#56 Python Tutorial for Beginners | Constructor in Inheritance
Summary
TLDRIn this video, Ivan 20 explains key concepts of inheritance in Python, focusing on constructors and method resolution order (MRO). The tutorial covers how constructors behave when inheriting from one or multiple classes, and how Python determines which constructor to call. The 'super()' method is introduced, demonstrating how it helps call parent class methods, even in cases of multiple inheritance. Ivan also highlights how MRO works, ensuring Python resolves methods from left to right when dealing with multiple inherited classes. This tutorial deepens the understanding of object-oriented programming in Python.
Takeaways
- 👨💻 Inheritance allows one class (B) to inherit features from another class (A), enabling code reuse.
- 🧩 Multiple inheritance occurs when a class inherits from two or more classes, such as Class C inheriting from both Class A and Class B.
- 🏗️ Constructors in inheritance: Creating an object of class B can call the constructor of class A even if B has its own constructor.
- 🔄 If both Class A and B have their own constructors, B's constructor will be called when an object of B is created, unless explicitly specified otherwise.
- ⚙️ The `super()` method is used to call the parent class's constructor or other methods within a subclass.
- 📜 If Class C inherits from both A and B, the `super()` method will call the constructor of the class listed first in the inheritance chain (left to right).
- 📐 Method Resolution Order (MRO) determines the sequence in which inherited methods are called in a class hierarchy.
- ⚡ MRO always follows a left-to-right approach when dealing with multiple inheritance scenarios.
- 📝 The `super()` method can also be used to call any other methods from the parent class, not just constructors.
- 🔄 If two classes have methods with the same name, the method from the class on the left side of the inheritance hierarchy will be called first.
Q & A
What is inheritance in Python?
-Inheritance in Python allows a class (subclass) to inherit methods and properties from another class (superclass). This helps in reusing the features of an existing class without rewriting code.
How does multiple inheritance work in Python?
-Multiple inheritance occurs when a class inherits from more than one parent class. For instance, Class C can inherit features from both Class A and Class B. This allows Class C to access the methods and properties of both classes.
What happens if both parent and child classes have their own constructors?
-If both the parent and child classes define their own `__init__` constructors, the child class's constructor will override the parent's constructor by default. To explicitly call the parent's constructor, the `super()` function is used.
What is the purpose of the `super()` function in inheritance?
-The `super()` function allows the child class to call methods from its parent class. It is commonly used to call the parent's `__init__` method within the child class, ensuring both the child and parent constructors are executed.
What is Method Resolution Order (MRO) in Python?
-MRO defines the order in which base classes are searched when looking for a method or attribute. In multiple inheritance, Python looks for methods from left to right in the class definition. This ensures a predictable order of inheritance.
What happens if multiple classes in an inheritance hierarchy have methods with the same name?
-If multiple parent classes have methods with the same name, Python will follow the MRO and execute the method from the first class in the hierarchy. For example, if Class C inherits from Class A and Class B, it will use the method from Class A first.
How does constructor inheritance work in Python?
-When an object is created from a child class that does not have its own constructor, the constructor of the parent class is automatically called. If the child class has its own constructor, the parent's constructor can still be called using `super()`.
Can `super()` be used to call methods other than constructors?
-Yes, `super()` can be used to call any method from a parent class, not just the constructor. This allows a child class to reuse methods from the parent class without directly accessing them.
What is the default behavior when creating an object in a class with multiple inheritance?
-When creating an object in a class with multiple inheritance, the constructor of the first parent class (based on the MRO) is called by default unless `super()` is used to call constructors of other parent classes.
How can you manually check the Method Resolution Order (MRO) of a class?
-You can check the Method Resolution Order (MRO) of a class using the `mro()` method. For example, `ClassC.mro()` will return a list of classes in the order they will be searched for methods.
