C_18 Operators in C - Part 6 | Bitwise Operators | C Programming Tutorials
Summary
TLDRThis tutorial delves into bitwise operators in C programming, explaining their importance in performing operations at the bit level. The video covers key operators like AND, OR, XOR, NOT, left shift, and right shift, providing detailed examples of their usage on integers and characters. It also clarifies operator precedence and how expressions involving multiple operators are evaluated. With clear examples and program demonstrations, the video ensures viewers understand how bitwise operations manipulate data efficiently at the binary level, making it ideal for low-level programming tasks.
Takeaways
- 😀 Bitwise operators in C allow you to manipulate data at the binary (bit) level, directly affecting the bits of integers and characters.
- 😀 Bitwise operations are useful for efficient data manipulation in lower-level programming, such as embedded systems or hardware-related programming.
- 😀 There are six main bitwise operators in C: AND (&), OR (|), XOR (^), NOT (~), Left Shift (<<), and Right Shift (>>).
- 😀 Bitwise AND (&) returns 1 only when both bits are 1, and is useful for masking specific bits.
- 😀 Bitwise OR (|) returns 1 if at least one of the bits is 1, and is used to set specific bits in a number.
- 😀 XOR (^) returns 1 if the bits are different, and 0 if they are the same, often used for toggling bits.
- 😀 Bitwise NOT (~) inverts all the bits of a number, flipping 0s to 1s and vice versa.
- 😀 Left Shift (<<) shifts bits to the left, effectively multiplying the number by 2 raised to the number of shifted positions.
- 😀 Right Shift (>>) shifts bits to the right, effectively dividing the number by 2 raised to the number of shifted positions.
- 😀 Bitwise operations can only be applied to integer types (like int or char), not to floating-point types (like float or double).
Q & A
What are bitwise operators in C?
-Bitwise operators in C are used to perform operations on individual bits of integer or character values. These operations are performed at the binary level, allowing direct manipulation of bits within variables.
How does the bitwise AND operator (`&`) work?
-The bitwise AND operator (`&`) compares corresponding bits of two operands. It returns `1` only if both bits are `1`; otherwise, it returns `0`. For example, `10 & 5` (binary `1010 & 0101`) results in `0`.
What is the difference between bitwise AND (`&`) and logical AND (`&&`)?
-The bitwise AND (`&`) works at the bit level and compares individual bits, returning `1` if both bits are `1`. In contrast, logical AND (`&&`) works with boolean expressions, returning `true` (1) only if both conditions are true, and `false` (0) otherwise.
Explain how the bitwise OR operator (`|`) functions.
-The bitwise OR operator (`|`) compares corresponding bits of two operands. It returns `1` if at least one of the bits is `1`; otherwise, it returns `0`. For example, `10 | 5` (binary `1010 | 0101`) results in `15`.
What is the behavior of the bitwise XOR operator (`^`)?
-The bitwise XOR operator (`^`) compares corresponding bits and returns `1` if the bits are different, otherwise it returns `0`. It is commonly used for toggling bits. For example, `10 ^ 5` (binary `1010 ^ 0101`) results in `15`.
How does the bitwise NOT operator (`~`) work?
-The bitwise NOT operator (`~`) is a unary operator that inverts all the bits of the operand, changing `1` to `0` and `0` to `1`. For example, `~10` (binary `1010`) results in `-11` in 32-bit signed integer format due to two's complement representation.
What is the purpose of the left shift (`<<`) and right shift (`>>`) operators?
-The left shift (`<<`) operator shifts the bits of a number to the left by a specified number of positions, effectively multiplying the number by powers of two. The right shift (`>>`) operator shifts the bits to the right, effectively dividing the number by powers of two.
Why are bitwise operators not applicable to floating-point values in C?
-Bitwise operators in C work only on integer and character values because floating-point numbers are stored differently in memory using IEEE 754 format, which does not directly allow bitwise manipulation of individual bits as integer values do.
Can you explain the significance of bitwise operators in C for low-level programming?
