State Pattern
Summary
TLDRThis video script introduces the concept of state patterns in software design, explaining how objects can have different behaviors based on their internal states. Through a detailed example of a rental property system, it demonstrates how states like 'waiting', 'receiving application', 'apartment rented', and 'fully rented' affect the behavior of the system. The script illustrates the benefits of using objects to manage states, avoiding complex conditionals. The example uses classes, state interfaces, and event transitions to show how behavior can change dynamically, making the software more efficient and easier to maintain.
Takeaways
- 😀 The State Pattern is a design pattern that allows objects to change their behavior based on their internal state, avoiding complex conditional statements.
- 😀 Each state in the system is modeled as a separate class implementing a common interface, which encapsulates state-specific behavior.
- 😀 State transitions are triggered by events (e.g., receiving an application or renting an apartment) and are handled by replacing the current state object.
- 😀 The system uses four main states: Waiting, Receiving Application, Apartment Rented, and Full Rented, each representing different stages in the rental process.
- 😀 By using the State Pattern, objects can behave differently depending on their state, ensuring flexibility and reducing complexity in handling different conditions.
- 😀 Each state has its own methods, allowing the object to respond differently to similar events depending on its current state.
- 😀 The Waiting state allows receiving applications, while the Receiving Application state processes those applications and decides whether to accept or reject them.
- 😀 If an application is accepted, the state transitions to Apartment Rented, where further actions like dispensing keys take place.
- 😀 When the rental reaches Full Rented status, no further applications can be accepted, and the system prevents any action that is not relevant to this state.
- 😀 The State Pattern reduces the need for multiple conditional checks (e.g., using `if` statements), making the code cleaner and easier to maintain.
- 😀 The implementation of the State Pattern helps manage complex systems with multiple states and transitions by treating each state as an independent object.
Q & A
What is the State Pattern in software design?
-The State Pattern is a design pattern in software engineering that allows an object to change its behavior when its internal state changes. It encapsulates state-specific behavior into separate state classes, which makes the object's behavior dependent on its current state.
Why is the State Pattern useful in managing object behavior?
-The State Pattern is useful because it simplifies handling objects whose behavior depends on their internal state. Without the State Pattern, you would have to use complex conditional checks to determine behavior, which can lead to messy and hard-to-maintain code.
How does the State Pattern help avoid complex conditional checks?
-By encapsulating state-specific behavior into separate state objects, the State Pattern removes the need for large if-else statements or switch cases. Each state object knows how to handle the events relevant to its state, thus reducing the complexity of conditionals.
Can you describe the example provided in the script about an apartment rental system?
-The example involves a system managing the rental of apartments. The system can be in one of four states: Waiting, Receiving an Application, Apartment Rented, or Full Rented. Based on the current state, the system will behave differently when events such as receiving an application or renting an apartment occur.
What are the four states described in the apartment rental example?
-The four states are: 1) Waiting (the system is waiting for applications), 2) Receiving an Application (the system is processing an application), 3) Apartment Rented (the apartment has been rented), and 4) Full Rented (all apartments are rented out).
How does the system transition between these four states?
-The system transitions from one state to another based on events or actions, such as receiving an application, checking an application, or renting out an apartment. For example, when an application is received, the system transitions from the Waiting state to the Receiving an Application state.
What role do the state classes play in the State Pattern?
-State classes represent the different states an object can be in. Each state class implements specific behaviors and defines methods for handling events that trigger state transitions. For instance, a WaitingState class will handle events like receiving an application, while an ApartmentRentedState class handles renting out an apartment.
What is the advantage of using state objects instead of managing state through methods?
-Using state objects reduces the need for complex conditionals within methods. Each state object manages its own behavior and transitions, leading to cleaner, more maintainable code. This also makes the system more flexible when adding new states or modifying existing ones.
How are state transitions managed in the State Pattern?
-State transitions are managed by calling methods defined in the state objects. When a specific event occurs (like receiving an application), the current state object changes to a new state object, which defines the behavior for the next state.
What does the implementation of the State Pattern in the script look like in terms of code?
-In the script's implementation, there is an interface called 'State', with methods defining possible events for each state. Classes like WaitingState, ReceivingApplicationState, and others implement this interface. The system then uses a state attribute that points to the current state object, allowing it to handle events and transition between states accordingly.
