C_64 Strings in C- part 3 | printf and puts function in C
Summary
TLDRThe video script discusses various programming concepts related to strings in C. It covers how to read a string, the use of functions like 'scanf' and 'gets', and their advantages. The script also explains the syntax and function of 'printf' and 'puts' for displaying strings on the screen. It delves into the implementation of these functions, how to handle string input, and the importance of proper formatting. The video aims to educate viewers on the intricacies of string handling in C programming, providing a comprehensive guide for beginners.
Takeaways
- 😀 The video discusses strings in C programming, focusing on how to read, use, and manipulate them.
- 🔍 It covers the use of 'scanf' and 'gets' functions to read strings at runtime, along with their advantages when used together.
- 📝 The 'printf' and 'puts' functions are introduced for printing strings on the screen, with 'puts' being a simpler alternative to 'printf' for single characters.
- 🔑 The syntax and use of 'printf' function are explained, including formatting specifiers and how to declare a string with a size of 30 characters.
- 👉 The importance of using '%s' to read a string and '%c' to read individual characters is highlighted.
- 📚 It explains how to use loops and address operators to print the entire string, including the null character at the end.
- 🎯 The concept of memory allocation for strings is introduced, showing how a string of 30 characters is allocated with a starting address.
- 📌 The video clarifies the difference between 'printf' and 'puts' functions, especially how 'puts' automatically adds a newline at the end of the string.
- 👓 It discusses the use of predefined functions like 'strlen', 'strcat', 'string reverse', and other functions for string manipulation.
- 📝 The video also touches on the implementation of 'printf' and 'puts' functions, explaining that they are pre-declared in the header files.
- 🔄 The script ends with a teaser for the next video, which will continue exploring strings and standard functions like 'strlen' and 'strchr'.
Q & A
What is the main topic discussed in the video?
-The main topic discussed in the video is learning programming with a focus on strings in the C programming language, including how to read, use, and manipulate strings.
What are some of the functions discussed in the video for handling strings in C?
-The video discusses functions like `printf`, `puts`, and `scanf` for handling strings in C, including how to print a string on the screen and how to read user input.
How does the video explain the use of `scanf` for reading strings?
-The video explains that `scanf` can be used to read strings by specifying the format specifier `%s` and providing the name of the string variable where the input will be stored.
What is the purpose of the `printf` function in the context of the video?
-The `printf` function is used to print formatted output to the screen, such as displaying a string or a message with specific formatting.
How does the video describe the process of printing a specific character from a string?
-The video describes that to print a specific character from a string, you can use a loop to iterate through the string and print each character individually using the `printf` function.
What is the difference between `scanf` and `gets` as mentioned in the video?
-The video mentions that `gets` is a function that was used to read strings, but it is not recommended due to security issues. Instead, `scanf` with a format specifier is suggested for safer string input.
How is the `puts` function used in the video to handle strings?
-The `puts` function is used to print a string followed by a newline character to the screen. It is a simpler alternative to `printf` when only a string needs to be displayed.
What is the significance of the format specifier `%s` in the context of string functions?
-The format specifier `%s` is significant as it tells the `scanf` or `printf` function to expect or display a string, respectively.
How does the video explain the concept of string pointers in C?
-The video explains that a string pointer in C points to the first character of a string. It discusses how to use pointers to access and manipulate strings, such as printing a string using a pointer.
What is the importance of null termination in strings as discussed in the video?
-The video emphasizes the importance of null termination in strings, which is the use of the null character (`'\0'`) to mark the end of the string. This is crucial for functions like `printf` to know where the string ends.
How does the video address the issue of buffer overflow when reading strings?
-The video addresses buffer overflow by discussing the use of `scanf` with format specifiers to limit the number of characters read, thus preventing the risk of overwriting memory beyond the string's allocated space.
