React tutorial for beginners ⚛️
Summary
TLDRThis video tutorial offers an introduction to ReactJS, a popular JavaScript library for building user interfaces. It explains that React uses components, similar to Lego blocks, to construct web applications. The video covers the basics of JSX, a syntax extension that allows HTML-like code within JavaScript files, and the concept of the virtual DOM for efficient rendering. The tutorial guides viewers through installing Node.js, setting up a React project, and creating simple components like Header, Footer, and a Food list. It also touches on project structure, including the roles of 'node_modules', 'public', and 'src' folders, and demonstrates how to use and reuse components within a React application.
Takeaways
- 😀 React is a JavaScript library for building user interfaces, not a framework.
- 🔧 Components in React are reusable sections of code, akin to Legos, that can be assembled to form complex web applications.
- 📝 JSX, or JavaScript XML, is a syntax extension that allows writing HTML-like code within JavaScript files.
- 🌐 The Virtual DOM in React is a lightweight representation of the real DOM, enabling efficient updates by only applying changes to the real DOM when necessary.
- 🛠️ Prerequisites for starting with React include knowledge of JavaScript, HTML, and CSS, as well as familiarity with ES6 features like arrow functions.
- 💻 Node.js is required for React development, providing a backend JavaScript runtime environment and the Node Package Manager (npm).
- 📂 The project structure of a React application includes folders like 'node_modules' for external libraries, 'public' for public assets, and 'src' for source code.
- 🔄 To create a new React project, use the `npm create vite@latest` command, which sets up a development environment with a modern toolchain.
- 🔗 Components are imported and used within other components by referencing them within the return statement, allowing for a modular and maintainable codebase.
- ⚙️ React components can contain both JavaScript logic and HTML structure, and can be styled with CSS, either through dedicated stylesheets or inline styles.
Q & A
What is ReactJS?
-ReactJS is a JavaScript library used to build and arrange user interfaces for web applications. It focuses on creating web applications using components, which are reusable building blocks of code.
What is a component in React?
-A component in React is a self-contained section of code that functions as a reusable building block, similar to Legos, where each Lego represents a section of reusable JavaScript and HTML code.
What is JSX and how is it used in React?
-JSX is a syntax extension of JavaScript known as JavaScript XML. It allows you to write HTML-like code within your JavaScript files, making it easier to integrate HTML with React components.
What is the Virtual DOM and why is it used in React?
-The Virtual DOM is a lightweight version of the real DOM of a web page. It helps keep track of changes made to it, and only applies those specific changes to the real DOM without needing to refresh the entire web page, reducing rendering performance overhead.
What are the prerequisites for learning React?
-To learn React, you should know JavaScript, including arrays, classes, objects, and ES6 features like arrow functions. HTML is also mandatory since React components involve rendering HTML elements.
How do you install Node.js and why is it necessary for React?
-Node.js can be downloaded from nodejs.org. It is necessary for React because it provides a backend JavaScript runtime environment and includes the Node Package Manager (npm), which is used to manage packages and dependencies for React projects.
What is a text editor and why is it needed for React development?
-A text editor is a program used for editing plain text files. For React development, a text editor like Visual Studio Code (VS Code) is recommended as it provides features that aid in coding, such as syntax highlighting and debugging tools.
How do you create a new React project using npm?
-To create a new React project, you use the command `npm create vite@latest` in the terminal or command prompt within your project folder. This command sets up a new React project with a development server.
What is the purpose of the 'node_modules' folder in a React project?
-The 'node_modules' folder contains external libraries and packages that the React project relies on, including build tools, utility libraries, and routing libraries.
What is the role of the 'public' folder in a React application?
-The 'public' folder in a React application contains public assets such as fonts, images, and videos that are not bundled during the final output and are typically available via a URL.
How do you create a new component in React?
-To create a new component in React, you create a new .jsx file, define a function with the name of the component, and export it using `export default`. Within this function, you can return a combination of JavaScript and HTML to define the component's structure.