Outlines
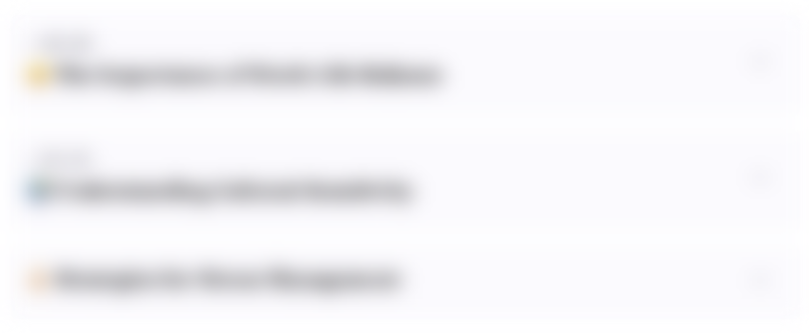
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
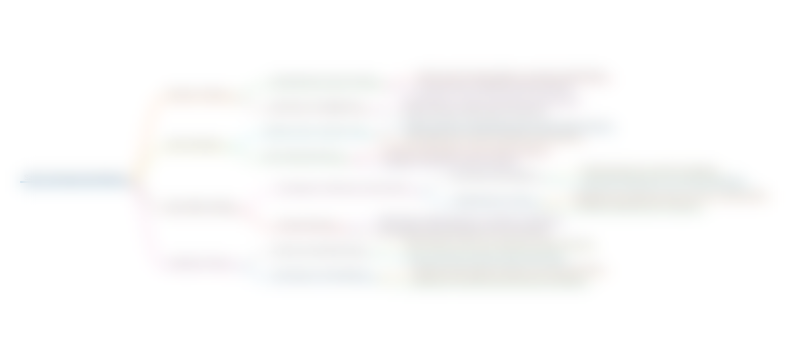
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
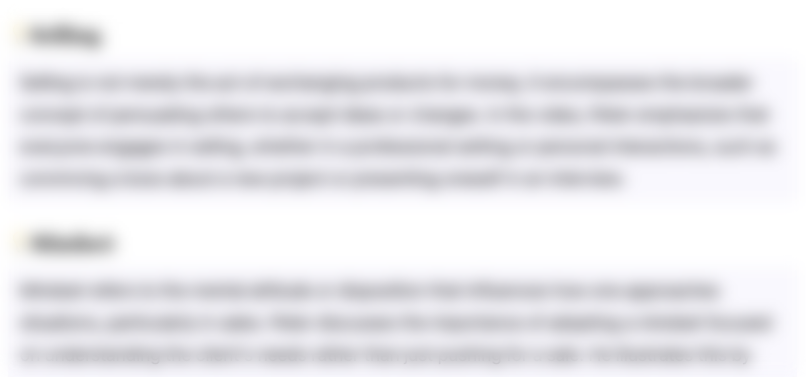
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