Outlines
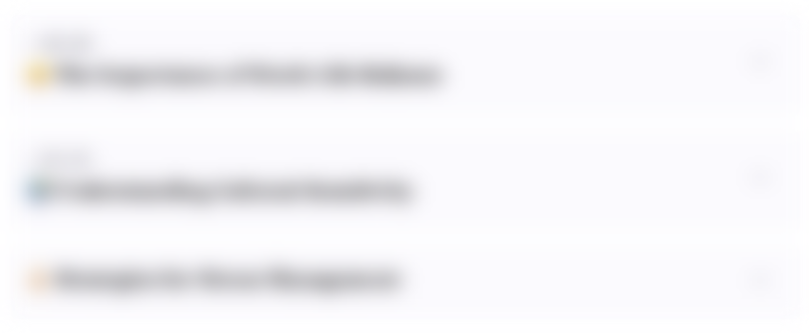
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
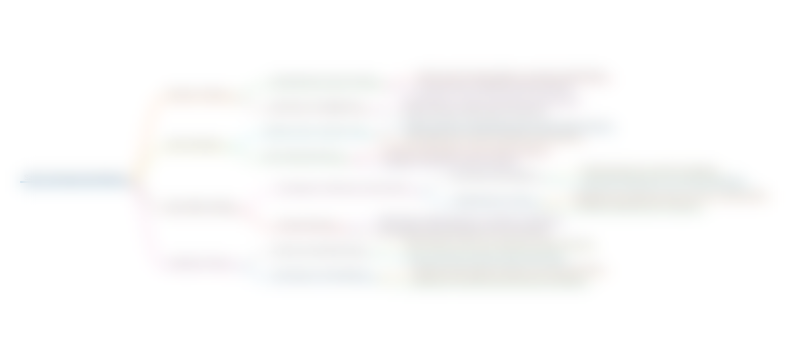
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
