Inheritance in Python | Python Tutorials for Beginners #lec89
Summary
TLDRThis video introduces the concept of inheritance in Python, explaining its importance in object-oriented programming. The instructor discusses how inheritance allows a class (child class) to acquire attributes and methods from another class (parent class), similar to how humans inherit traits from their parents. The video highlights the efficiency inheritance brings, reducing redundant code and increasing maintainability. Key points include class syntax, method overriding, and accessing parent class methods using the `super()` function. The session concludes by emphasizing how inheritance promotes code reusability, clarity, and customization in Python programming.
Takeaways
- 🧬 Inheritance in Python is analogous to inheriting traits from parents in real life, where properties, appearances, and behaviors are passed down.
- 🏗️ In object-oriented programming, inheritance allows a class (child/derived) to inherit attributes and methods from another class (parent/base/super), promoting code reusability.
- 🔑 The base class is the class being inherited from, while the derived class is the new class that inherits properties and behaviors.
- 📝 The syntax for inheritance in Python involves using the `class` keyword, followed by the derived class name and the base class name in parentheses.
- 🛠️ Inheritance reduces redundancy by allowing methods and attributes from an existing class to be used in a new class without rewriting them.
- 🔄 Derived classes can have their own unique attributes and methods, in addition to those inherited from the base class.
- 🔧 Method overriding allows a derived class to provide a specific implementation of a method that is already defined in the base class.
- 🔄 The `super()` function is used in Python to access methods and attributes of the base class, including calling the base class's constructor.
- 🔑 If a derived class defines its own constructor, it must call the base class's constructor using `super().__init__()` to ensure proper initialization.
- 📈 Inheritance enhances code maintainability and readability by allowing for a clear hierarchy and structure in the codebase, making it easier to manage and understand.
Q & A
What is inheritance in Python?
-Inheritance in Python is the ability of a class to inherit properties, methods, and attributes from another class. The class from which properties are inherited is called the base or parent class, while the class that inherits is called the derived or child class.
Why do we use inheritance in Python?
-Inheritance is used to increase the reusability of code. Instead of writing duplicate code in different classes, a derived class can inherit methods and attributes from a base class, making the code more efficient, readable, and maintainable.
How does inheritance relate to real life?
-In real life, inheritance refers to inheriting traits or properties from parents, like physical appearance or behavior. In programming, inheritance allows one class to inherit properties and behaviors (methods and attributes) from another class, similar to how a child inherits traits from parents.
What is the basic syntax for inheritance in Python?
-The syntax for inheritance in Python involves defining a derived class and passing the base class as a parameter within parentheses. For example: `class DerivedClass(BaseClass):` followed by the class definition.
What are the benefits of using inheritance in large-scale projects?
-In large-scale projects, inheritance helps save time by reducing redundancy, lowering the number of lines of code, improving readability, and enhancing maintainability. It also promotes code reuse, which is vital for managing large projects with multiple classes.
What is method overriding in Python?
-Method overriding occurs when a derived class defines its own version of a method that is already defined in its base class. This allows the derived class to provide specific behavior for the method while keeping the same method name.
How can you call the base class method from a derived class in Python?
-You can call the base class method from a derived class by using the `super()` function. This function gives access to the methods and attributes of the base class, allowing the derived class to extend or modify the behavior of the base class method.
What is the purpose of the 'super()' function in inheritance?
-The `super()` function in Python is used to call methods or access attributes from the base class. It allows the derived class to inherit functionality from the base class without redefining it and can be used to extend or add new behavior to existing methods.
Can a derived class have its own attributes and methods in Python?
-Yes, a derived class can have its own attributes and methods in addition to the ones it inherits from the base class. This allows the derived class to add specific functionality or properties while still using the inherited features.
What happens if a derived class defines an '__init__' method but also wants to use the base class's '__init__' method?
-If a derived class defines its own `__init__` method but also wants to use the base class's `__init__`, it needs to call the base class's `__init__` method using `super().__init__()`. This ensures that the base class’s initialization is also performed.
