#67 More on Interfaces in Java
Summary
TLDRThe video script discusses the concept of interfaces in object-oriented programming, highlighting that a class can implement multiple interfaces but only extend one abstract class. It explains the use of 'implements' for classes and 'extends' for interfaces, emphasizing the need to define all methods declared in the interfaces a class implements. The script also clarifies that an interface can extend another, inheriting its methods. It concludes with the importance of using the correct reference type to access specific methods, illustrating the distinction between class and interface inheritance.
Takeaways
- 📚 A class can implement multiple interfaces, but it can only extend one abstract class.
- 🔍 When a class implements multiple interfaces, it must define all the methods declared in those interfaces to avoid errors.
- 🔑 The 'implements' keyword is used when a class is implementing an interface.
- 🔄 Interfaces can also extend other interfaces using the 'extends' keyword, allowing for inheritance of methods.
- 🚫 If an interface extends another, and a class implements the extending interface, it must provide implementations for all abstract methods from both interfaces.
- 🛑 An error will occur if a class does not implement all the required methods from the interfaces it claims to implement.
- 🔄 The concept of inheritance is valid for interfaces, allowing for the creation of a hierarchy of interfaces.
- 📝 When creating an object, the methods that can be called are limited to those defined in the interface through which the object is referenced.
- 🔍 If a method is defined in an interface, any class implementing that interface must also define that method.
- 🔄 The 'extends' keyword is used for both class-to-class and interface-to-interface relationships, indicating inheritance.
- 📌 It's important to remember the correct terminology: 'extends' for class-to-class or interface-to-interface, and 'implements' for class-to-interface.
Q & A
Can a class implement multiple interfaces?
-Yes, a class can implement multiple interfaces. This is demonstrated in the script where Class B implements both Interface A and Interface X without any errors.
What happens if a class implements multiple interfaces and there is a method name conflict?
-If there is a method name conflict, the class must define the conflicting method as well. The script shows an error when the 'run' method is not defined in Class B, which implements two interfaces with a 'run' method.
What is the difference between a class implementing an interface and a class extending another class?
-A class can extend only one other class, but it can implement multiple interfaces. The script explains that while a class can have only one superclass, it can implement multiple interfaces.
How does a class implement multiple methods from different interfaces?
-A class can implement multiple methods from different interfaces by defining all the methods declared in those interfaces. The script shows Class B implementing three methods from two different interfaces.
Can interfaces extend other interfaces?
-Yes, interfaces can extend other interfaces using the 'extends' keyword. The script demonstrates Interface Y extending Interface X.
What is the consequence of an interface extending another interface?
-When an interface extends another, it inherits the methods of the parent interface. The script shows Interface Y inheriting the 'run' method from Interface X.
What error occurs if a class implements an interface that extends another interface but doesn't implement all required methods?
-The class must implement all abstract methods from the interfaces it implements. The script shows an error when Class B implements Interface Y but does not implement the 'one' method inherited from Interface X.
What is the correct syntax for a class to implement an interface?
-The correct syntax is to use the 'implements' keyword followed by the interface name. The script mentions this when discussing Class B implementing Interface X.
What is the correct syntax for an interface to extend another interface?
-The correct syntax is to use the 'extends' keyword followed by the interface name. The script demonstrates this with Interface Y extending Interface X.
Why can't a reference of a class object call methods from an interface that the class does not implement?
-A reference of a class object can only call methods that the class implements. The script explains that a reference of Class A cannot call the 'run' method because Class A does not implement Interface X, which contains the 'run' method.
How can a class object call methods from an interface it implements?
-A class object can call methods from an interface it implements by creating a reference of the interface type and assigning an object of the class to it. The script shows creating a reference of Interface X and assigning an object of Class B to it to call the 'one' method.
