Belajar Tipe Data Buatan (Record) dalam Bahasa C++ (Kuliah Struktur Data - bag. 2)
Summary
TLDRIn this lecture on data structures, the concept of user-defined data types, or 'records,' is explained using C++. The instructor demonstrates how records group multiple related variables—such as student information (NIM, name, program, IPK)—into a single custom data type using the `struct` keyword. The benefits of using records to streamline code, improve readability, and efficiently manage complex data are highlighted. Examples include declaring and accessing record variables, assigning values, and getting user input. The lecture emphasizes the importance of clear naming conventions for better code organization and understanding.
Takeaways
- 😀 **Understanding Data Types**: In programming languages like C++, data types are categorized into primitive (native) data types (e.g., integer, float, string) and user-defined types.
- 😀 **User-Defined Data Types**: User-defined data types, like records, allow programmers to combine multiple variables into a single entity for efficiency and clarity.
- 😀 **What is a Record?**: A record, also called a struct in C++, is a user-defined data type that holds multiple variables of different types, grouped together under a single name.
- 😀 **Record Declaration**: In C++, records are declared using the `struct` keyword, followed by the fields (variables) it will contain, each with a specific data type.
- 😀 **Example of a Record**: A `Mahasiswa` (student) record can store fields such as `NIM`, `name`, `program of study`, and `IPK` (GPA).
- 😀 **Efficiency with Records**: By using records, related data like a student’s name, NIM, and GPA can be stored in one place instead of creating multiple individual variables.
- 😀 **Accessing Record Elements**: To access or modify the fields within a record, the dot notation (`.`) is used (e.g., `mhs1.NIM = 12345;`).
- 😀 **Record Fields and User Input**: Record fields can be filled either by hardcoding values or asking the user to input them using `cin` (e.g., `cin >> mhs1.nama;`).
- 😀 **Benefits of Using Records**: Records make the code more organized and manageable, especially when handling complex data like student or book information.
- 😀 **Variable Naming and Record Types**: It is important to name variables and record types logically, so the code remains readable and easy to maintain (e.g., `Mahasiswa` for a student record).
Q & A
What is the main topic of the lecture in the transcript?
-The main topic of the lecture is 'Data Structures,' specifically focusing on custom or user-defined data types, using C++ as the programming language for implementation.
What are the two categories of data types discussed in the lecture?
-The two categories of data types discussed are 'primitive data types' (such as integer, float, double, string, and boolean) and 'user-defined data types' (such as records).
What is a 'record' in the context of programming?
-A 'record' is a user-defined data type that groups together multiple variables, each with its own primitive data type, into one cohesive structure. It allows us to combine related data into a single unit.
How do you declare a record in C++?
-In C++, a record is declared using the 'struct' keyword, followed by the record name and the variables (with their respective data types) that it contains. For example, 'struct Mahasiswa { int NIM; string name; string Prodi; float IPK; };' is a declaration for a 'Mahasiswa' record.
Why would you use a record to store data like student information?
-A record is useful for grouping related data, such as student information (NIM, name, program of study, IPK), into a single unit. This makes the program more efficient and manageable by avoiding the need to create multiple separate variables for each piece of data.
Can you provide an example of declaring and using a record in C++?
-Sure! In C++, to declare a record, you might write: 'struct Mahasiswa { int NIM; string name; string Prodi; float IPK; };'. Then, you can create a variable 'Mahasiswa mhs1;' and access its fields with dot notation, such as 'mhs1.NIM' or 'mhs1.name'.
How can you assign values to the fields of a record in C++?
-You can assign values to the fields of a record by using dot notation. For example, 'mhs1.NIM = 112233;' and 'mhs1.name = 'John';'. Alternatively, you can use 'cin' to get user input for the fields, like 'cin >> mhs1.name;'
What is the advantage of using records in programming?
-The main advantage of using records is that they allow related data to be grouped together, making the code more efficient and easier to manage. Instead of declaring multiple separate variables, you can bundle them into one structure, simplifying the code.
In the lecture, what is the example of a second record type used, and what does it represent?
-Another example of a record type given in the lecture is 'Buku' (Book). This record contains information such as title, author, publisher, price, ISBN, and page count, all of which are grouped together to represent a book's data.
How do you access the individual elements of a record in C++?
-To access individual elements of a record in C++, you use dot notation. For example, 'mhs1.NIM' will access the 'NIM' field of the 'mhs1' record, and 'mhs2.IPK' will access the 'IPK' field of the 'mhs2' record.