Outlines
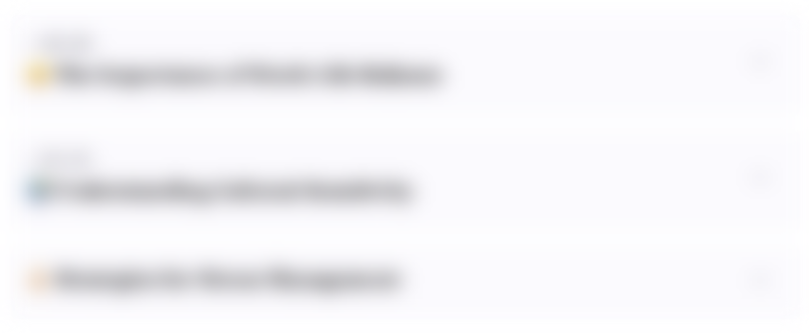
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
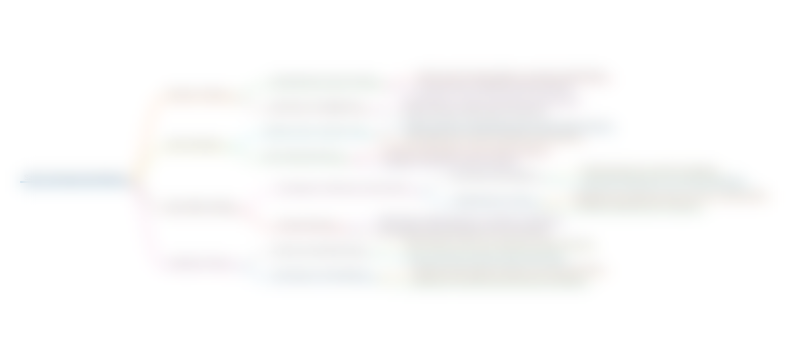
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
