Encapsulation in Java Tutorial #83
Summary
TLDRIn this engaging tutorial, Alex introduces the concept of encapsulation in Java, explaining it as a method to protect and manage class variables. Using a practical example of a `Student` class, he demonstrates how to implement getters and setters to control access to the object's attributes. By abstracting the variables and making them private, encapsulation simplifies user interactions and promotes best practices in object-oriented programming. Alex emphasizes that encapsulation, while often perceived as complex, is fundamentally straightforward, making it accessible for beginners. This tutorial is a valuable resource for anyone looking to grasp the basics of encapsulation in Java.
Takeaways
- 😀 Encapsulation in Java allows control over class variables using methods, promoting data protection.
- 😀 The concept is likened to a capsule, where all variables are contained within methods, preventing direct access.
- 😀 A common example of encapsulation is creating a `Student` class with private attributes like `name` and `age`.
- 😀 Getters and setters are used to interact with private variables, ensuring that any necessary validation can be included.
- 😀 Setter methods (e.g., `setName` and `setAge`) allow external code to modify private attributes safely.
- 😀 Getter methods (e.g., `getName` and `getAge`) provide a way to retrieve private attributes while keeping them hidden.
- 😀 Marking class attributes as private enhances security and encapsulation by preventing unauthorized access.
- 😀 Encapsulation simplifies the interface of an object, making it easier for users to interact with it without knowing its internal structure.
- 😀 The practice of using getters and setters is a common approach in object-oriented programming for encapsulating data.
- 😀 Overall, encapsulation is a key principle in Java that promotes better organization, maintainability, and simplicity in code.
Q & A
What is encapsulation in Java?
-Encapsulation in Java is the concept of restricting direct access to certain components of an object, allowing access only through methods that set or retrieve the values of private variables.
Why is encapsulation important in object-oriented programming?
-Encapsulation is important because it protects the internal state of an object, prevents unauthorized access and modification, and simplifies the interface for users, making the object easier to use.
What are getters and setters?
-Getters and setters are methods used in encapsulation to set and retrieve the values of private variables. Getters return the value of a variable, while setters assign a value to a variable.
What access modifier is typically used for encapsulated variables?
-Private access modifier is typically used for encapsulated variables to restrict direct access from outside the class.
How do you create a setter method in Java?
-A setter method in Java is created as a public method with a void return type that takes a parameter. It assigns the parameter value to the private variable, e.g., 'public void setName(String newName) { this.name = newName; }'.
What is the purpose of the 'this' keyword in setters?
-The 'this' keyword is used in setters to distinguish between class variables and method parameters when they have the same name, ensuring the correct variable is assigned.
Can encapsulated variables be accessed directly from outside the class?
-No, encapsulated variables cannot be accessed directly from outside the class if they are declared as private; they can only be accessed through public getter and setter methods.
What happens if you try to access a private variable directly?
-If you try to access a private variable directly from outside its class, you will receive a compilation error, indicating that the variable is not accessible.
How can encapsulation enhance code maintainability?
-Encapsulation enhances code maintainability by allowing developers to change the internal implementation of a class without affecting the code that uses the class, as long as the public interface (getters and setters) remains the same.
What are the key components of a class that utilizes encapsulation?
-The key components of a class that utilizes encapsulation are private variables, public getter and setter methods, and a clear interface that allows controlled access to the variables.
Outlines
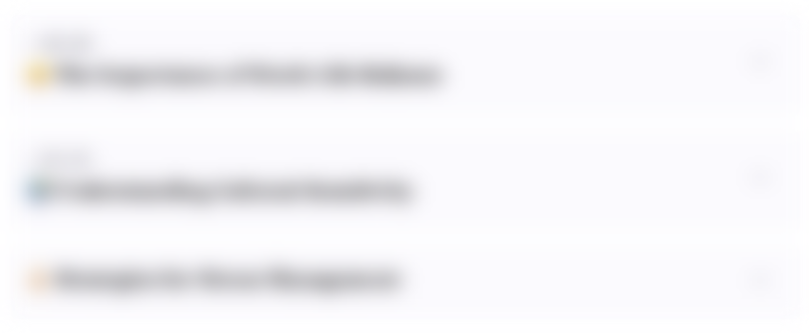
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
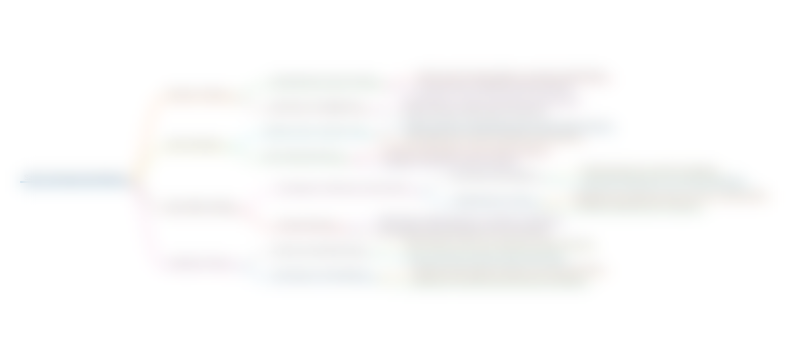
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
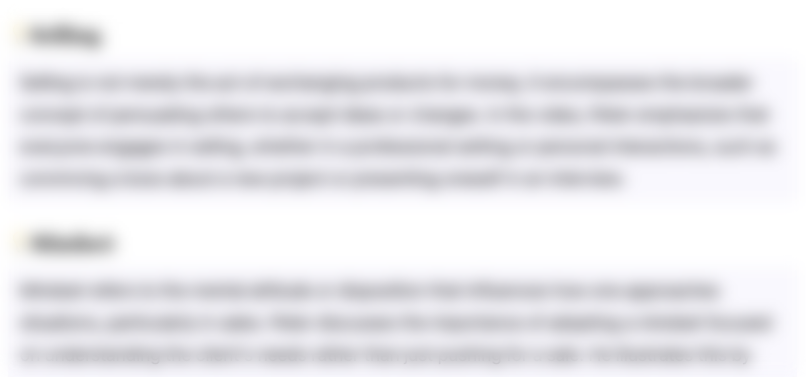
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
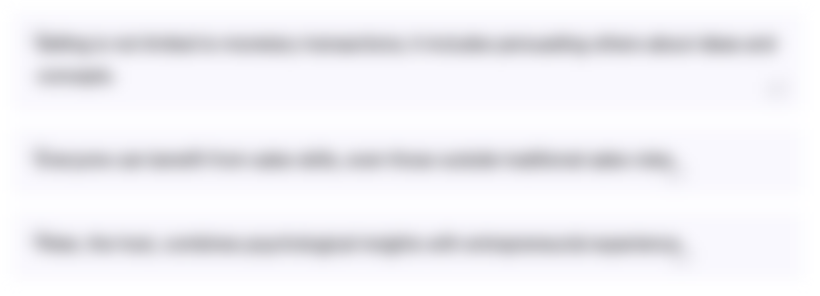
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
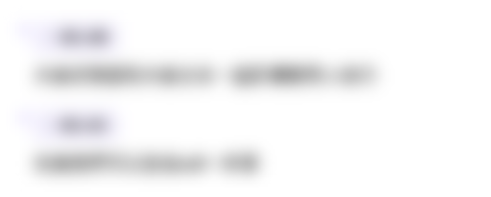
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)