Reviewing Functions | JavaScript đ„ | Lecture 034
Summary
TLDRThis comprehensive video lecture delves into the intricacies of functions in programming. It covers the three types of functions: function declarations, function expressions, and arrow functions, highlighting their distinct characteristics and use cases. The lecturer meticulously breaks down the structure of a typical function, explaining the components such as function names, parameters, function bodies, and return statements. Additionally, the video explores the concepts of function invocation, argument passing, and how functions process and output data. Through clear examples and explanations, the lecturer aims to solidify the viewers' understanding of functions, laying a strong foundation for their coding journey.
Takeaways
- đ The script reviews the three types of functions in JavaScript: function declarations, function expressions, and arrow functions. It explains their differences and when to use each.
- đ§© Functions have a structure consisting of a name, parameters (placeholders for input data), a body (where the code logic resides), and often a return statement to output a value.
- đ Calling a function is done by using parentheses after the function name, and passing arguments (actual values) to the parameters.
- đ The return statement immediately terminates the function execution and returns the specified value.
- đ console.log() is a function itself, used for printing messages to the developer console, distinct from returning values from a function.
- âš Functions can receive input data, transform it, and output data, though these steps are optional.
- đ Functions are useful for reusing code logic across different parts of a program.
- đŻ The script emphasizes understanding the data flow and variable scoping within functions.
- đ Parameter names can be reused across different functions without conflict, as they are treated as separate local variables within their respective functions.
- đ The script prepares the learner for an upcoming coding challenge involving writing their first function.
Q & A
What are the three types of functions discussed in the script?
-The three types of functions discussed are: 1) Function declarations, 2) Function expressions, and 3) Arrow functions (which are a special form of function expressions).
What is the main difference between function declarations and function expressions?
-Function declarations can be used before they are declared in the code, while function expressions are essentially function values stored in a variable and cannot be used before they are defined.
What are the advantages of using arrow functions?
-Arrow functions are great for writing quick one-line functions, as they don't require the explicit use of the 'return' keyword or curly braces. They also don't have their own 'this' keyword.
What are the three main operations that functions can perform?
-Functions can receive input data (parameters), transform or process that data (function body), and output data (return statement).
What is the purpose of the 'return' statement in a function?
-The 'return' statement is used to output a value from the function, and it also immediately terminates the function's execution.
How do you call or invoke a function?
-A function is called or invoked by writing its name followed by parentheses, e.g., 'functionName()'.
What is the difference between parameters and arguments in a function?
-Parameters are placeholders for input values in a function declaration, while arguments are the actual values passed into the function when it is called.
What is the purpose of the 'console.log()' function in JavaScript?
-The 'console.log()' function is used to print messages or values to the developer console in the browser. It is a separate function call within another function and is not related to the 'return' statement.
Can you explain the data flow when calling a function from another function?
-When a function is called from within another function, the arguments are first passed to the inner function, which then performs its operations and returns a value. This returned value can then be used or processed further in the outer function.
What is the purpose of the 'if-else' statement used in the script?
-The 'if-else' statement is used to handle different scenarios based on a condition. In the script, it is used to return a specific value (e.g., -1) if the 'retirement' value is less than or equal to zero, indicating that the person has already retired.
Outlines
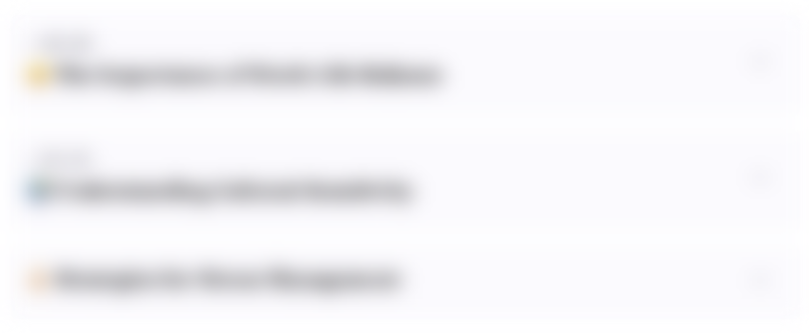
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenMindmap
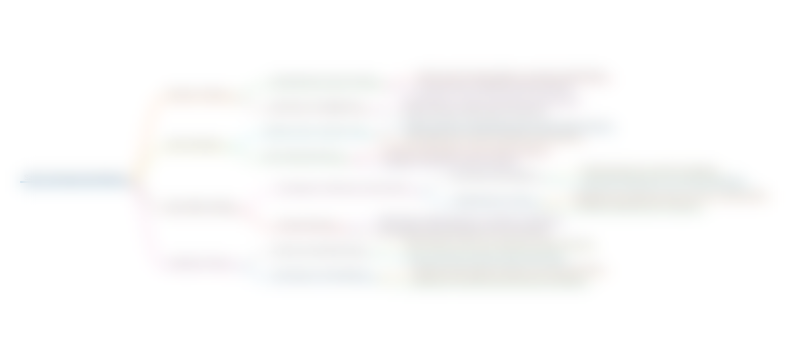
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenKeywords
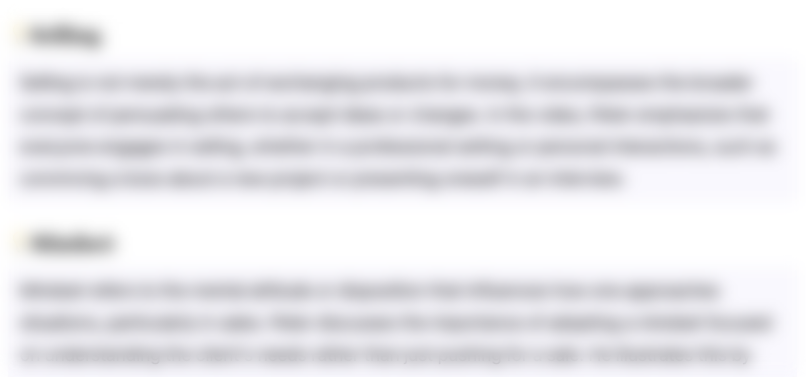
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenHighlights
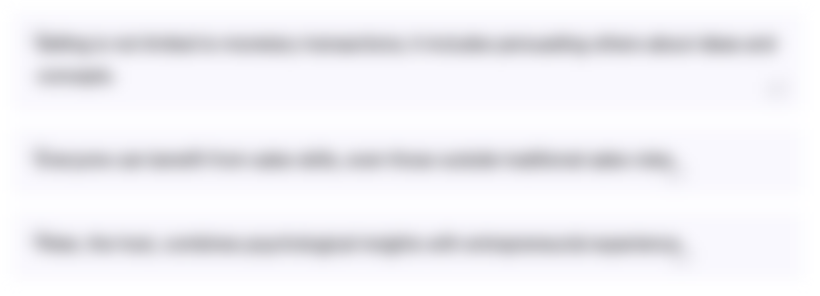
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenTranscripts
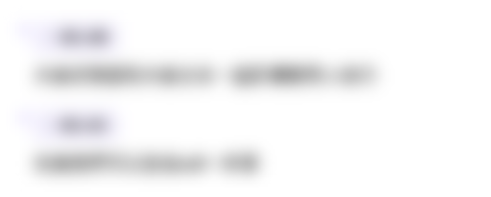
Dieser Bereich ist nur fĂŒr Premium-Benutzer verfĂŒgbar. Bitte fĂŒhren Sie ein Upgrade durch, um auf diesen Abschnitt zuzugreifen.
Upgrade durchfĂŒhrenWeitere Ă€hnliche Videos ansehen
5.0 / 5 (0 votes)