Two Sum - Leetcode 1 - HashMap - Python
Summary
TLDRThe script discusses an efficient algorithm to find two values in an array that sum to a target number, such as 9. Initially, it describes a brute force method with a time complexity of O(N^2), then introduces a hash map approach to reduce the complexity to O(N). The hash map stores the indices of array values, allowing for a single pass check to find the complementary value to reach the target sum. The script explains the process and assures that this method will always find a solution due to the guaranteed presence of two summing elements in the array.
Takeaways
- 🔍 The script discusses a coding problem where the goal is to find two values in an array that sum up to a given target, in this case, 9.
- 🔑 The problem guarantees exactly one solution, so there's no need to handle multiple solutions or the absence of a solution.
- 🛠️ The initial approach described is a brute force method, checking every combination of two values, which has a time complexity of O(N^2).
- 🚀 An alternative, more efficient method is introduced using a hash map to reduce the time complexity to O(N).
- 📚 The hash map stores each value from the array with its corresponding index, allowing for constant time lookups.
- 🔄 The script explains that by the time the second value that sums to the target is encountered, the first value must already be in the hash map.
- 💡 It's highlighted that the hash map should not be initialized with the entire array to avoid reusing the same value.
- 🔎 The script provides a step-by-step explanation of how to iterate through the array and use the hash map to find the two values.
- 👩💻 The solution involves checking if the complement of the current value (target minus the value) exists in the hash map before adding the current value to it.
- 📝 The script concludes with a Python code snippet implementing the efficient solution using a hash map.
- 🎯 The final code is demonstrated to work correctly, providing a neat trick to solve the problem in a single pass through the array.
Q & A
What is the primary goal of the algorithm discussed in the script?
-The primary goal of the algorithm is to find two values in an input array that sum up to a given target value, in this case, 9, and return their indices.
What is the initial brute force approach to solving the problem?
-The initial brute force approach involves checking every possible combination of two values in the array to see if they sum up to the target, which results in a time complexity of O(N^2).
Why is the brute force method considered inefficient?
-The brute force method is inefficient because it requires multiple passes through the array for each element, resulting in a worst-case time complexity of O(N^2), where N is the length of the array.
What alternative method is suggested to improve efficiency?
-The alternative method suggested is using a hash map to store the values and their indices from the input array, allowing for constant time checks and a single pass through the array.
How does the hash map help in reducing the time complexity of the algorithm?
-The hash map allows for constant time operations to check if a value exists and to add a new value, reducing the time complexity to O(N) since the array is iterated only once.
What is the memory complexity of using the hash map approach?
-The memory complexity of using the hash map approach is O(N) because it may potentially store every value from the input array.
Why can't the same value be used twice in the solution?
-The same value cannot be used twice because the problem statement guarantees exactly one solution, and reusing the same value would imply multiple solutions or a different combination.
What is the significance of the 'previous map' in the algorithm?
-The 'previous map' stores every element that comes before the current element in the iteration, allowing for the efficient checking of the required difference against the target sum.
How does the algorithm ensure that it finds the correct pair of indices?
-The algorithm ensures the correct pair is found by checking if the difference between the target and the current element exists in the 'previous map'. If it does, it means the pair that sums to the target has been found.
What is the final step in the algorithm once the correct pair is found?
-The final step is to return the indices of the two values that sum to the target. If the difference is not found in the 'previous map', the current value and its index are added to the map, and the iteration continues.
