Python OOP Tutorial 1: Classes and Instances
Summary
TLDRThis video tutorial introduces the concept of classes in Python, emphasizing their importance in modern programming for logical data and function grouping. It covers the basics of creating and instantiating classes, explaining the distinction between class and instance, and the use of instance variables. The video demonstrates how to utilize the special `__init__` method for automatic attribute assignment and introduces the creation of methods, using a simple 'Employee' class as an example. It also highlights the importance of the 'self' argument in methods and the difference in calling methods on instances versus classes. The content is aimed at beginners, providing a solid foundation for understanding object-oriented programming in Python.
Takeaways
- ๐ Introduction to Python classes and object-oriented programming concepts.
- ๐ฏ Classes are used to logically group data and functions for easy reuse and expansion.
- ๐๏ธ The basics of creating and instantiating classes are covered, emphasizing their structure and usage.
- ๐ Explanation of the difference between a class (blueprint) and an instance (unique implementation).
- ๐ Instance variables store unique data for each instance, while class variables are shared across all instances.
- ๐ ๏ธ Use of the special `__init__` method to automatically set up instance variables when creating new instances.
- ๐ Manual assignment of instance variables is error-prone and not efficient; the `__init__` method mitigates this.
- ๐ Instance methods are defined and used to perform actions on the instance data, with `self` referring to the instance.
- ๐ Demonstration of a method to display an employee's full name, highlighting the use of instance variables within methods.
- โ ๏ธ Importance of including the `self` argument in instance methods to reference the instance data.
- ๐ Clarification on invoking methods using both the instance and the class name, and how `self` is handled in these cases.
Q & A
Why are classes useful in programming?
-Classes are beneficial because they allow us to logically group data and functions together, making it easier to reuse and build upon the code when necessary.
What is the main difference between a class and an instance of a class?
-A class serves as a blueprint for creating specific objects, while an instance is a unique object created from that class with its own set of attributes and methods.
What are instance variables and how do they relate to each instance of a class?
-Instance variables contain data that is unique to each instance of a class. They store the specific attributes of each object that is created from the class blueprint.
How is the 'init' method in a class used?
-The 'init' method is a special method used to initialize the instance variables of a class when a new instance is created. It is automatically called with the instance as its first argument, which is conventionally named 'self'.
What is the purpose of methods in a class?
-Methods in a class are functions associated with the class that allow instances to perform actions or operations. They help to encapsulate behavior and promote code reusability.
How can you create a method within a class to display an employee's full name?
-You can create a method called 'full_name' within the class that concatenates the instance variables 'first' and 'last' to display the full name. The method should return this concatenated string.
Why is the 'self' argument important in class methods?
-The 'self' argument is crucial because it represents the instance of the class when the method is called. It allows the method to access and modify the instance's attributes and other methods associated with it.
What happens if the 'self' argument is omitted from a method definition?
-If the 'self' argument is omitted, Python will raise a TypeError when the method is called because it expects the instance as the first argument to properly access the instance's attributes and methods.
How do you call a class method using the class name?
-To call a class method using the class name, you prefix the method name with the class name and use parentheses, manually passing the instance as an argument, like 'Classname.MethodName(instance)'.
What is the advantage of using the 'init' method over manually setting instance variables?
-Using the 'init' method allows for automatic initialization of instance variables when creating new instances, reducing the amount of code and the likelihood of errors that come from manual assignment.
How can you ensure that your class methods are reusable and less prone to mistakes?
-By encapsulating common behaviors and actions within methods, you reduce the need for repeating code and manual variable assignments, thus minimizing errors and making the code more maintainable and reusable.