Outlines
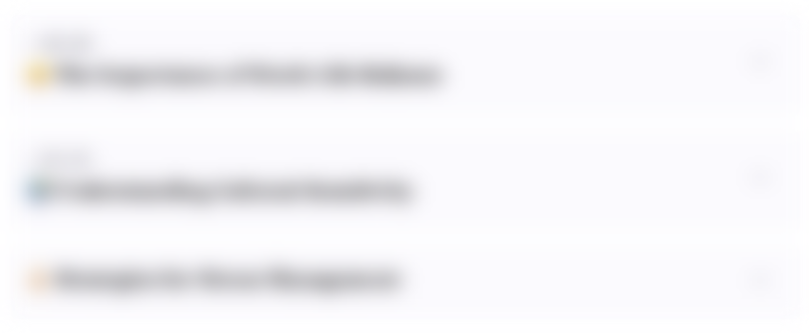
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
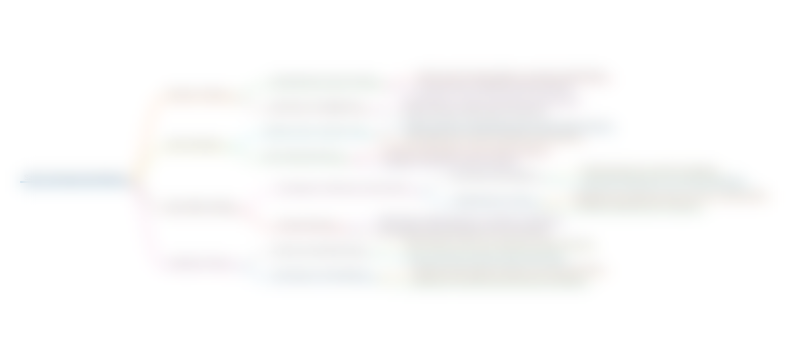
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
