Image Compression with Python
Summary
TLDRThis video demonstrates how to compress an image using Singular Value Decomposition (SVD) in Python. By converting the image into a grayscale matrix, the script performs SVD to break the image into its essential components: U, Sigma, and V. The tutorial shows how varying the number of principal components affects the image quality and compression, explaining the trade-off between data size and quality. Through this step-by-step guide, viewers learn how to reduce image size for storage or transmission while maintaining key features, illustrating the power of linear algebra in image processing.
Takeaways
- 😀 SVD (Singular Value Decomposition) is used in image compression by breaking down the image into three matrices: U, Σ, and V.
- 😀 The process begins by importing necessary libraries and loading the image into Python using `PIL` and `matplotlib`.
- 😀 The image is first converted into grayscale to simplify the compression process, reducing the complexity of color channels.
- 😀 After converting the image into a matrix, it is stored as a numpy array to enable numerical operations like SVD.
- 😀 Singular values (Σ) are the key components in SVD, representing the principal components of the image data.
- 😀 By keeping fewer singular values, you can compress the image, reducing the data required for storage or transmission.
- 😀 Retaining just the first singular value creates a blurry, low-quality image, but significantly reduces data size.
- 😀 As more singular values are retained, the image quality improves, but so does the amount of data that needs to be transmitted.
- 😀 The script demonstrates how retaining different numbers of principal components (e.g., 1, 2, 3, etc.) progressively improves the image quality.
- 😀 A trade-off exists between image quality and data size; after a certain number of principal components, additional values have minimal effect on image quality.
- 😀 The analysis of singular values reveals that most of the influence comes from the first few values, while later values contribute less to the image's quality.
Q & A
What is the purpose of using Singular Value Decomposition (SVD) in image compression?
-SVD is used in image compression to reduce the dimensionality of the image, allowing it to be represented with fewer data points while still approximating the original image. By retaining only the most significant singular values and corresponding vectors, the image can be compressed without sacrificing too much quality.
What programming environment and tools are being used to demonstrate the process?
-The tutorial is demonstrated using Python within a Jupyter Notebook environment. The key libraries used include `numpy` for matrix operations, `matplotlib` for visualization, and `PIL` (Python Imaging Library) for image processing.
Why is the image converted to grayscale before performing SVD?
-Converting the image to grayscale simplifies the problem by reducing the image to a single intensity channel, rather than dealing with three separate RGB channels. This makes the SVD process more straightforward and reduces computational complexity.
How does the process of converting an image to a matrix help in compression?
-When the image is converted into a matrix (numerical array), each pixel’s value is represented numerically, enabling the application of linear algebra techniques like SVD. This allows us to manipulate the image's underlying data structure and perform compression by keeping only the most significant components.
What are the components of the SVD decomposition, and what do they represent?
-SVD decomposes the image matrix into three components: `U`, `Sigma`, and `V`. `U` contains the left singular vectors, `Sigma` contains the singular values (which represent the importance of each component), and `V` contains the right singular vectors. These components are used to approximate the original image.
Why do we keep only a subset of the singular values when reconstructing the image?
-By keeping only a subset of the singular values, we reduce the amount of data needed to represent the image. However, fewer components result in a blurrier image. The challenge is to balance data reduction with maintaining image quality, which is why selecting the right number of singular values is important.
What happens to the image quality as more principal components are retained?
-As more principal components are retained, the reconstructed image becomes clearer and more accurate, closely resembling the original. This is because more singular values and vectors contribute to the reconstruction, preserving finer details of the image.
How does the number of principal components affect the amount of data sent for compression?
-The number of principal components directly influences the amount of data that needs to be sent. Fewer components require less data to represent the image, but this results in a lower quality. As more components are retained, the data size increases, but the image quality improves.
How do we calculate the size of the original image and the compressed version?
-The size of the original image is determined by its total number of pixels (rows x columns). The compressed version is calculated by considering the sizes of the `U`, `Sigma`, and `V` matrices for the retained principal components. For example, sending only 40 components requires fewer values than the original image, which can result in significant data size reduction.
What is the significance of the plot of singular values, and what does it tell us about image compression?
-The plot of singular values shows how much each singular value contributes to the image. It typically reveals that the first few singular values are large and contribute significantly to the image’s structure, while the later values are much smaller and have little effect. This helps in determining how many components are necessary to achieve a good balance between compression and image quality.
