Functional Programming (Part 3)
Summary
TLDRThis lecture is the third and final part of functional programming concepts, focusing on key functions in Python such as lambda, enumerate, zip, map, and filter. The instructor explains how lambda functions can simplify code by allowing anonymous, one-line functions. The enumerate function pairs list items with their indices, while the zip function couples values from multiple lists. The map function applies operations to list elements without loops, and the filter function selectively processes list elements. These concepts streamline programming tasks and enhance efficiency in Python.
Takeaways
- 😀 Lambda functions in Python allow for anonymous, one-line functions without naming them.
- 💻 Lambda functions help simplify the code by replacing traditional functions when only a single statement is involved.
- 📜 Enumerate function in Python couples the index and value of a list, making it easier to keep track of list items and their positions.
- 🔗 The zip function combines values from two lists into pairs, making it easy to work with related data from multiple lists.
- ➕ The map function applies a specified operation to elements of multiple lists simultaneously, automating repetitive tasks.
- 🔄 Map function can be used to apply a function to every element of a list without writing explicit loops.
- 🚫 The filter function helps filter out unwanted values from a list before applying operations like map.
- 🧮 The filter function is useful in skipping invalid or unwanted values, such as negative numbers when computing square roots.
- ⚡ Lambda, map, filter, and zip functions are powerful tools in Python's functional programming to optimize code length and performance.
- 🎓 Functional programming in Python helps in simplifying complex code structures by focusing on mapping, filtering, and reducing repetitive tasks.
Q & A
What is a lambda function in Python?
-A lambda function in Python is an anonymous function that can be defined without a function name. It is typically used for small, single-line functions and can be assigned to variables for reuse.
Why might lambda functions be preferred over regular functions in some cases?
-Lambda functions are often preferred when the function contains only one line or a simple operation. They help reduce the length of the code and eliminate the need for writing separate function definitions.
How do you define a simple addition lambda function in Python?
-You can define a simple addition lambda function as follows: `add = lambda x, y: x + y`. This creates an anonymous function that takes two parameters, `x` and `y`, and returns their sum.
What is the purpose of the `enumerate` function in Python?
-The `enumerate` function in Python couples the elements of an iterable (like a list) with their corresponding index values. This allows you to iterate through both the index and the value simultaneously.
What problem does `enumerate` solve when working with lists?
-The `enumerate` function helps when you need to keep track of the index of an element in a list. Without `enumerate`, you would have to manually manage indices, which can become error-prone, especially with large lists.
What is the `zip` function used for in Python?
-The `zip` function is used to combine elements from two or more iterables (such as lists) into pairs or tuples. It creates a new iterable where the elements from the input iterables are paired together.
How would you use the `zip` function to combine two lists?
-To combine two lists `fruits` and `size` using `zip`, you would write: `zip(fruits, size)`. This pairs each element from `fruits` with the corresponding element in `size`.
What is the purpose of the `map` function in Python?
-The `map` function applies a given function to each item in one or more iterables (such as lists). It returns a map object that can be converted to a list or another collection type.
Can you explain how the `map` function works with an example?
-For example, if you have two lists `a = [10, 20]` and `b = [5, 10]`, and you want to subtract `b` from `a` element-wise, you can define a function `subtract(x, y)` and use `map(subtract, a, b)`. This will apply the `subtract` function to each corresponding pair of elements from `a` and `b`.
What is the difference between `map` and `filter` in Python?
-The `map` function applies a function to all items in an iterable, while `filter` applies a function to each item but only includes those that meet a certain condition (i.e., for which the function returns `True`).
How would you use `filter` to remove negative numbers from a list?
-You can use `filter` with a custom function like `is_positive = lambda x: x >= 0` to remove negative numbers from a list. For example, `filter(is_positive, [-10, 25, -3, 8])` would return only `[25, 8]`.
Why might you use both `map` and `filter` together?
-You might use `map` and `filter` together when you need to first filter the elements of a list based on a condition and then apply a function to the filtered elements. This allows for efficient processing in one line of code.