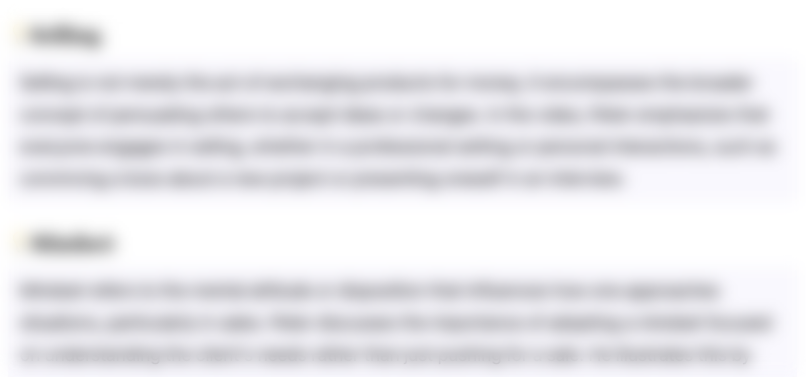
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
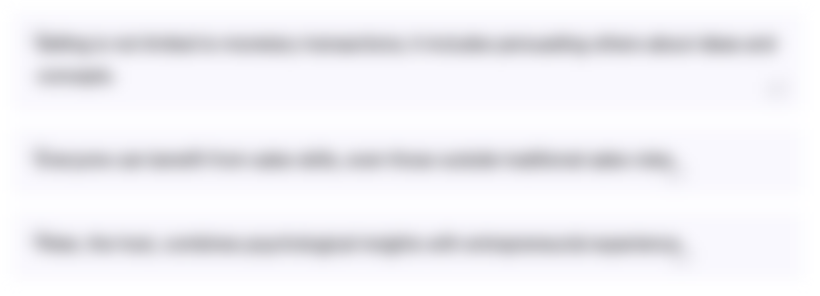
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
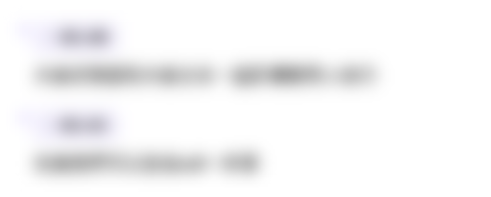