Outlines
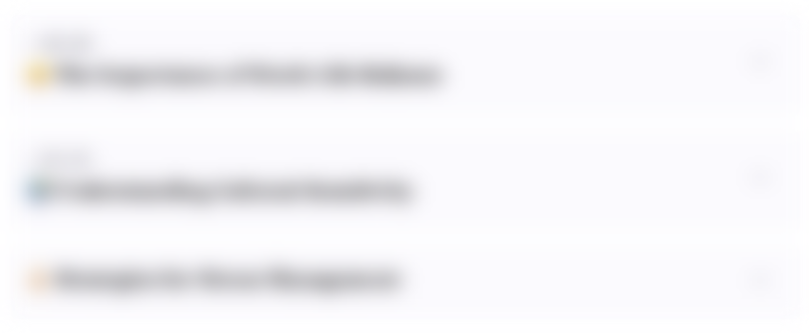
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenMindmap
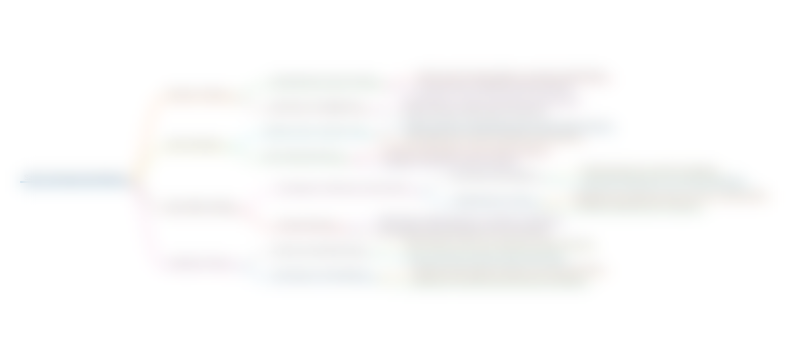
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenKeywords
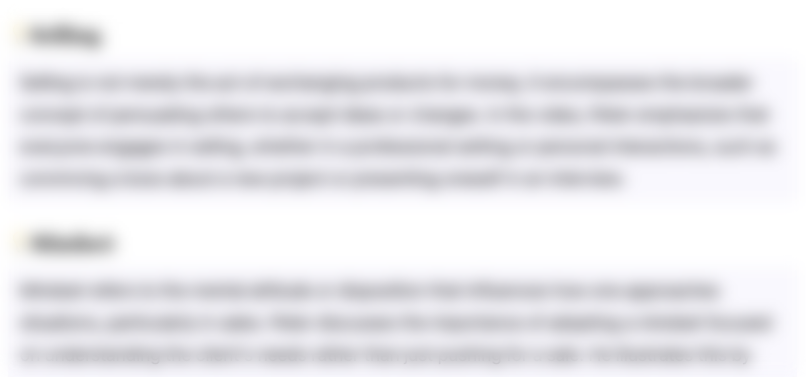
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenHighlights
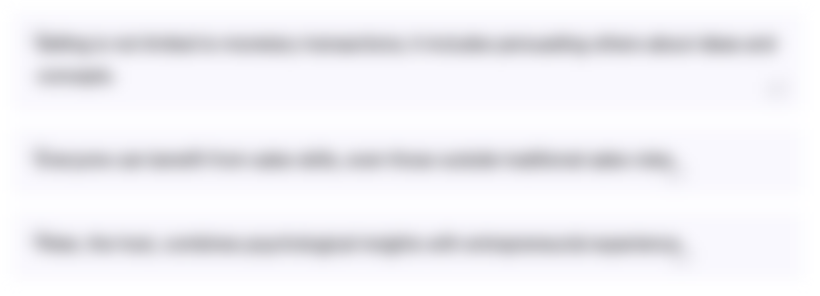
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenTranscripts
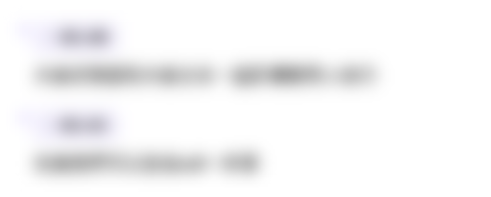
Dieser Bereich ist nur für Premium-Benutzer verfügbar. Bitte führen Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchführenWeitere ähnliche Videos ansehen
5.0 / 5 (0 votes)