Fundamental Concepts of Object Oriented Programming
Summary
TLDRThis script introduces the core principles of object-oriented programming (OOP): abstraction, encapsulation, inheritance, and polymorphism. It explains how abstraction simplifies real-world complexity for software applications, encapsulation hides object complexities, and inheritance allows classes to derive properties and methods from others, creating a hierarchy. Polymorphism enables subclasses to implement inherited methods uniquely, showcasing OOP's power in creating flexible, reusable code.
Takeaways
- π§© **Abstraction**: Simplifies reality by focusing only on the data and processes relevant to the application being built.
- π¦ **Encapsulation**: Binds data and the programs that manipulate it together, hiding their complexity from the outside.
- π **Inheritance**: Allows a class to derive its methods and properties from another class, creating a hierarchy of superclasses and subclasses.
- π **Polymorphism**: Enables a class to implement an inherited method in its own way, allowing different subclasses to behave differently despite sharing the same interface.
- π **Objects in OOP**: Represent real-world entities or concepts that the software application is interested in, both physical and abstract.
- π **Classes as Templates**: Serve as blueprints for creating objects, defining their attributes and operations (methods).
- π **Properties and Methods**: Properties describe an object's data, while methods are the actions that can be performed on or by the object.
- π **Instantiation**: The process of creating an object from a class, making each object a unique instance with its own state.
- π **Constructors**: Special methods used during instantiation to assign initial values to an object's properties.
- π‘οΈ **Information Hiding**: A principle of encapsulation that protects the internal state of an object from external interference and misuse.
- π **Type Relationships**: Inheritance defines 'is-a' relationships, where subclasses are specific types of their base classes.
Q & A
What are the four fundamental concepts of object-oriented programming?
-The four fundamental concepts of object-oriented programming are abstraction, encapsulation, inheritance, and polymorphism.
What is an object in the context of object-oriented programming?
-An object in object-oriented programming is anything of interest to the software application being built, which can be a physical thing like a car or a non-physical entity like a bank account.
What is abstraction in object-oriented programming?
-Abstraction in object-oriented programming is the process of simplifying reality to focus only on the data and processes that are relevant to the application being built.
What is a class and how does it relate to objects?
-A class is a template for creating objects, defining the attributes (properties) and operations (methods) of an object. It is used to instantiate objects, which are instances of the class.
What is encapsulation and why is it important?
-Encapsulation is the concept of hiding the complexity of an object's inner workings from the outside. It is important for information hiding and ensuring the integrity and safety of the data and operations within the object.
How does inheritance work in object-oriented programming?
-Inheritance allows a class to derive its methods and properties from another class, creating a hierarchy of classes where a subclass inherits features from its superclass.
What is polymorphism and how does it relate to object-oriented programming?
-Polymorphism allows a class to implement an inherited method in its own way, enabling different subclasses of the same superclass to behave differently despite sharing the same interface.
What is a constructor in the context of object-oriented programming?
-A constructor is a special method used during the instantiation of an object, allowing the assignment of values to properties at the time of object creation.
How does the concept of a 'state' of an object relate to its properties?
-The 'state' of an object refers to the values assigned to its properties, making each object of the same type a unique entity.
What is the difference between a base class and a subclass in terms of inheritance?
-A base class is the class at the start of the inheritance hierarchy, while a subclass is any class that derives from another class, inheriting its methods and properties.
Why is it beneficial for a programmer to use a class library?
-Using a class library is beneficial because it simplifies the use of objects and ensures that the data and operations encapsulated within are safe, without needing to understand the inner workings of the class.
