Understanding the Void Pointers
Summary
TLDRThis presentation delves into the concept of void pointers in C programming, highlighting their unique ability to point to any data type without being associated with a specific one. It demonstrates how void pointers can be typecast to different types and used in scenarios like memory allocation with malloc and calloc functions, which return void pointers. The importance of typecasting before dereferencing void pointers to avoid errors is emphasized, illustrating their utility in dynamic memory allocation.
Takeaways
- 📌 Void pointers are unique in that they do not have an associated data type, allowing them to point to any data type.
- 🔄 The versatility of void pointers is demonstrated by their ability to be typecast to any other pointer type before being dereferenced.
- 💡 The script introduces the concept of void pointers as a precursor to more advanced topics like dynamic memory allocation.
- 👀 An example is provided where a void pointer is used to point to an integer variable, emphasizing the necessity of typecasting before dereferencing.
- ⚠️ Direct dereferencing of a void pointer is not allowed; it must be typecast to a specific data type first to avoid errors.
- 🤔 The necessity of void pointers is questioned, highlighting the need for understanding their utility in certain programming scenarios.
- 🔧 The use of void pointers is justified by their role in functions like malloc and calloc, which return void pointers and are used for dynamic memory allocation.
- 🧠 Understanding that malloc and calloc return void pointers is crucial as it explains their ability to allocate memory for any data type without knowing the data type in advance.
- 📚 The script provides the syntax for the malloc function, indicating that it returns a void pointer, although deeper understanding of its usage is reserved for later.
- 🔑 The importance of void pointers is underscored by their integral role in memory allocation functions, which are fundamental to dynamic memory management.
- 👋 The presentation concludes with a thank you, summarizing the key points about void pointers and setting the stage for further learning about dynamic memory allocation.
Q & A
What is a void pointer?
-A void pointer is a type of pointer that has no associated data type. It can point to any type of data and must be typecasted to another pointer type before dereferencing.
Why is typecasting important when using a void pointer?
-Typecasting is important because a void pointer does not have a specific data type. Before dereferencing it, the void pointer must be typecasted to the correct data type to ensure the correct interpretation of the memory it points to.
Can a void pointer be dereferenced directly?
-No, a void pointer cannot be dereferenced directly. It must first be typecasted to the appropriate data type before dereferencing.
Why might someone use a void pointer instead of an integer pointer directly?
-A void pointer is used because it is flexible and can point to any data type. This is useful in functions like `malloc` and `calloc`, which allocate memory dynamically and need to return a pointer that can point to any data type.
What are `malloc` and `calloc` functions, and how are they related to void pointers?
-`malloc` and `calloc` are built-in functions used for dynamic memory allocation. They return a void pointer, which allows them to allocate memory for any data type, without needing to know the type of data in advance.
How does a void pointer help in dynamic memory allocation?
-A void pointer helps in dynamic memory allocation by allowing functions like `malloc` and `calloc` to allocate memory for any type of data. Since a void pointer can be typecasted to any data type, it provides the flexibility needed in dynamic memory management.
What happens if you try to use a void pointer without typecasting?
-If you try to use a void pointer without typecasting, it will result in a compilation error because the compiler does not know the data type of the memory the pointer is referring to.
What is the output of the example given in the script when the void pointer is correctly typecasted and dereferenced?
-The output of the example will be 10, as the void pointer is typecasted to an integer pointer, and when dereferenced, it returns the value stored at the memory address, which is 10.
Why is it said that void pointers are 'interesting'?
-Void pointers are considered interesting because they are versatile and can point to any data type, offering flexibility in memory management. This ability to point to different types of data and be typecasted to any pointer type is unique to void pointers.
What is the syntax of the `malloc` function and what does it return?
-The syntax of the `malloc` function is: `void* malloc(size_t size);`. It returns a void pointer, which points to a block of memory of the specified size. This memory can then be typecasted to any data type.