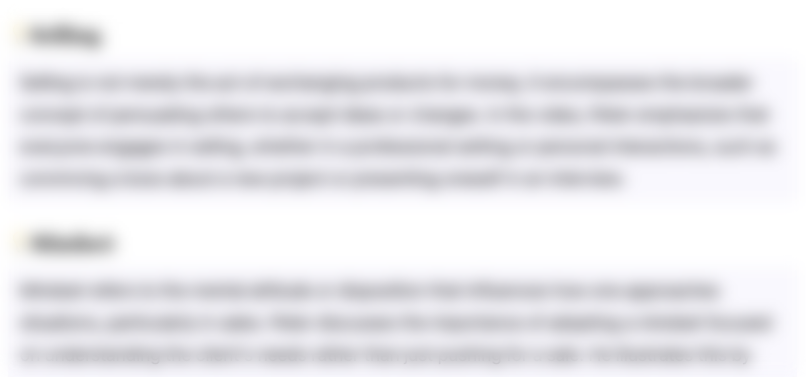
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
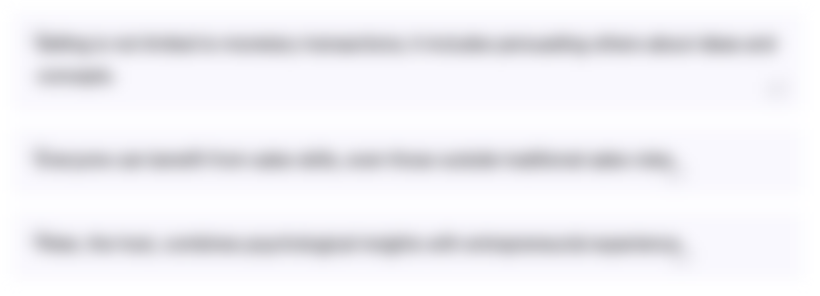
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
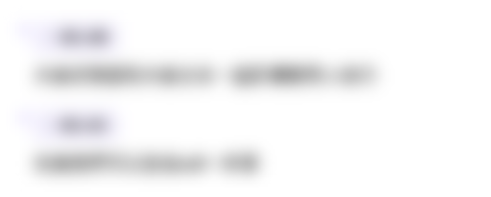
