Criando Métodos de Objetos - Curso Python Orientado a Objetos [Aula 03]
Summary
TLDRThis video tutorial introduces object-oriented programming (OOP) concepts in Python, focusing on methods within classes. It guides viewers through creating classes, instantiating objects, and working with attributes. The tutorial emphasizes how to encapsulate business logic within methods to avoid repetitive code. Through practical exercises, viewers learn to calculate product profit using both manual calculations and functions, then refactor the function into a method using the `self` parameter. The lesson also explores string formatting and adding new attributes to objects. By the end, viewers understand how methods improve code modularity and maintainability, with a preview of upcoming lessons on class methods.
Takeaways
- 😀 Method in OOP encapsulates functionality specific to an object, allowing reuse without repeating code.
- 😀 The `calcula_lucro` method allows calculating profit for any product object without retyping the calculation logic.
- 😀 Functions are general-purpose, while methods are tied to a specific class or object and can access its attributes directly via `self`.
- 😀 Using methods improves code organization by encapsulating related functionality within a class, leading to better maintainability.
- 😀 `self` is crucial in methods, as it refers to the specific instance of the class, allowing access to instance attributes like `valor_custo` and `valor_venda`.
- 😀 Converting a function to a method involves adding `self` as the first parameter and removing external parameters, as they are now accessible through the object.
- 😀 The `__init__` method initializes object attributes like `nome`, `valor_custo`, and `valor_venda`, ensuring proper object setup.
- 😀 The use of formatted strings (`.2f`) ensures that numerical values like prices are consistently displayed with two decimal places.
- 😀 The video emphasizes the importance of object-oriented practices in Python, especially for simplifying code and avoiding duplication.
- 😀 A well-structured class with methods can encapsulate business logic, making it easier for developers to maintain and extend the system as needed.
Q & A
What is the first prerequisite for understanding methods in object-oriented programming (OOP)?
-The first prerequisite is that you should be familiar with creating classes, objects, and manipulating their attributes. If you're not clear on these concepts, it's recommended to revisit earlier lessons on these topics.
What is the main purpose of methods in object-oriented programming?
-Methods are functions that belong to a class and are used to interact with or manipulate an object's attributes. They provide a way to encapsulate functionality that is specific to an object, improving code organization and reusability.
Why was there a need to refactor the profit calculation from a function to a method within the product class?
-Refactoring the profit calculation from a standalone function to a method within the class helps encapsulate the logic within the object itself. This prevents the need to repeatedly pass parameters (such as cost and sale price) and ensures that each product object can compute its own profit based on its attributes.
What role does the 'self' parameter play in a method within a class?
-The 'self' parameter refers to the current instance of the object that is calling the method. It allows the method to access the object's attributes and makes the method specific to the object that invoked it.
How is the method 'calcular_lucro' invoked in the tutorial, and what does it do?
-The 'calcular_lucro' method is invoked by calling it on a specific product object, such as 'produto.calcular_lucro()'. It calculates the profit by subtracting the product's cost from its sale price, both of which are accessed through the 'self' parameter.
What difference does using a method (like 'calcular_lucro') make compared to directly performing calculations inside the code?
-Using a method encapsulates the calculation logic, making the code cleaner and easier to maintain. It reduces redundancy by allowing the same calculation to be reused across different product objects without duplicating the code each time.
What is the significance of using string formatting (e.g., '{:.2f}') in the tutorial?
-String formatting with '{:.2f}' ensures that the product's price is always displayed with two decimal places, which is important for financial data presentation. It guarantees a consistent output, even when the value doesn't have two decimal points initially.
How can the concept of 'encapsulation' benefit a software development project?
-Encapsulation helps in hiding the internal workings of an object and exposes only necessary methods to interact with the object. This improves code maintainability, reduces errors, and makes the code easier to manage by isolating specific functionalities within objects.
How would you modify the 'Produto' class to add a 'nome' (name) attribute, and what changes are necessary?
-To add a 'nome' attribute, the class constructor should be updated to accept a 'nome' parameter, and this parameter should be saved as an instance attribute (e.g., 'self.nome'). Additionally, a method should be created to return a string with the product's name and price, using string formatting for the price.
What will be the outcome if you attempt to access an attribute like 'valor_venda' outside of a class method, without referencing 'self'?
-If you try to access an attribute like 'valor_venda' without referencing 'self', Python will not recognize the attribute as it belongs to the specific instance of the object. You must use 'self' to access object attributes within methods.