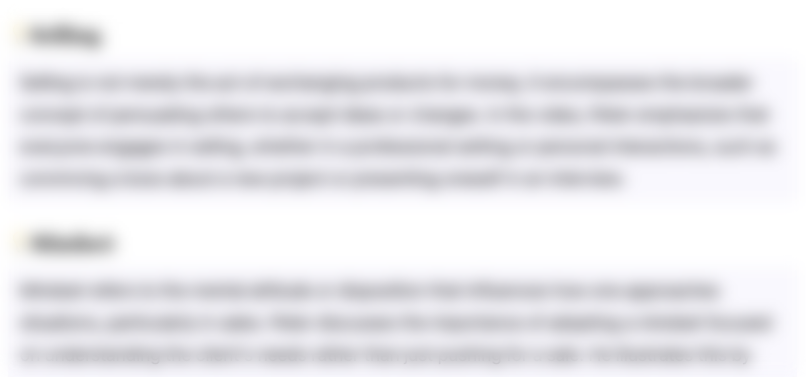
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
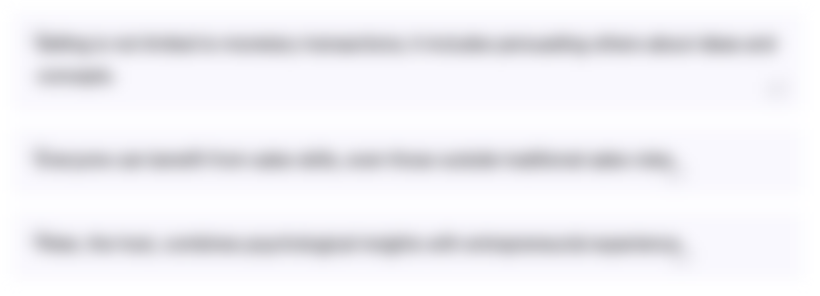
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
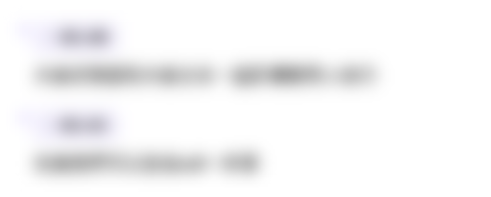