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
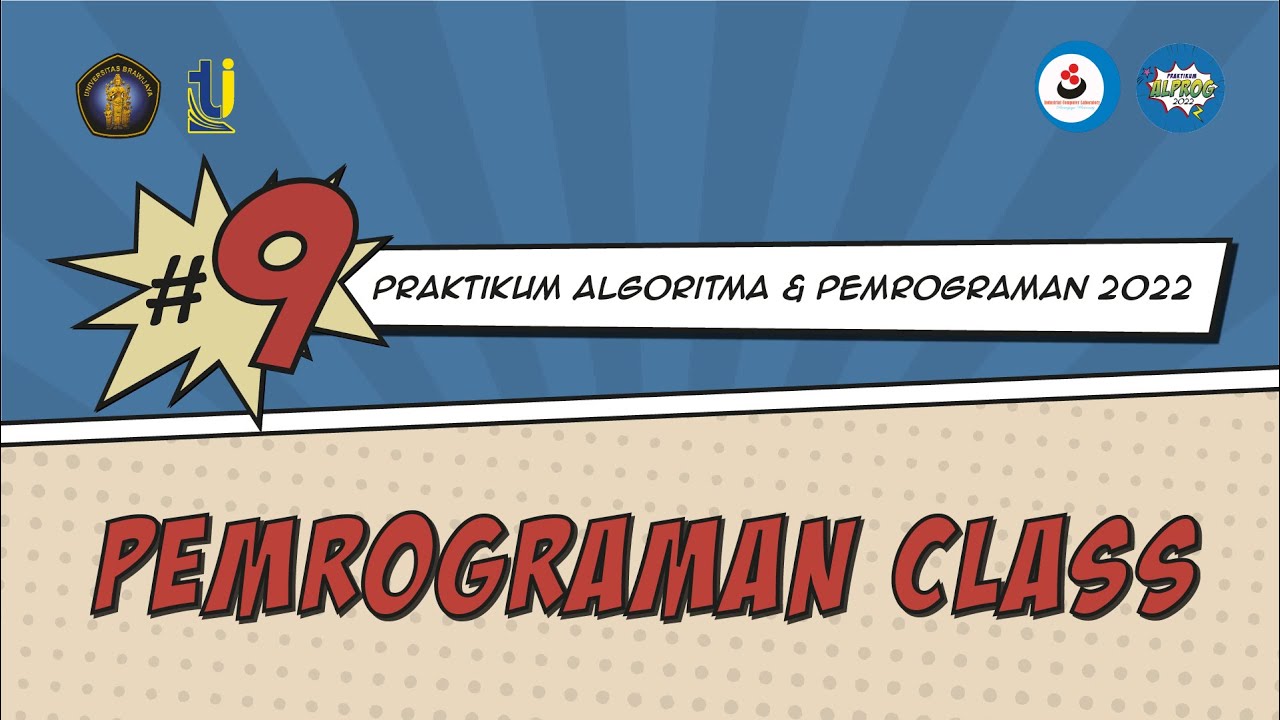
9. Pemrograman Class - Praktikum Algoritma dan Pemrograman 2022

SUPER() in Python explained! 🔴