Outlines
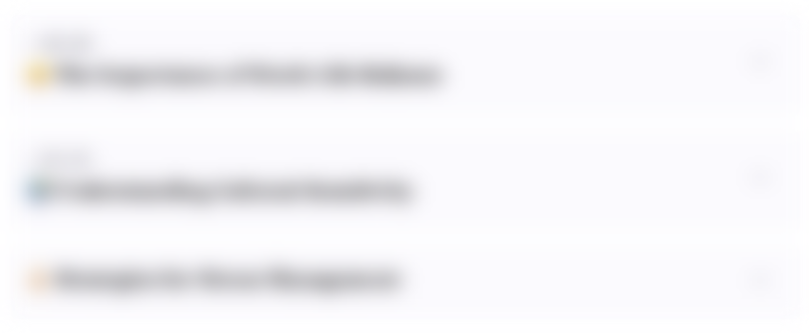
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
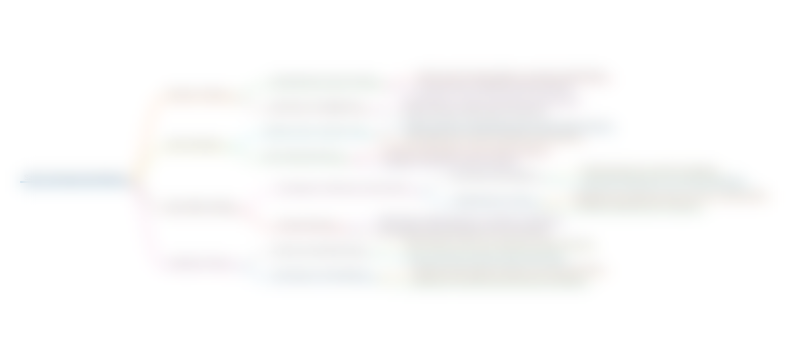
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