Outlines
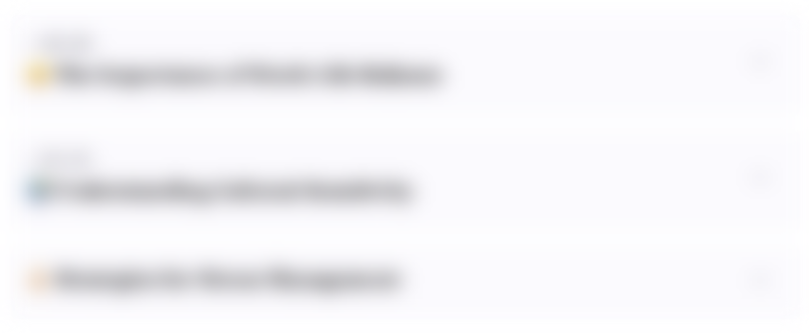
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
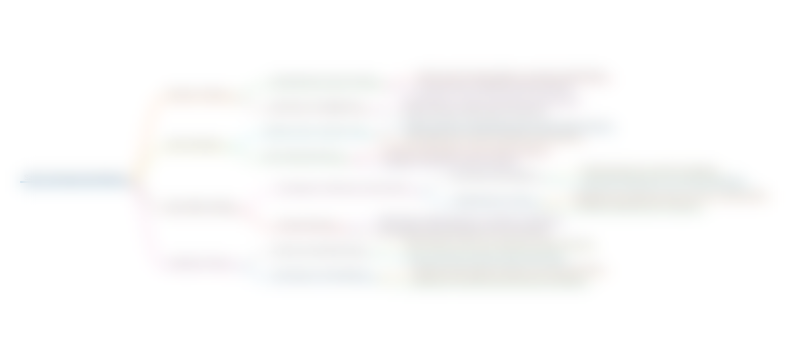
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
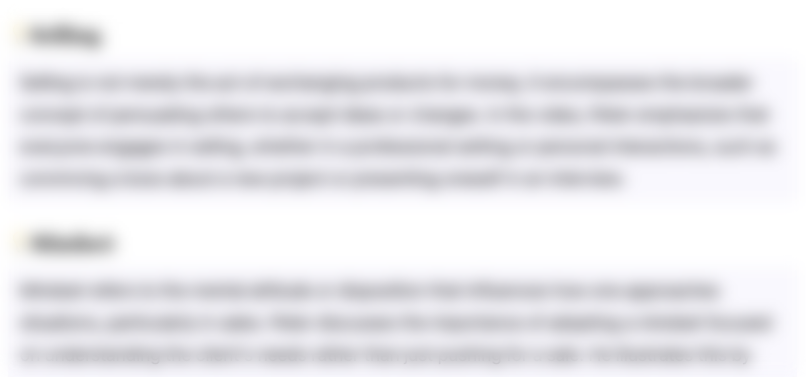
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
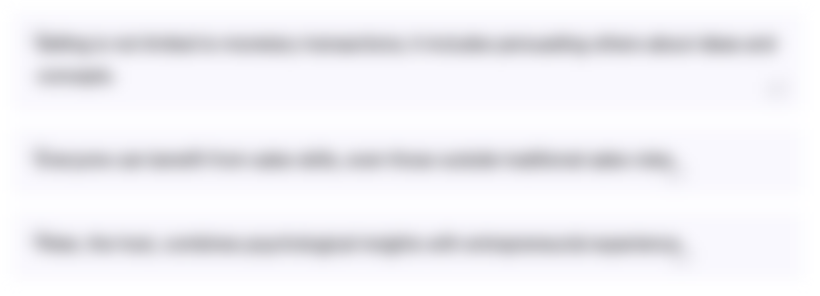
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
