SUPER() in Python explained! π΄
Summary
TLDRThis video script explains the concept and utility of the 'super' function in Python, which is instrumental in a child class to invoke methods from a parent class. It demonstrates the function's role in avoiding code redundancy by utilizing inheritance, showcasing how to define constructors and methods in a 'Shape' parent class and its child classes like 'Circle', 'Square', and 'Triangle'. The script also illustrates method overriding and extending functionality of inherited methods using 'super', providing practical examples of object instantiation and method calls to clarify these concepts.
Takeaways
- π The 'super()' function in Python is used to call methods from a parent class within a child class, promoting code reusability.
- π¨βπ§ The child class is often referred to as a 'subclass', and the parent class as the 'superclass', which is the origin of the 'super' function's name.
- π 'super()' allows for extending the functionality of inherited methods, which can be useful for method overriding or extending.
- π The script provides an example using shape classes like Circle, Square, and Triangle, demonstrating how attributes like color and fill status can be inherited.
- π§ The use of 'super()' eliminates the need to manually assign common attributes in each child class constructor, streamlining the code.
- ποΈ By placing shared attributes in a parent class, any changes to these attributes need to be made only once, reducing the potential for errors.
- π The script illustrates how to use 'super()' to call the parent class constructor, passing necessary arguments to initialize child class objects.
- π¨ It demonstrates object instantiation with attributes like color, fill status, and specific shape dimensions, using the 'super()' function for clarity.
- π The 'super()' function can also be used to extend the functionality of a method, as shown with the 'describe' method in the shape classes.
- π οΈ Method overriding is shown where a child class has a similar method to the parent class, and the child's version is used instead of the parent's.
- π The script concludes by emphasizing the utility of 'super()' in both constructors for attribute assignment and in methods for functionality extension.
Q & A
What is the purpose of the 'super' function in Python?
-The 'super' function in Python is used within a child class to call methods from a parent class. It allows for the extension of functionality of inherited methods and helps in reusing code to avoid redundancy.
What is the term used for the class that inherits from another class?
-The class that inherits from another is called a 'subclass' or 'child class', while the class from which it inherits is referred to as the 'superclass' or 'parent class'.
What are some attributes that might be common among different shape classes like Circle, Square, and Triangle?
-Common attributes among shape classes could include 'color' and 'is filled', which are shared by all shapes regardless of their specific geometric properties.
How does the script suggest handling attributes that are unique to each shape class?
-The script suggests defining unique attributes like 'radius' for Circle, 'width' for Square, and 'width' and 'height' for Triangle within their respective class constructors after calling the superclass constructor with 'super'.
What is the advantage of using 'super' in the constructors of child classes?
-Using 'super' in the constructors of child classes allows the child class to inherit the initialization of common attributes from the parent class, reducing the need for repetitive code and potential for errors.
Can you provide an example of how to instantiate a Circle object with the given script?
-A Circle object can be instantiated by calling `Circle(color='red', is_filled=True, radius=5)`, which passes the color, fill status, and radius to the Circle class constructor.
What is the purpose of the 'describe' method in the script?
-The 'describe' method is used to print out the attributes of a shape, such as its color and whether it is filled or not. It can also be overridden or extended in child classes to include additional information specific to the shape type.
How does method overwriting work in the context of the script?
-Method overwriting occurs when a child class defines a method with the same name as a method in the parent class. In such cases, the child class's version of the method is used instead of the parent's.
What is the significance of using 'super' to extend the functionality of a method in a child class?
-Using 'super' to extend the functionality of a method allows the child class to call the parent class's method and then add additional functionality on top of it, combining the behaviors of both methods.
Can you explain how the script demonstrates the concept of inheritance and method overriding with the shape classes?
-The script demonstrates inheritance by having Circle, Square, and Triangle classes inherit common attributes from a Shape parent class. Method overriding is shown by redefining the 'describe' method in each child class to include specific attributes like area calculations, while still being able to call the parent class's 'describe' method using 'super'.