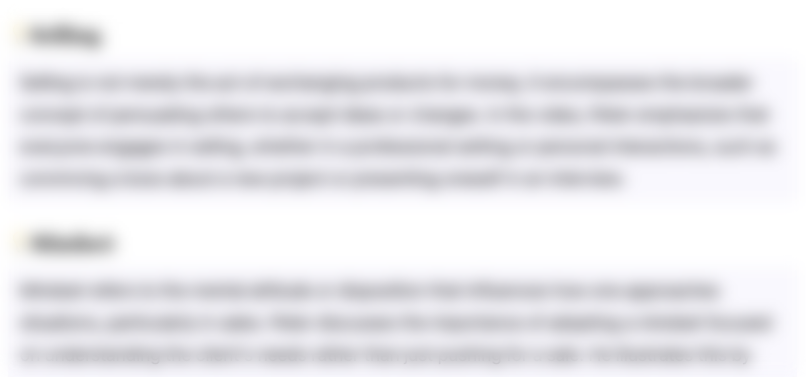
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
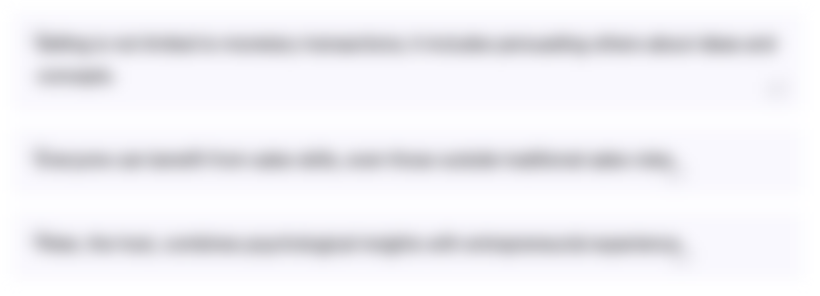
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
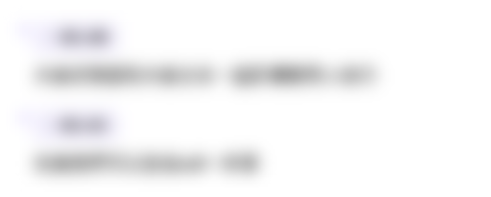