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
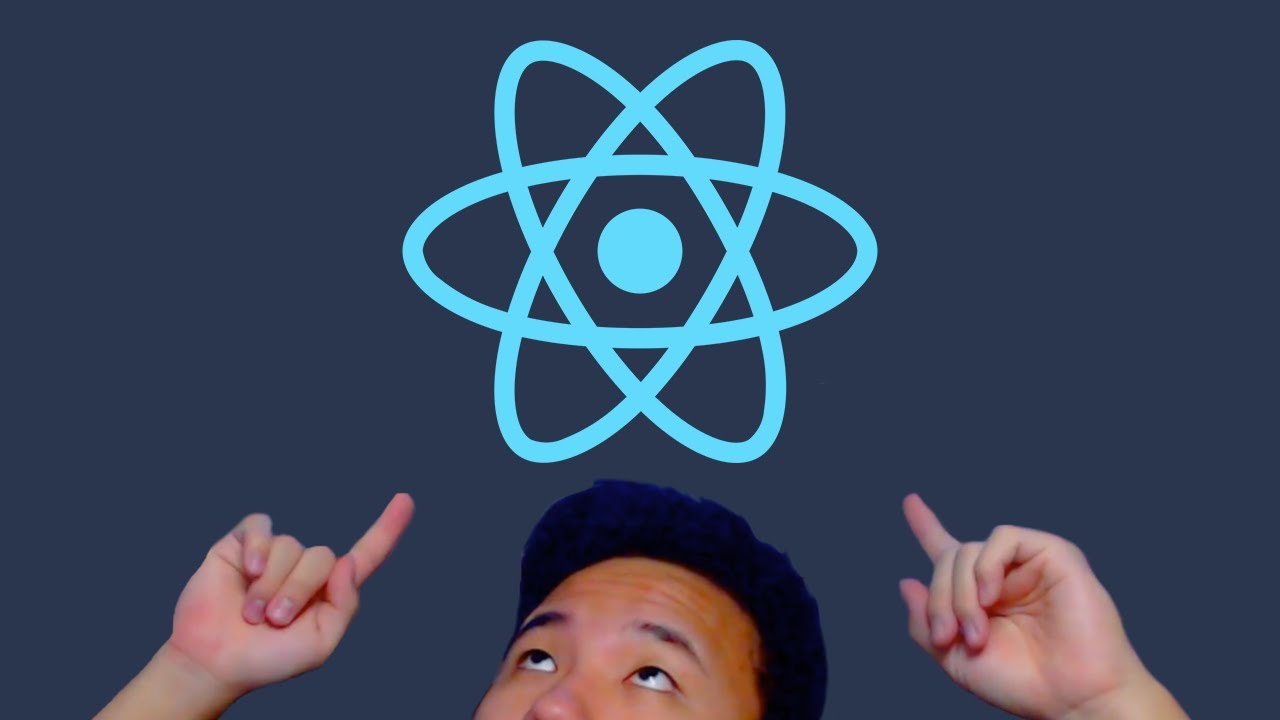
Aprende React en 45 Minutos
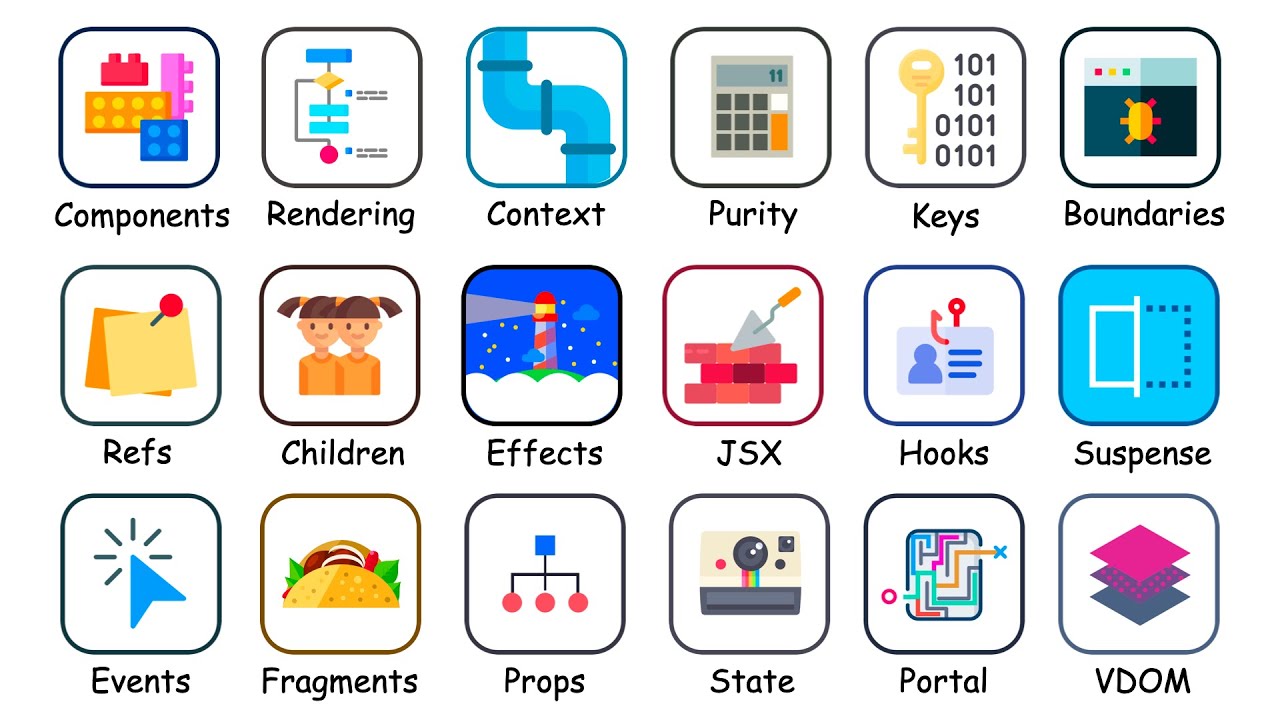
Every React Concept Explained in 12 Minutes

