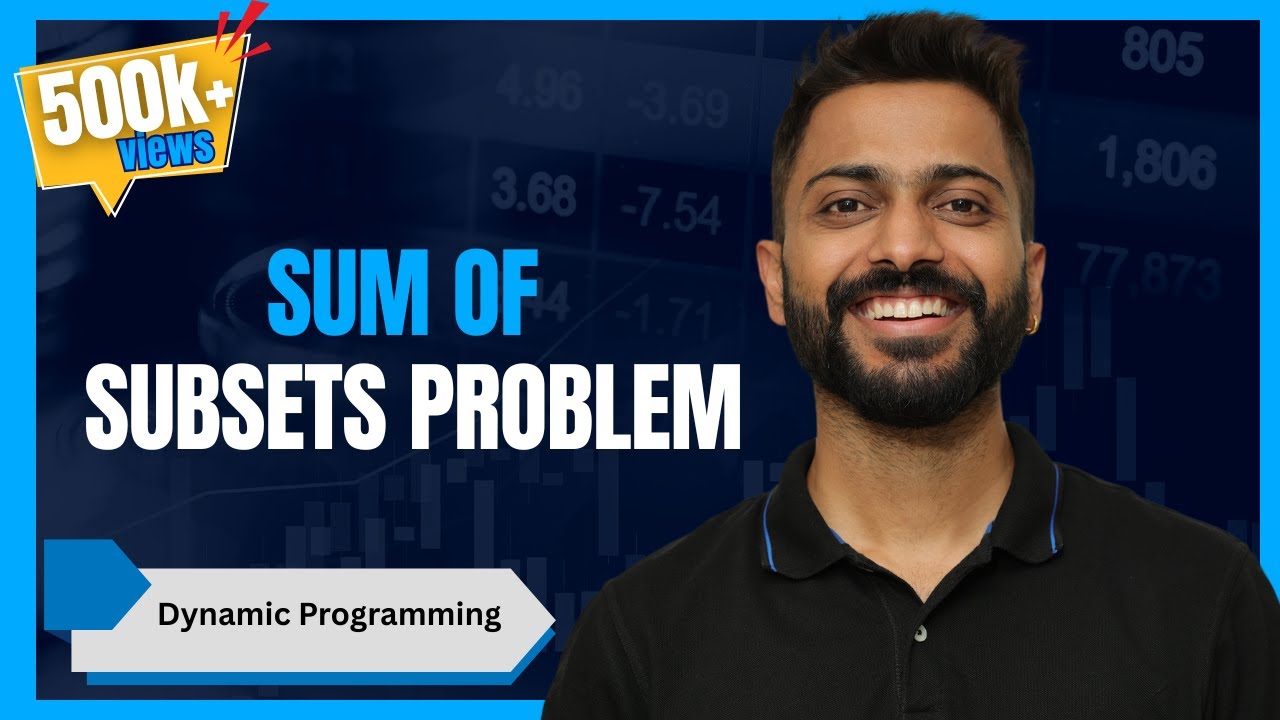
L-5.5: Sum of Subsets Problem | Dynamic Programming
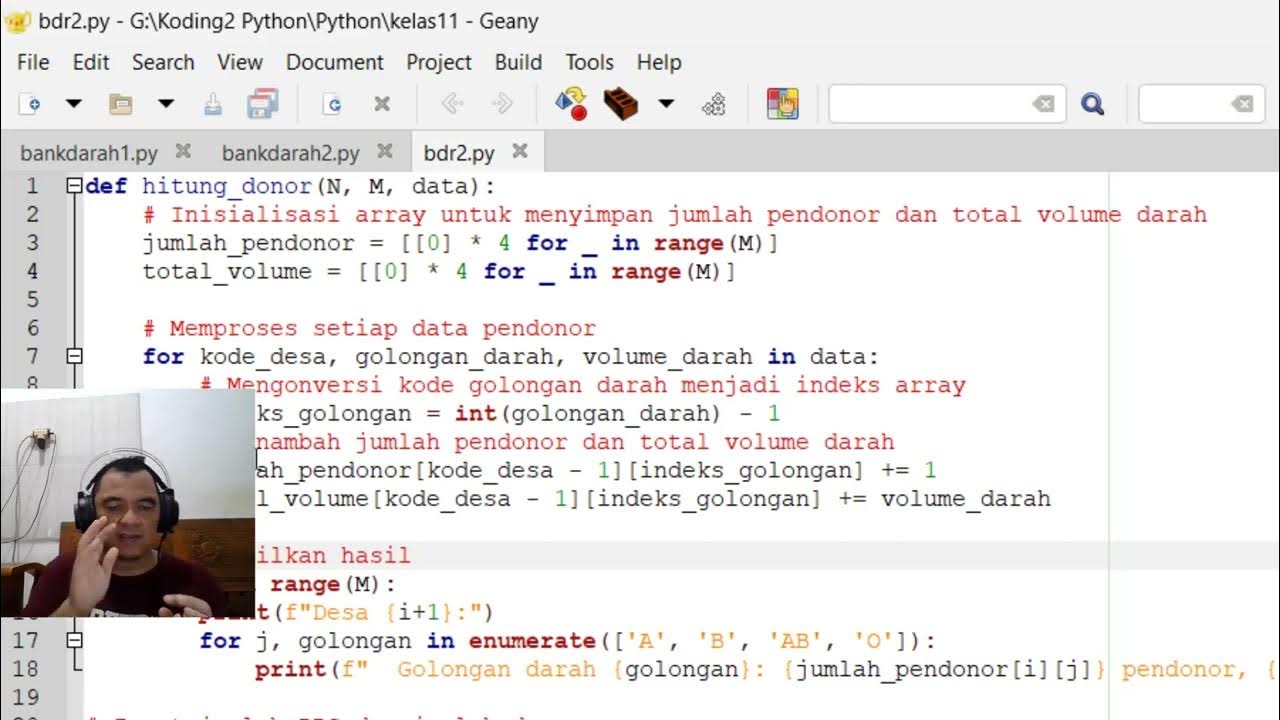
Penyelesaian Problem Bank Darah Kelas 11 [Bag. 2]

Examples of Antenna Array | Solved Examples based on Parameters of Antenna Array | Engineering Funda
5.0 / 5 (0 votes)