Outlines
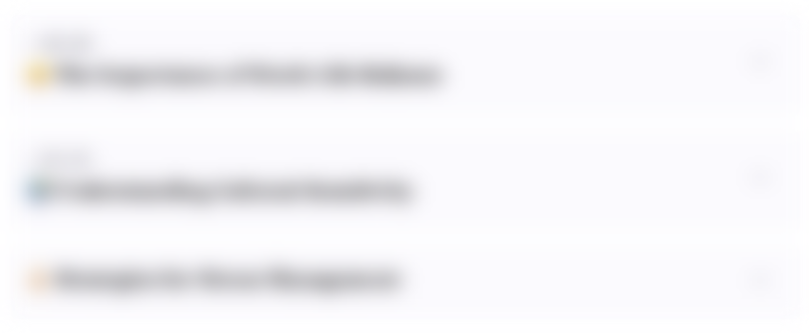
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
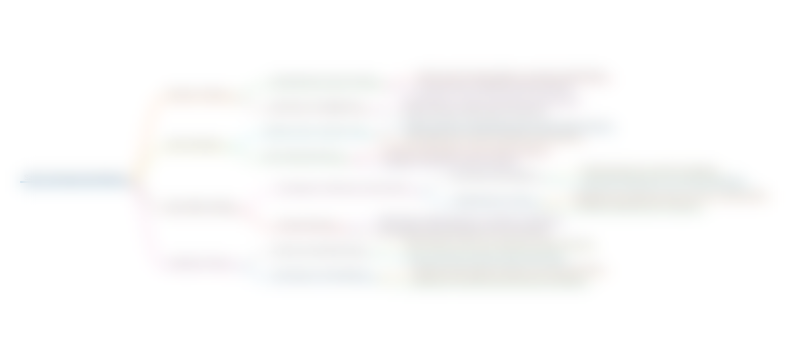
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
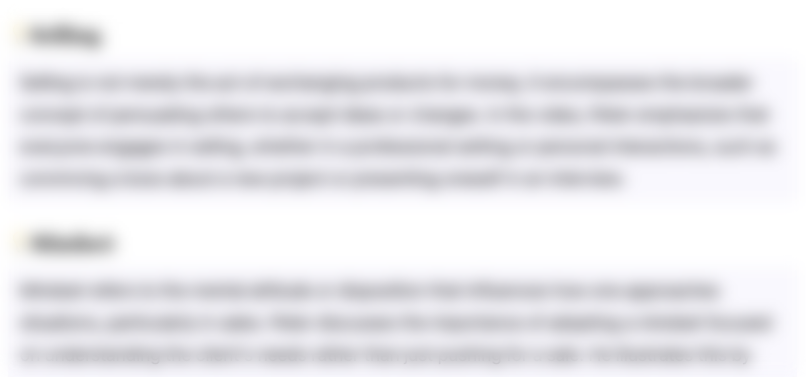
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
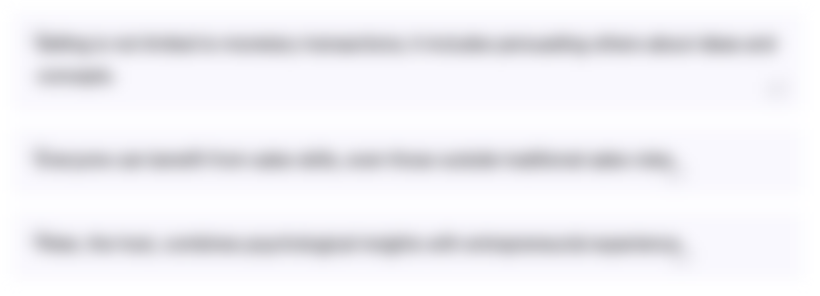
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
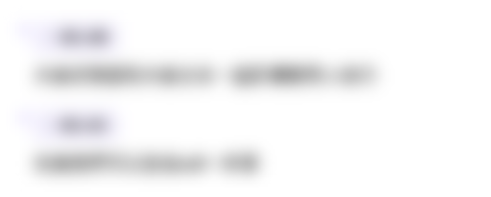
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