Outlines
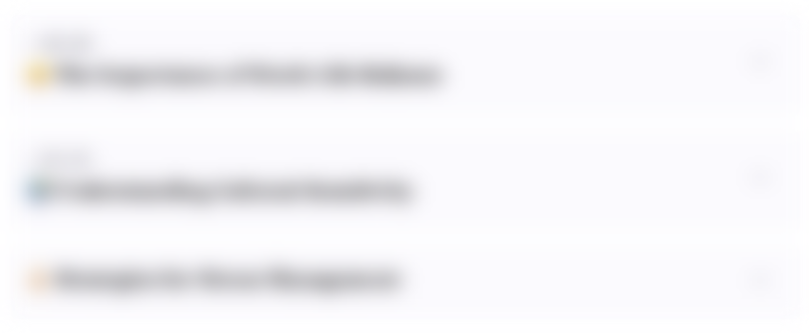
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
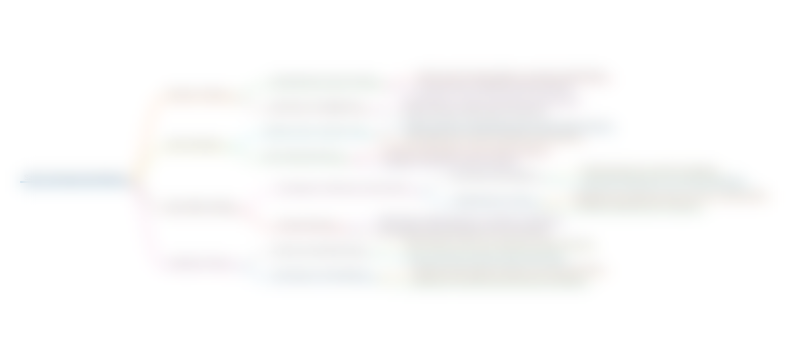
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
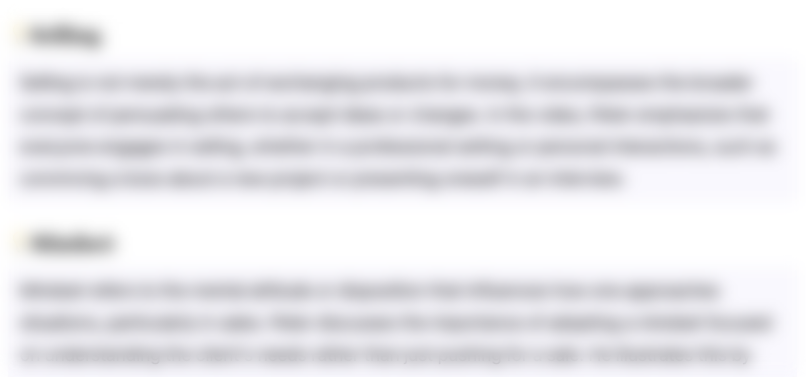
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
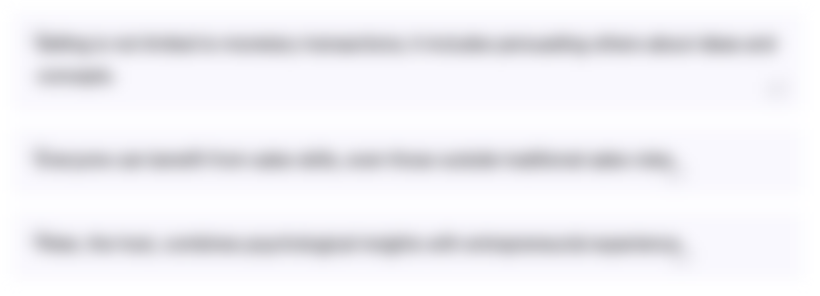
