Java inheritance ๐ช
Summary
TLDRIn this tutorial on Java inheritance, the instructor explains how one class can inherit attributes and methods from another. Using the example of vehicles, cars, and bicycles, the video demonstrates how inheritance reduces redundancy by allowing child classes to reuse code from a parent class. Key concepts such as creating subclasses, using 'extends' to inherit features, and adding unique attributes to each class are covered. The tutorial also highlights the benefits of inheritance, such as code reusability and maintainability, with practical Java code examples to reinforce the concepts.
Takeaways
- ๐ Inheritance in Java allows one class to acquire the attributes and methods of another class, reducing code duplication.
- ๐ The 'extends' keyword is used to create a subclass that inherits from a superclass, allowing access to its methods and attributes.
- ๐ A superclass (parent class) provides common functionality to its subclasses (child classes), which can then use or modify that functionality.
- ๐ The car and bicycle classes in the example inherit common attributes like 'speed', and methods like 'go' and 'stop', from the vehicle superclass.
- ๐ Even though the car and bicycle classes don't list their own methods or attributes, they still have access to those from the vehicle class due to inheritance.
- ๐ You can define unique attributes in subclasses, like 'wheels' for both car and bicycle, and 'doors' for the car class, which are not inherited from the superclass.
- ๐ The car class and the bicycle class can have their own unique behavior and attributes, while still sharing common attributes (e.g., speed) from the vehicle class.
- ๐ Inheritance helps avoid redundant code by allowing subclasses to automatically inherit common properties and methods from a superclass.
- ๐ The main advantage of using inheritance is that it allows for the reuse of code, making the program more modular and easier to maintain.
- ๐ Inheritance is useful when you have multiple classes that share common functionality but also require unique attributes or behavior, like cars and bicycles.
Q & A
What is inheritance in Java?
-Inheritance in Java is the process by which one class acquires the attributes and methods of another class. This allows subclasses to reuse code from a superclass without needing to redefine it.
How does inheritance help avoid code duplication?
-By using inheritance, subclasses can inherit methods and attributes from a superclass, preventing the need to repeat the same code in each subclass. This reduces redundancy and enhances maintainability.
What does the 'extends' keyword do in Java?
-The 'extends' keyword in Java is used to define a subclass that inherits the attributes and methods from a superclass. It tells Java that the class being defined is a child class of the specified superclass.
What are the terms 'superclass' and 'subclass' in inheritance?
-'Superclass' refers to the parent class that provides attributes and methods to other classes. 'Subclass' refers to the child class that inherits from the superclass.
What is an example of a superclass and subclass in the script?
-In the script, 'Vehicle' is the superclass, and 'Car' and 'Bicycle' are subclasses that inherit attributes and methods from the 'Vehicle' class.
What methods and attributes are inherited by the 'Car' and 'Bicycle' classes?
-The 'Car' and 'Bicycle' classes inherit the 'speed' attribute and the 'go' and 'stop' methods from the 'Vehicle' class.
How can subclasses add their own unique attributes in Java?
-Subclasses can add their own unique attributes by defining them within the subclass. For example, the 'Car' class can have an attribute for 'doors', and the 'Bicycle' class can have an attribute for 'pedals'. These attributes are specific to each subclass.
Why are the 'go' and 'stop' methods available in both the 'Car' and 'Bicycle' classes?
-The 'Car' and 'Bicycle' classes inherit the 'go' and 'stop' methods from the 'Vehicle' class, which is why they can access and use these methods even though they are not defined directly in the 'Car' or 'Bicycle' classes.
Can you access attributes or methods that are not part of the subclass?
-No, you cannot access attributes or methods that are not part of the subclass. For example, the 'Car' class cannot access the 'pedals' attribute, and the 'Bicycle' class cannot access the 'doors' attribute because these are not part of their respective classes.
What are the benefits of using inheritance in object-oriented programming?
-Inheritance promotes code reusability, reduces redundancy, and allows for more efficient and manageable code. It enables developers to create more specialized classes while maintaining a shared base of functionality in the superclass.