-Bitwise operators are crucial in low-level programming because they allow efficient manipulation of individual bits in data, which is often necessary for tasks like hardware control, encryption, performance optimization, and flag manipulation in memory-constrained environments.
What happens when multiple operators (like bitwise and logical operators) are used in a single C expression?
-When multiple operators are used in a single expression, the operators are evaluated based on their precedence and associativity. For example, relational operators have higher precedence than bitwise operators, and logical operators typically follow the bitwise operations. The result depends on this order of evaluation.
Outlines
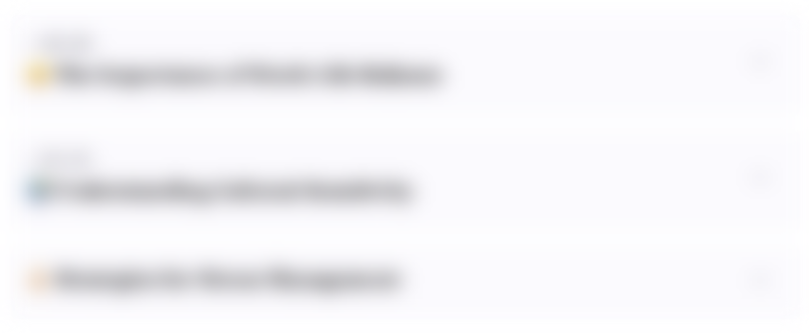
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
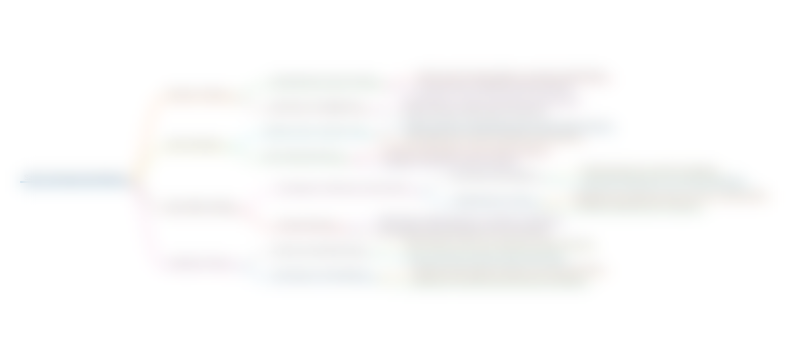
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
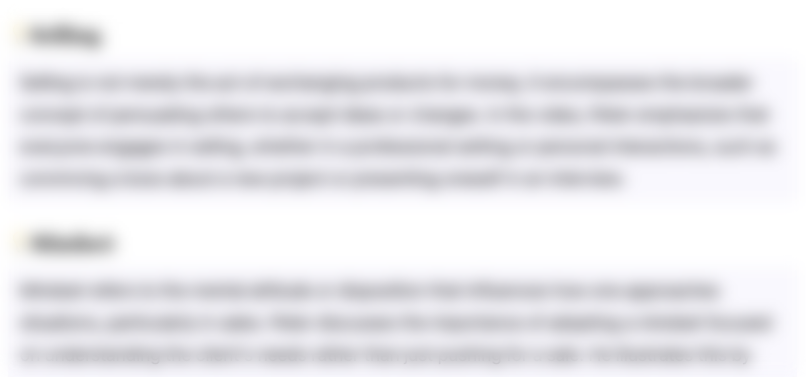
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
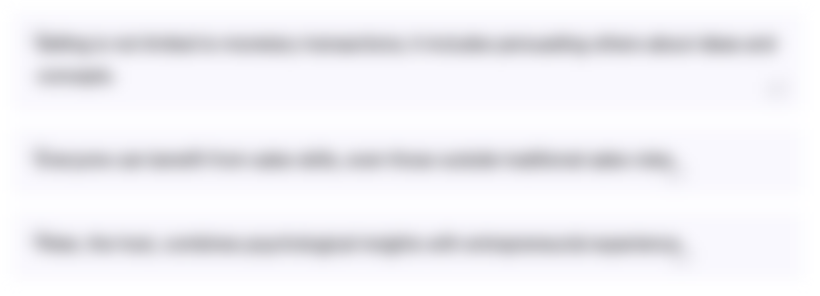
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
