Python Methods and self
Summary
TLDRThis video tutorial introduces the concept of methods in Python classes. The instructor explains how functions defined within classes are referred to as methods. Using a simple example, the video demonstrates how to create a class, define a method, and then instantiate the class into an object. The tutorial covers key concepts like 'self' and dot notation, explaining why 'self' is needed to tie methods to specific instances of a class. The video also includes a debugging example where adding 'self' resolves an error, illustrating how object-oriented programming works in Python.
Takeaways
- 💻 Methods in Python classes are similar to functions but defined within a class and are called 'methods.'
- 📝 The 'demoore' method in the example class simply prints 'I am the demonstration method.'
- 📦 When an object is created from a class, that object inherits the methods defined in the class.
- ❌ A TypeError occurs if a method does not include the 'self' parameter because Python implicitly passes the object itself as the first argument.
- 🔄 The 'self' parameter in a method refers to the instance of the class, ensuring the method is bound to the object created from the class.
- 🔧 By adding 'self' to the method's definition, the TypeError is fixed, and the method can be called without crashing.
- 📜 The 'dot notation' (e.g., object.method()) is used to invoke methods on objects, where 'self' ensures the method is associated with a specific object.
- 🆔 Python’s 'id()' function can show that the 'self' parameter and the object ID are the same, proving that 'self' refers to the instance of the object.
- 🏗️ Object-oriented programming in Python involves creating instances (objects) from classes, and methods within those objects are tied to the instance through 'self.'
- 🔍 The script emphasizes the importance of the 'self' keyword in object-oriented programming, helping methods within classes know which object they are associated with.
Q & A
What is a method in Python, according to the script?
-A method in Python is a function defined within a class. It is similar to a regular function but is called a 'method' when it belongs to a class. In the script, the example method is named 'demoore_method'.
How do you create an instance of a class in Python?
-An instance of a class is created by using the class name followed by parentheses, which calls the class constructor. For example, in the script, 'my_object = demo_class()' creates an instance of the class 'demo_class'.
What is the purpose of the 'self' keyword in a method?
-The 'self' keyword is used in a method to refer to the current instance of the class. It allows the method to access attributes and methods associated with that specific instance.
What error occurs when the 'self' keyword is missing in a method, and why?
-A 'TypeError' occurs, specifically stating that the method takes 0 positional arguments but 1 was given. This happens because Python automatically passes the instance as the first argument (i.e., 'self') to the method, but if 'self' is not included in the method definition, it leads to a mismatch in expected arguments.
How can you fix the 'TypeError' when calling a method?
-To fix the 'TypeError', include 'self' as the first parameter in the method definition. This ensures the method can receive the instance of the class when it's called.
What is the significance of the dot notation in Python classes?
-Dot notation is used to access methods or attributes of an object. For example, 'my_object.demo_method()' calls the 'demo_method' of the instance 'my_object'.
What is the role of 'self' when a method is invoked on an instance?
-When a method is invoked on an instance, 'self' automatically receives the reference to the current instance, allowing the method to know which instance it is operating on.
What happens when you print the ID of 'self' and the object?
-When printing the ID of 'self' and the object, both IDs will be the same because 'self' refers to the instance of the object, showing that the method belongs to that particular instance.
What is the output of a method when called using an instance?
-When a method is called using an instance (e.g., 'my_object.demo_method()'), the method will execute and output whatever is inside it, such as printing a string to the console. In this case, it prints 'I am the demonstration method'.
Why does Python automatically pass the instance as the first argument to a method?
-Python automatically passes the instance as the first argument to a method to allow the method to access the attributes and methods specific to that instance. This helps bind the method to the instance it is called on.
