Asynchronous Programming in C# Explained (Task.Run, Task.WaitAll, Async and Await)
Summary
TLDRThis script explains asynchronous programming using a relatable analogy of moving house and doing chores. It contrasts synchronous and asynchronous programming with C# examples, demonstrating how tasks can run in parallel without waiting for each other. The script further clarifies scenarios where tasks depend on each other, using 'Task.WaitAll' and 'await' keywords to synchronize. It also touches on the practical use of callbacks and async/await for a more straightforward, synchronous-like coding experience in asynchronous programming.
Takeaways
- 🏠 **Asynchronous Programming Defined**: Asynchronous programming allows for tasks to be performed without waiting for the completion of other tasks, similar to how you can pack while your house is being cleaned.
- 💻 **Synchronous vs Asynchronous**: Synchronous tasks must be completed in a specific order, whereas asynchronous tasks can be done in parallel without waiting for each other.
- 🛠️ **C# Implementation**: In C#, asynchronous programming is implemented using tasks, which can be run concurrently without blocking the main thread.
- 🔄 **Task.Run Usage**: `Task.Run` is used in C# to execute functions asynchronously, allowing for non-blocking code execution.
- 🔄 **Simulating Long-Running Tasks**: The `Sleep` method is used in C# to simulate long-running tasks, which is useful for demonstrating asynchronous behavior.
- 🔄 **Order of Execution**: The order of task execution in asynchronous programming can vary, as seen with the different outputs when running tasks concurrently.
- 🔗 **Dependency Management**: When tasks depend on each other, `Task.WaitAll` or `Task.WaitAny` can be used to ensure that dependent tasks are completed before proceeding.
- 🔄 **Callbacks in Asynchronous Programming**: Callbacks are used in C# to handle the results of asynchronous tasks once they are completed.
- 🔄 **Async and Await Keywords**: The `async` and `await` keywords in C# are used to simplify asynchronous programming, making it appear synchronous while still running tasks in parallel.
- 🔄 **Control Flow with Async/Await**: Using `async` and `await` allows developers to write asynchronous code that looks like synchronous code, maintaining a clear sequence of operations.
- 🔄 **Static Methods and Async**: Static methods can also be made asynchronous by using the `async` keyword, allowing for non-blocking calls within the method.
Q & A
What is asynchronous programming?
-Asynchronous programming is a programming paradigm where tasks can be executed concurrently without waiting for the completion of other tasks. It allows for tasks that are independent of each other to run in parallel, improving efficiency and performance.
How does the analogy of moving houses relate to asynchronous programming?
-The analogy of moving houses is used to illustrate the concept of asynchronous programming. Just as you can start packing your belongings while your new house is being cleaned or painted, asynchronous programming allows you to start new tasks while others are still running, without waiting for them to complete.
What is synchronous programming in the context of the script?
-Synchronous programming refers to the execution of tasks in a sequence where one task must complete before the next one begins. In the script, it is used to contrast with asynchronous programming, where tasks can be executed concurrently.
How is asynchronous programming implemented in C#?
-In C#, asynchronous programming is implemented using the `Task` class and the `async` and `await` keywords. The `Task` class allows for the execution of operations on separate threads, while `async` and `await` provide a way to write asynchronous code that looks and behaves more like synchronous code.
What is the purpose of the `Task.Run` method in C#?
-The `Task.Run` method is used to execute a method asynchronously. It allows the calling code to continue executing without waiting for the task to complete, which can improve the responsiveness of an application.
Why is the `await` keyword used in C#?
-The `await` keyword is used to pause the execution of the current method until the awaited task is completed. It allows for the continuation of the method after the awaited task has finished, providing a way to handle asynchronous operations in a more synchronous-like manner.
What is the difference between `Task.WaitAll` and `Task.WaitAny` in C#?
-`Task.WaitAll` is used to wait for all specified tasks to complete, while `Task.WaitAny` waits for any of the specified tasks to complete. In the script, `WaitAll` is used when a task depends on the completion of multiple other tasks.
How can you handle dependencies between tasks in C#?
-Dependencies between tasks can be handled by waiting for the completion of prerequisite tasks before starting a dependent task. This can be done using `await` to wait for the task to complete and then using its result as needed.
What is a callback function in the context of asynchronous programming?
-A callback function is a function that is called after another function has completed its execution. In the context of asynchronous programming, it is used to handle the result of an asynchronous operation once it has completed.
How does the `async` keyword simplify asynchronous programming in C#?