Outlines
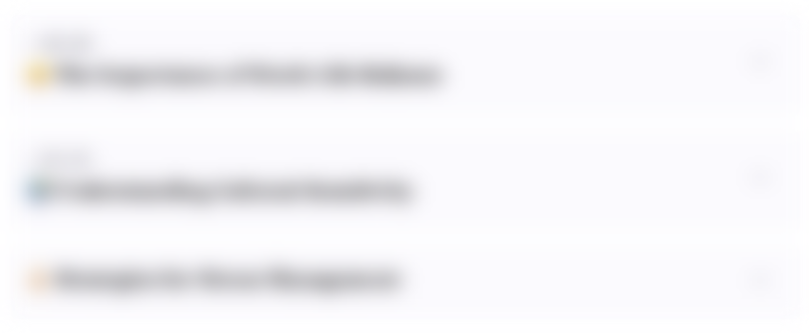
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
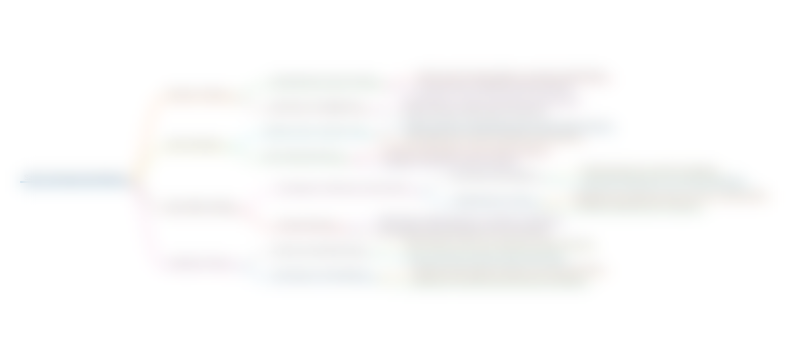
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
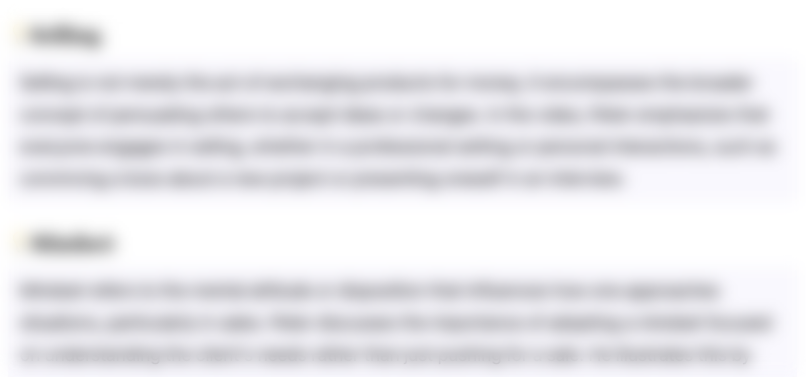
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
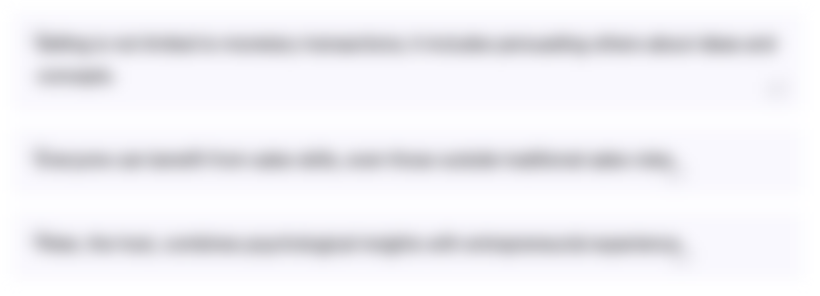
