ReactJS Tutorial - 8 - JSX
Summary
TLDRThis video introduces JSX, a JavaScript extension widely used in React to simplify coding by allowing developers to write XML-like syntax. While JSX is not required, it makes React code cleaner and easier to read. The tutorial demonstrates how JSX translates into JavaScript using React's `createElement` method, showing the differences between coding with and without JSX. Key differences between JSX and HTML, like using `className` instead of `class`, are discussed, along with potential future changes in React. The video emphasizes JSX's ability to streamline complex code.
Takeaways
- 📜 JSX stands for JavaScript XML and is an extension to JavaScript syntax, used with React for writing XML-like code for components.
- 🛠 JSX is not mandatory for writing React applications, but it simplifies the process and makes the code more elegant and readable.
- ⚙️ JSX ultimately compiles into regular JavaScript that browsers can understand.
- 📂 JSX code can be written in a simpler format compared to using React’s `createElement` method directly.
- 🧑💻 React’s `createElement` method takes three parameters: the HTML tag, an object for properties, and the children elements.
- 🔧 JSX elements are syntactic sugar for calling `React.createElement`, simplifying component creation with a more familiar syntax.
- 🔠 Some differences exist between JSX and HTML, such as using `className` instead of `class` and `htmlFor` instead of `for` due to JavaScript keyword restrictions.
- ⚛️ Even when JSX is not explicitly seen in the code, React must be imported because JSX is translated into `React.createElement` calls.
- 🔄 The React team may introduce breaking changes in future versions, including reverting `className` back to `class`.
- 📅 Developers should stay updated on upcoming React changes by reviewing the latest documentation, especially with React 18 and 19 on the horizon.
Q & A
What is JSX?
-JSX stands for JavaScript XML and is an extension to the JavaScript syntax used with the React library. It allows developers to write XML-like code for elements and components in React.
Why is JSX commonly used in React?
-JSX simplifies the process of writing React components by making the code more elegant and readable. Although JSX is not a necessity, it provides a familiar syntax for developers and ultimately translates to pure JavaScript that browsers understand.
How does JSX work behind the scenes?
-Behind the scenes, JSX is transformed into `React.createElement()` calls. Each JSX element is syntactic sugar for this method, which constructs the virtual DOM nodes that React uses to efficiently update the browser’s DOM.
Can React be used without JSX?
-Yes, React can be used without JSX. Developers can use `React.createElement()` to create elements and components, but JSX makes the code cleaner and easier to write, especially when working with multiple elements.
How does the `React.createElement()` method work?
-The `React.createElement()` method takes three parameters: the HTML tag (e.g., 'div'), an object of properties (can be `null` if there are no additional properties), and the children (contents) of the element.
What are some key differences between JSX and regular HTML?
-In JSX, certain attributes differ from HTML. For instance, `class` is replaced by `className` because 'class' is a reserved keyword in JavaScript. Similarly, `for` becomes `htmlFor`, and properties like `onClick` are camel-cased (e.g., `onClick` instead of `onclick`).
Why do we need to import React even if we don't see it explicitly being used?
-When JSX is used, it is converted into `React.createElement()` calls. These calls rely on the React library, which is why `import React` is necessary in files that use JSX, even if React isn't directly referenced.
How does JSX improve the code compared to using `React.createElement()` directly?
-JSX significantly improves code readability and simplicity. For components with many elements, JSX is less verbose and more intuitive, while directly using `React.createElement()` can make the code cumbersome and harder to maintain.
What happens if we need to add attributes to an element in `React.createElement()`?
-The second parameter of `React.createElement()` is an object of key-value pairs that represents the attributes. For example, to add an ID attribute to a `div`, we would pass `{ id: 'hello' }` as the second argument.
What future changes related to JSX are mentioned in the video?
-The video mentions that the React team is working on breaking changes that may impact JSX. One notable change is replacing `className` with `class`. These changes are targeted for future versions of React (React 18 or 19).