-The `async` keyword allows you to write asynchronous code that looks and behaves more like synchronous code. It enables the use of `await`, which allows you to pause the method execution until an awaited task is completed, making the code easier to read and maintain.
What is the significance of the order of task execution in the script?
-The order of task execution in the script demonstrates the non-deterministic nature of asynchronous programming. Since tasks can run concurrently, the order in which they complete and their results are printed can vary, depending on the duration of each task.
Outlines
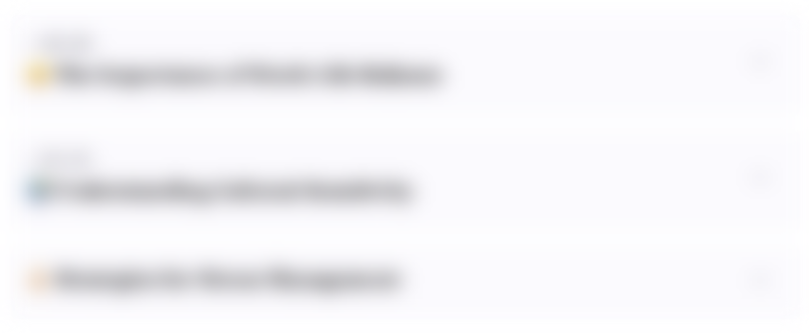
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
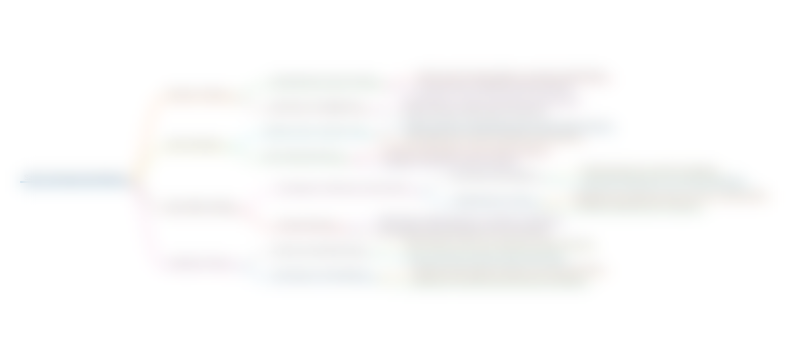
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
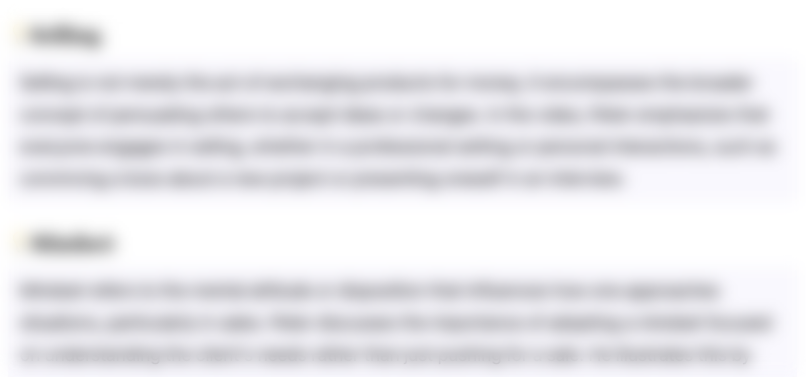
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
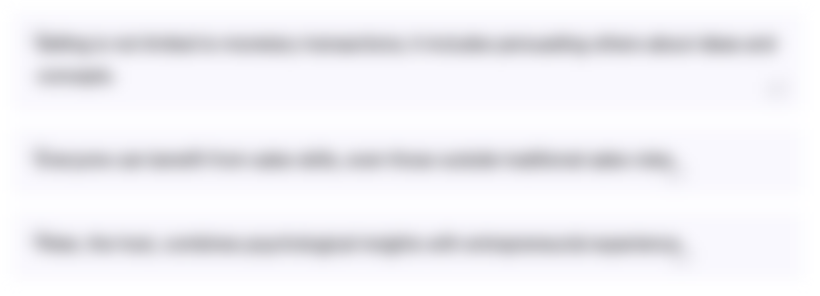
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
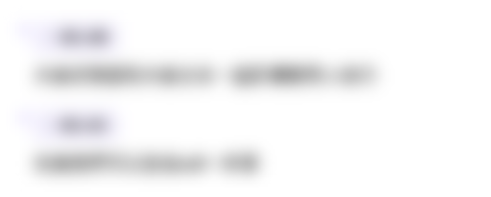
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
5.0 / 5 (0 votes)