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
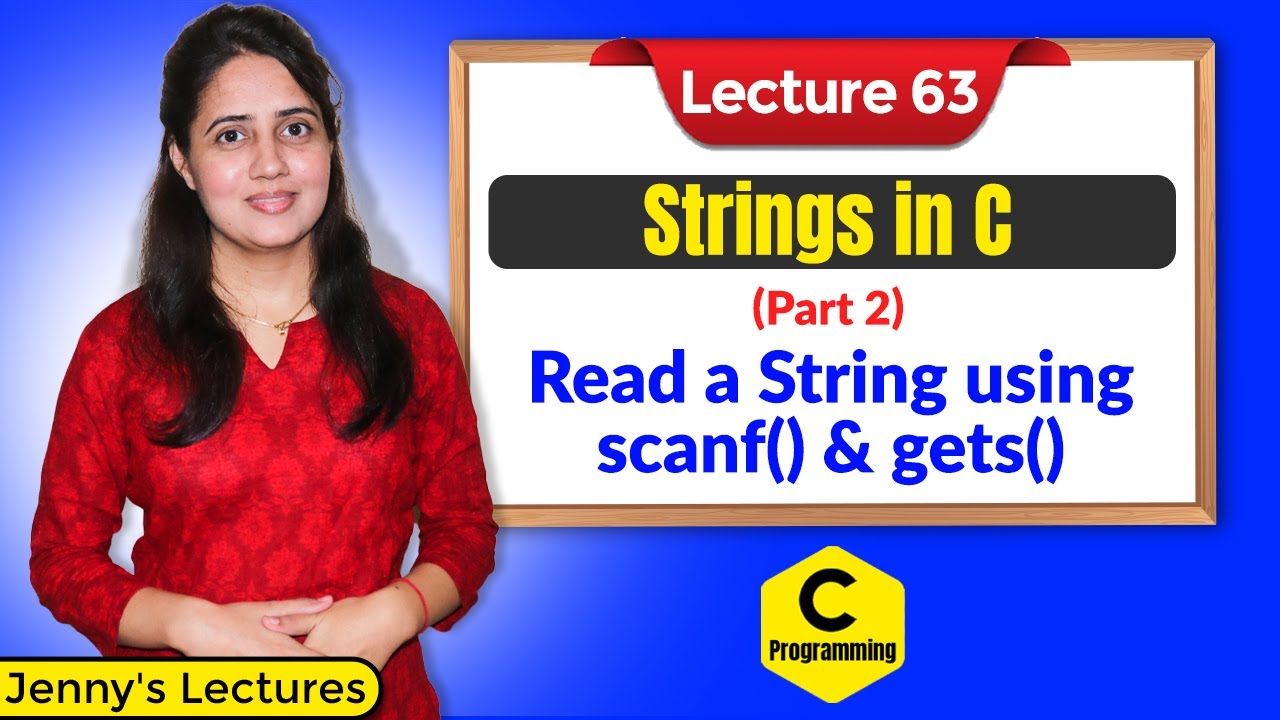
C_63 Strings in C-part 2 | Read a String using scanf and gets function

C++ Strings | What is String? full Explanation