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
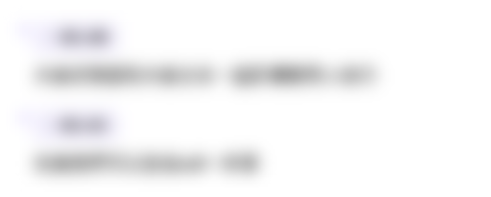
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
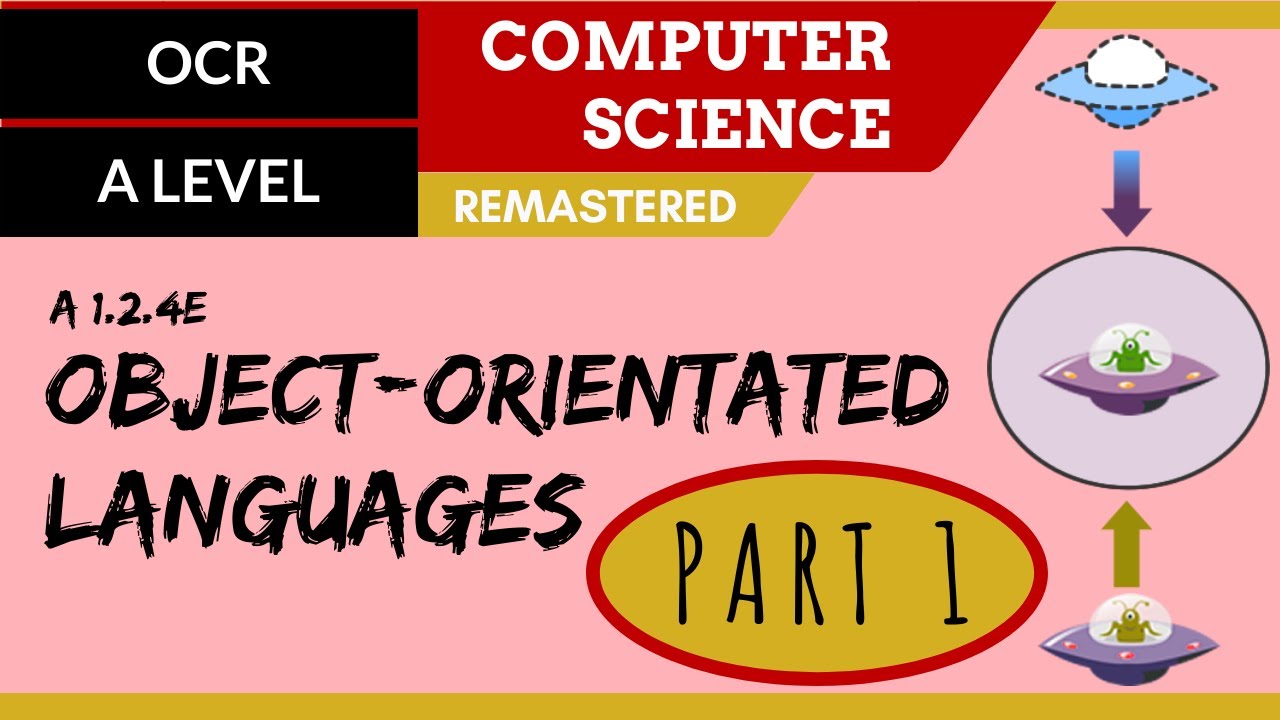
36. OCR A Level (H446) SLR7 - 1.2 Object-oriented languages part 1

Classes and Objects in Python | OOP in Python | Python for Beginners #lec85