What is the practical application of using 'super' for both constructors and methods in Python classes?
-Using 'super' for constructors ensures that common attributes are initialized properly across all subclasses, while using it for methods allows for extending or modifying inherited behavior without losing the functionality of the parent class, promoting code reusability and maintainability.
Outlines
π Introduction to Python's Super Function
This paragraph introduces the concept of the 'super' function in Python, which is used in child classes to call methods from a parent class. It explains the terminology 'sub-class' and 'super-class' and how the 'super' function helps in extending the functionality of inherited methods. An example is given to illustrate creating shape objects like Circle, Square, and Triangle, and how they can share common attributes such as 'color' and 'filled'. The paragraph further discusses the redundancy in manually assigning these attributes in each class constructor and highlights the benefits of using inheritance and the 'super' function to avoid repetitive code.
π Utilizing Super for Constructor Inheritance
The second paragraph delves into the practical application of the 'super' function by demonstrating how to use it in the constructors of child classes to inherit and initialize attributes from a parent class. It shows how to create a 'Shape' parent class with common attributes 'color' and 'is_filled', and how child classes like 'Circle', 'Square', and 'Triangle' can inherit these attributes by calling the 'super' function within their constructors. The paragraph also covers the instantiation of objects for each shape, using both positional and keyword arguments for clarity, and confirms that the 'super' function successfully assigns the inherited attributes.
π Method Overwriting and Extension with Super
The final paragraph discusses advanced uses of the 'super' function, including method overwriting and extending the functionality of inherited methods. It explains how a child class can have a method with the same name as the parent class, and in such cases, the child's method will be used. The paragraph provides an example of adding a 'describe' method to the 'Shape' class and then extending this method in the 'Circle', 'Square', and 'Triangle' classes to include specific calculations for the area of each shape. It also demonstrates how to use the 'super' function to call the parent class's 'describe' method and then add additional functionality, thus combining the inherited behavior with new features.
Mindmap
Keywords
π‘super function
π‘child class
π‘parent class
π‘inheritance
π‘constructor
π‘attributes
π‘method overwriting
π‘method extending
π‘F-string
π‘object instantiation
Highlights
Introduction to the 'super' function in Python, which allows a child class to call methods from a parent class.
Explanation of the term 'super' as derived from 'super class' and 'sub class'.
Demonstration of creating shape objects with unique attributes like color, filled status, and specific geometric properties.
The importance of constructors in initializing objects with attributes.
Identifying common attributes among different shape classes to avoid code repetition.
The concept of inheritance and how it simplifies code maintenance by centralizing shared attributes.
Using the 'super' function to call the parent class constructor within child class constructors.
Example of creating a 'Circle' object with color, filled status, and radius, showcasing the use of 'super'.
Creating a 'Square' object emphasizing the use of 'super' for shared attributes and unique attributes like width.
Constructing a 'Triangle' object, highlighting the use of 'super' and specific attributes like width and height.
The practicality of using 'super' to avoid manual attribute assignment in each child class.
Introduction of a 'describe' method in the 'Shape' class to demonstrate method functionality.
Method overwriting explained through the creation of a 'describe' method in child classes.
Extending the functionality of a parent class method using 'super' in child class methods.
Calculating and printing the area of a 'Circle' using the overridden 'describe' method.
Describing a 'Square' with an area calculation in its 'describe' method, showcasing method extension.
Describing a 'Triangle' with an area calculation, further illustrating method extension and overwriting.
The 'super' function's role in simplifying the extension and maintenance of inherited methods.