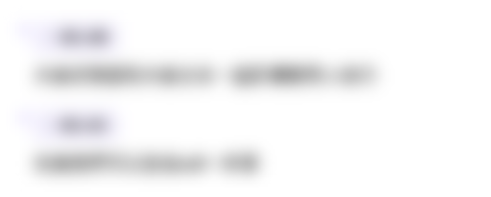
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Overview of Object-oriented programming in C# | C# object-oriented programming | C# oops
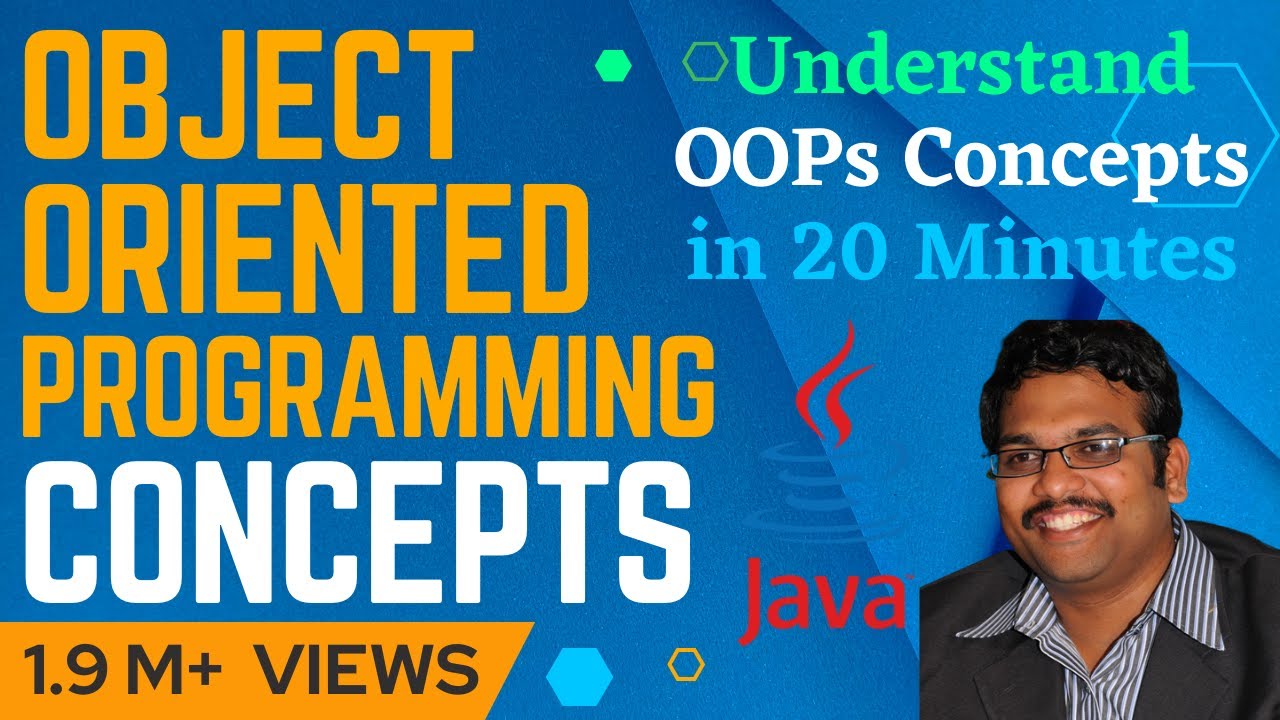
OOPS CONCEPTS - JAVA PROGRAMMING
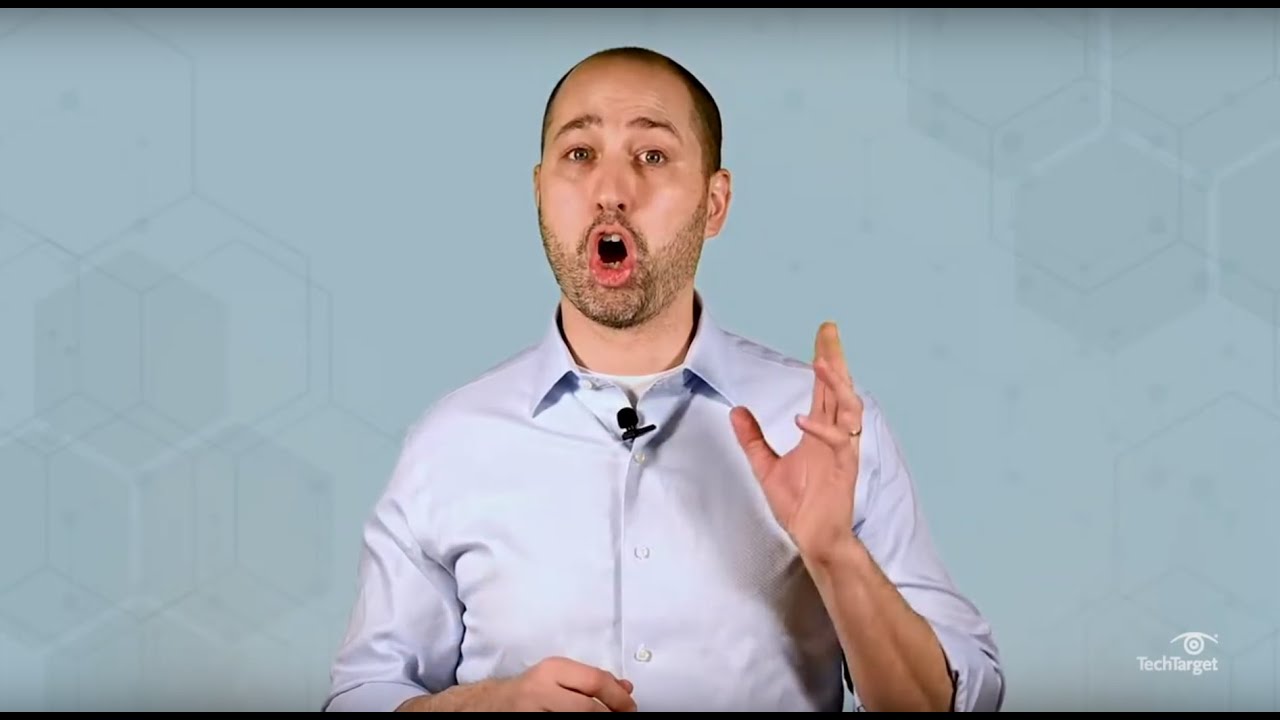
What is Object-Oriented Programming (OOP)?