What is React | Lecture 09 | React.JS 🔥
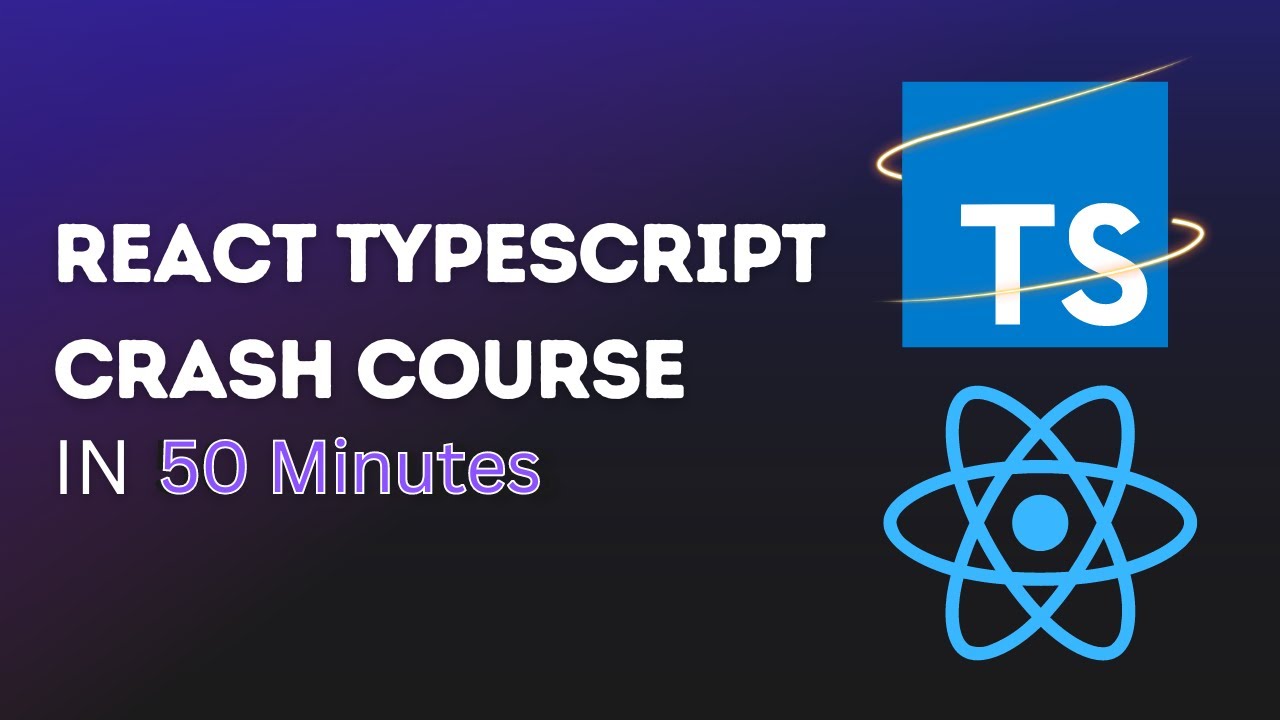
Learn TypeScript For React in 50 Minutes - React TypeScript Beginner Crash Course
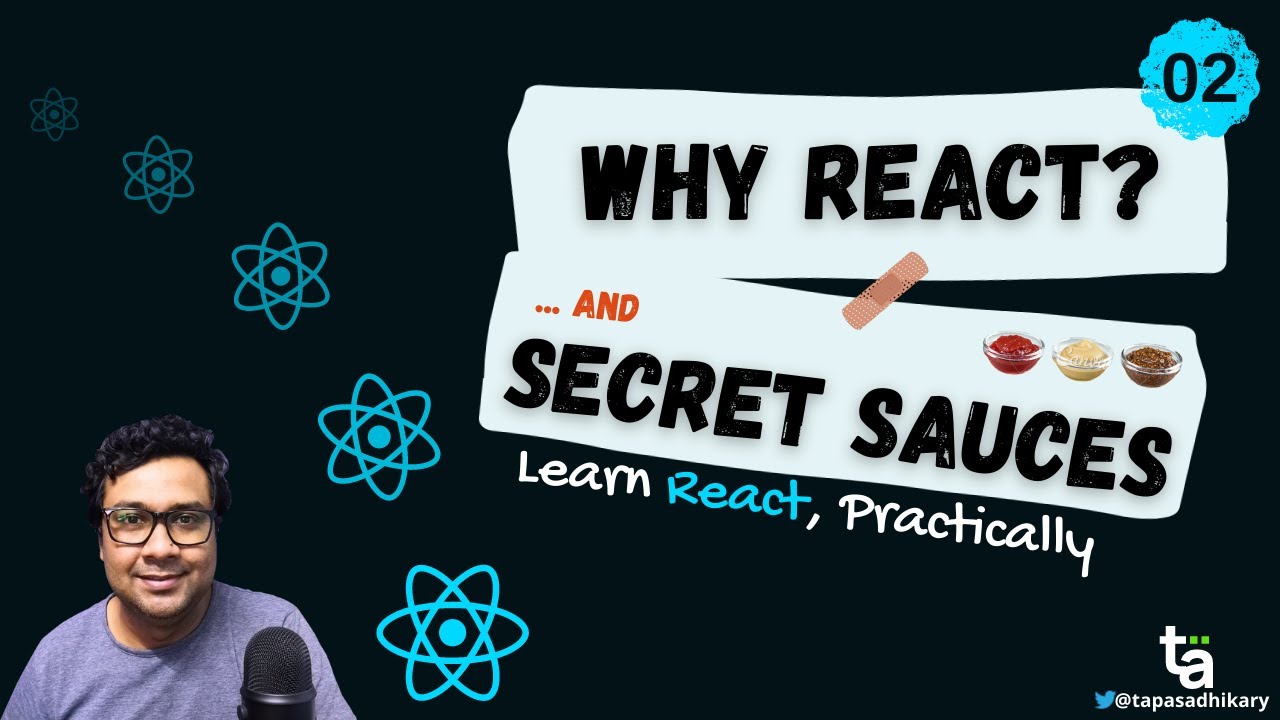