Transcripts
hey everybody so today I got to talk
about the super function in Python super
is a function it's used within a child
class to call methods from a parent
class the child class is the subass the
parent class is the super class hence
why this function is named the super
function using the super function it
allows you to extend the functionality
of the inherited methods here's an
example we'll create a few shape objects
we'll need to set up the classes though
we'll have class
Circle for the time being I'll just
write pass we'll fill it in later class
square and
class
triangle for each of these classes in
order to instantiate objects we'll need
a Constructor we will Define our
Constructor our init
method when creating circles what sorts
of attributes should a circle have let's
say a color what's the color of the
circle is it filled or not filled will
be another attribute and a
radius then let's assign
these self. color equals the color that
we receive
self. filed equals
filled self. radius equals
radius let's do this with the square and
triangle really I'll just copy our
Constructor and paste it squares don't
have a radius with a square the width
and the height are the same let's
replace radius with width we'll also
keep the color and filled attributes
self. width equals width now with
triangles again let's copy our
Constructor we'll need a width and a
height self. height equals
height so with programming we try not to
repeat ourselves if we don't have to
what do all of these classes have in
common they all share the attributes of
color and
filled the ways in which they are
different is that Circle has a radius
attribute square has a width triangle
has a width and a height if we have to
make any changes to one of these
attributes we would have to do so
manually for example let's replace
filled with isil
now I need to look throughout my code
for any instance of filled and replace
it with is
filled it's a lot of work and I might
make a
mistake such as here and here it's
better to write your code once and try
and reuse it so that's where inheritance
and the super function can come in handy
we're going to take the attributes of
color and is filled and place it within
app parent class these children classes
will inherit those
attributes so class what do they all
have in common they're all shapes class
shape and for now I'll write pass circle
is going to inherit from its parent of
shape that also applies with square and
triangle we'll set up a Constructor for
shape Define
init we will pass in the color and is
filled then we will assign these add
attributes self. color equals
color self. is filled equals is filled
we don't need to manually assign these
attributes within each of these
Constructors for the
children instead what we have to do is
within the Constructor for each of these
children classes we have to call the
Constructor for the parent also known as
the super class of shape so we will
eliminate these two lines of
code use the super
function dot call The Constructor of the
parent that is the dunder init method
but we need to pass in the color that we
receive and is filled this will be a
Boolean and let's do this with the
square
class and the triangle
class we still need radius for the
circle width for the square width and
height for the triangle we're going to
call the super function to take care of
whatever our attributes all these types
of shapes have in common such as color
and is filled now let's see if this
works let's construct a few objects we
will create a circle named
Circle call The Constructor for Circle
we have to pass in a color a Boolean if
it's filled or not and a radius so for
the color of the circle let's say
red is filled let's say that is true and
a radius of five you could even use
keyword arguments for better readability
although not necessary but for clarity
let's say color equals
red is filled equals
true radius equals
5 let's see if this works I will print
our circles
color it is red
print our colors is filled
attribute the circle is filled that is
true and the
radius print
circle. rius the radius of the circle is
five we could even convert this to an F
string I'll add a
placeholder then add centimeters
5 cm let's construct a square
object square equals
Square we'll need a color is filled and
a width I'll just copy what we have and
make a few changes replace radius with
width the color will be blue is filled
will be false the width will be six we
don't need a height because squares have
an even width and height if we ever need
the height we can assume it's the same
as the width in this case six let's
check out our square square do color
Square do is filled Square do
width our square is blue it's not filled
in the width is 6
cm let's create a triangle object
triangle equals
triangle pass in our
arguments the color will be yellow is
filled will be true
the width will be seven and the height
will be
eight let's print our triangle's color
is it filled its width and its
height our triangle is yellow it's
filled in the width is 7 cm the height
is 8
cm so that's how you can use the super
function to reuse the Constructor of a
parent CL class we don't need to
manually assign each of these attributes
within each of the children classes we
can do that in just one place when we
refer to Super imagine that we're
replacing this with the parent class
name such as
shape that might be a good way to think
of
it use the Constructor of the parent
class of shape and pass these arguments
in what you could do as well is extend
the functionality of a method So within
our shape class let's create a method of
describe we will describe the attributes
of this shape we will
print use an FST string when we want to
describe our shape let's say it is at a
placeholder self. color what is the
color of this shape and is it fil or not
and add a placeholder we'll use a tary
operator print filled
if self. is filled is true else we will
print not
filled each of these types of shapes
circle square and triangle will have
access to a describe
method let's attempt to use
it take our Circle use the describe
method that's
inherited it is red and filled Square
it is blue and not filled
triangle it is yellow and
filled so then we also have method
overwriting what if we create a similar
method of describe within circle square
and triangle let's do
that
Define a describe
method within our Circle let's calculate
the area what's the area of the circle
I'll use an F string it is a
circle with an area of then we'll
calculate the area given the
radius to calculate the area of a circle
we can take Pi I'll just say 3.14 just
to keep it simple times the radius
squared self. rius time self.