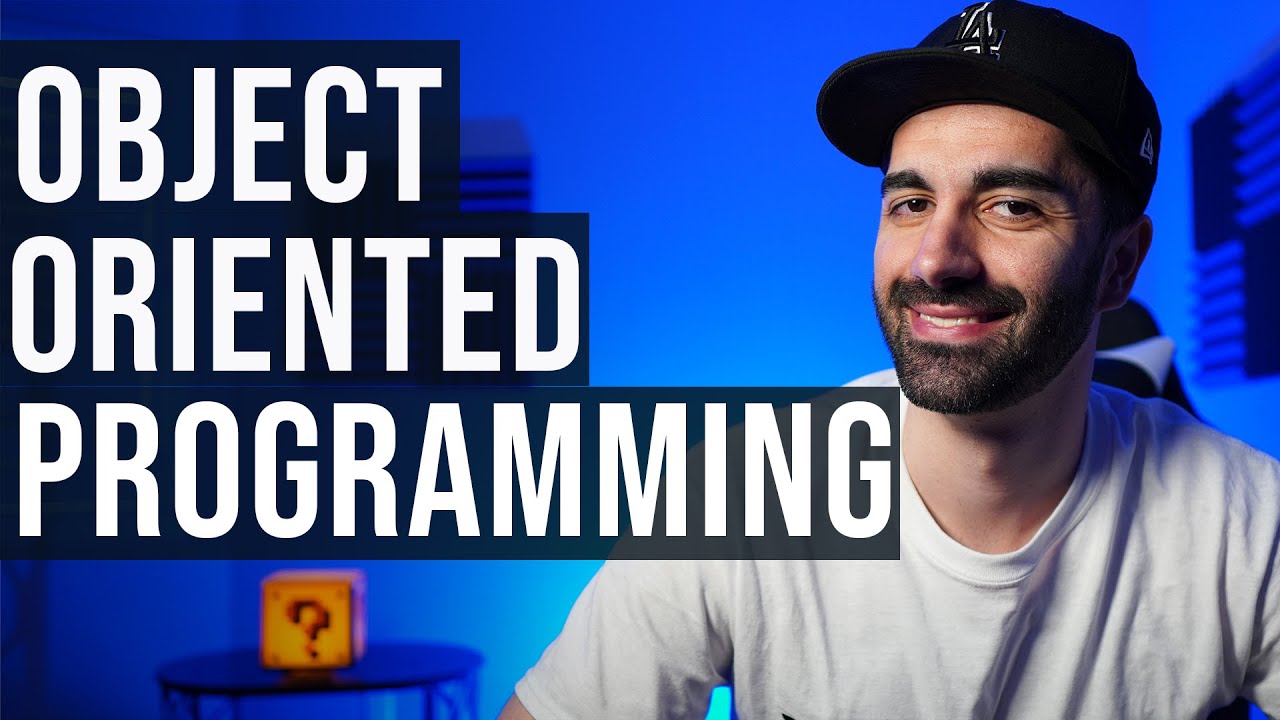
Object Oriented Programming - The Four Pillars of OOP
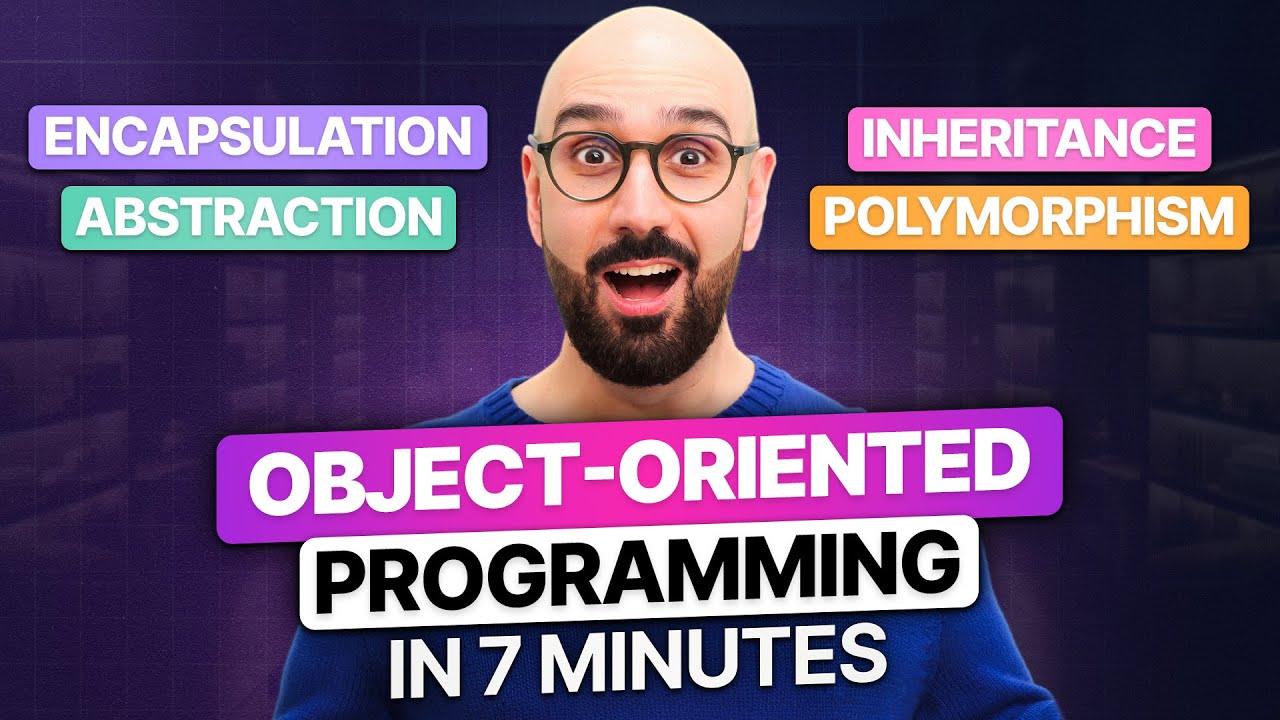
Object-oriented Programming in 7 minutes | Mosh