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
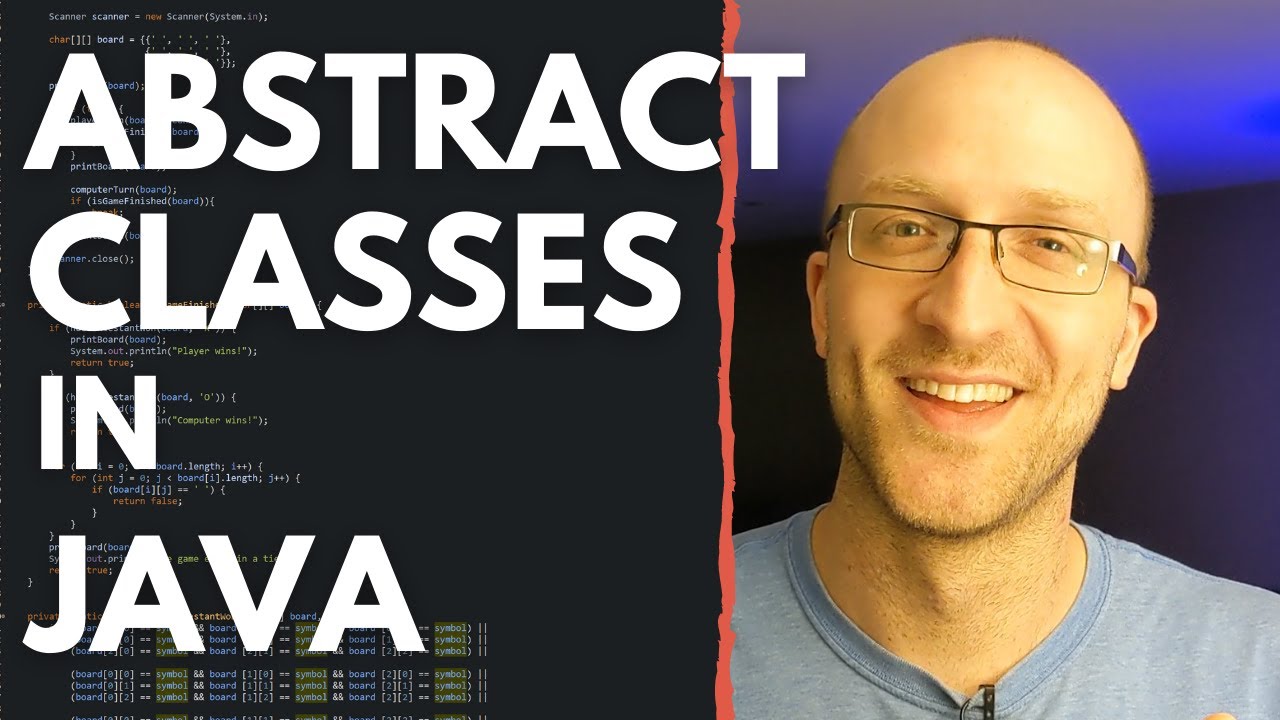
Abstract Classes and Methods in Java Explained in 7 Minutes
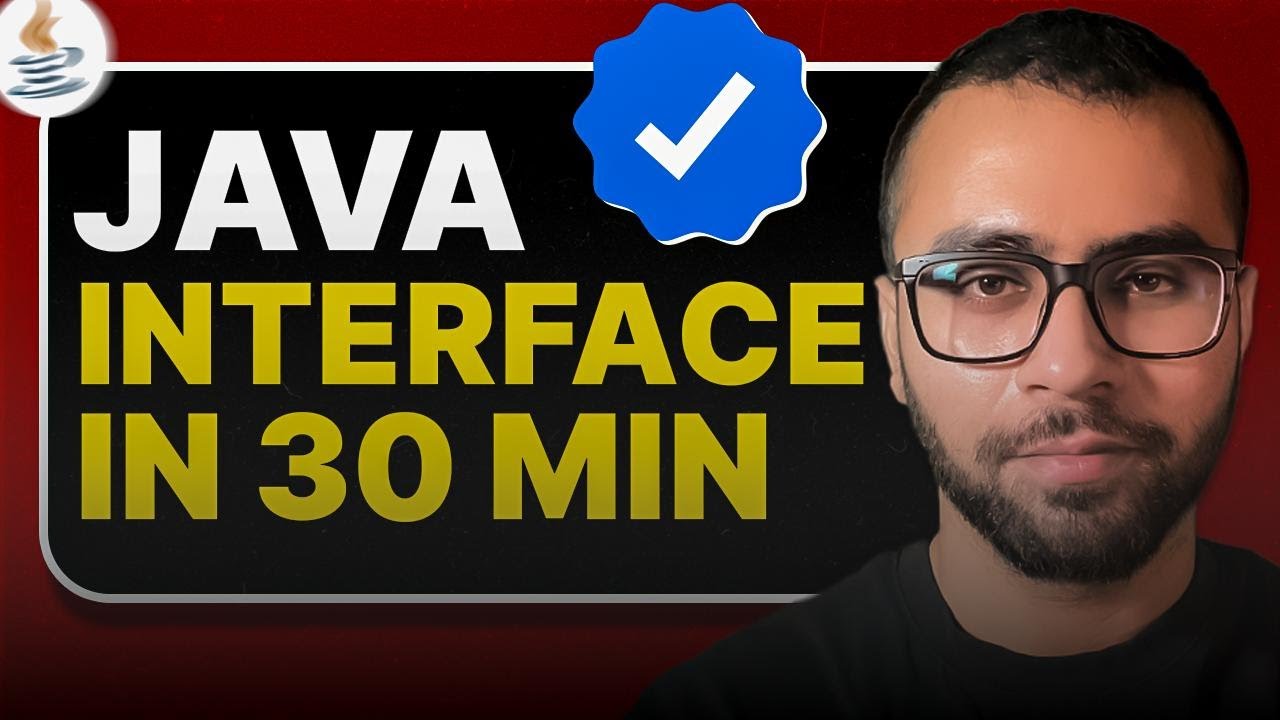