Outlines
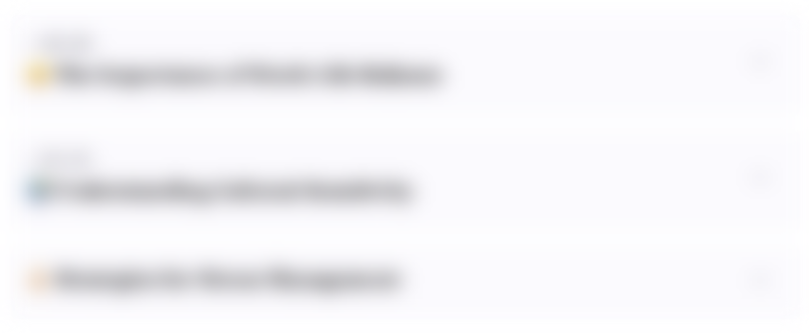
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
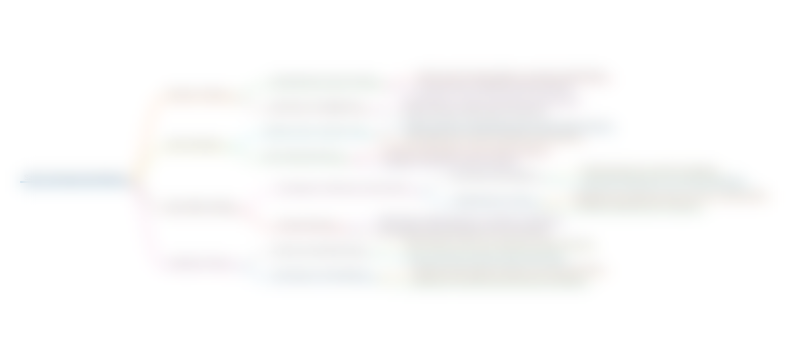
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
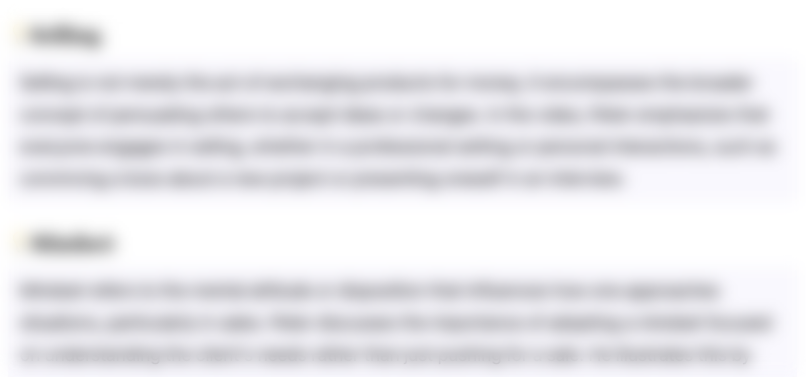
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
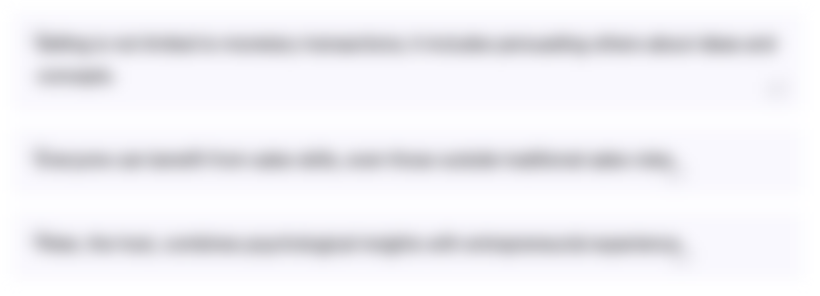
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
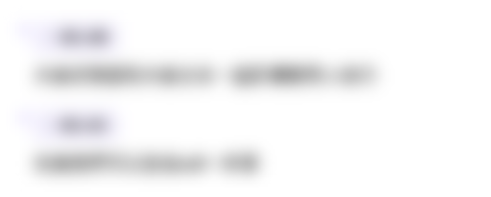
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenant5.0 / 5 (0 votes)