Outlines
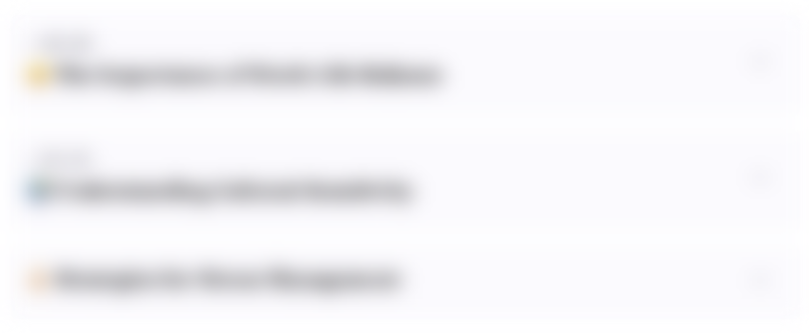
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
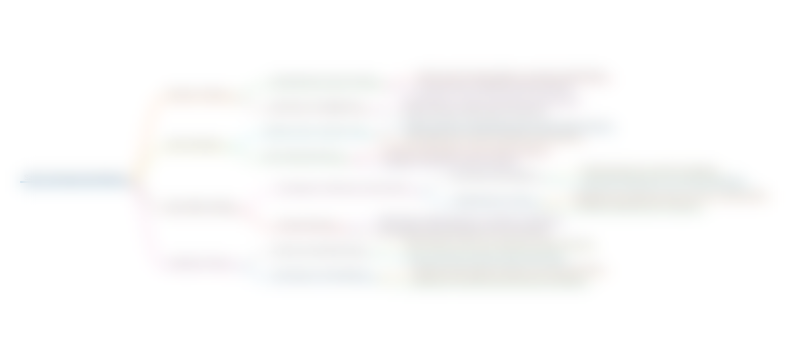
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
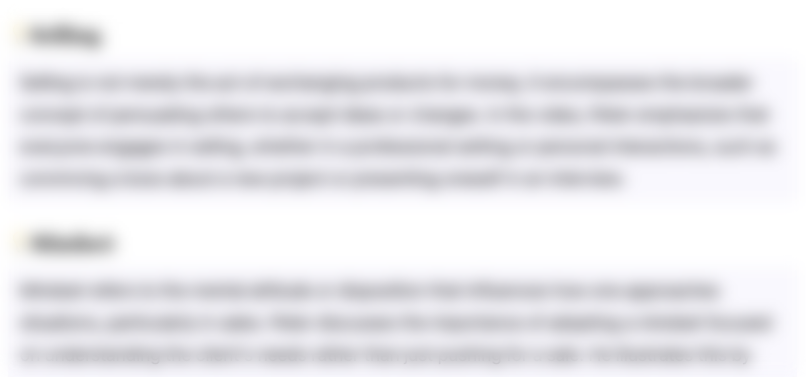