Mastering Java Interfaces: Static & Default Methods, Multiple Inheritance Explained
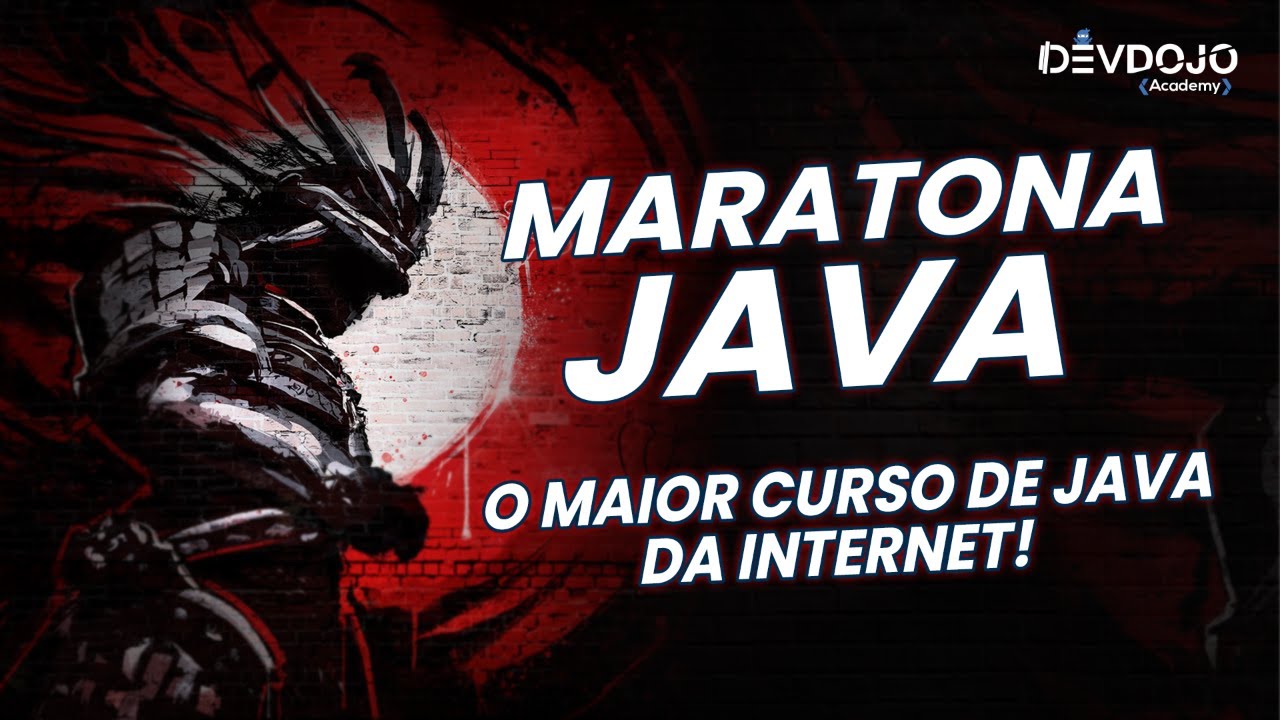
90 - Orientação Objetos - Polimorfismo pt 01 - Introdução
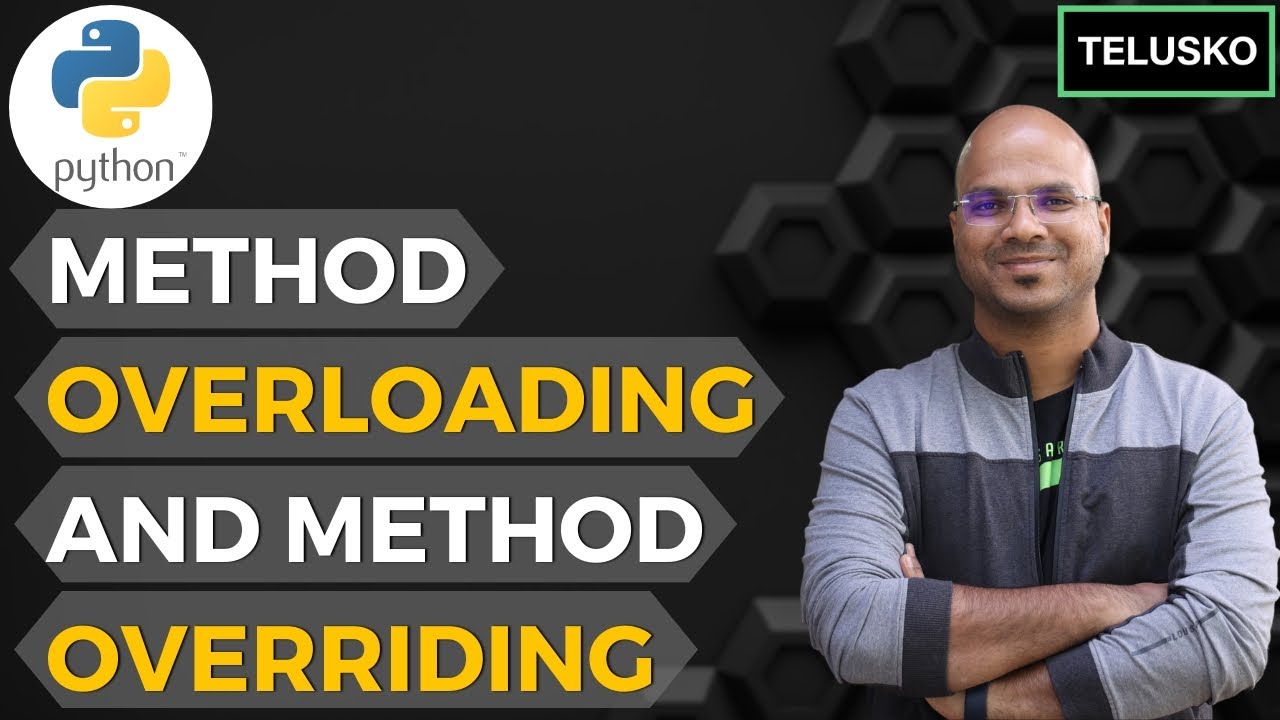
