Interfaces vs Abstract Classes
Summary
TLDRThis video script delves into the nuances of abstract classes and interfaces, contrasting their syntactical differences and use cases. It highlights that while both cannot be instantiated and can contain static members, they serve different purposes in code design. Abstract classes encapsulate commonality among related entities, whereas interfaces decouple components, enhancing flexibility. The script uses analogies like shapes and vehicles to illustrate concepts and discusses scenarios where one might be favored over the other, emphasizing the trade-offs between inheritance and composition in structuring code for maintainability and flexibility.
Takeaways
- 📜 Abstract classes and interfaces are common interview topics and are used to encapsulate common behaviors or roles in code.
- 🔍 The syntactical difference lies in that interfaces can only have public methods and properties, while abstract classes allow for various access modifiers and constructors.
- 🚫 Neither abstract classes nor interfaces can be instantiated directly.
- 🔗 A class can implement multiple interfaces but can only inherit from one abstract class due to the concept of identity.
- 🎯 Abstract classes are used when there is a commonality among classes, while interfaces are chosen for their role in decoupling components and increasing flexibility.
- 📈 Interfaces can provide scope control and enable immutability, whereas abstract classes cannot offer this level of control.
- 🏗️ Traits or roles, a programming paradigm, can be implemented using interfaces but not abstract classes, as a class can play multiple roles through interfaces.
- 🔄 In scenarios where additional responsibilities are anticipated, abstract classes might be more suitable than interfaces.
- 🔧 Inheritance leads to succinct code but may limit reusability, whereas composition with interfaces allows for greater flexibility but can increase code volume.
- 🔎 The choice between inheritance and composition depends on the specific needs of the project, with composition generally preferred for its flexibility.
- 💡 Understanding the differences and appropriate use cases for abstract classes and interfaces is crucial for writing maintainable and flexible code.
Q & A
What is the primary purpose of discussing abstract classes and interfaces in the context of the video?
-The primary purpose is to clarify the syntactical differences, use cases, and to provide examples of when to use abstract classes or interfaces in programming, especially in the context of interviews and software design decisions.
What are some key syntactical differences between abstract classes and interfaces?
-Abstract classes can have access modifiers, default implementation methods, fields, and constructors. Interfaces, on the other hand, can only have public methods, default implementation methods (but not consumed within the implementing class), and properties (but without fields).
Why might you choose to use an interface over an abstract class in a particular scenario?
-You might choose an interface when you want to introduce more flexibility into your code by decoupling components, allowing for multiple roles or behaviors to be played by a class, and when you need to maintain a contract without enforcing a specific implementation.
How do abstract classes and interfaces handle the concept of immutability and state management?
-Interfaces can be used to enforce immutability by defining properties that can only be initialized and not reinitialized, effectively controlling the state of an object. Abstract classes do not inherently support this pattern without additional implementation.
What is the significance of the shape and vehicle analogies in understanding abstract classes?
-The analogies help to illustrate that a class can represent a general concept (like a shape or a vehicle) and share common properties or behaviors with other classes within that concept, which is the essence of using an abstract class to encapsulate commonality.
How can you explain the use of default implementation methods in abstract classes versus interfaces?
-In abstract classes, default implementation methods can be consumed within the inheriting class, whereas in interfaces, the default implementation cannot be directly accessed by the implementing class; instead, the class must provide its own implementation or use the default implementation from the interface.
What is the main advantage of using composition with interfaces over inheritance with abstract classes?
-Composition with interfaces provides greater flexibility, as it allows for the decoupling of components and the ability to mix and match behaviors in different contexts. It enables reusability of interfaces across various parts of an application, whereas inheritance may lead to tightly coupled and less flexible code structures.
Why might you prefer an abstract class in a scenario where multiple context-specific behaviors are needed?
-In such scenarios, abstract classes can be used to define a common structure or behavior that is shared across different contexts, making it easier to manage and reuse the shared logic without having to implement multiple interfaces for each context-specific behavior.
What are the trade-offs between using inheritance and composition in software design?
-Inheritance provides a succinct way to express related concepts and share common logic, but it can lead to tightly coupled code that is difficult to refactor. Composition, on the other hand, offers more flexibility and decoupling but may require more code and explicit orchestration of components.
How does the video script illustrate the concept of 'scopes' in relation to interfaces?
-The concept of 'scopes' is illustrated by showing how interfaces can define a set of behaviors or properties that are accessible only within a certain scope or state of an object, providing a structured way to control access and maintain immutability.