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
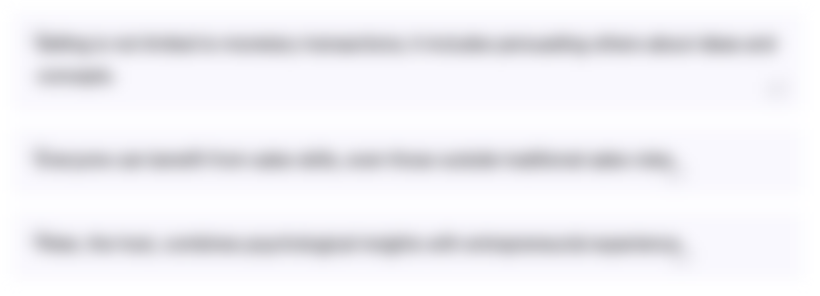
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
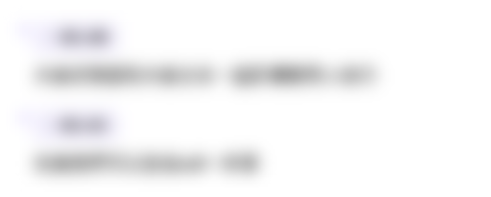
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Lec-10: Two 2️⃣ Pointer👆 Technique | Two 2️⃣ Sum Problem in Data Structure
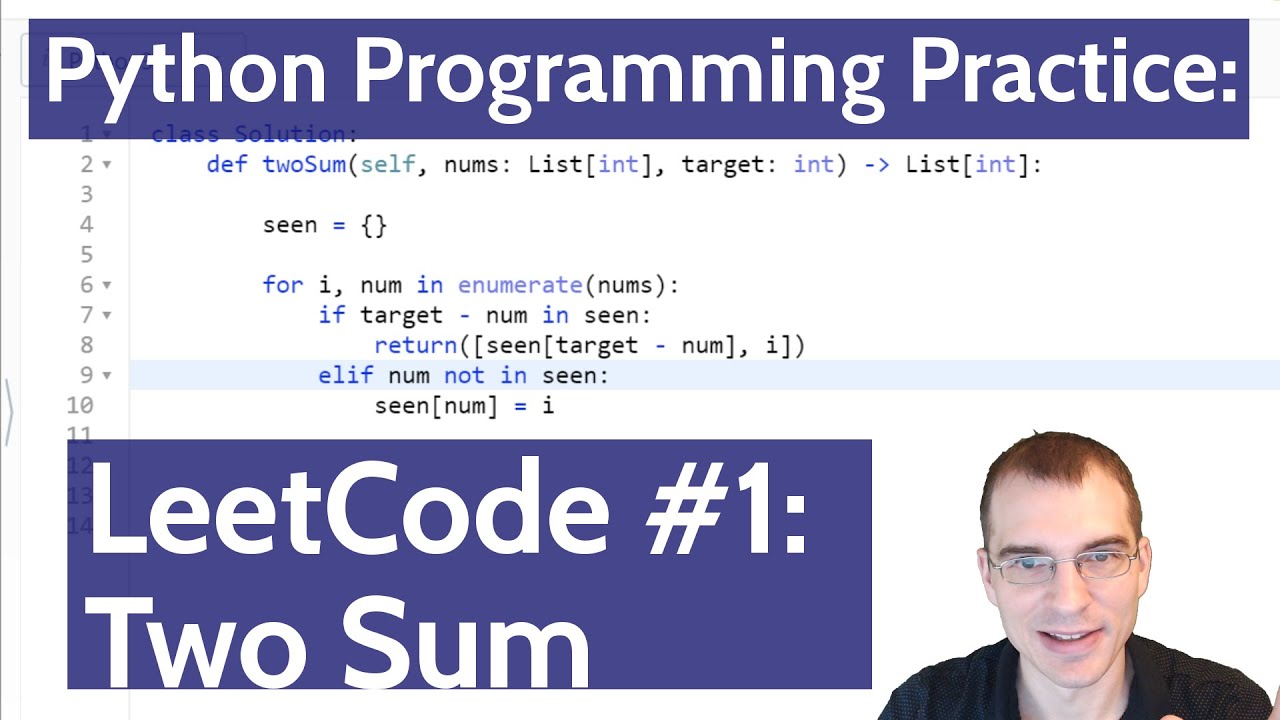
Python Programming Practice: LeetCode #1 -- Two Sum
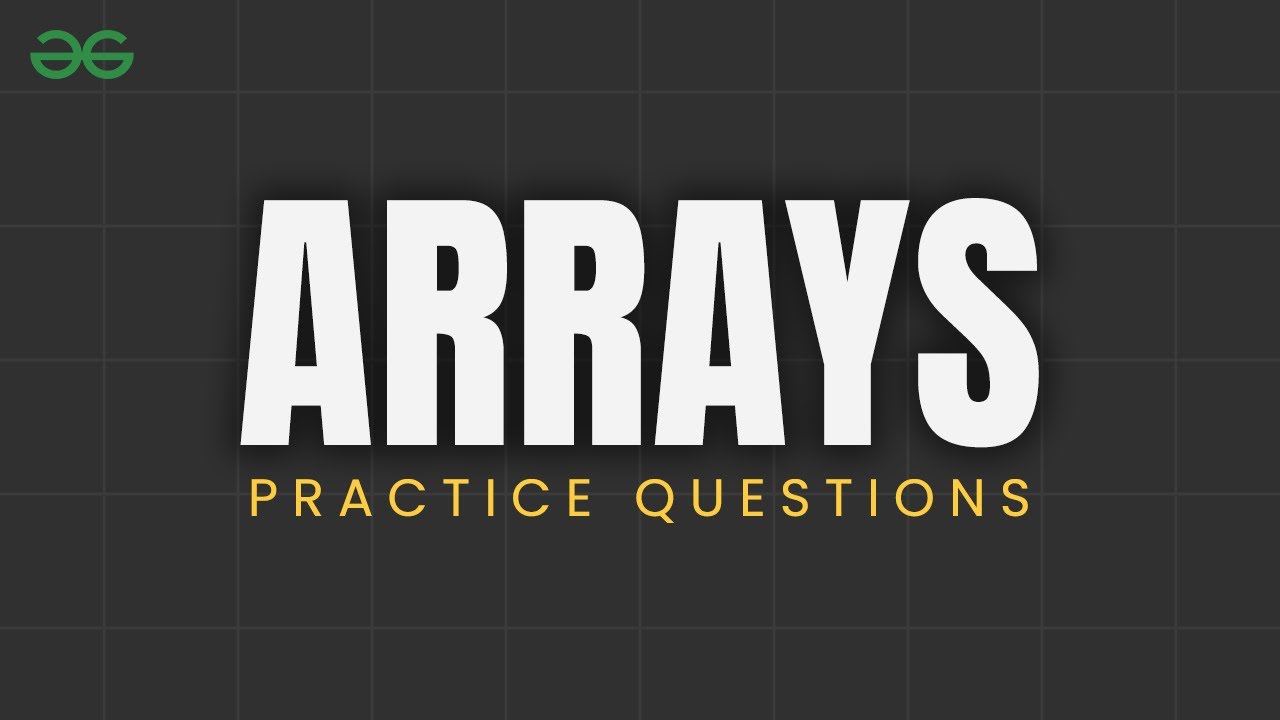
ARRAY PRACTICE PROBLEMS | Must do Array Questions | DSA Problems | GeeksforGeeks