Outlines
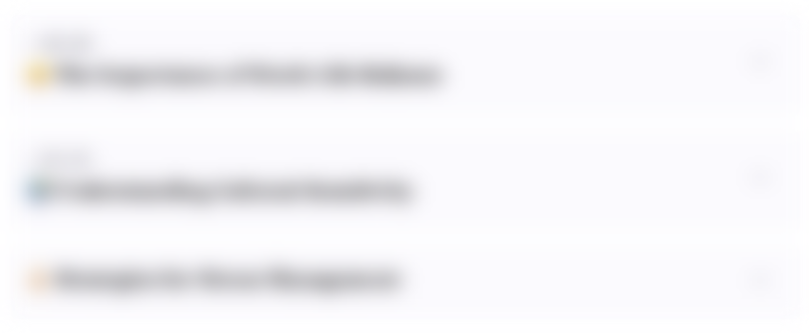
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
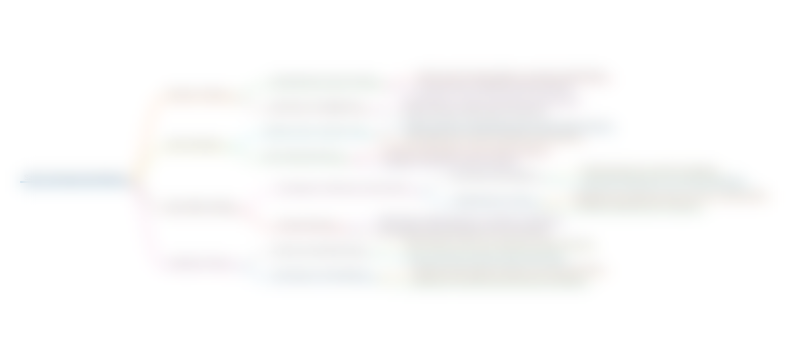
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
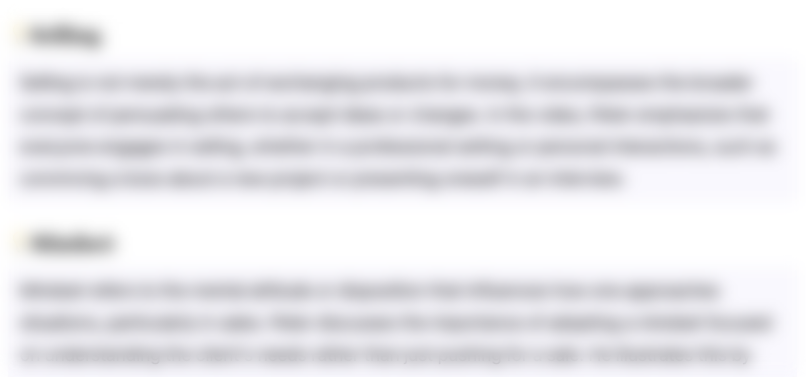
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