Outlines
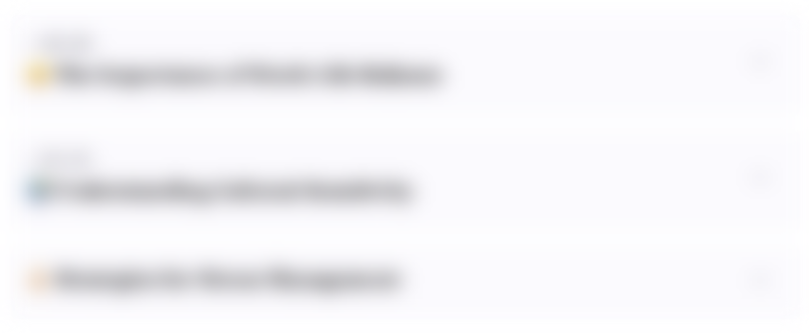
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
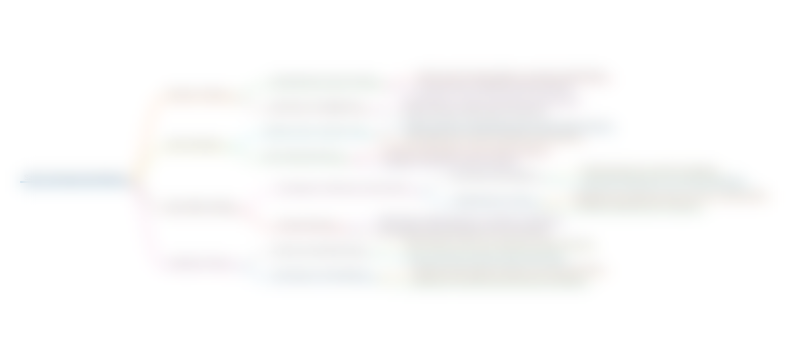
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
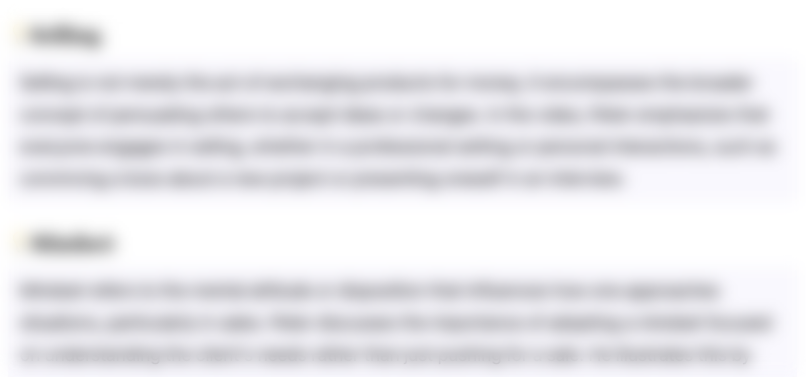
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
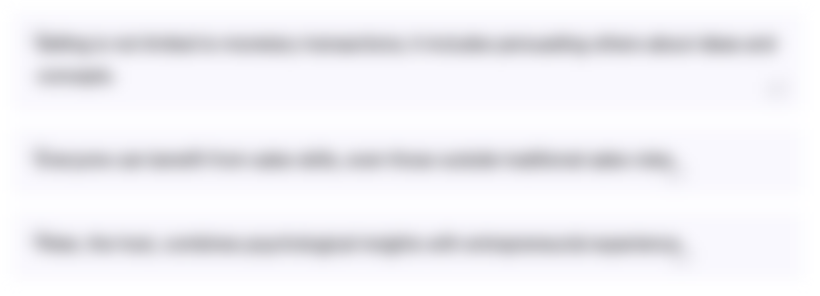