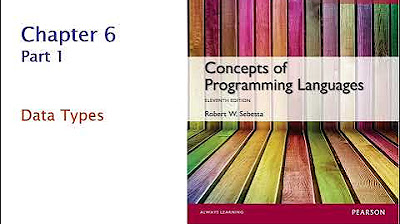
COS 333: Chapter 6, Part 1

C_09 Data Types in C Language | C Programming Tutorials
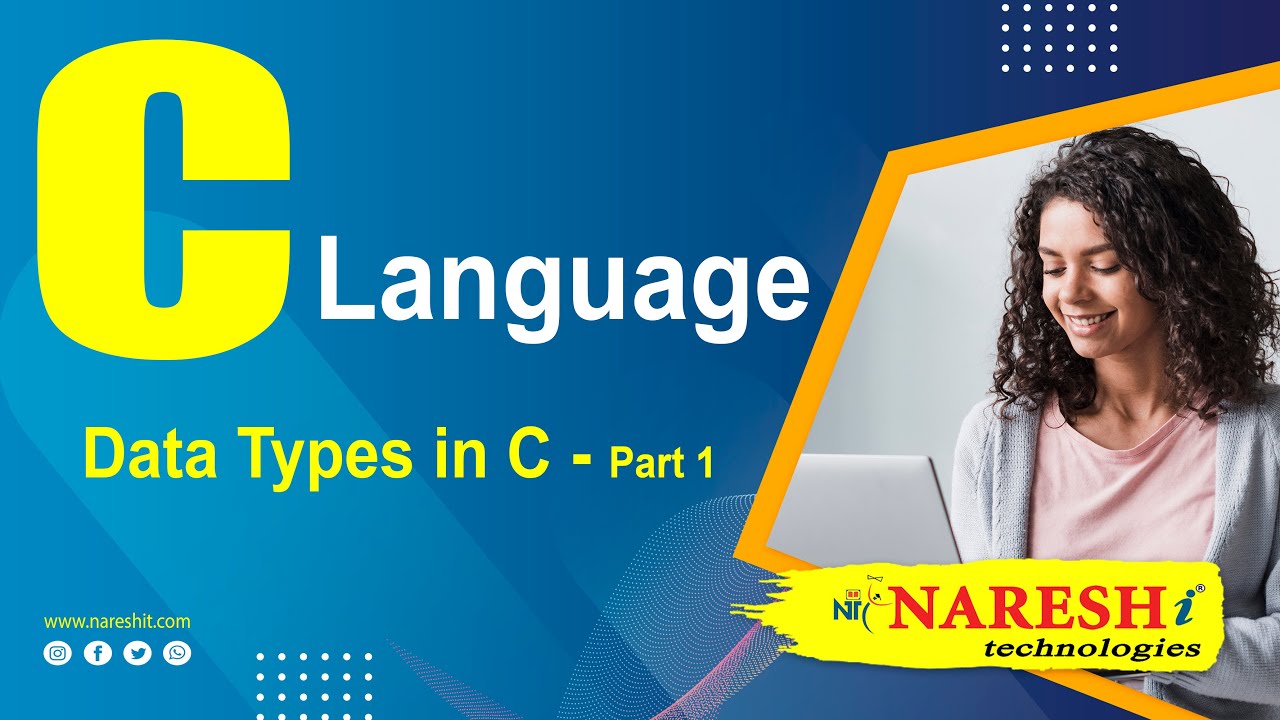
Data Types in C - Part 1 | C Language Tutorial

Structure dalam Mata Kuliah Struktur Data dan Algoritma

COS 333: Chapter 11, Part 1
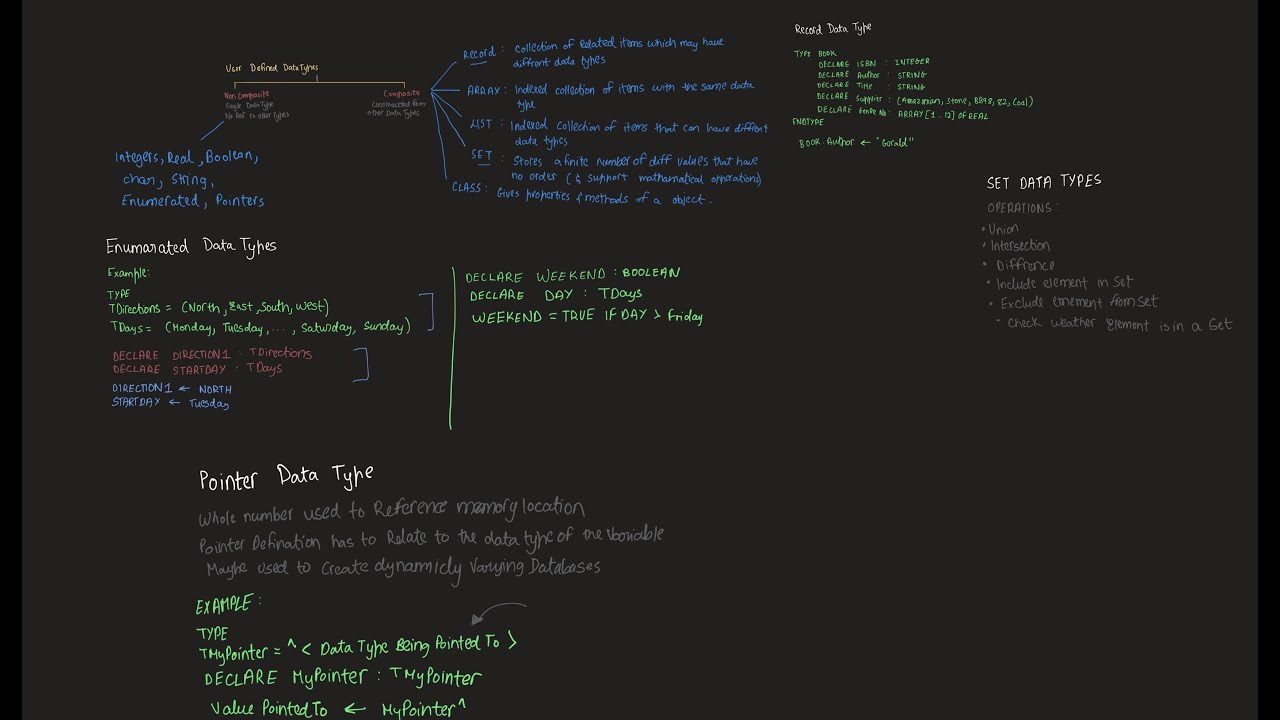
Data Representation - User Defined Data Types Part 1 - (A Level Computer Science Made Easy (A2) )
5.0 / 5 (0 votes)