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
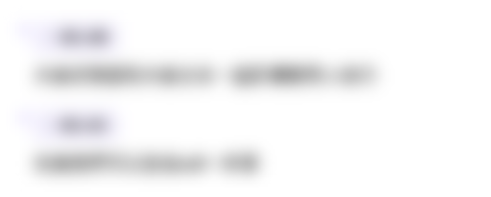
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Belajar Java [OOP] - 22 - Latihan Inheritance
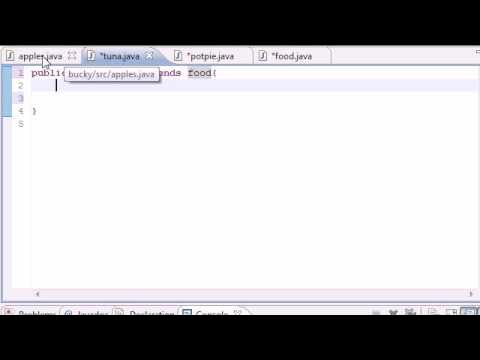
Java Programming Tutorial - 49 - Inheritance
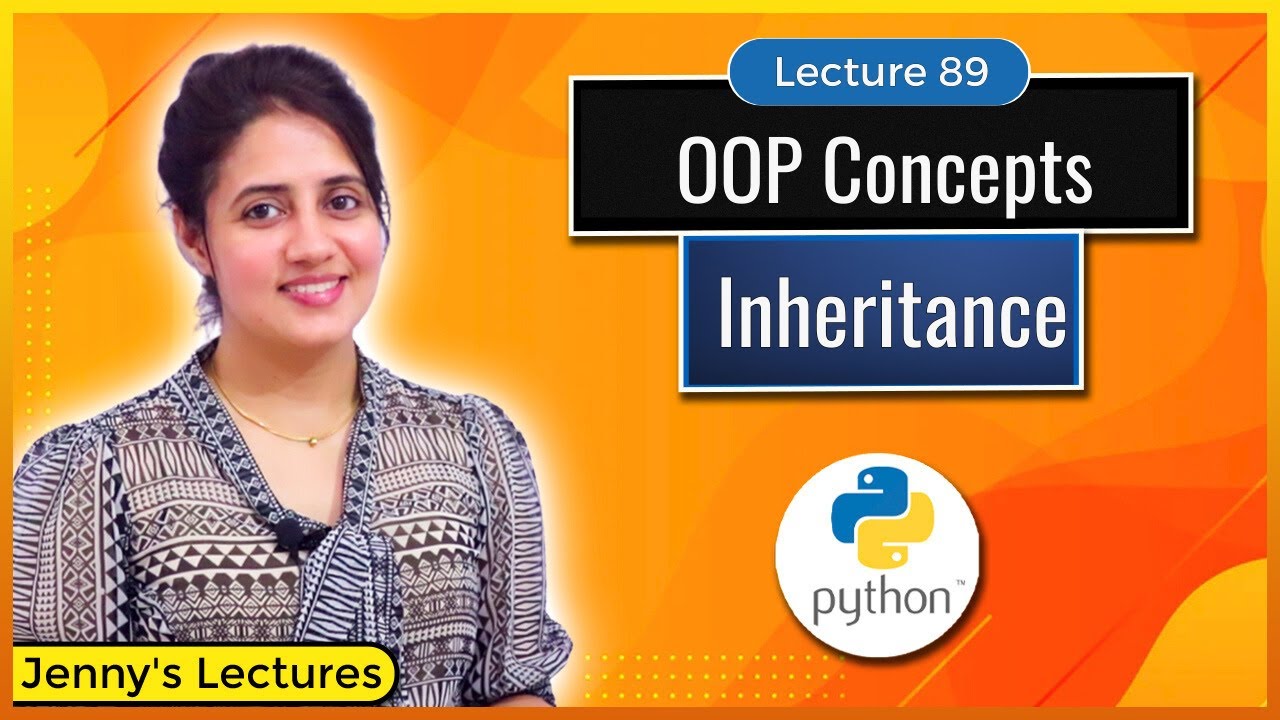
