A First Look at Effects | Lecture 141 | React.JS 🔥
Summary
TLDRThis video script delves into the concept of side effects in React, distinguishing them from event handler functions. It explains that side effects are interactions between a React component and external systems, such as fetching data from an API. The script highlights the importance of not performing side effects during component rendering and instead using event handlers for event-triggered actions or the useEffect hook for automatic execution during component lifecycle events. The useEffect hook is used to keep components synchronized with external data, and the script emphasizes the preference for event handlers over the useEffect hook whenever possible.
Takeaways
- 🔍 The `useEffect` hook is used to fetch data as a component mounts, creating side effects.
- 🌐 A side effect in React is any interaction between a component and the outside world, such as fetching data from an API.
- ⚠️ Side effects should not occur during component render; they should be separate from render logic.
- 📌 Side effects can be created in two places in React: inside event handlers or using the `useEffect` hook.
- 🎯 Event handlers are functions triggered by specific events, while effects run automatically as the component renders.
- 🔄 Effects can run at different lifecycle moments: on mount, re-render, or unmount.
- 🔄 The timing of an effect's execution depends on its dependency array, which can also trigger it after re-renders.
- 🧹 Each effect can return a cleanup function that runs before the component re-renders or unmounts.
- 🔄 Understanding the component lifecycle (mounting, re-rendering, unmounting) helps grasp how effects work.
- 🔄 The primary purpose of effects is to keep components synchronized with external systems, not just to run code at lifecycle points.
- 🚫 Event handlers are the preferred method for creating side effects; the `useEffect` hook should be used sparingly.
Q & A
What is an effect in the context of React?
-An effect in React refers to any interaction between a React component and the world outside that component, such as fetching data from an API. It's a way to make something useful happen.
How is an effect different from an event handler function?
-An effect is used to execute code automatically as a component renders, re-renders, or unmounts, while an event handler function is triggered by a specific event happening in the user interface.
Why should side effects not happen during the component render?
-Side effects should not occur during the component render to keep the render logic clean and focused on updating the component's state based on its props and state, without external interactions.
When might you want to fetch movie data using an event handler function?
-You might want to fetch movie data using an event handler function when the data retrieval is tied to a specific user action or event, such as a button click.
What is the purpose of the useEffect hook?
-The useEffect hook is used to create effects, allowing you to execute code at different moments in a component's lifecycle, such as when the component mounts, re-renders, or unmounts.
What are the three parts that an effect can have?
-An effect can have three parts: the effect code itself, a dependency array, and a cleanup function that is called before the component re-renders or unmounts.
How does the dependency array in useEffect work?
-The dependency array in useEffect determines when the effect should run. If the values in the array change, the effect will re-run after the component re-renders.
What is the main reason for using effects in a React component?
-The main reason for using effects is to keep the component synchronized with an external system, such as keeping it up-to-date with data from an external API.
Why are event handlers preferred over the useEffect hook for creating side effects?
-Event handlers are preferred because they are more specific and directly tied to user actions or events, which makes the code more predictable and easier to manage. The useEffect hook should be used sparingly and only when necessary.
When is it appropriate to use the useEffect hook to fetch data?
-The useEffect hook is appropriate for fetching data when the data retrieval is not directly tied to a user event, such as when the component first mounts and needs to fetch initial data.
What is the cleanup function in an effect used for?
-The cleanup function in an effect is used to perform any necessary cleanup before the component re-renders or unmounts, such as canceling a subscription or stopping a timer.
Outlines
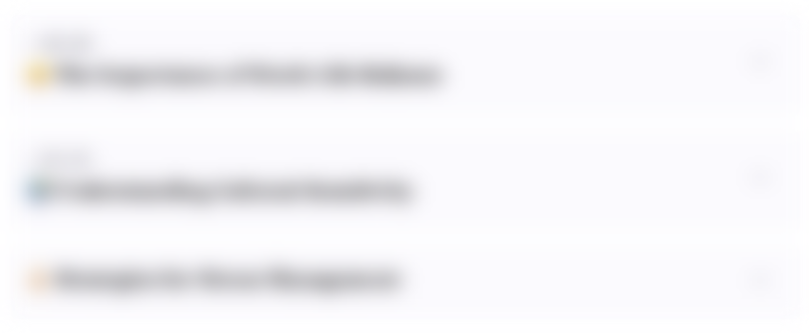
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
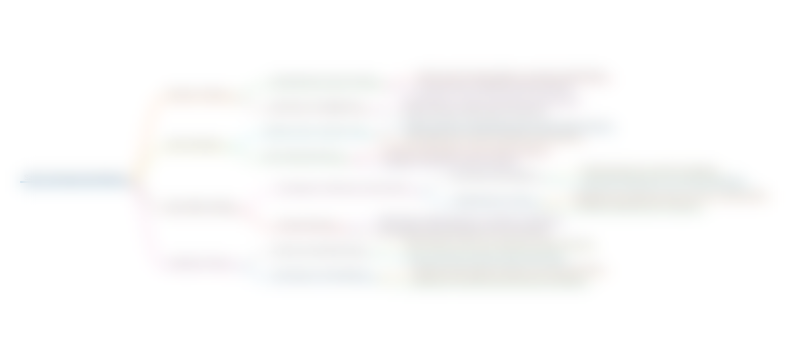
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
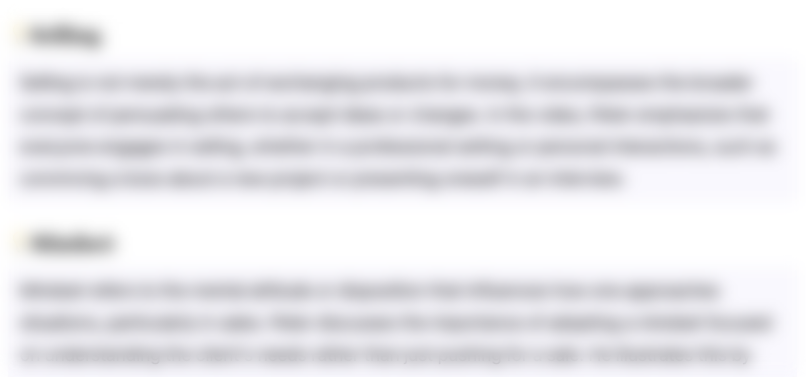
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
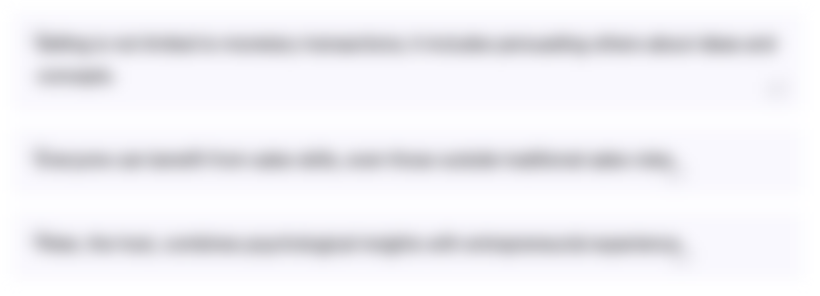
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
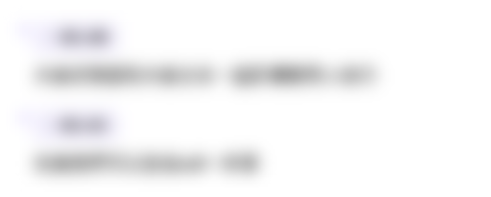
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes
5.0 / 5 (0 votes)