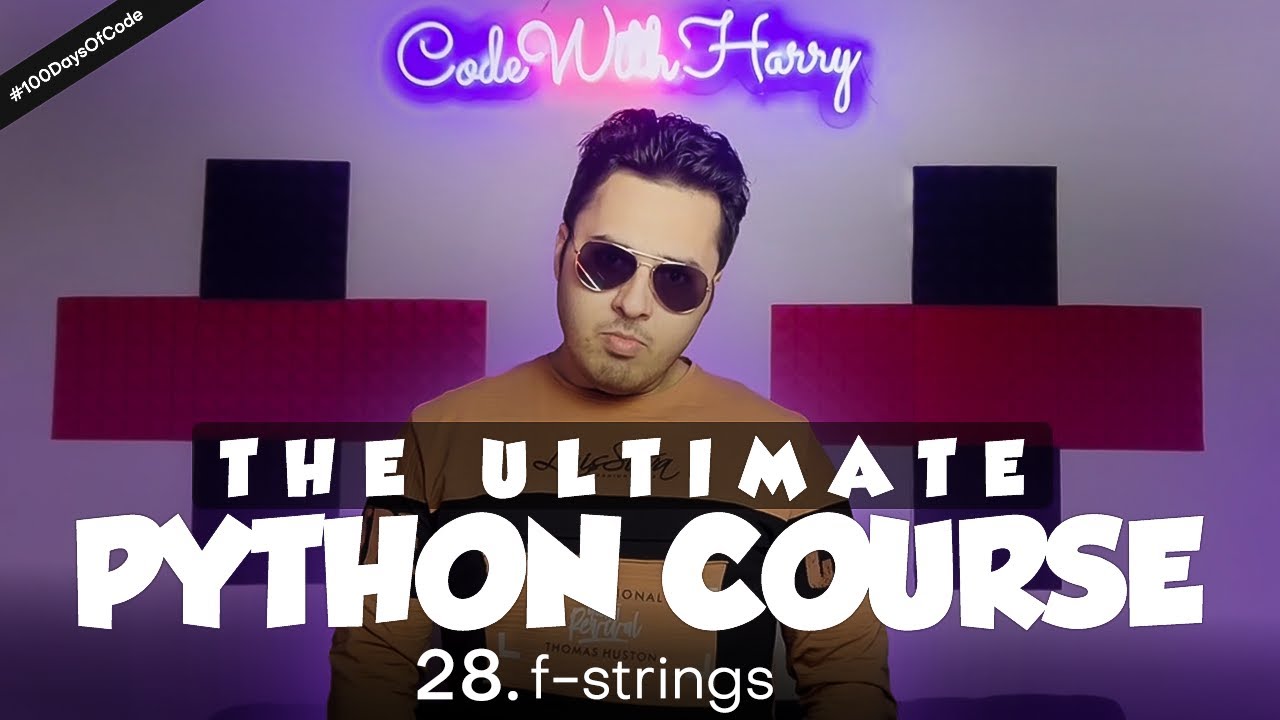
f-strings in Python | Python Tutorial - Day #28
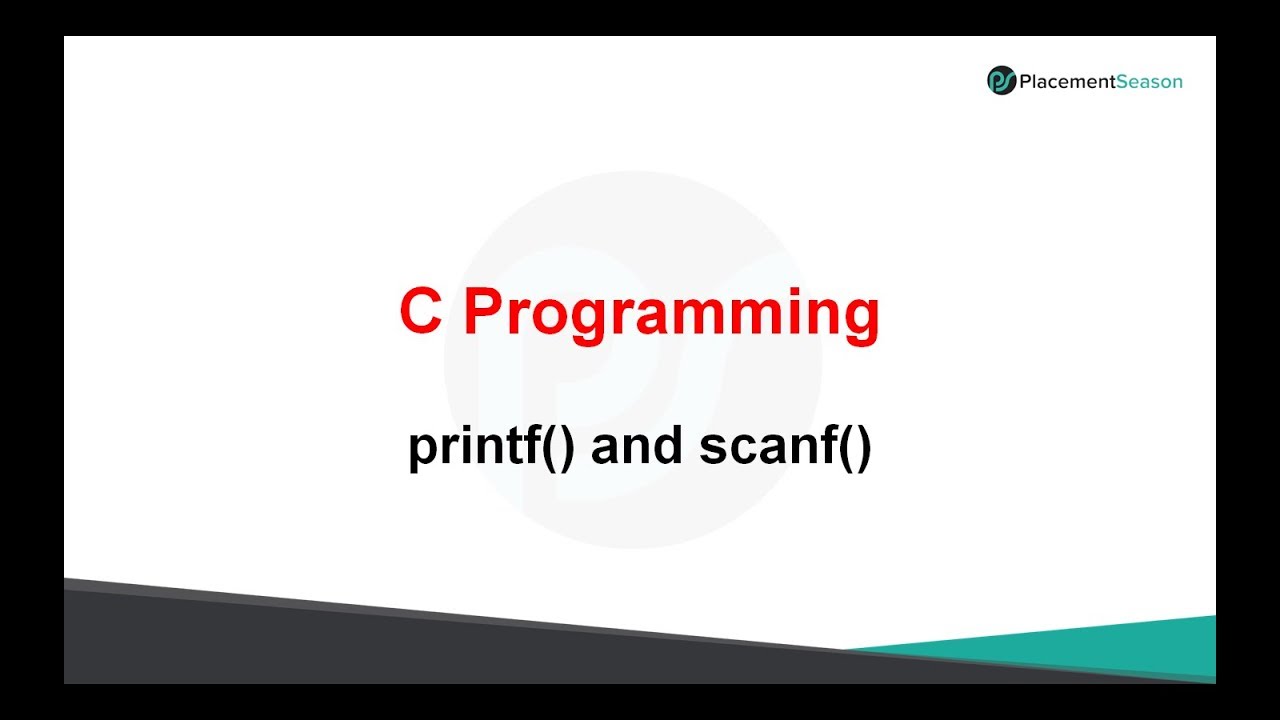
printf and scanf functions in C
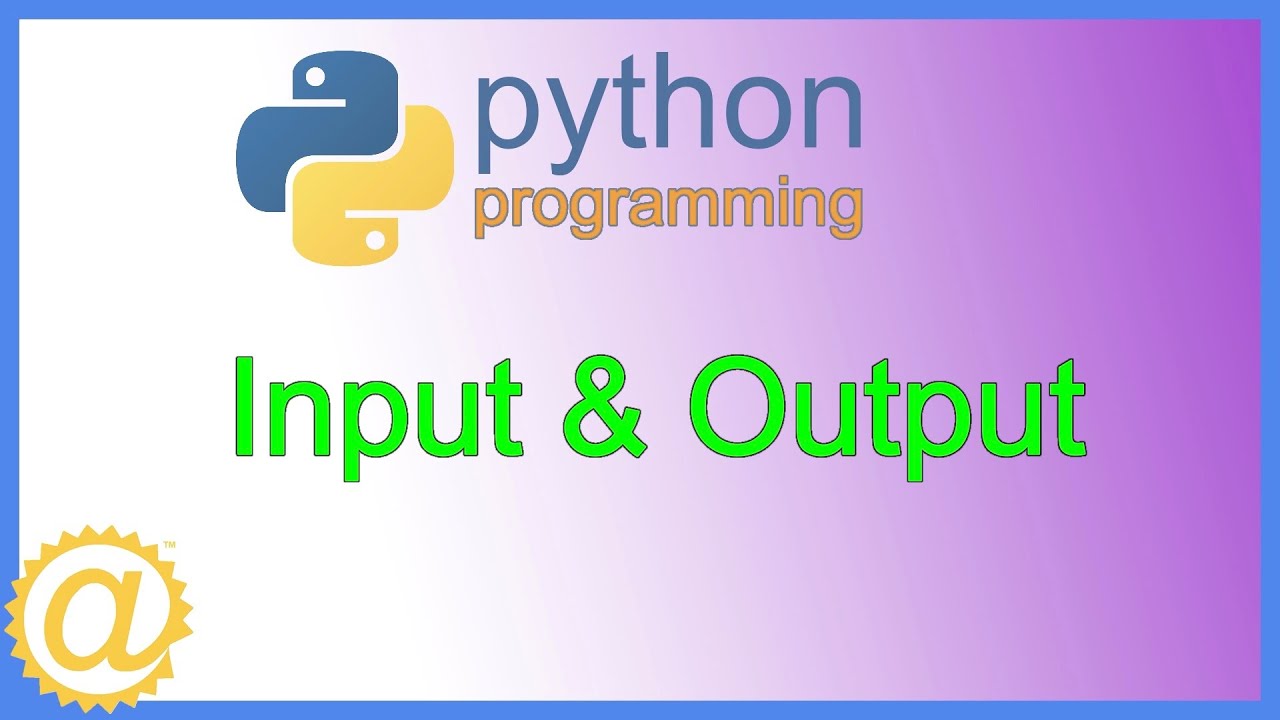