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
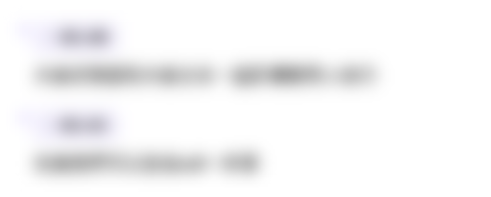
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Python OOP Tutorial 1: Classes and Instances
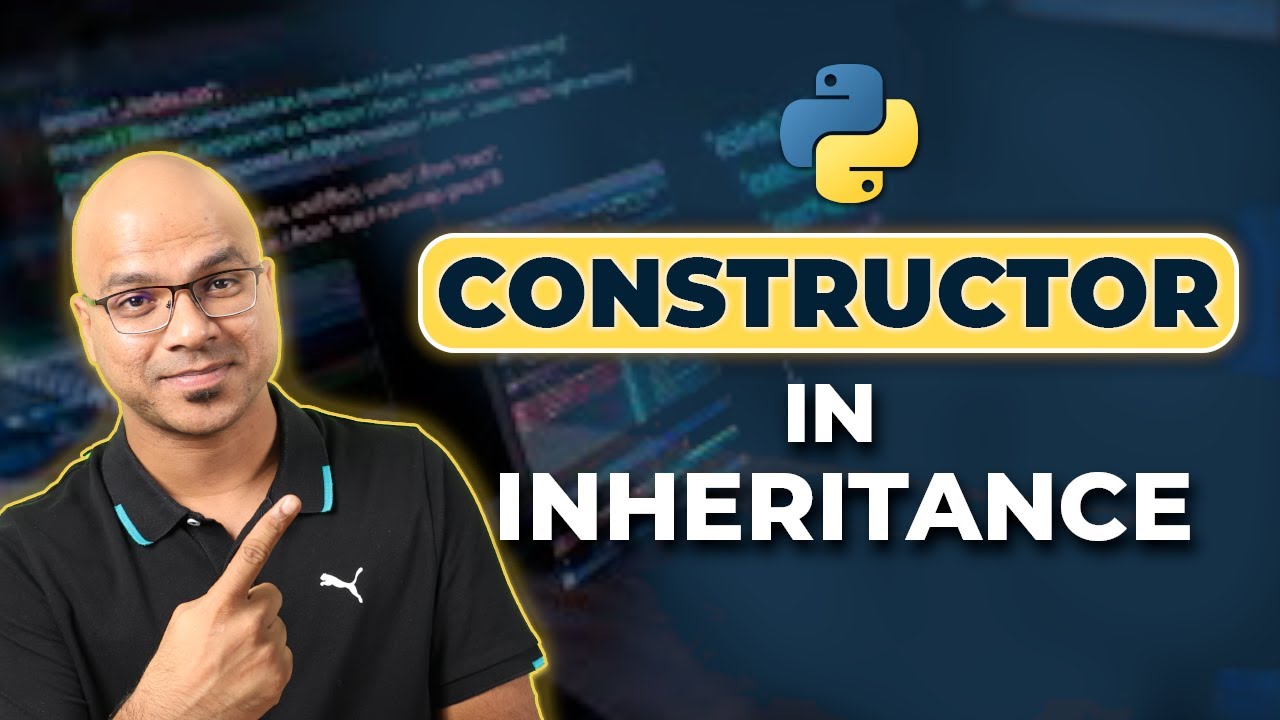
#56 Python Tutorial for Beginners | Constructor in Inheritance
