CH01_VID02_Encapsulation
Summary
TLDRThe script discusses the concept of 'encapsulation' in object-oriented programming, using the analogy of a house design. It explains how a class represents the blueprint, while objects are the actual instances built from it. The video script delves into attributes, methods, and the importance of private attributes to maintain object integrity. It also touches on the idea of creating a separate class for a programmer's design, emphasizing the distinction between the class as a blueprint and the object as a real-world representation.
Takeaways
- 📦 Encapsulation: The script emphasizes the concept of encapsulation, where each unit or object is self-contained, and interaction with attributes is done through specific functions.
- 🏗️ Class and Object: A class is a design blueprint, while an object is a real instance of that class. The script compares a class to a house design and an object to the actual house built from that design.
- 🔒 Private and Public: The script discusses how attributes in a class can be private, meaning they can't be accessed directly but only through designated functions.
- 💡 Attribute Types: When defining attributes in a class, their types (like strings, dates, numbers) must be specified, which determines how they will be stored in memory.
- 📝 Naming Conventions: Class names should be nouns, start with a capital letter, and be easily understood to make the code more readable and maintainable.
- ⚙️ Functions and Methods: Functions in a class are actions that the object can perform, often named with verbs to represent their behavior.
- 🔄 Object Instances: Different objects created from the same class may have different values for their attributes, even though they share the same structure.
- 🔗 Object Relations: The script mentions that objects can be related or interact with one another, and this interaction is managed through functions and attributes.
- 📑 UML Diagrams: UML (Unified Modeling Language) diagrams are highlighted as tools to visualize object-oriented designs, making it easier to understand the relationships and structure.
- 🛠️ Design and Implementation: The process of designing a program involves thinking about which classes are needed, what attributes they should have, and how they will interact through methods.
Q & A
What does encapsulation mean in the context of object-oriented programming?
-Encapsulation means that each unit (object) is self-contained, with its attributes and methods accessible only through specific functions, ensuring that there are no global variables and everything is related to a specific object.
Why is it important to define attributes as private in a class?
-Defining attributes as private ensures that they cannot be accessed directly from outside the object, promoting data encapsulation and security. They can only be interacted with through specific methods provided by the class.
What is the significance of using classes in object-oriented design?
-Classes act as blueprints for objects, defining the attributes and behaviors that the objects created from the class will have. This allows for creating multiple instances (objects) with the same structure but different data.
How does creating an object differ from defining a class?
-Defining a class involves outlining the structure and behaviors of the objects, whereas creating an object involves instantiating a specific instance of that class, with actual values assigned to its attributes.
Can you explain the analogy between designing a house and defining a class?
-Designing a house with a blueprint is similar to defining a class. The blueprint outlines the structure and features of the house (attributes and methods of the class), but it is not the house itself. The actual house is the object created from that blueprint.
Why is it important to name classes using nouns and starting with a capital letter?
-Naming classes using nouns and starting with a capital letter follows standard naming conventions, making the code more readable and understandable for anyone who views the design or code later.
What is the purpose of methods in a class?
-Methods define the behaviors or actions that the objects created from the class can perform. They allow interaction with the object's data while maintaining encapsulation.
How do diagrams like UML help in understanding object-oriented programming?
-UML diagrams visually represent the design of a system, including the relationships between classes and objects, making it easier to understand and communicate the structure and behavior of the system.
What happens when you create multiple objects from the same class?
-Each object will have the same structure as defined by the class but can hold different values in its attributes. This allows for creating diverse instances while maintaining a consistent design.
What does it mean for an object to be an instance of a class?
-An object being an instance of a class means it is a specific realization of the class blueprint, with actual data and state, created based on the structure and behaviors defined in the class.