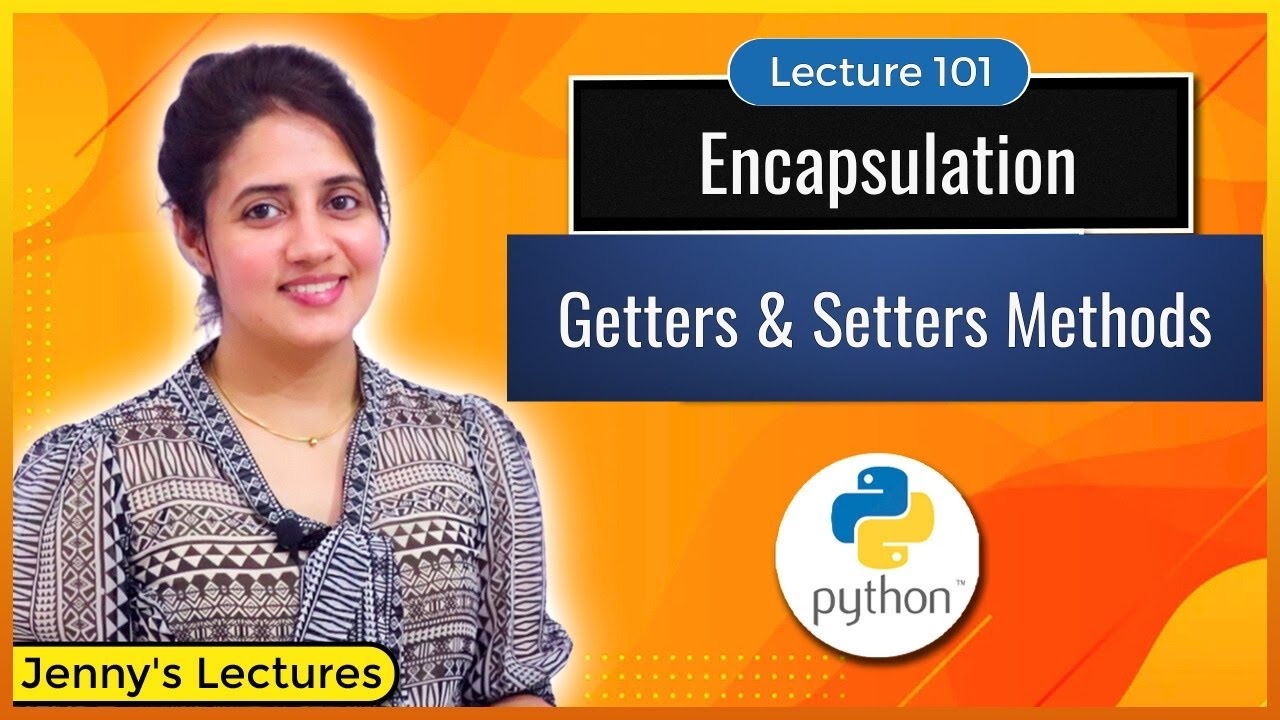
Encapsulation in Python | Getters & Setters methods | Python Tutorials for Beginners #lec101
5.0 / 5 (0 votes)