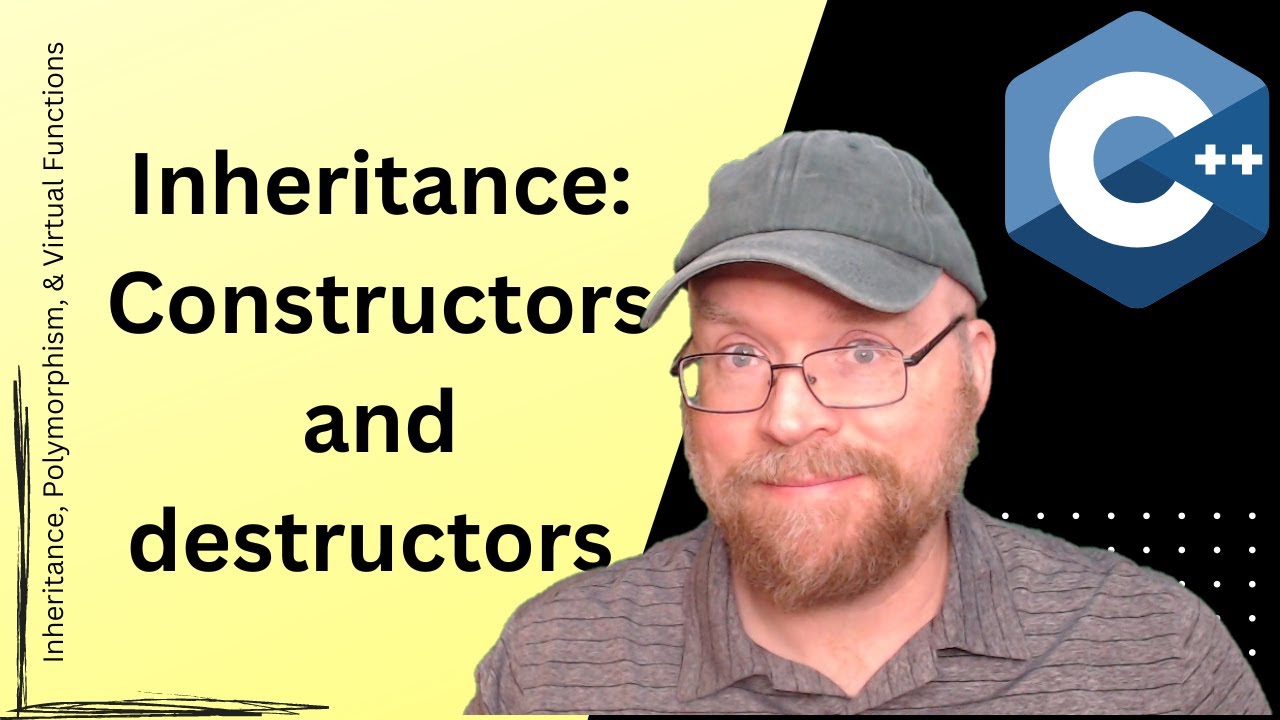
C++ Inheritance: constructors and destructors in base and derived classes [3]
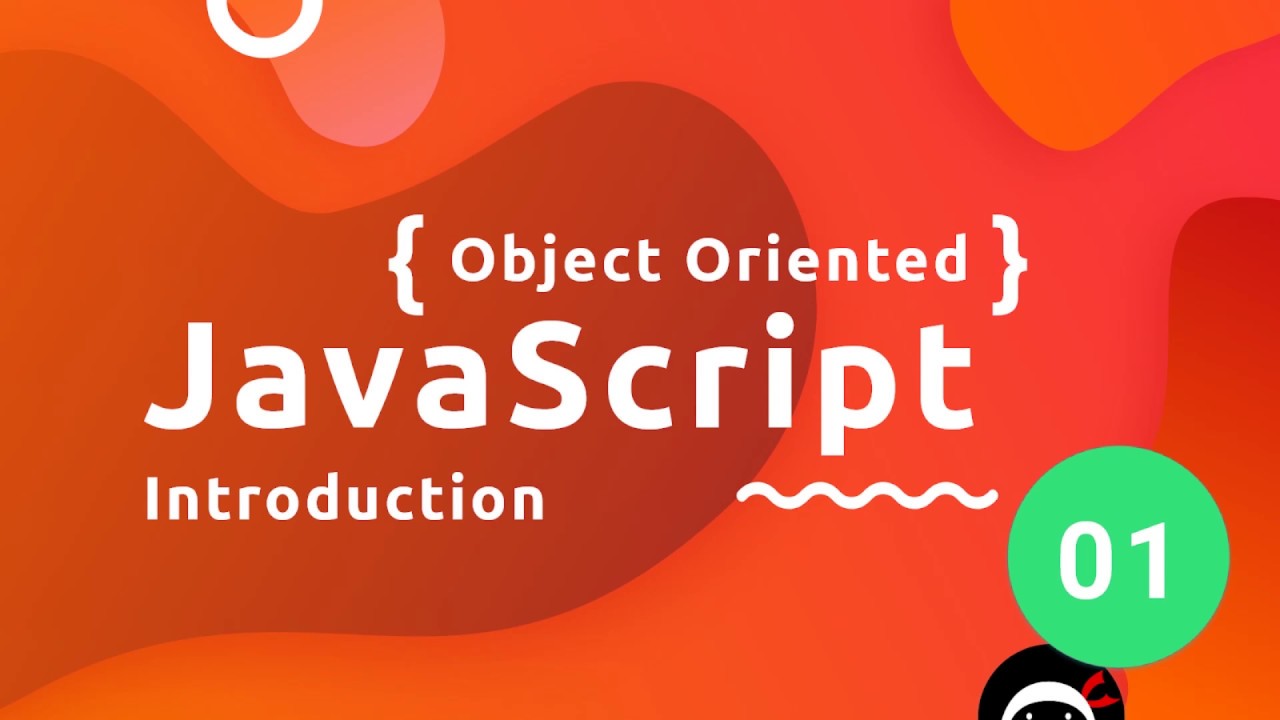
Object Oriented JavaScript Tutorial #1 - Introduction