Outlines
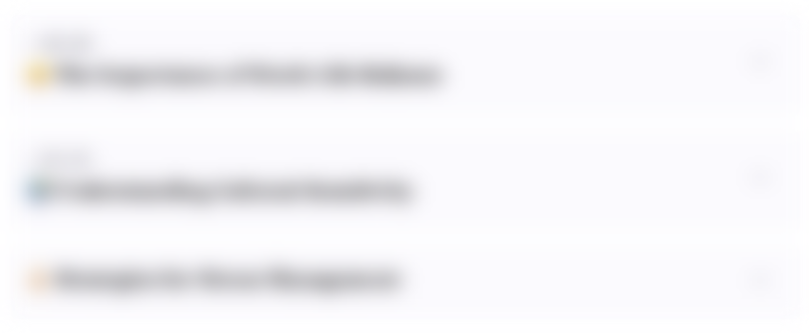
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
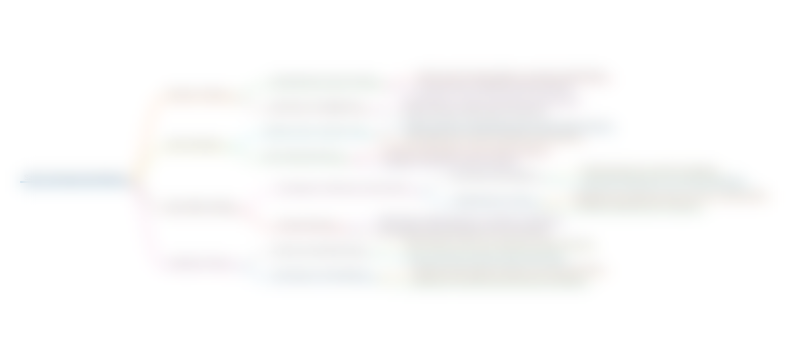
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
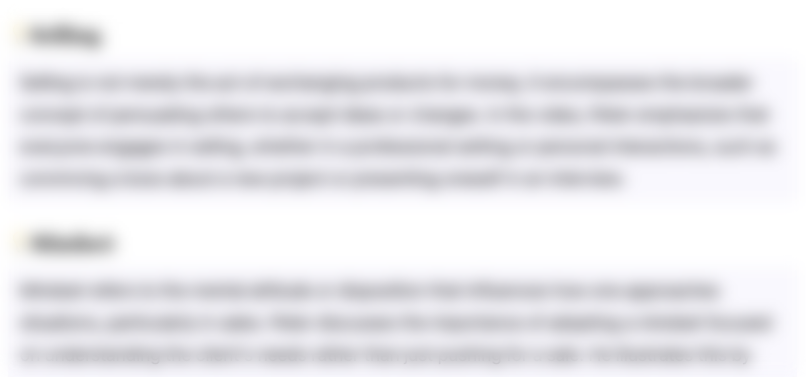
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
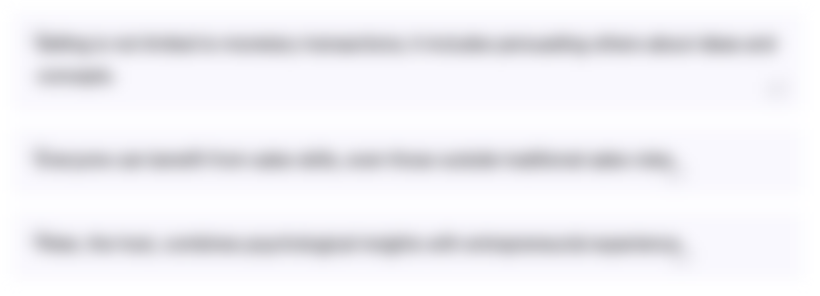