Outlines
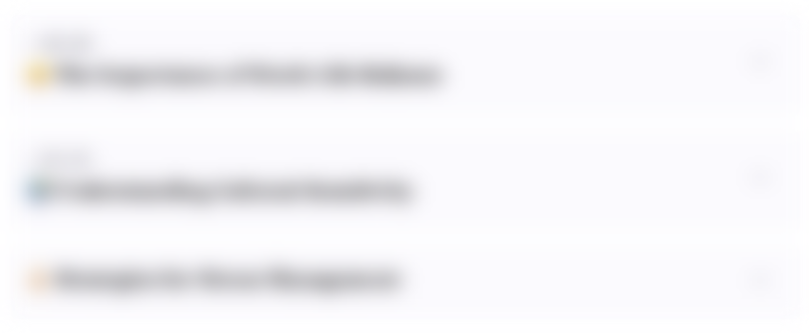
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
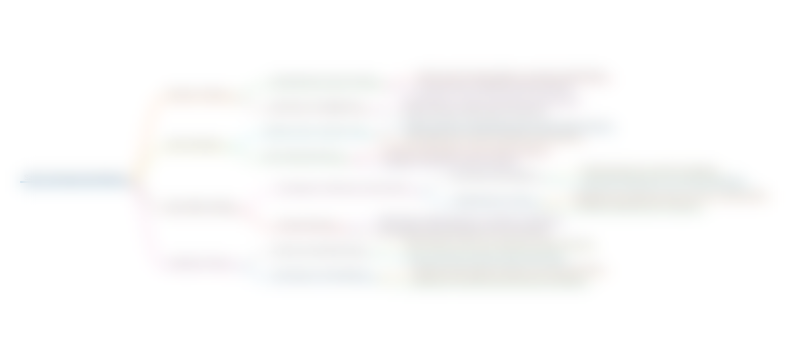
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
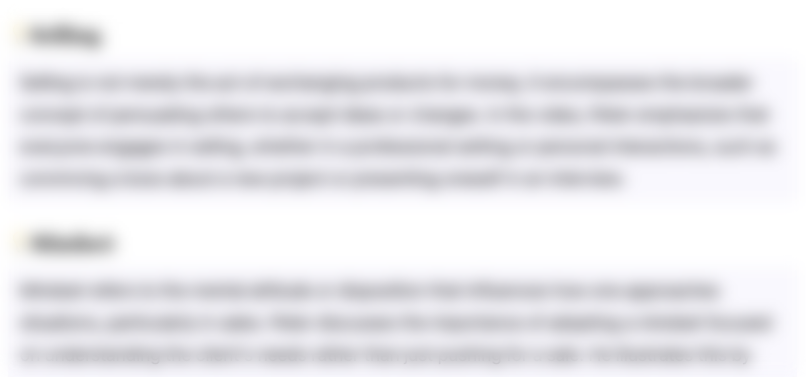
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
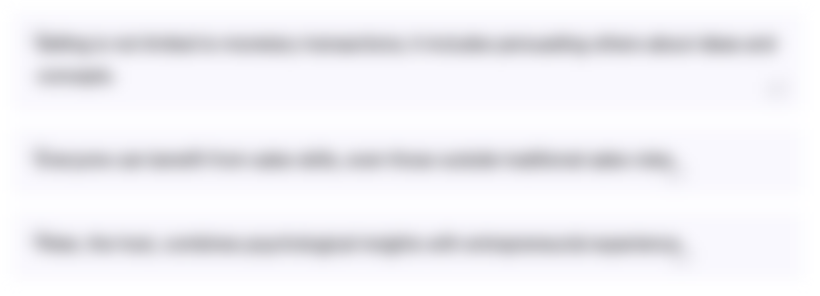
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
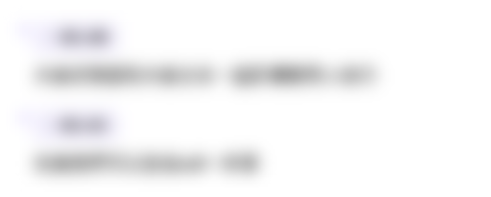
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
5.0 / 5 (0 votes)