Python Programming - Basic Input and Output - print and input functions
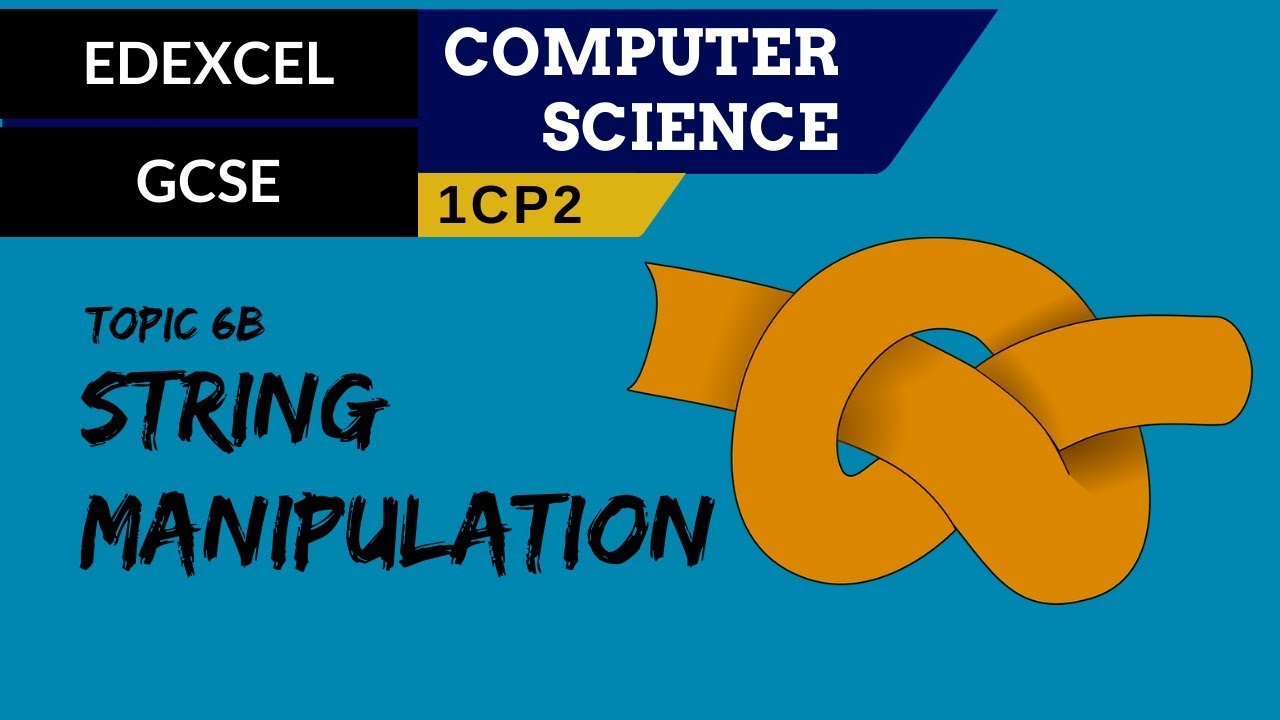
77. EDEXCEL GCSE (1CP2) The use of basic string manipulation
5.0 / 5 (0 votes)