What is the role of traits or roles in object-oriented programming, and how do interfaces support this concept?
-Traits or roles represent specific behaviors or functionalities that an object might adopt within certain contexts. Interfaces support this concept by allowing a class to implement multiple interfaces, effectively playing multiple roles, which is not possible with abstract classes that enforce a single role or identity.
Outlines
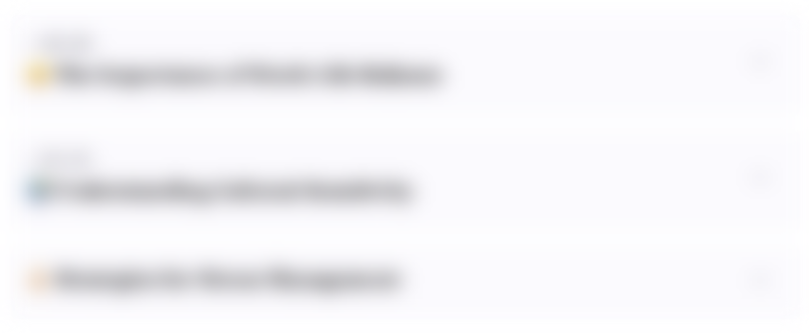
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
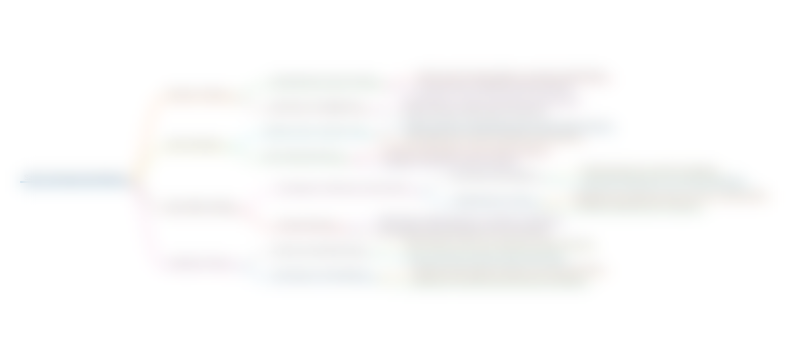
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
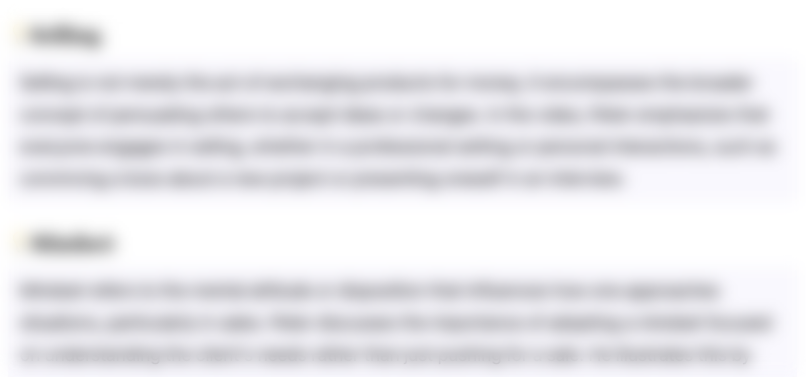
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
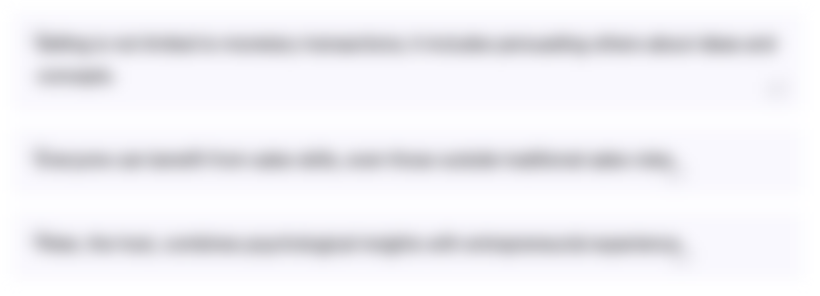