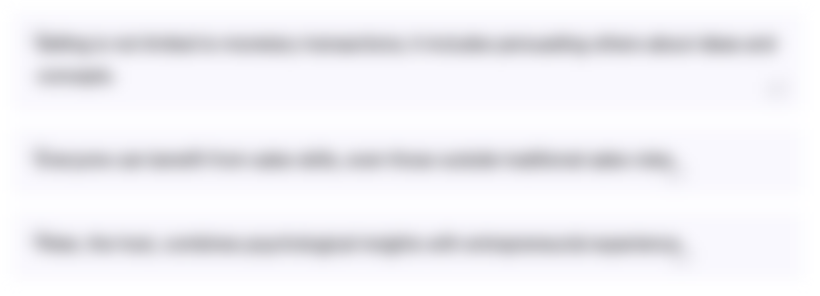
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
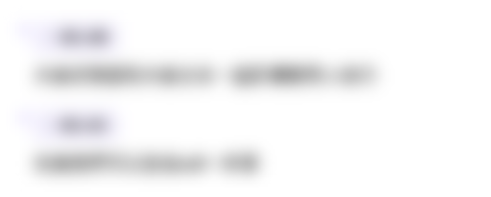
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
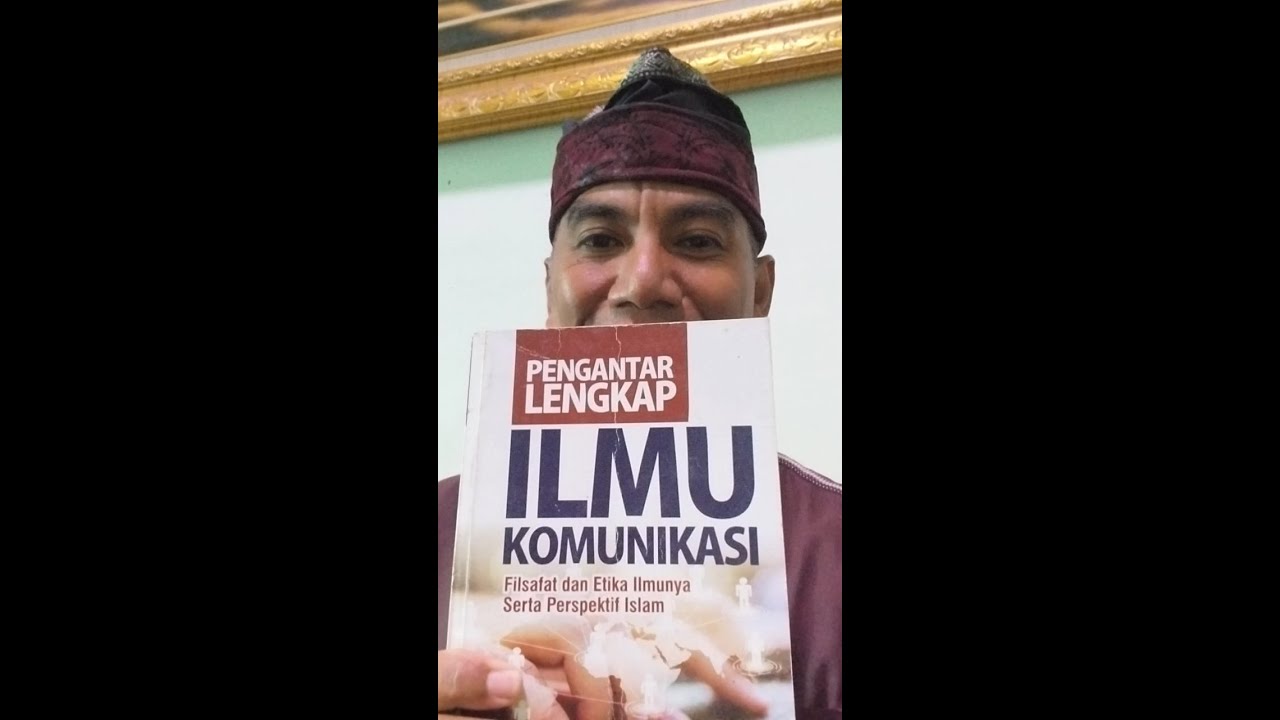