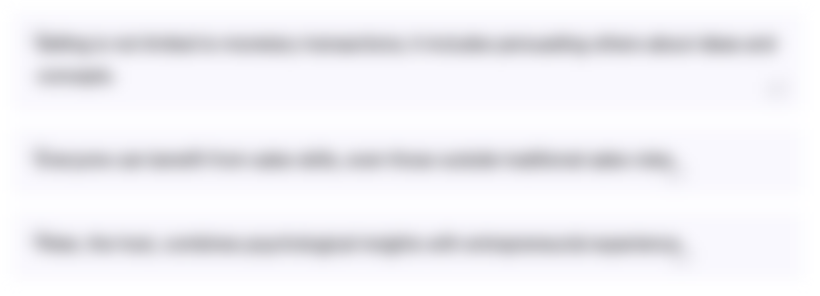
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
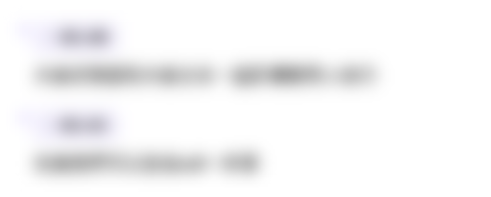
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
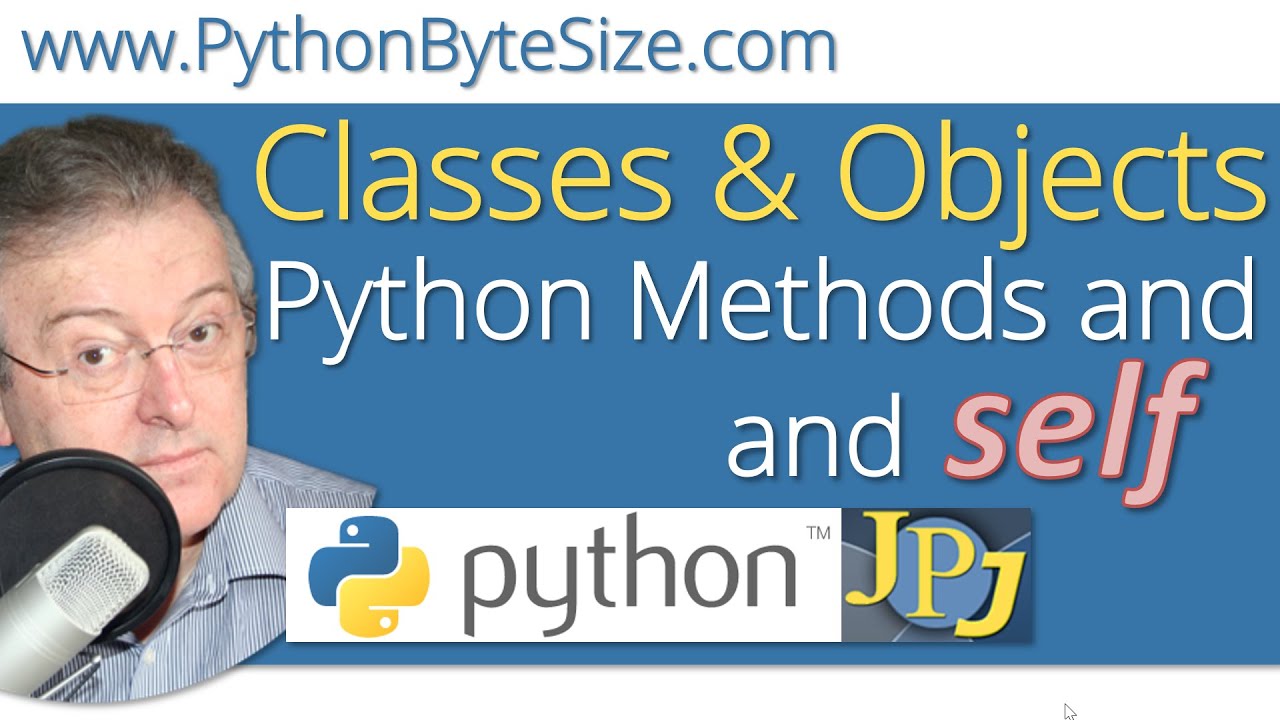
Python Methods and self
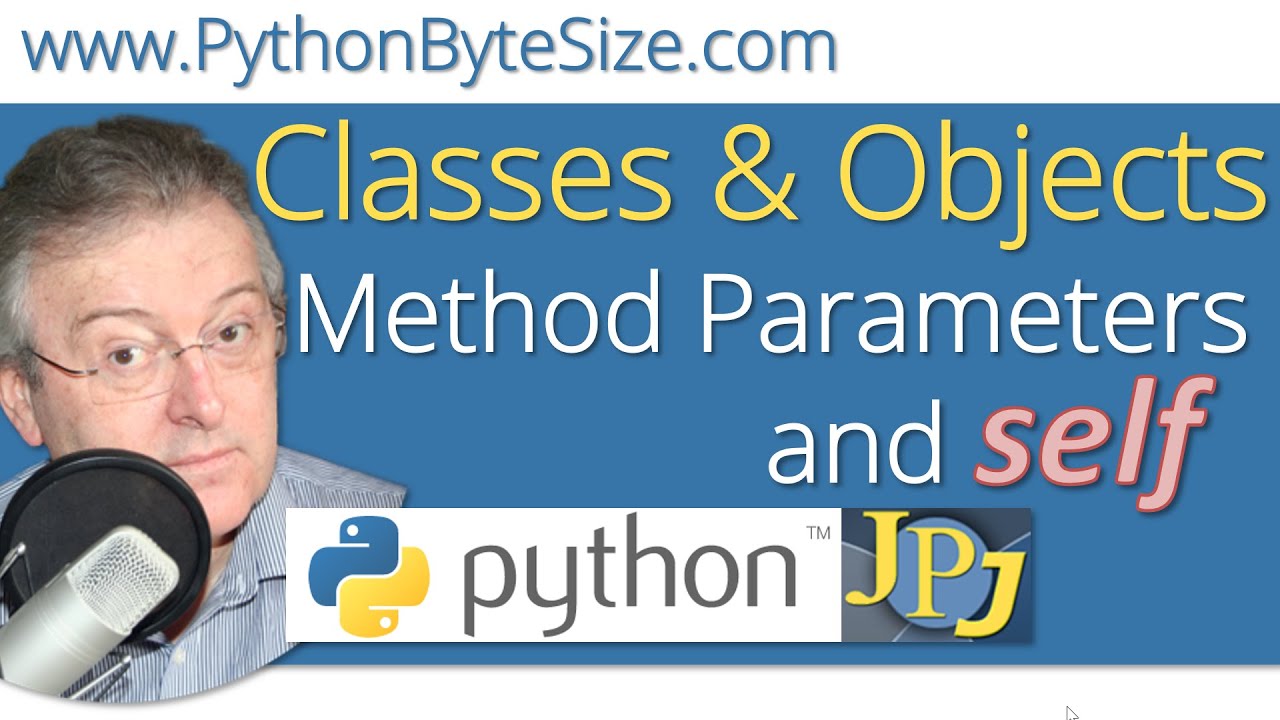
Python Method Parameters and self