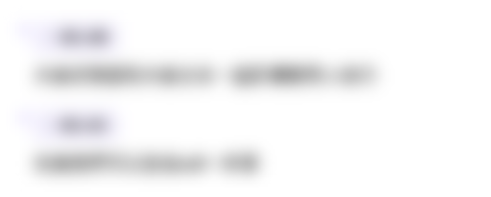
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
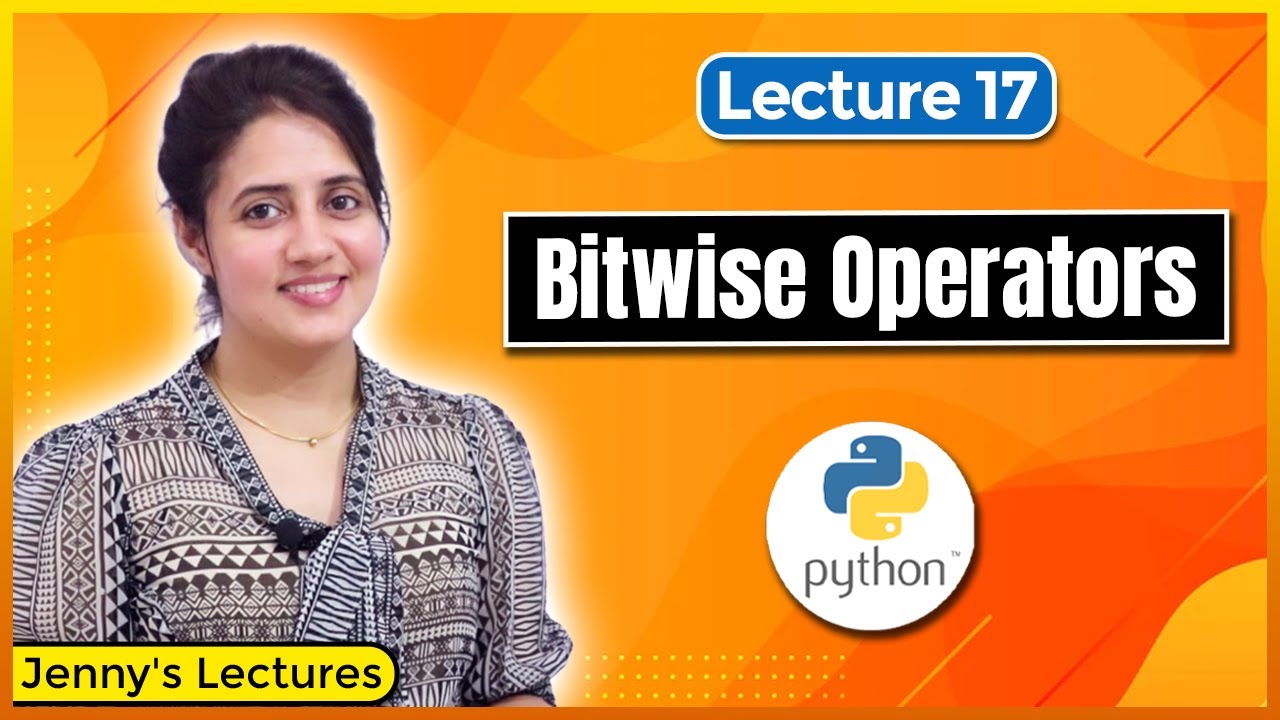
Operators in Python | Bitwise Operators | Python Tutorials for Beginners #lec17
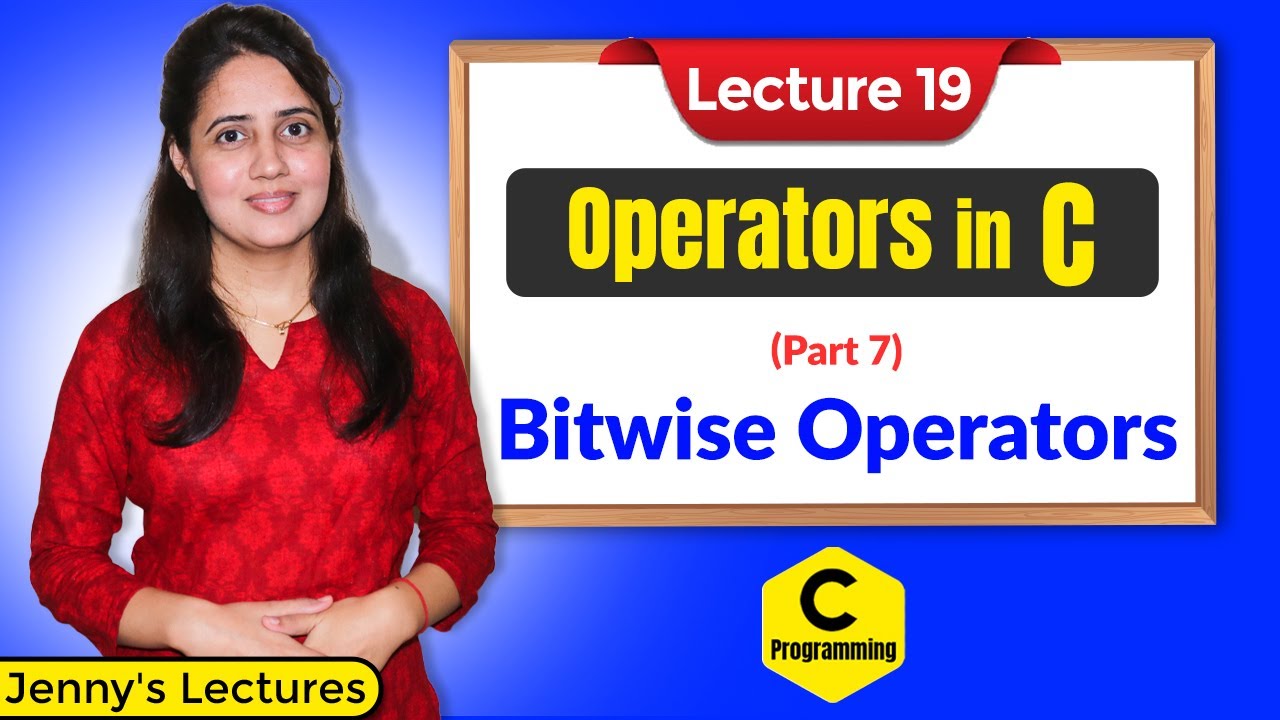
C_19 Operators in C - Part 7 (Bitwise Operators-II) | C Programming Tutorials