PROF RACHMAT KRIYANTONO (PROF RK): SIMBOL, TANDA, IKON & INDEKS- BUKU PENGANTAR LENGKAP KOMUNIKASI
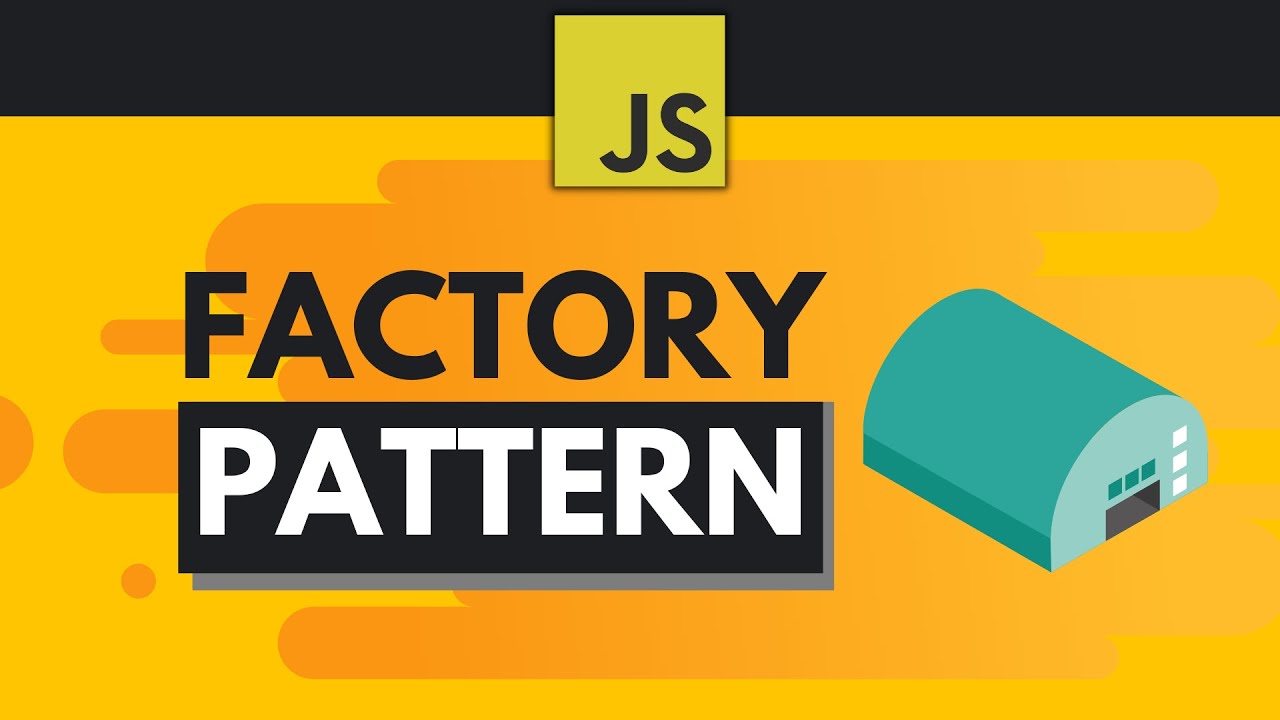
Javascript Design Patterns #1 - Factory Pattern

AP CS A - 2.1 Instances of Classes

Video Simulasi Mengajar Pola Gambar (Membesar dan mengecil) - 5D Shelsa Angginova Rahmadea