02 - Why React & Secret Sauces - React Fundamentals - Why React is a Declarative - Mastering Reactjs
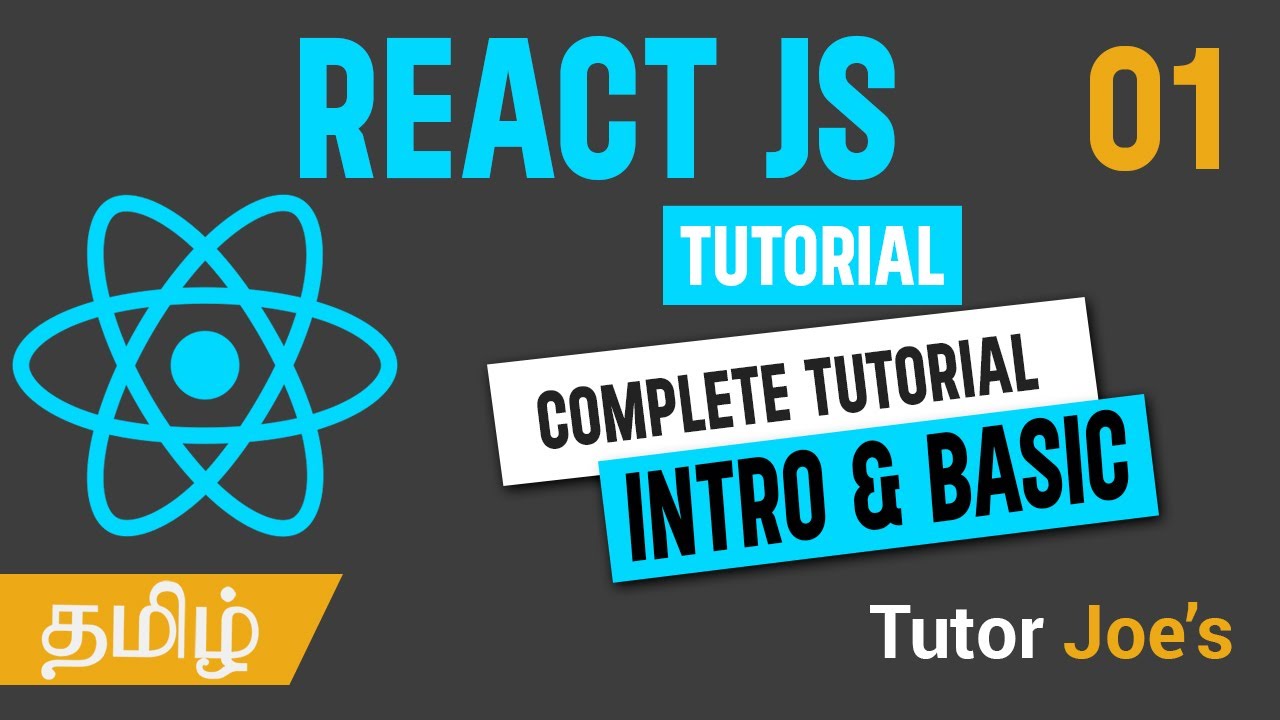
React JS Complete Tutorial in Tamil | Day - 01 | React JS in Tamil
5.0 / 5 (0 votes)