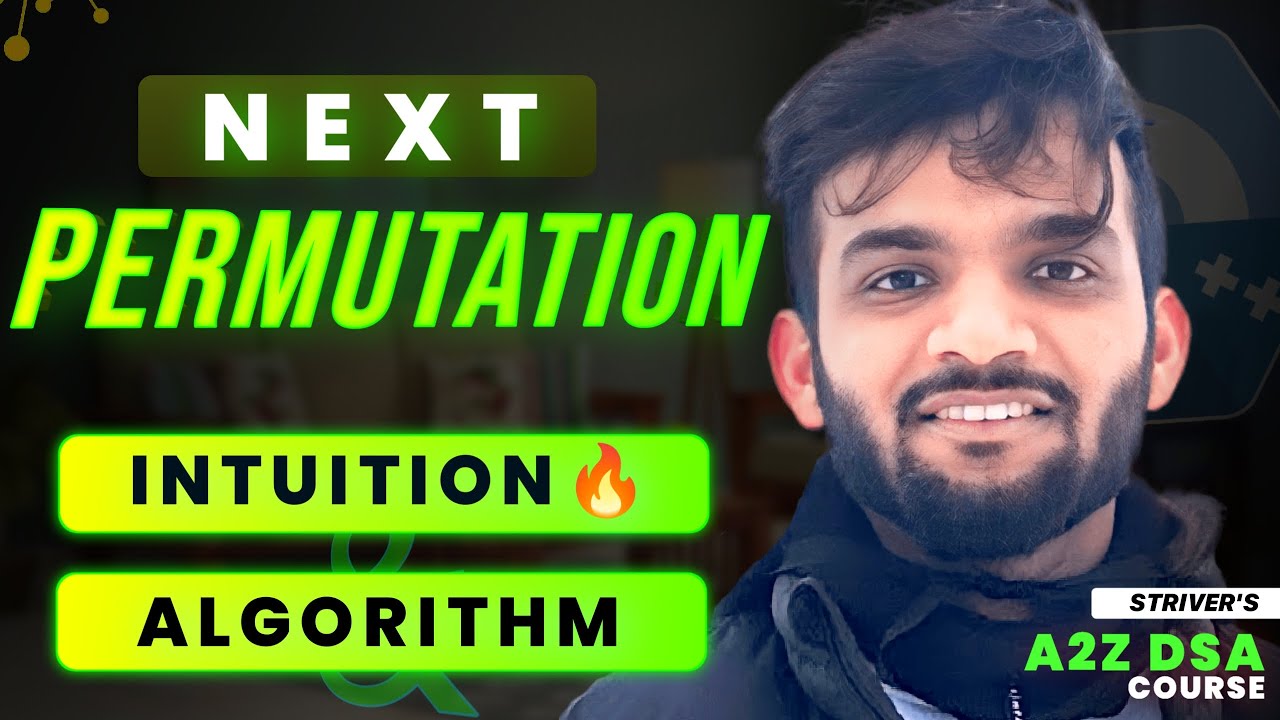
Next Permutation - Intuition in Detail 🔥 | Brute to Optimal
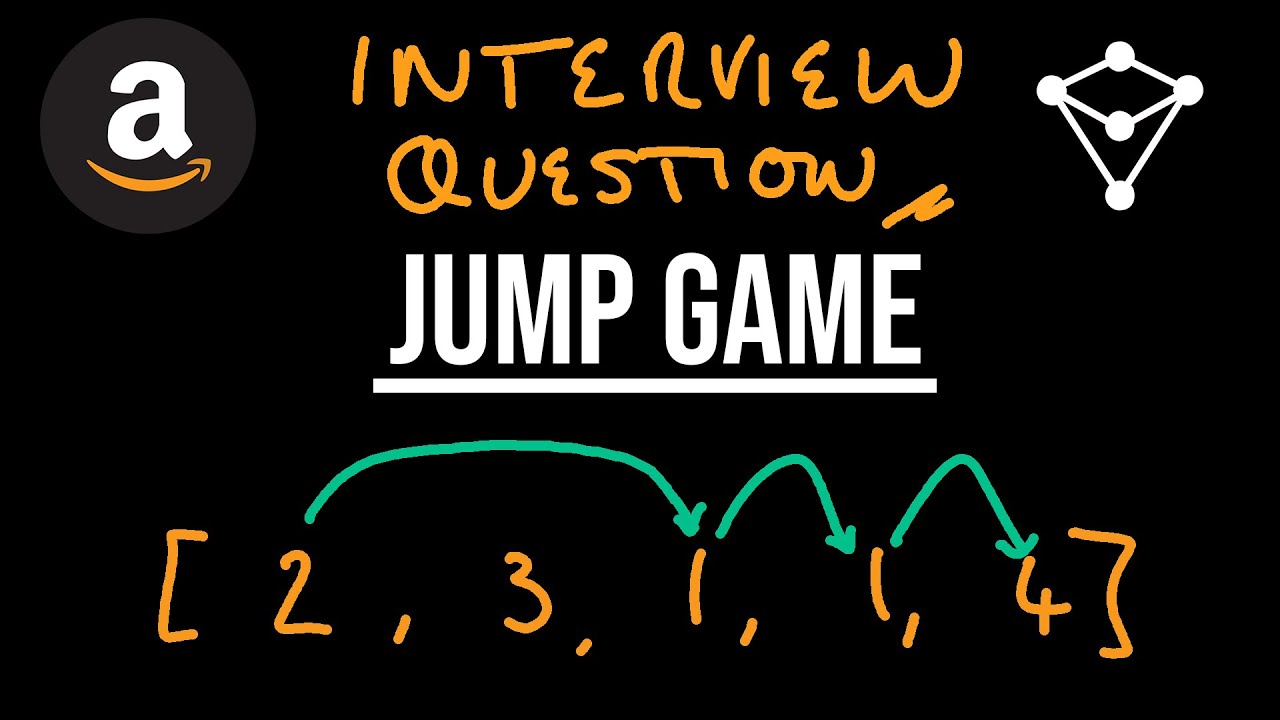
Jump Game - LeetCode 55 - JavaScript
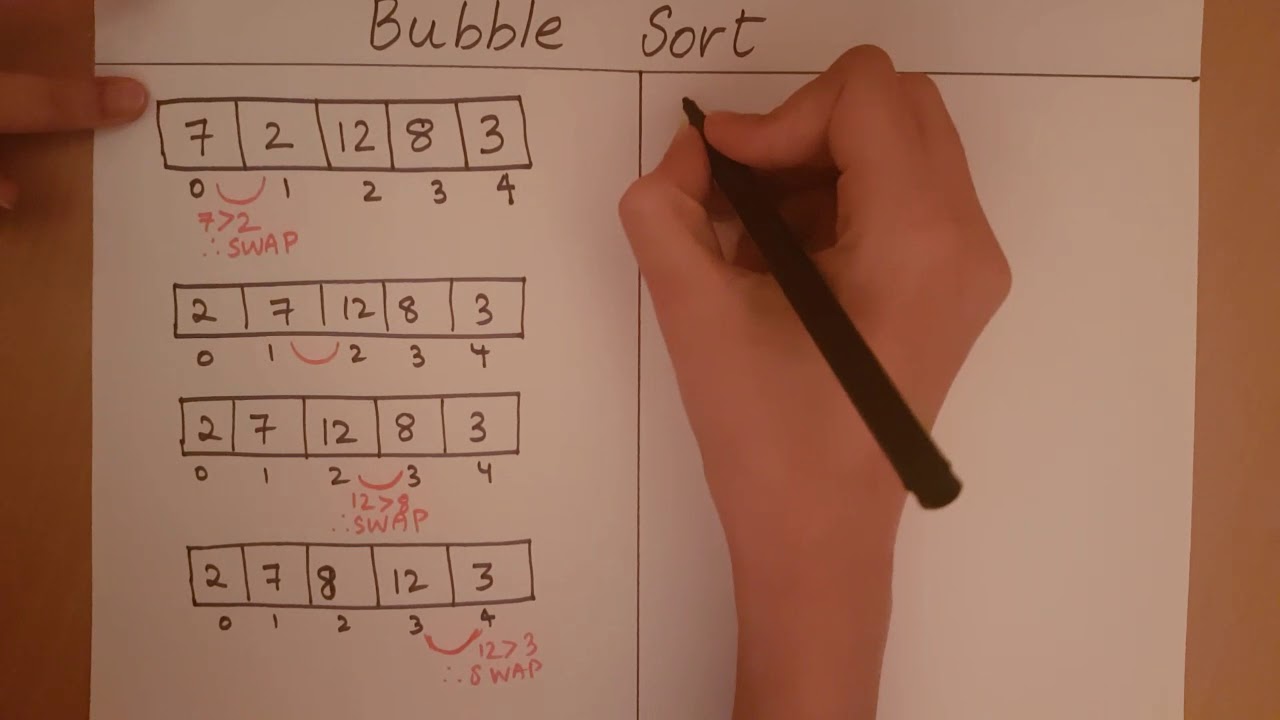
Introduction to Bubble Sort
5.0 / 5 (0 votes)