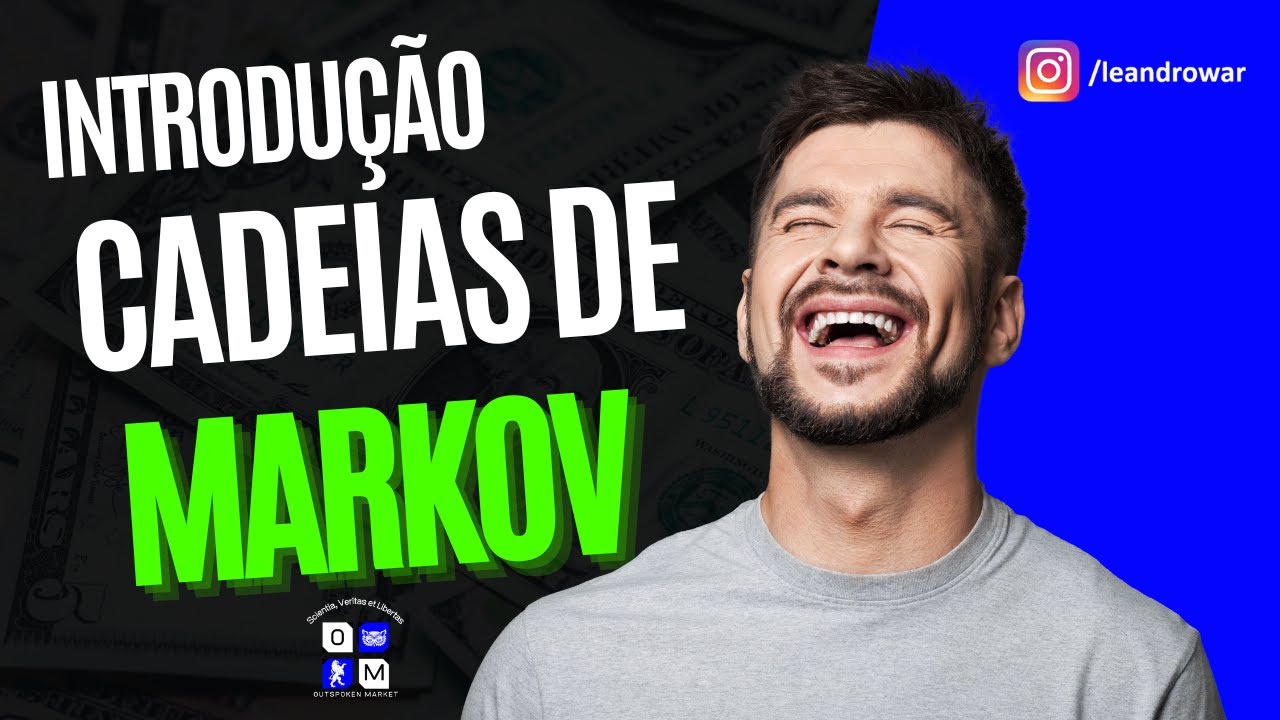
Introdução - Cadeias de Markov (Markov Chains) - Outspoken Market
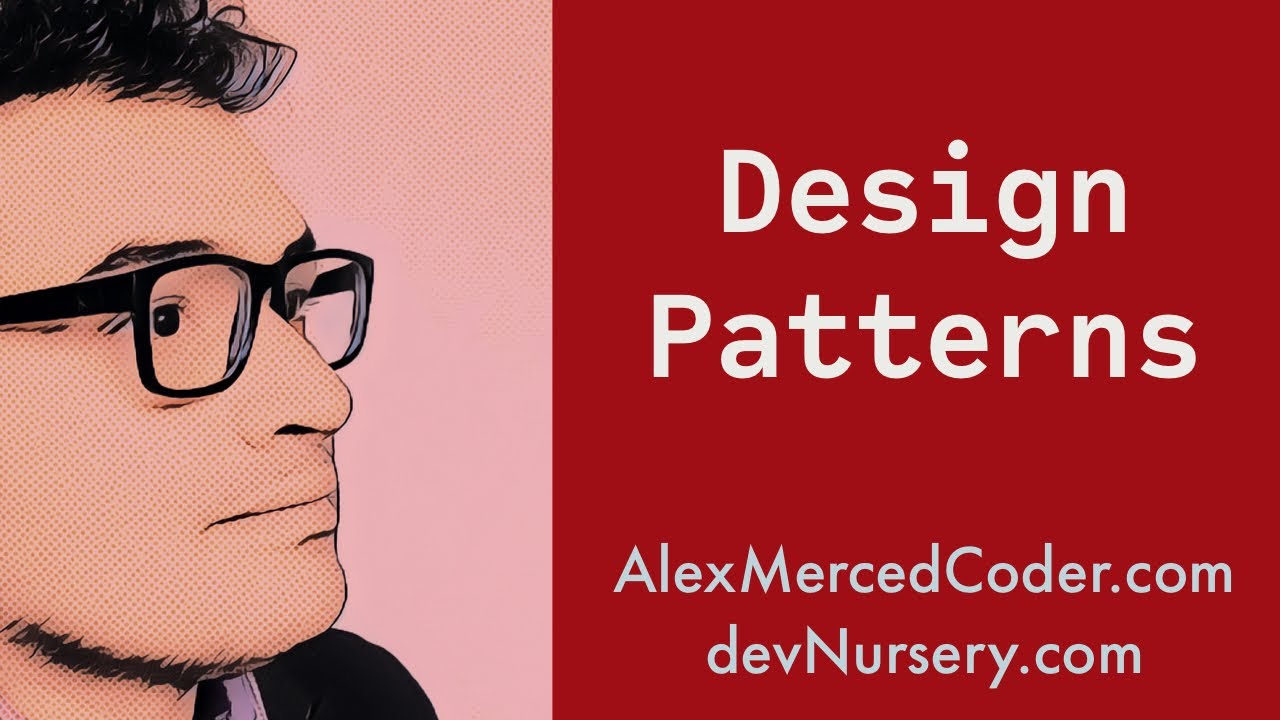
Programming Concepts - Design Patterns (Creational, Structural and Behavioral)
5.0 / 5 (0 votes)