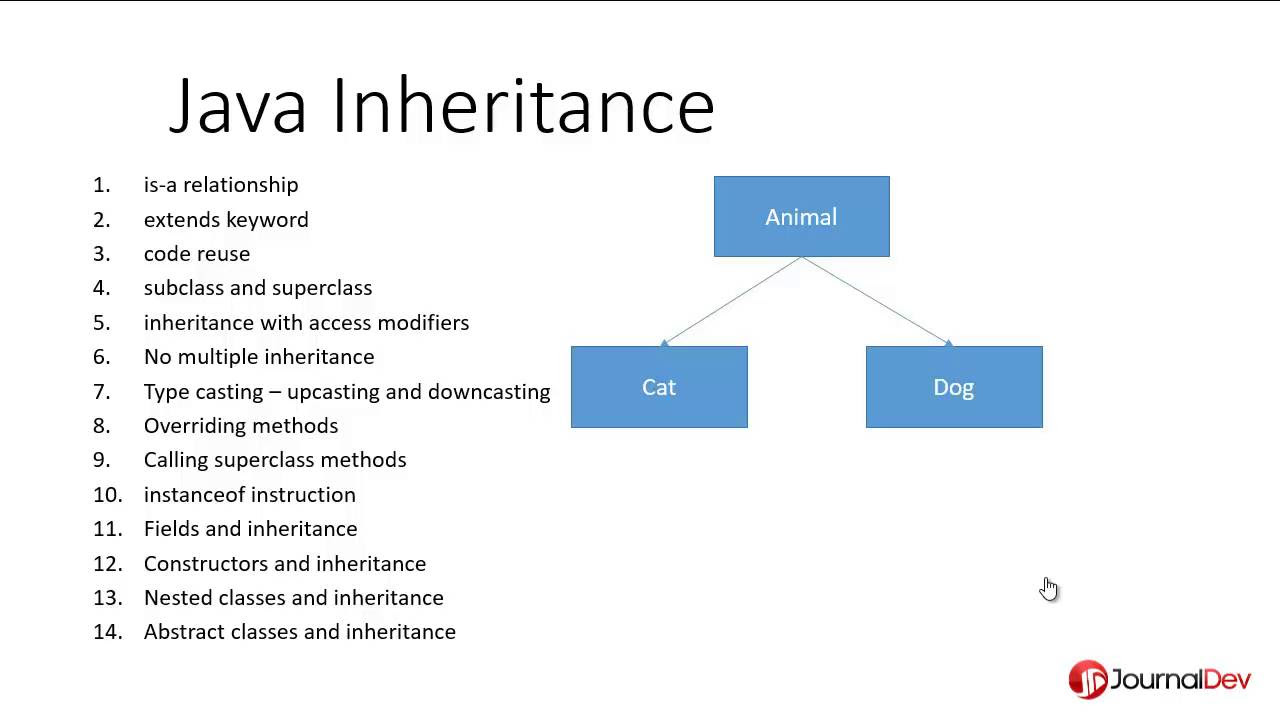
Inheritance in Java - Java Inheritance Tutorial - Part 2
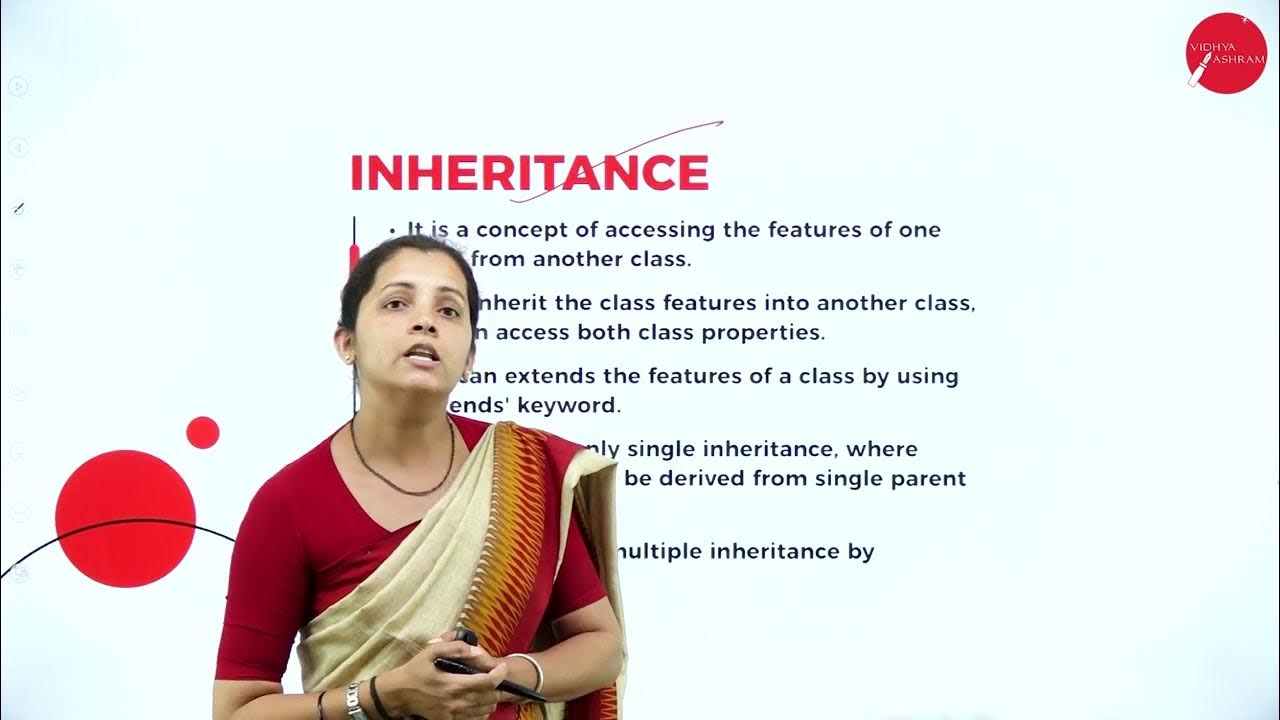
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
5.0 / 5 (0 votes)