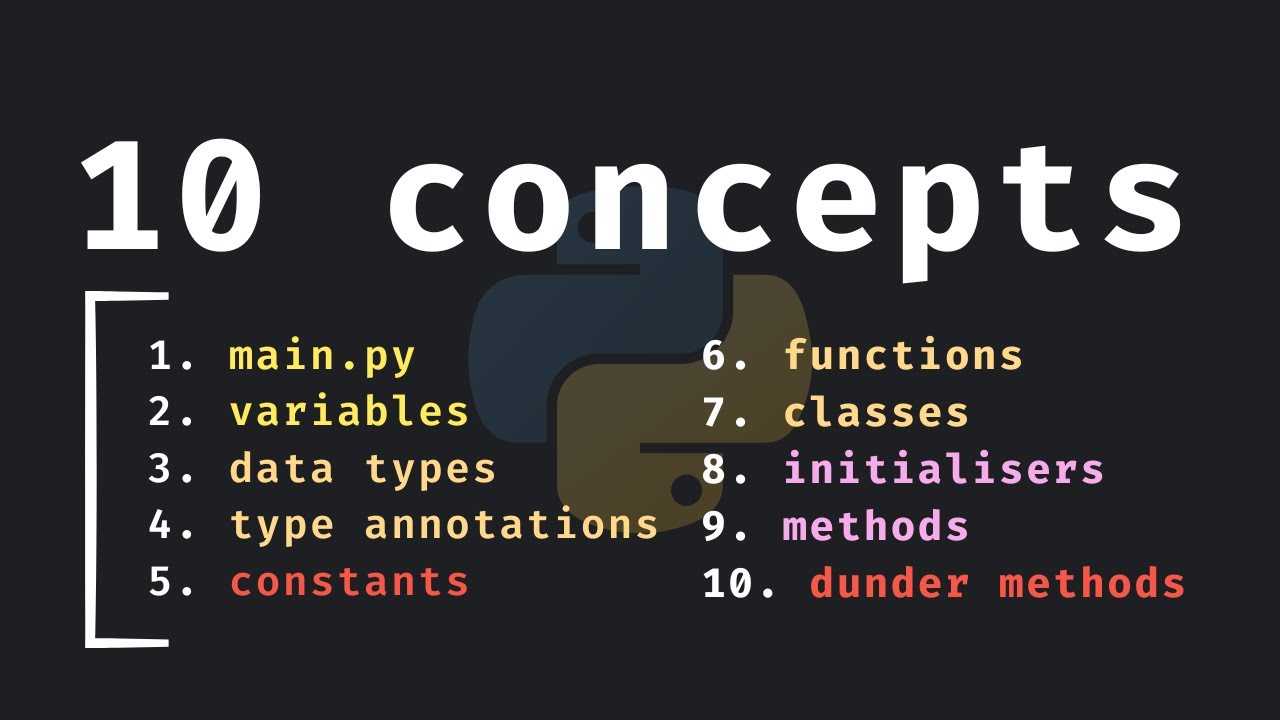
10 Important Python Concepts In 20 Minutes

Criando Métodos de Objetos - Curso Python Orientado a Objetos [Aula 03]
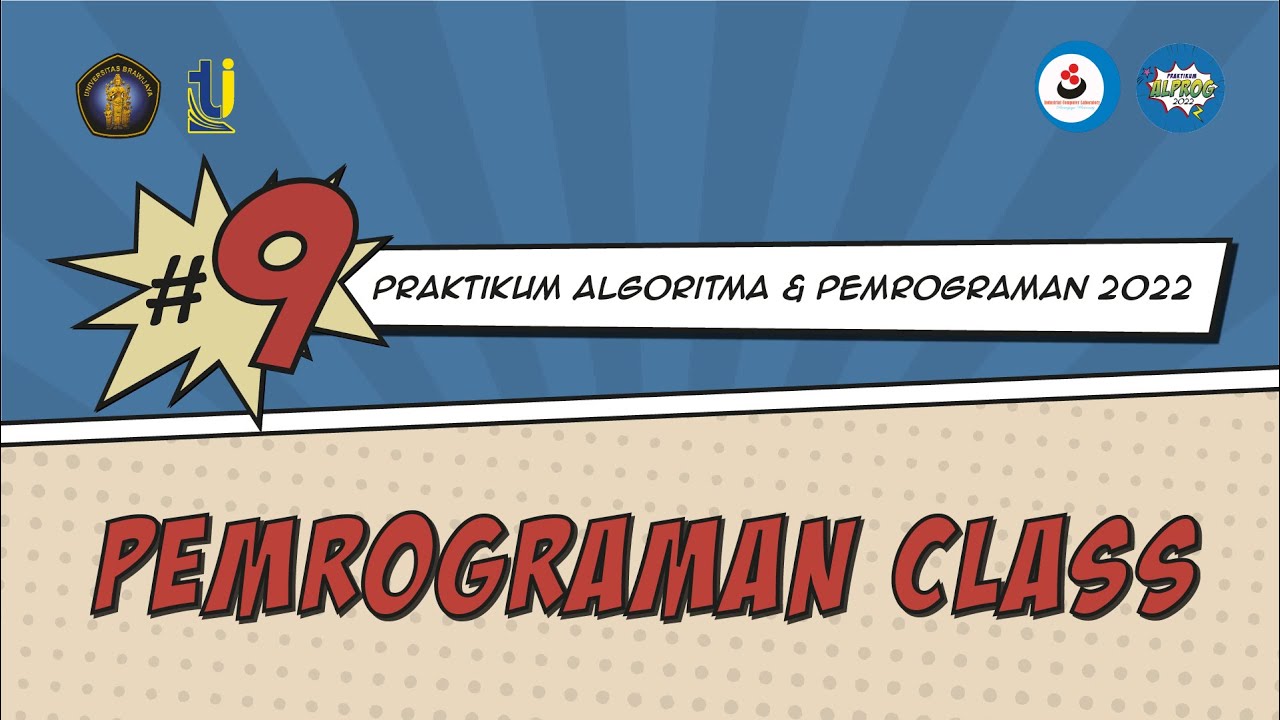
9. Pemrograman Class - Praktikum Algoritma dan Pemrograman 2022

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85
5.0 / 5 (0 votes)