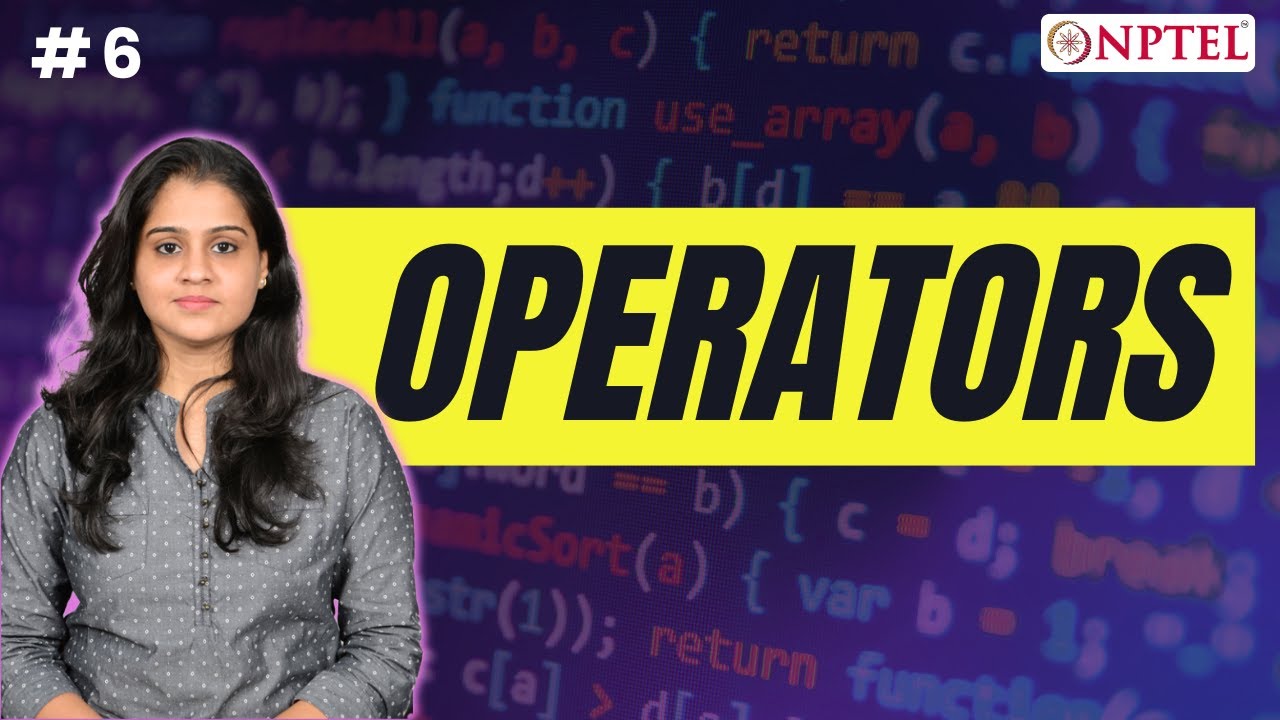
Operators
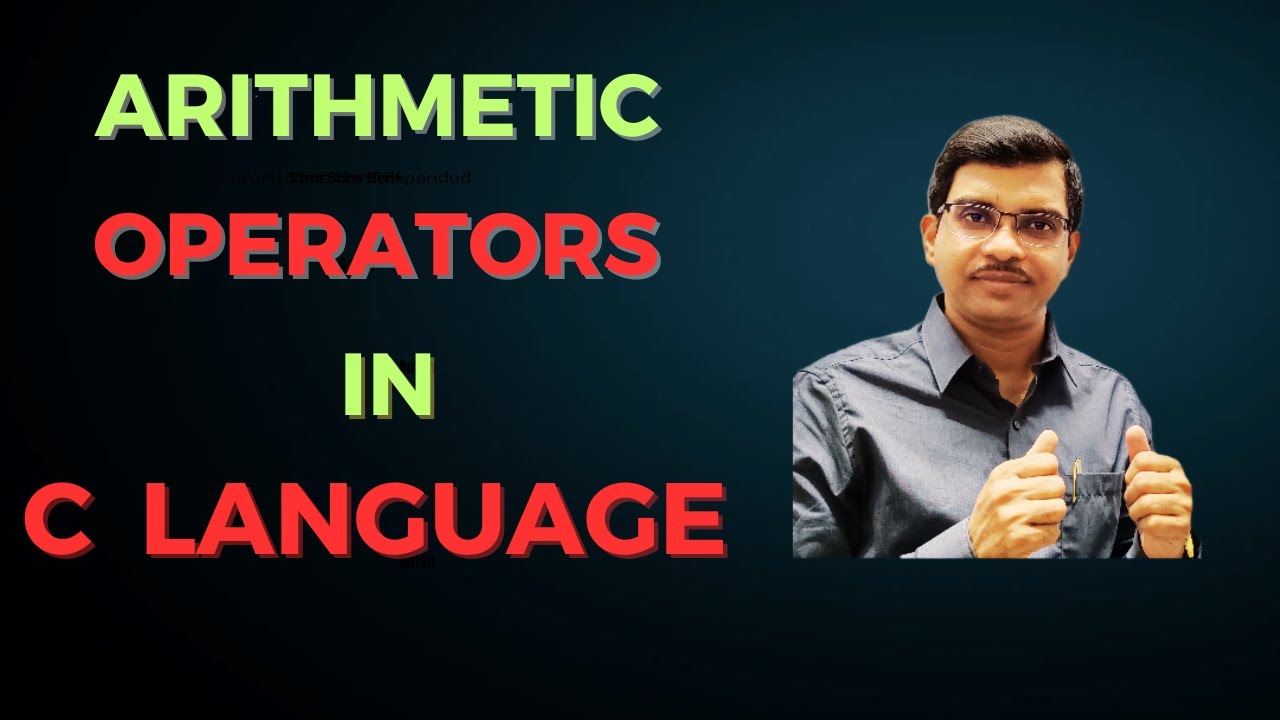
C_12 Arithmetic Operators in C Language | C Programming Tutorials

C_13 Operators in C - Part 1 | Unary , Binary and Ternary Operators in C | C programming Tutorials
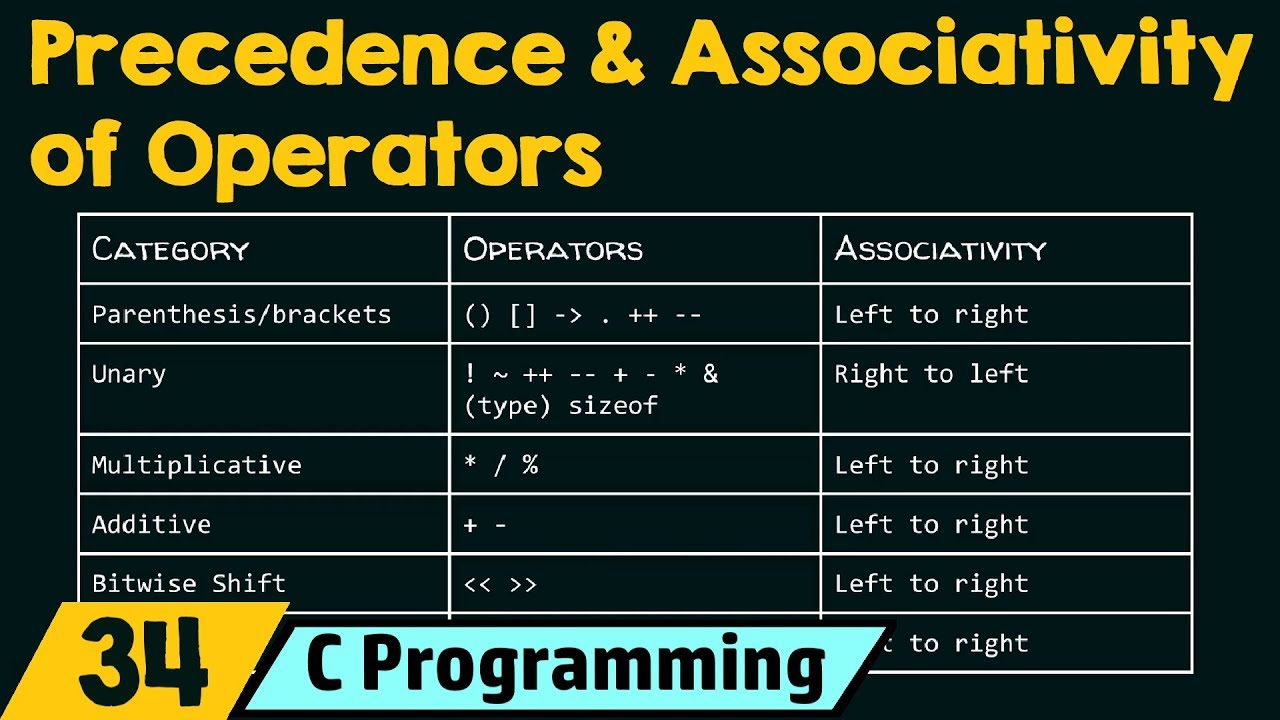
Precedence and Associativity of Operators
5.0 / 5 (0 votes)