rius if I were to call the describe
method will we use the parents version
of describe
or the
child so let's take our Circle use the
describe
method the
result it is a circle with an area of
78.5 I should really add cenim squar
after
that cm
squared this is called method
overwriting if a child shares a similar
method with a parent you'll use the
child's version and not the parents this
is Method overwriting if you would like
to extend the functionality of a method
from a parent you can use the super
function not only do I want to use the
describe method of the child I would
also like to use the describe method of
the parent So within this function we
will use the super function access the
describe method of the
parent what we're doing is extending the
functionality of the describe
method if it is a circle with an area of
78.5 cm squared the circle is red and
it's
filled or you can change up the
order let's use the parent classes
describe method and extend the
functionality with our own print
statement it is red and filled it is a
circle with an area of 78.5 cm squared
let's finish this with the square and
triangle classes I'll copy what we have
for the described method Within the
circle
class but we'll make a different
calculation describe the square it is a
square with an area of take self. width
times self.
width the height and the width are going
to be the same if it's a
square then describe our
triangle it is a triangle
with an area of width time height we
have a height in this case divided two
we've already described our Circle let's
describe our
Square it is a square with an area of 36
CM squar it is blue and not filled let's
describe our
triangle it is a triangle with an area
of 28.0 CM squar it is yellow and
filled all right everybody that is the
super function it's used in a child
class to call the methods from a parent
