How to use RxJS with React Hooks
Summary
TLDREn este video tutorial, exploramos el uso de RxJS en combinación con React Hooks, asumiendo un conocimiento previo de React. Comenzamos con una introducción a RxJS y los Observables, destacando su capacidad para manejar múltiples valores a lo largo del tiempo, a diferencia de las promesas. Después, nos sumergimos en ejemplos prácticos usando CodeSandbox, donde implementamos Observables básicos y los integramos en componentes de React, primero con clases y luego con Hooks para una sintaxis más limpia y menos verbosa. Finalmente, aplicamos estos conceptos en un ejemplo real de búsqueda de sugerencias usando la API de Pokémon, demostrando la potencia y eficiencia de RxJS para el manejo de eventos y datos asincrónicos en aplicaciones React.
Takeaways
- 😀 RxJS es una biblioteca de extensiones reactivas para JavaScript que permite la programación reactiva utilizando observables.
- 🤔 Los observables son colecciones invocables de futuros valores o eventos que pueden enviar múltiples valores a lo largo del tiempo, a diferencia de las promesas que solo se resuelven una vez.
- 🚀 RxJS permite la ejecución perezosa, lo que significa que las operaciones complejas no se ejecutan hasta que se suscriben a ellos.
- ✨ Los operadores de RxJS permiten transformar y componer observables, lo que brinda una forma poderosa de procesar datos asincrónicos.
- 🎣 Puedes utilizar RxJS con React Hooks para manejar flujos de datos asincrónicos y reactivos de una manera más limpia y declarativa.
- 🧩 Los Hooks personalizados, como useObservable, pueden encapsular la lógica de suscripción y cancelación, lo que facilita el uso de observables en componentes de React.
- 🔍 RxJS es especialmente útil para implementar casos de uso como búsquedas con sugerencias automáticas, donde se necesita manejar flujos de datos asincrónicos y aplicar operadores como debounce y distinctUntilChanged.
- 🧪 Los Subjects de RxJS actúan como observables ejecutables, lo que permite compartir datos y eventos entre múltiples observables.
- 🌍 Existen bibliotecas de terceros, como rxjs-hooks, que simplifican aún más el uso de RxJS con React Hooks.
- 📚 RxJS es una biblioteca madura y ampliamente utilizada, con una gran comunidad y recursos disponibles para aprender y profundizar en su uso.
Outlines
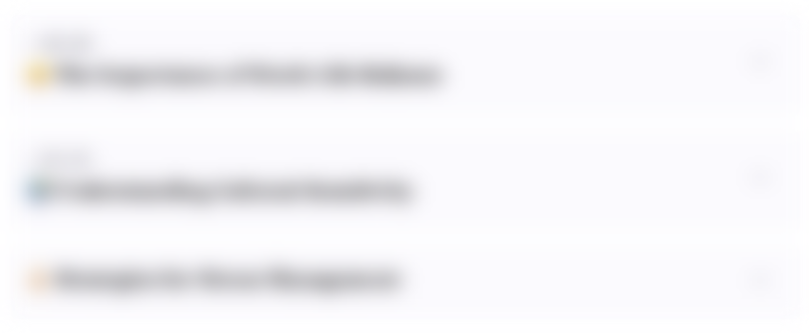
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraMindmap
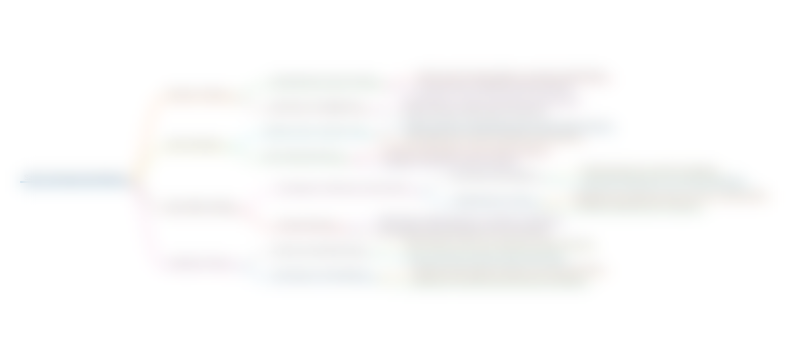
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraKeywords
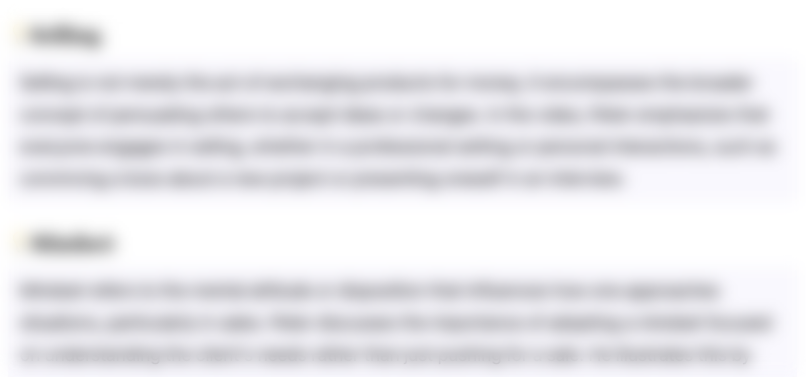
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraHighlights
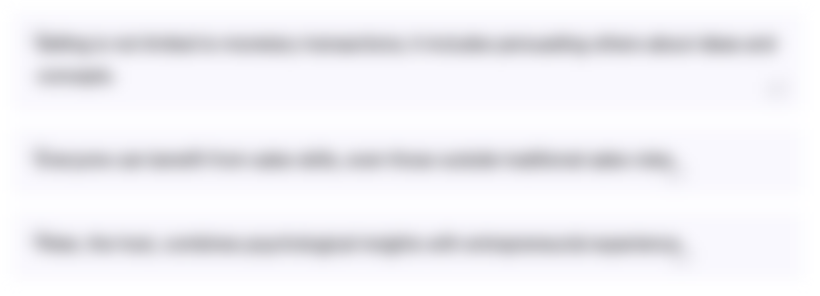
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraTranscripts
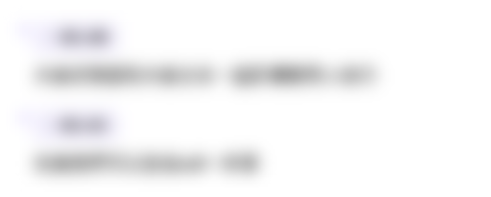
Esta sección está disponible solo para usuarios con suscripción. Por favor, mejora tu plan para acceder a esta parte.
Mejorar ahoraVer Más Videos Relacionados
5.0 / 5 (0 votes)