Inheritance in Python | Python Tutorials for Beginners #lec89
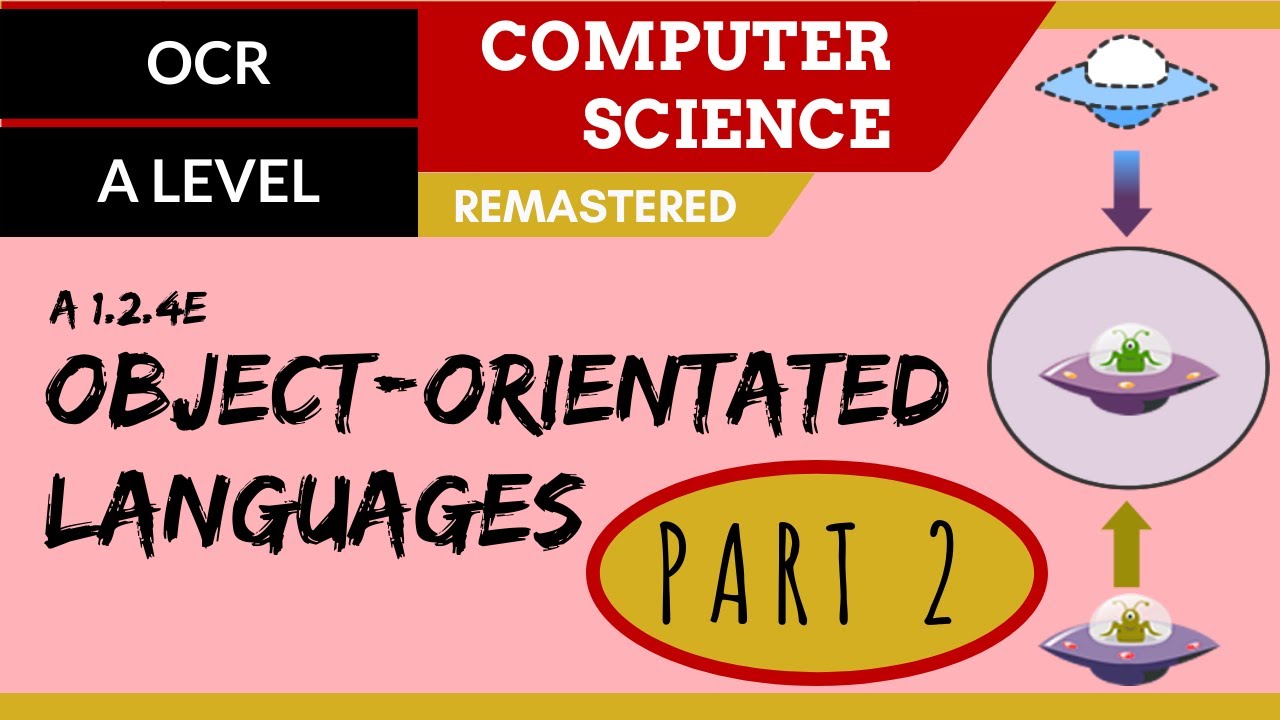
37. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 2

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn
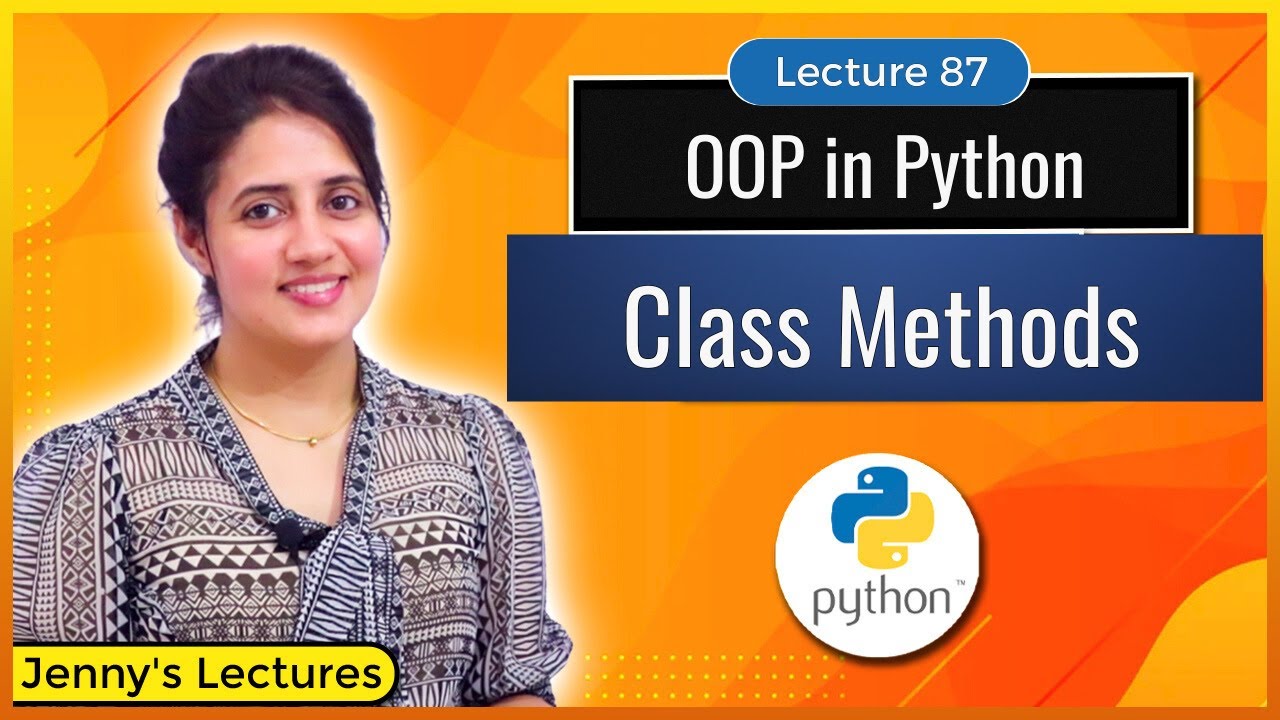
Class Methods in Python | How to add Methods in Class | Python Tutorials for Beginners #lec87
5.0 / 5 (0 votes)