Pythons self parameter

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85

Criando Mรฉtodos de Objetos - Curso Python Orientado a Objetos [Aula 03]
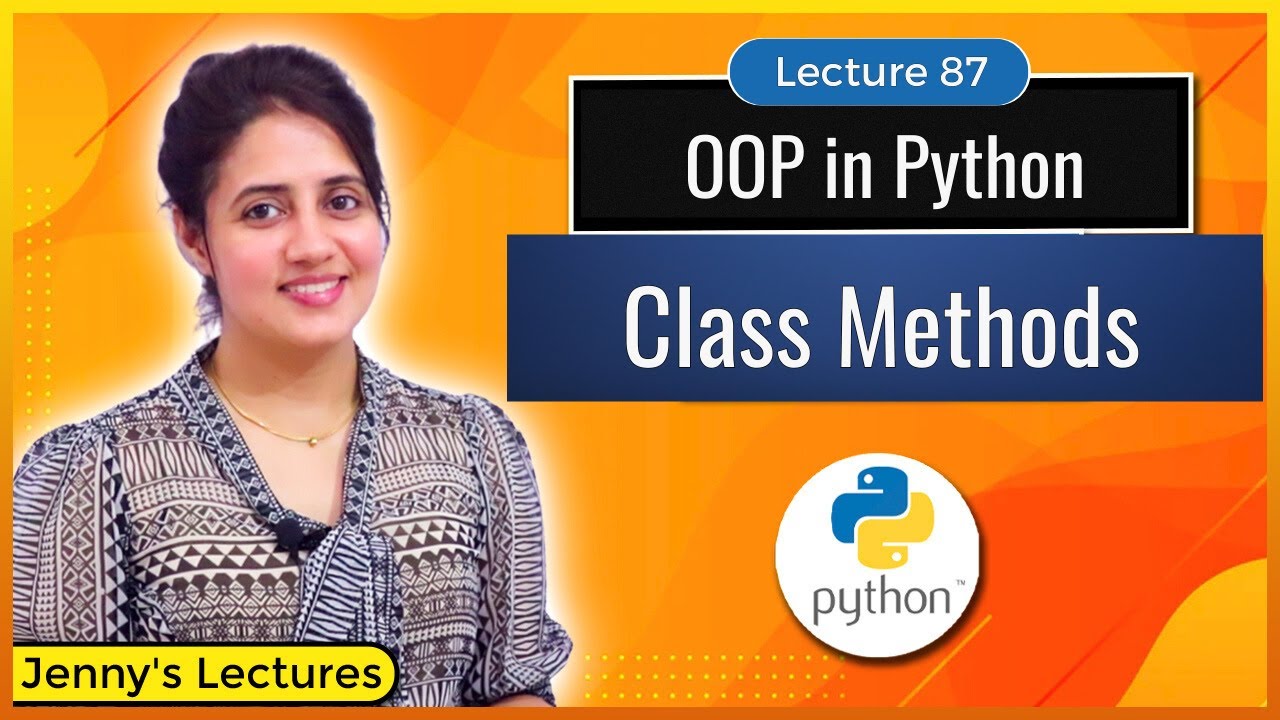
Class Methods in Python | How to add Methods in Class | Python Tutorials for Beginners #lec87
5.0 / 5 (0 votes)