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
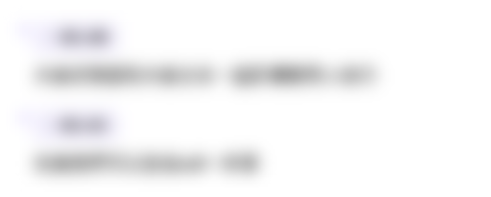
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Singular Value Decomposition (SVD) and Image Compression

Singular Value Decomposition (SVD): Mathematical Overview
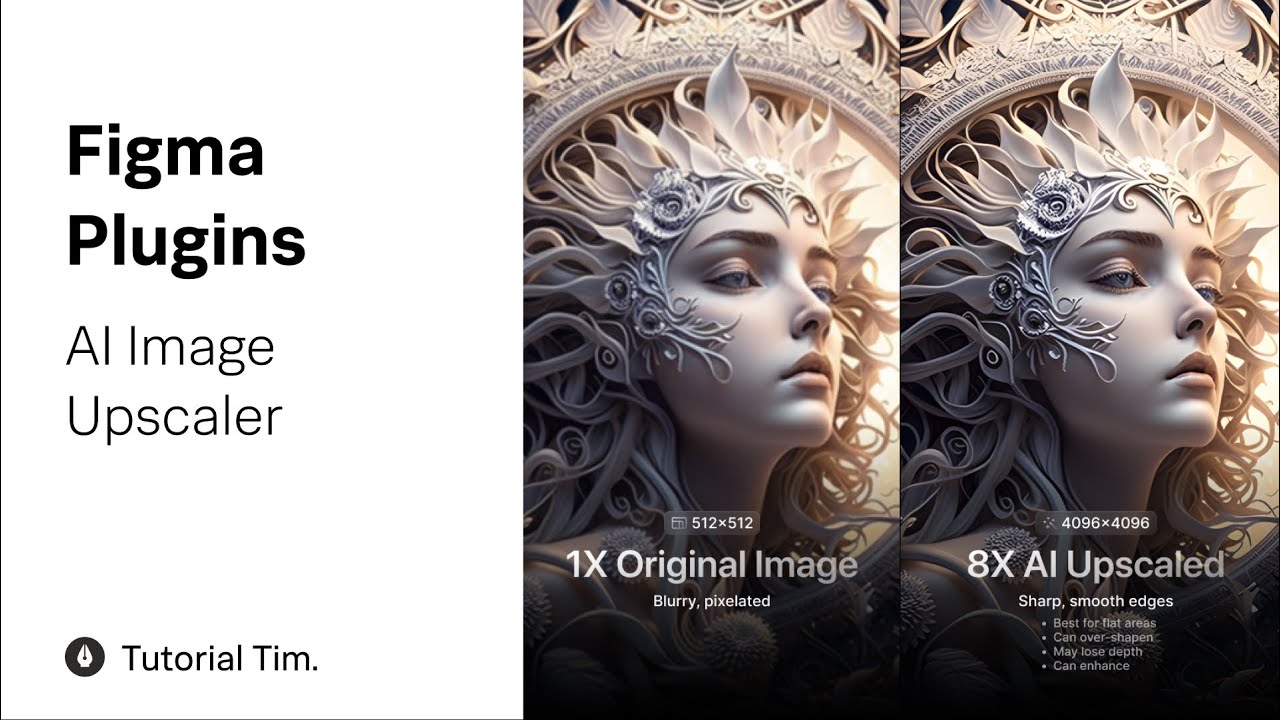
Figma Plugins: AI Image Upscaler