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
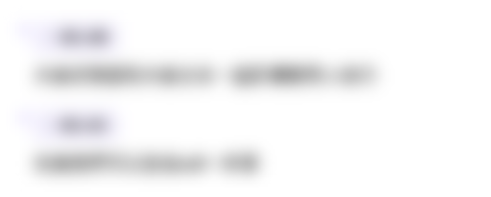
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
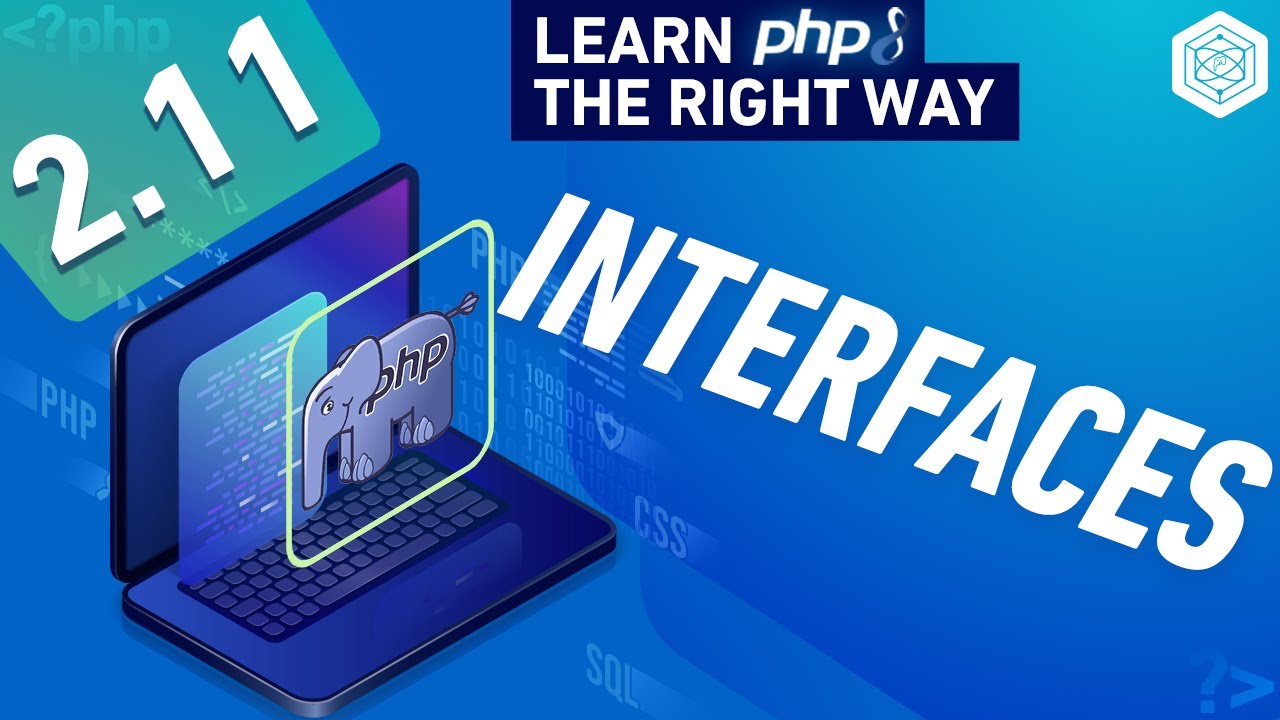
PHP Interfaces & Polymorphism - Interfaces Explained - Full PHP 8 Tutorial
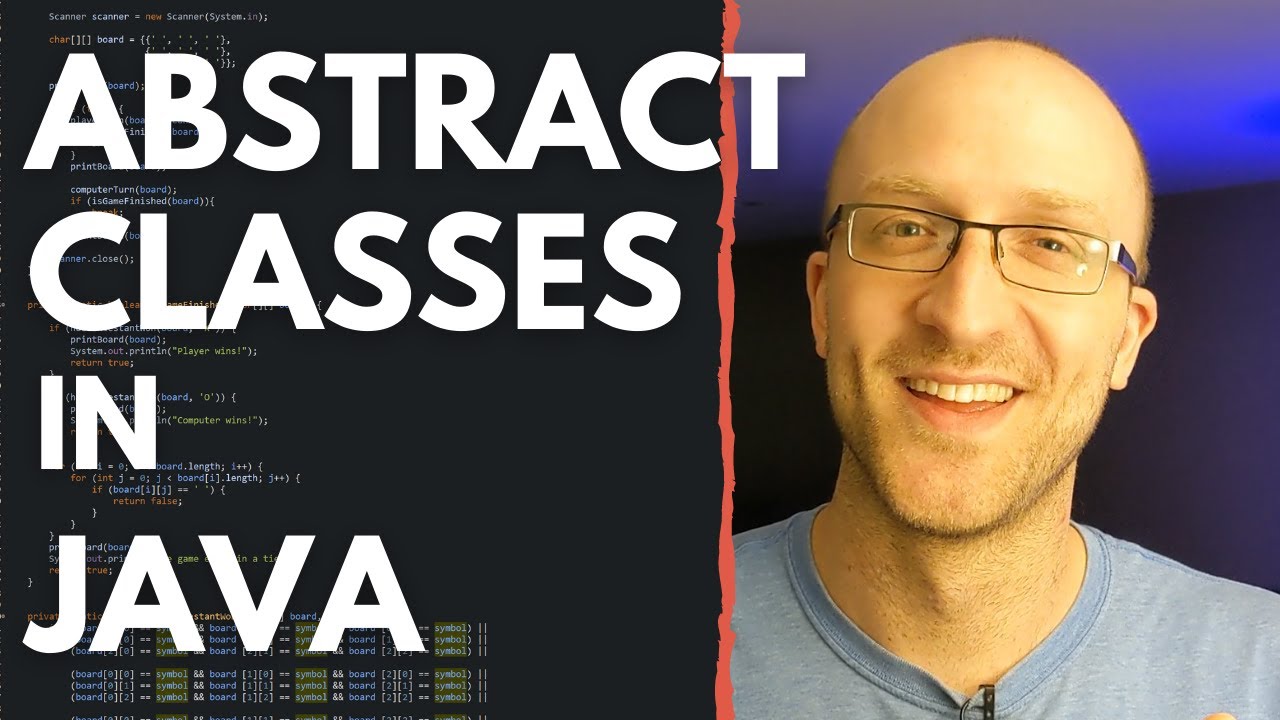
Abstract Classes and Methods in Java Explained in 7 Minutes