Self and __init__() method in Python | Python Tutorials for Beginners #lec86
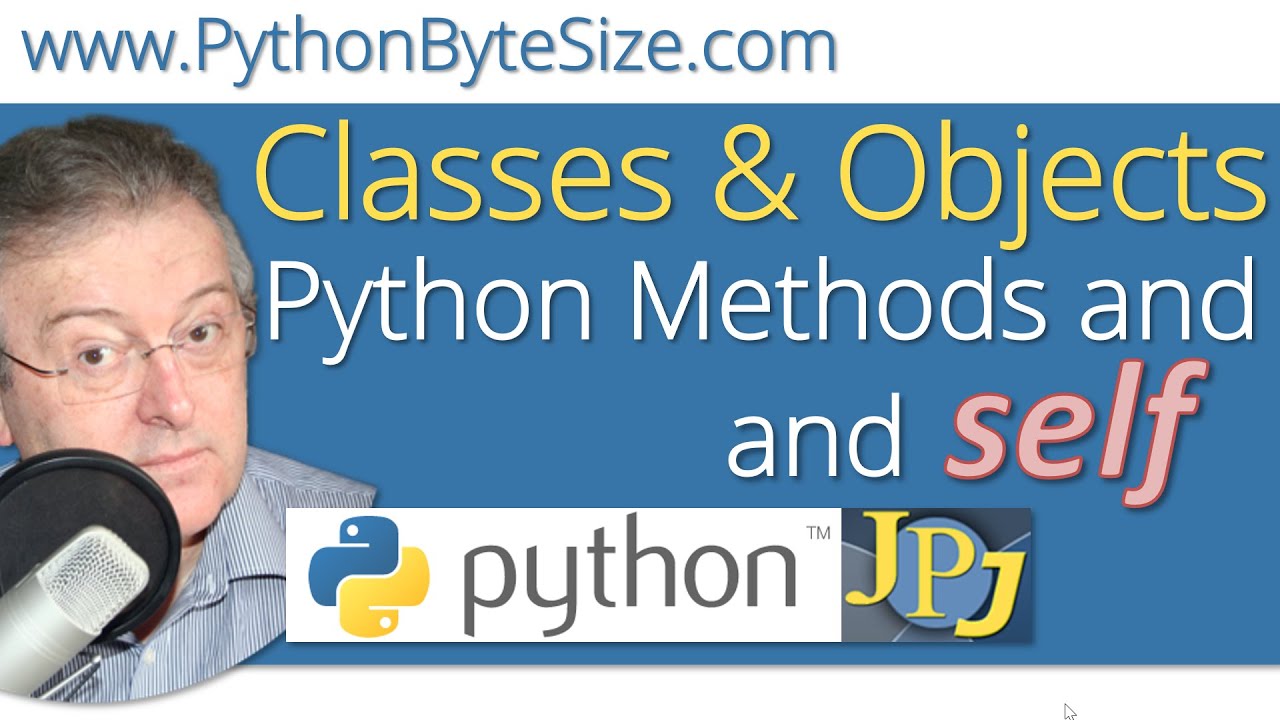
Python Methods and self
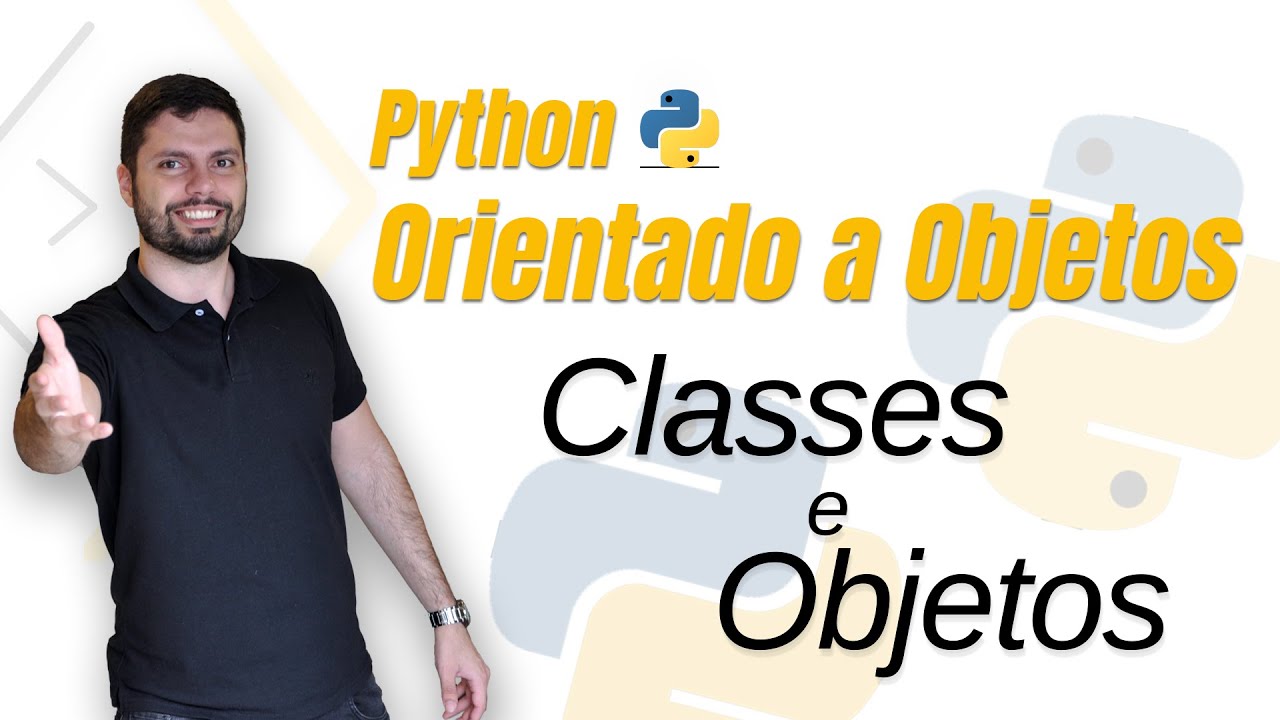
Como criar Classes e Objetos - Curso Python Orientado a Objetos [Passo a Passo]

Pythons self parameter
5.0 / 5 (0 votes)