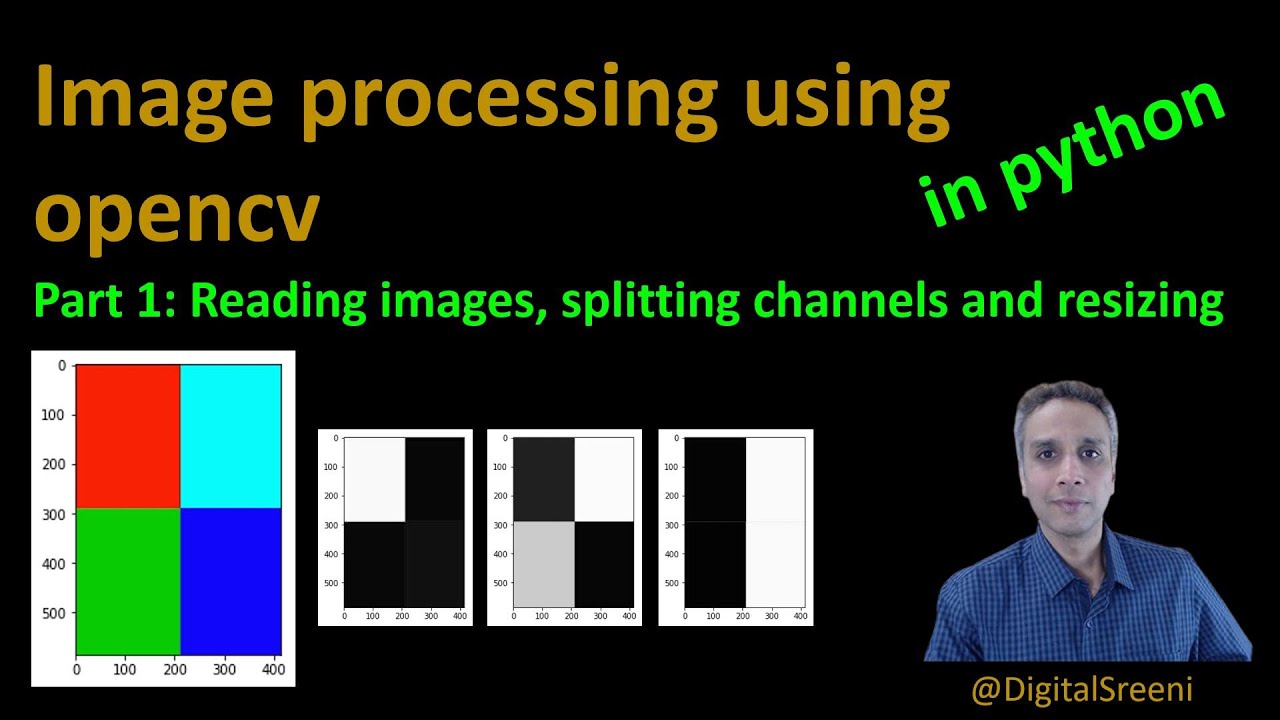
25 - Reading Images, Splitting Channels, Resizing using openCV in Python
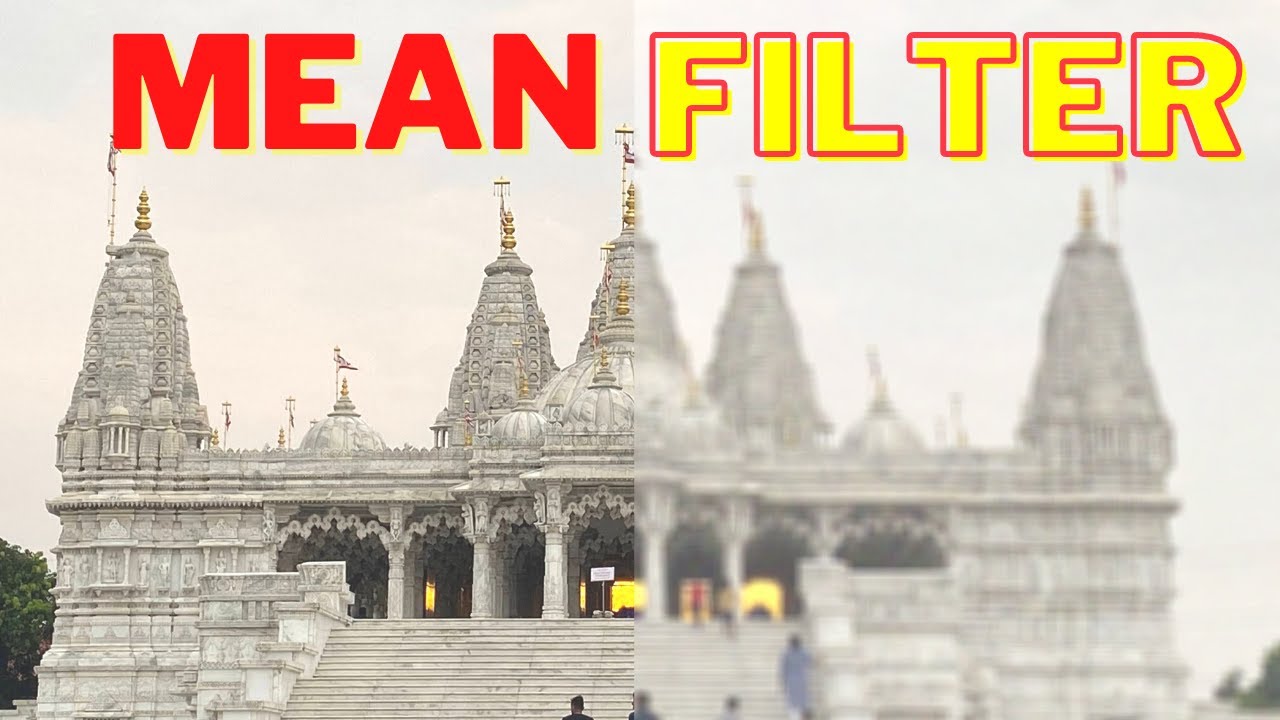
How mean filter in Image Processing works ? | Computer Vision | OpenCV | Image Smothing blur

StatQuest: Principal Component Analysis (PCA), Step-by-Step
5.0 / 5 (0 votes)