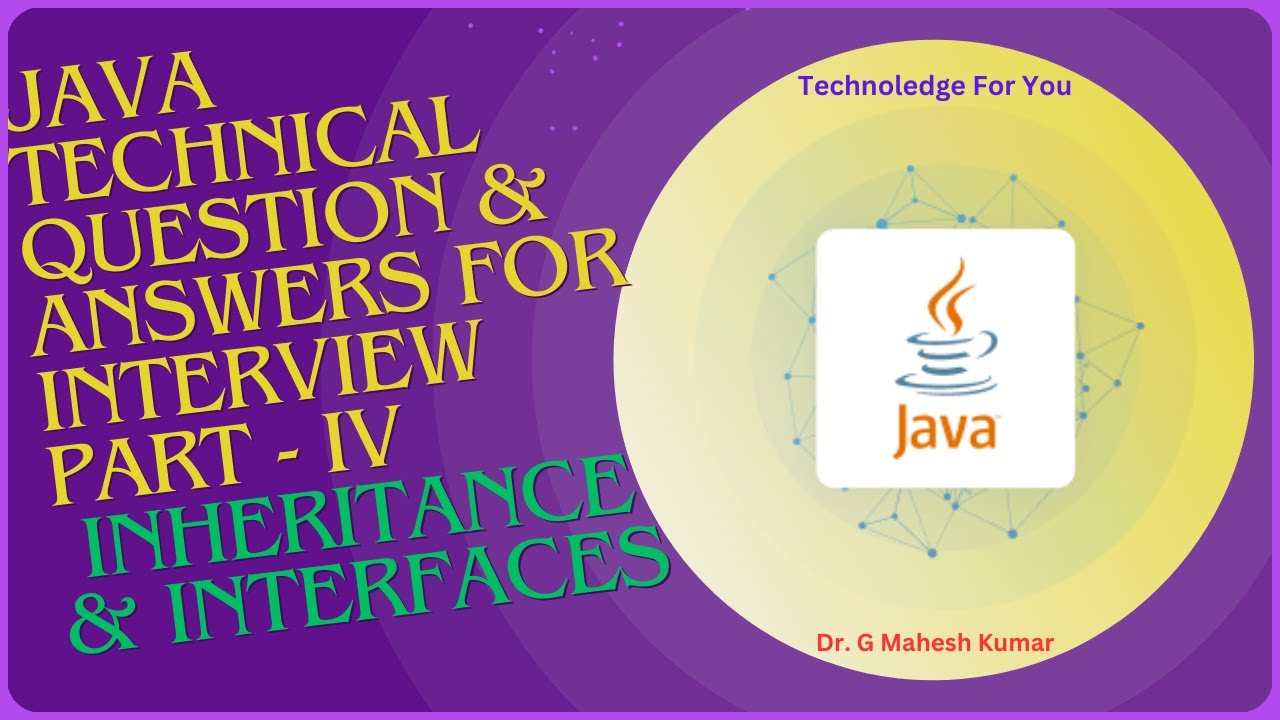
JAVA TECHNICAL QUESTION AND ANSWERS FOR INTERVIEW PART IV INHERITANCE & INTERFACES
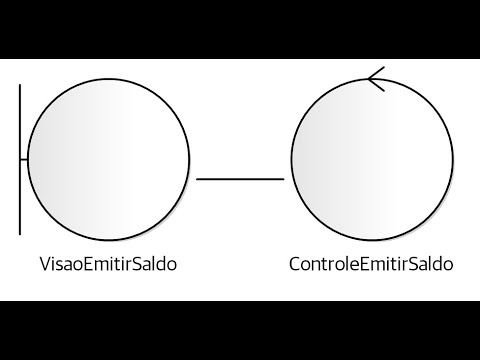
Estereótipos II - Nova Edição - Diagrama de Classes V
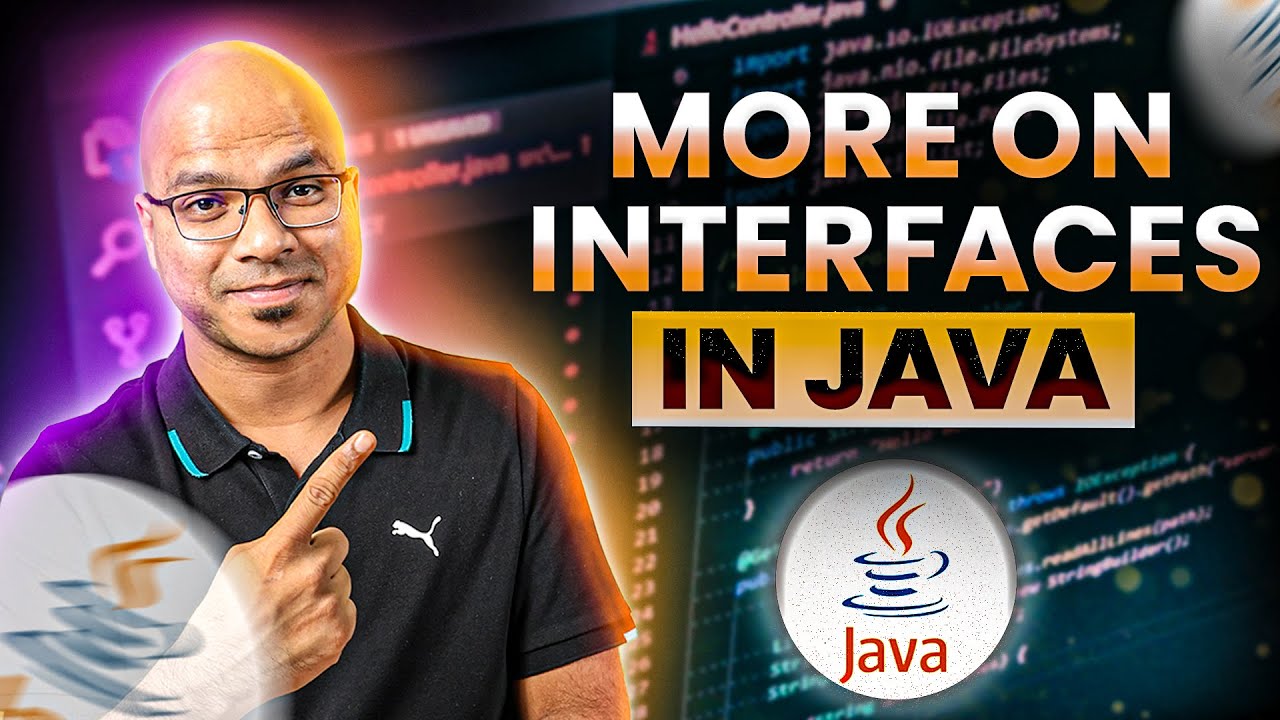
#67 More on Interfaces in Java

Computer Concepts - Module 4: Operating Systems and File Management Part 1A (4K)
5.0 / 5 (0 votes)