10 Important Python Concepts In 20 Minutes
Summary
TLDRThis video introduces 10 essential Python concepts that every beginner should know. It covers topics such as variables, data types (integers, floats, strings, and more), type annotations, constants, and the creation of reusable code through functions and classes. The video also explains the importance of methods, including Dunder methods, to customize object behavior. Key concepts like the significance of functions, using classes as blueprints, and improving code safety with type annotations and constants are discussed. The tutorial provides a solid foundation for further exploration of Python programming.
Takeaways
- 😀 Download Python and create files with the '.py' extension to run Python code.
- 😀 Use variables to store data, and print them to display values in your program.
- 😀 Python supports various data types, including integers, floats, strings, booleans, lists, tuples, sets, and dictionaries.
- 😀 Type annotations in Python help clarify the expected types of variables and functions, though they don't affect the execution of the program.
- 😀 Constants can be represented in Python by using the 'final' type annotation and the uppercase naming convention.
- 😀 Functions in Python allow you to create reusable code, making it easier to avoid code duplication and maintain consistency across the program.
- 😀 Classes in Python serve as blueprints for creating objects, and can store data (attributes) and define behavior (methods) for those objects.
- 😀 Methods inside classes can access and modify object-specific data using the 'self' keyword, enabling custom behavior for each instance of the class.
- 😀 Dunder methods (e.g., '__str__', '__add__') are special methods that allow you to define custom behavior for operators and string representations in Python.
- 😀 Understanding and using dunder methods allows for more intuitive use of operators, such as adding objects together or printing them with custom formats.
Q & A
What is the purpose of downloading Python from python.org or another source?
-Downloading Python allows you to install the Python interpreter on your computer, which enables you to run Python scripts and programs locally.
How do you create a Python file and what extension should it have?
-To create a Python file, you can simply name the file with a '.py' extension (e.g., 'main.py'). This extension tells the Python interpreter that it is a Python script.
What are the basic data types in Python?
-The basic data types in Python include integers, floats, strings, booleans, lists, tuples, sets, and dictionaries.
What is the difference between a list and a tuple in Python?
-A list is mutable, meaning you can change its contents by adding or removing elements, while a tuple is immutable, meaning once it is created, its contents cannot be modified.
What are type annotations in Python and what is their purpose?
-Type annotations are optional in Python and allow developers to specify the expected data type of variables and function parameters. They help improve code readability and prevent certain types of errors but do not affect the program's execution.
How can you define constants in Python?
-Python does not have built-in constant support, but constants can be defined by using uppercase variable names, following the convention. You can also use type annotations with the 'final' keyword from the typing module to indicate that a value should not be reassigned.
What is the advantage of using functions in Python?
-Functions allow you to write reusable blocks of code, which can simplify maintenance and reduce redundancy. By defining a function, you can execute the same logic multiple times without copying and pasting code.
What are classes in Python, and how do they help in organizing code?
-A class in Python is a blueprint for creating objects with shared properties and methods. It allows you to encapsulate data and behavior together, making your code more modular and easier to maintain.
What is the 'self' keyword used for in Python classes?
-The 'self' keyword refers to the instance of the class itself. It is used to access instance attributes and methods within the class and distinguish them from local variables or methods.
What are Dunder methods, and how are they used in Python?
-Dunder methods (short for 'double underscore') are special methods in Python that allow you to customize the behavior of built-in operations. For example, the '__str__' method is used to define the string representation of an object, and the '__add__' method allows you to define how objects of a class can be added together.
Outlines
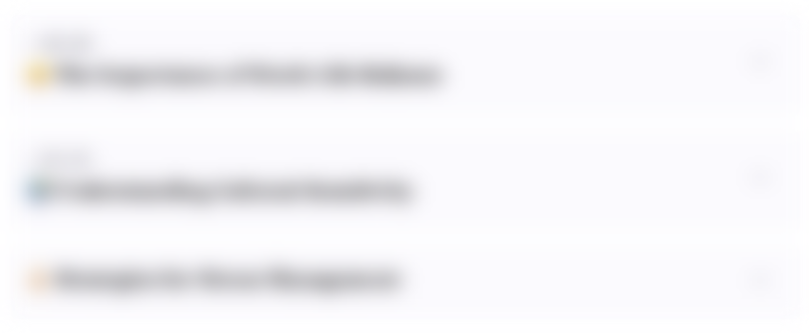
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
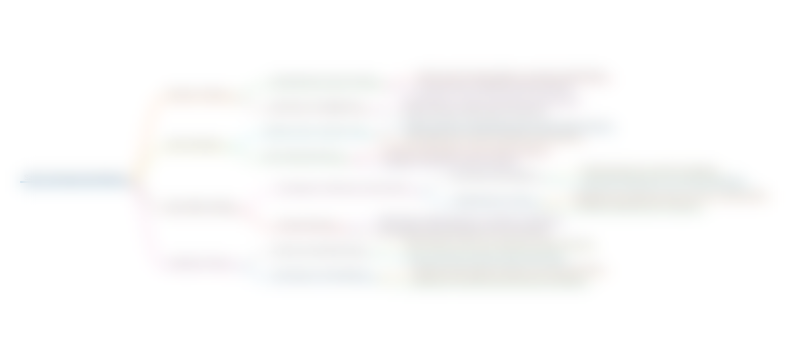
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
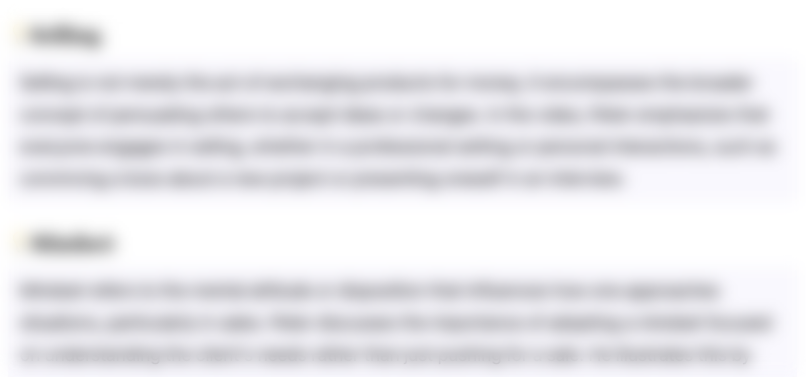
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
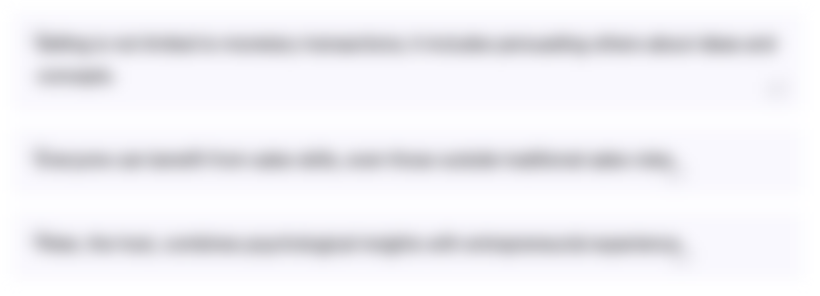
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
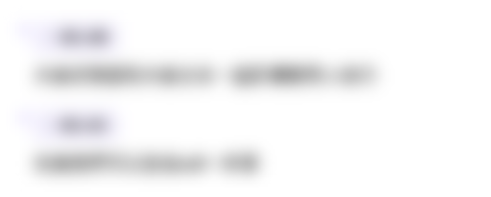
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)