Outlines
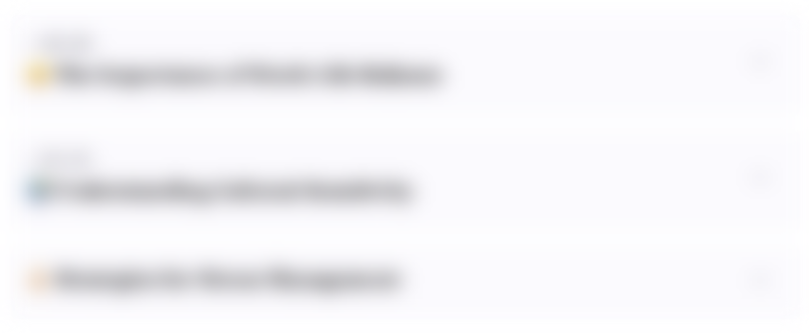
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
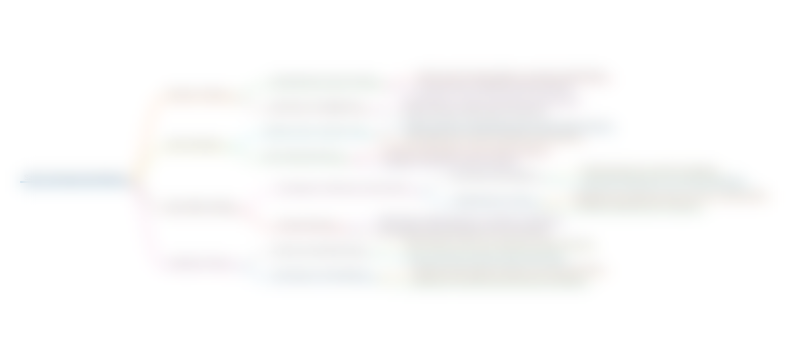
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
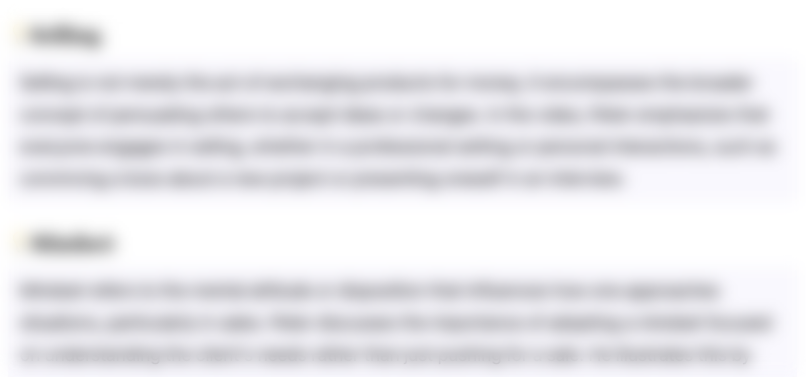
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
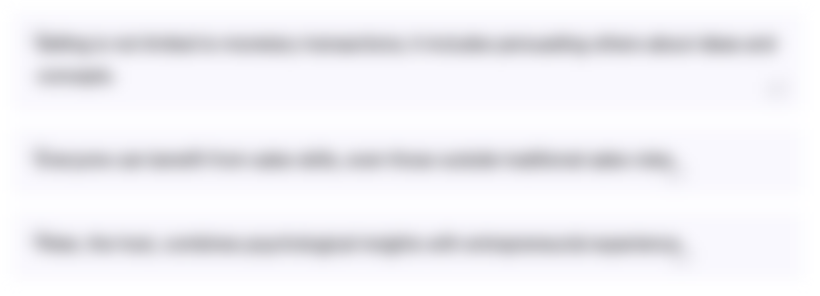
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
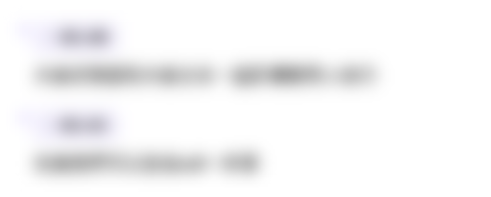
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
5.0 / 5 (0 votes)