class also known as the super class it
allows you to extend the functionality
of the inherited methods within a child
class you could use it within a
Constructor to assign any attributes
that all of its siblings have in common
such as color or if that shape is filled
when used within any other method you
can extend the functionality of that
method not only are we printing this
message from the parent we're tacking on
another PR statement before that and
well everybody that is the super
function in Python
Browse More Related Video
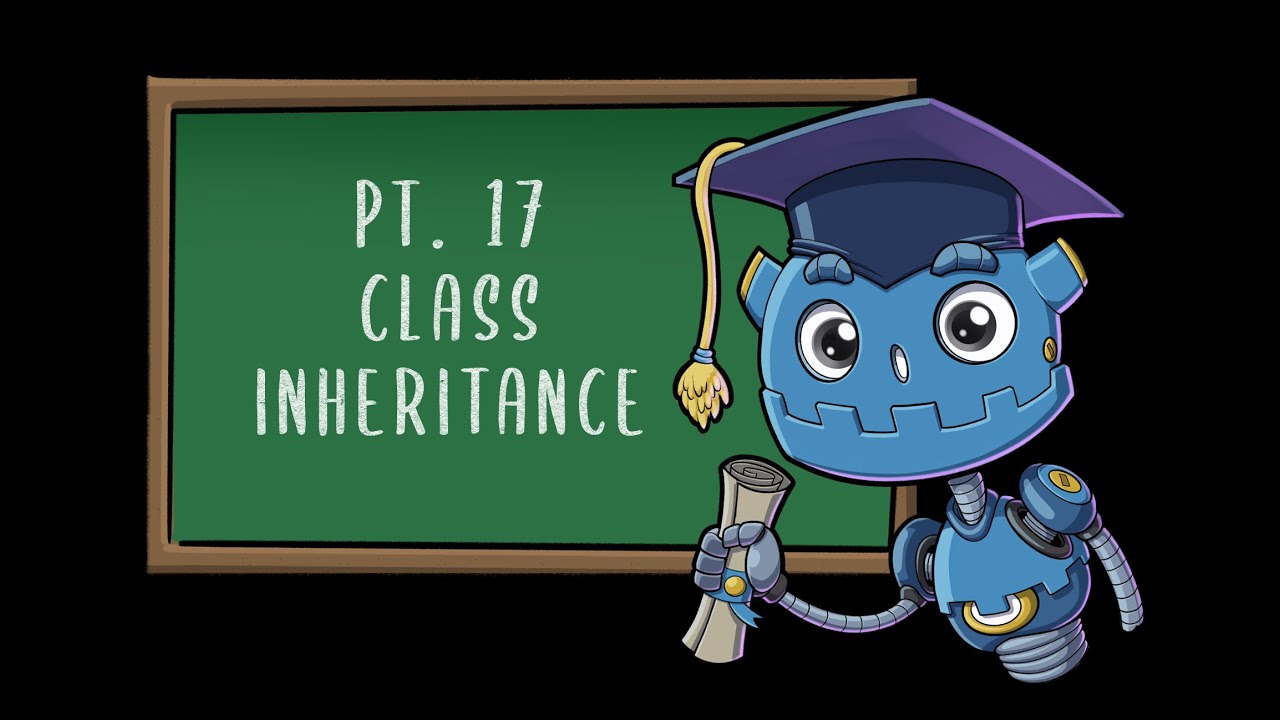
Class Inheritance | Godot GDScript Tutorial | Ep 17

Learn Python OOP in under 20 Minutes

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn

Python OOP Tutorial 1: Classes and Instances
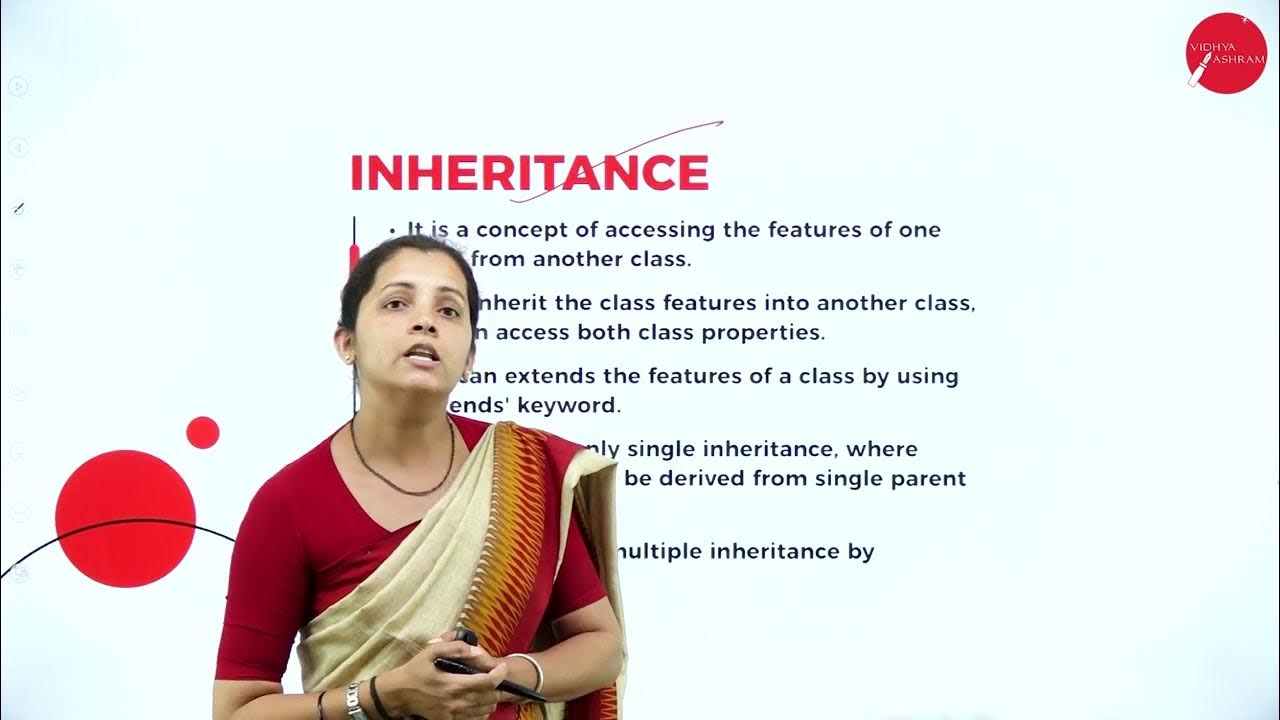
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
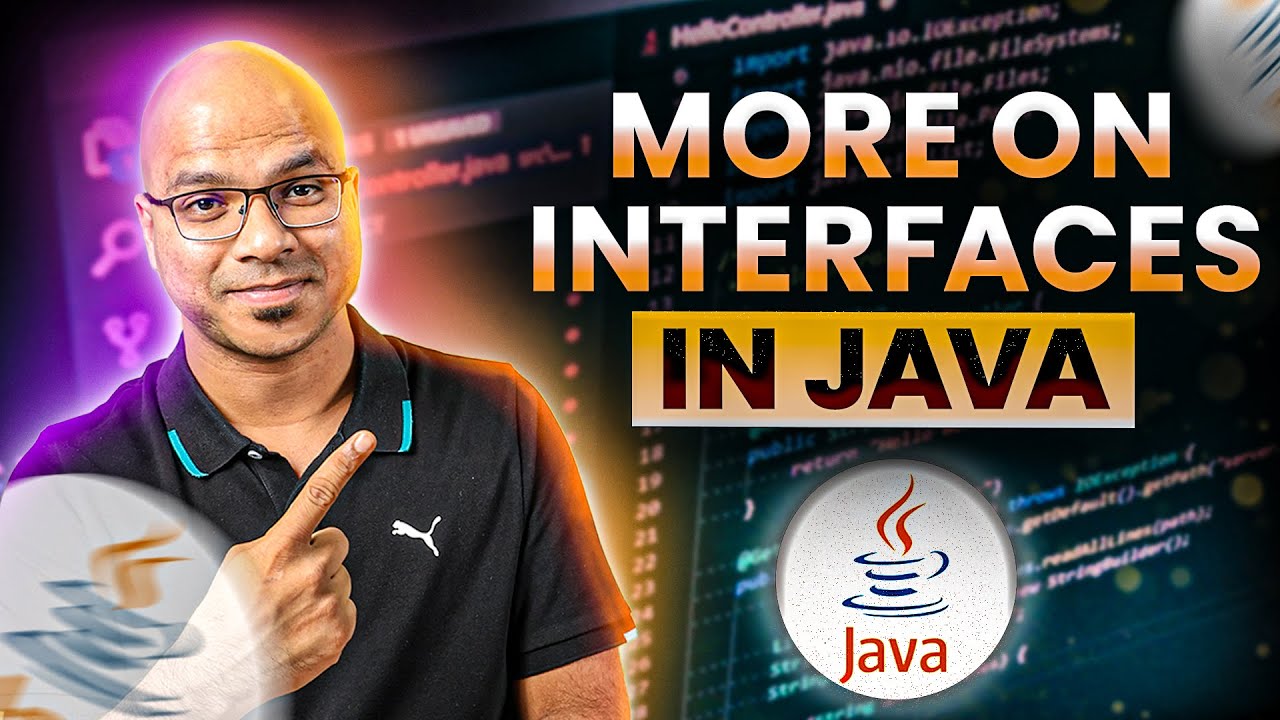
#67 More on Interfaces in Java
5.0 / 5 (0 votes)