Outlines
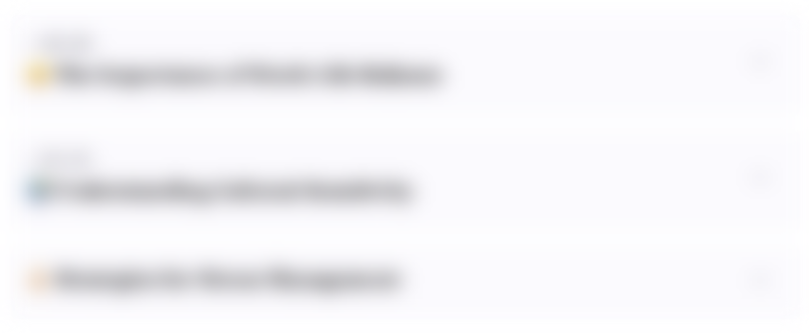
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
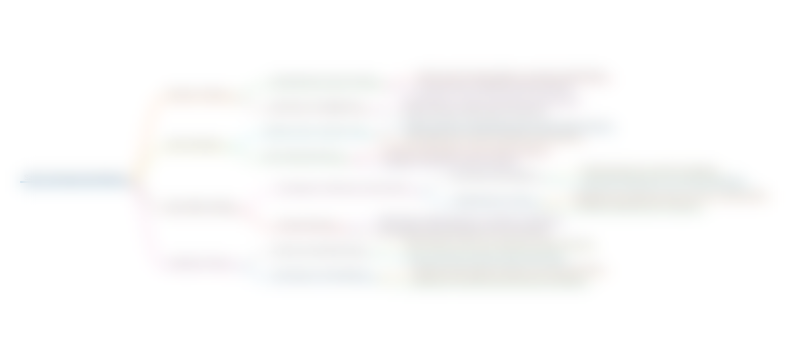
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
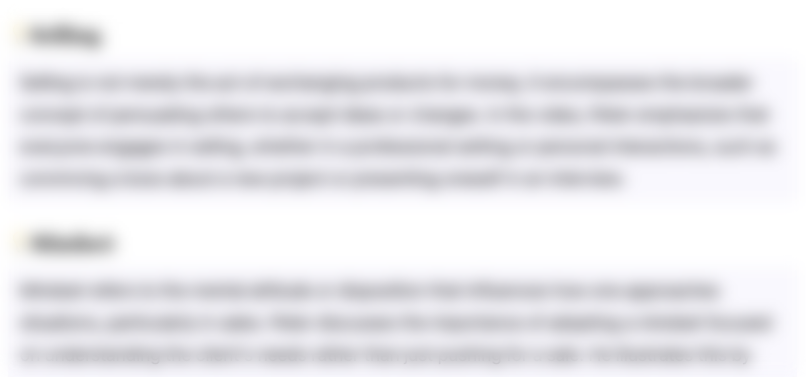
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
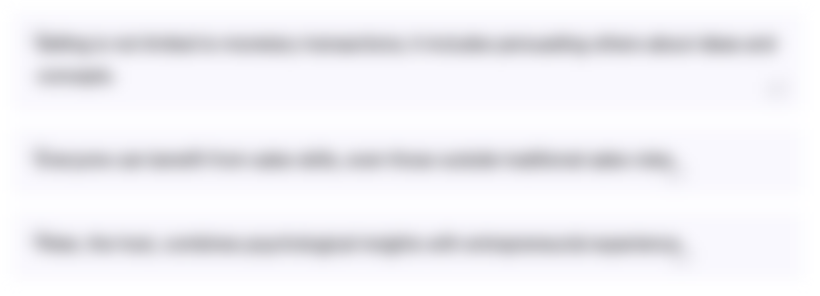
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
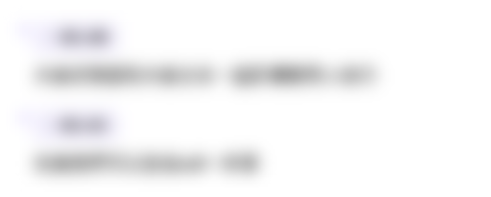
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)