#60 Python Tutorial for Beginners | Method Overloading and Method Overriding
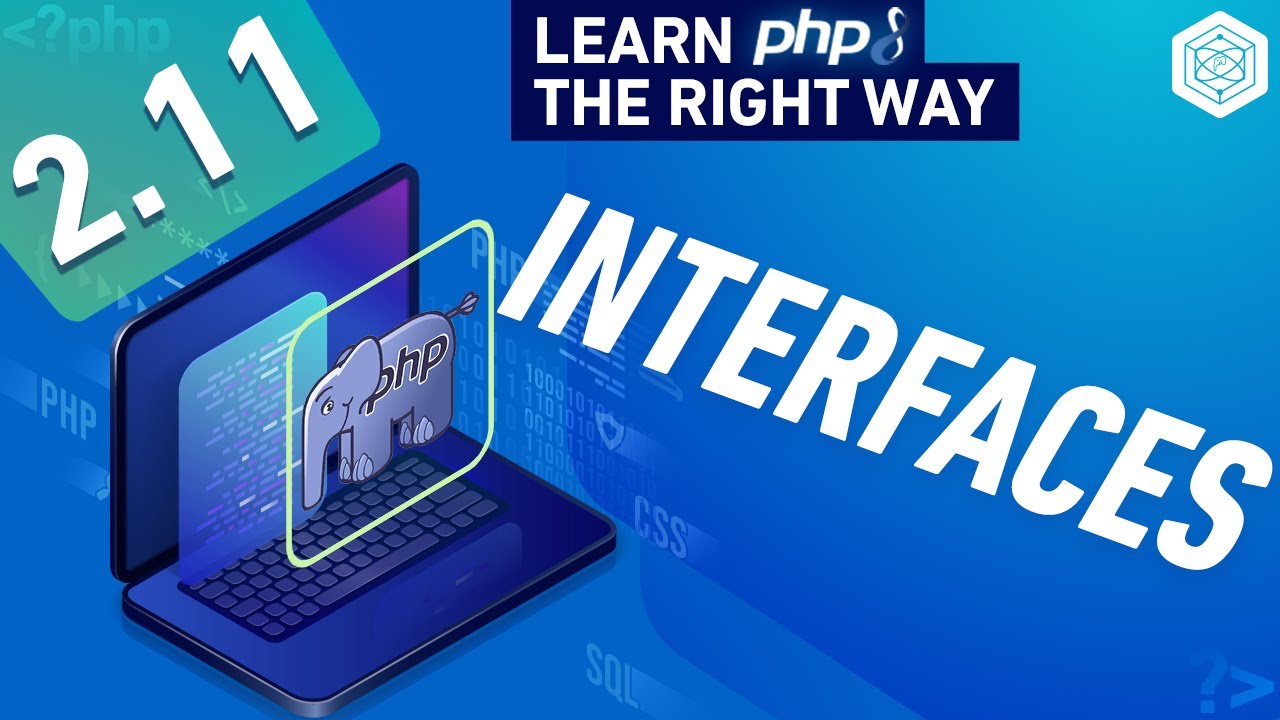
PHP Interfaces & Polymorphism - Interfaces Explained - Full PHP 8 Tutorial
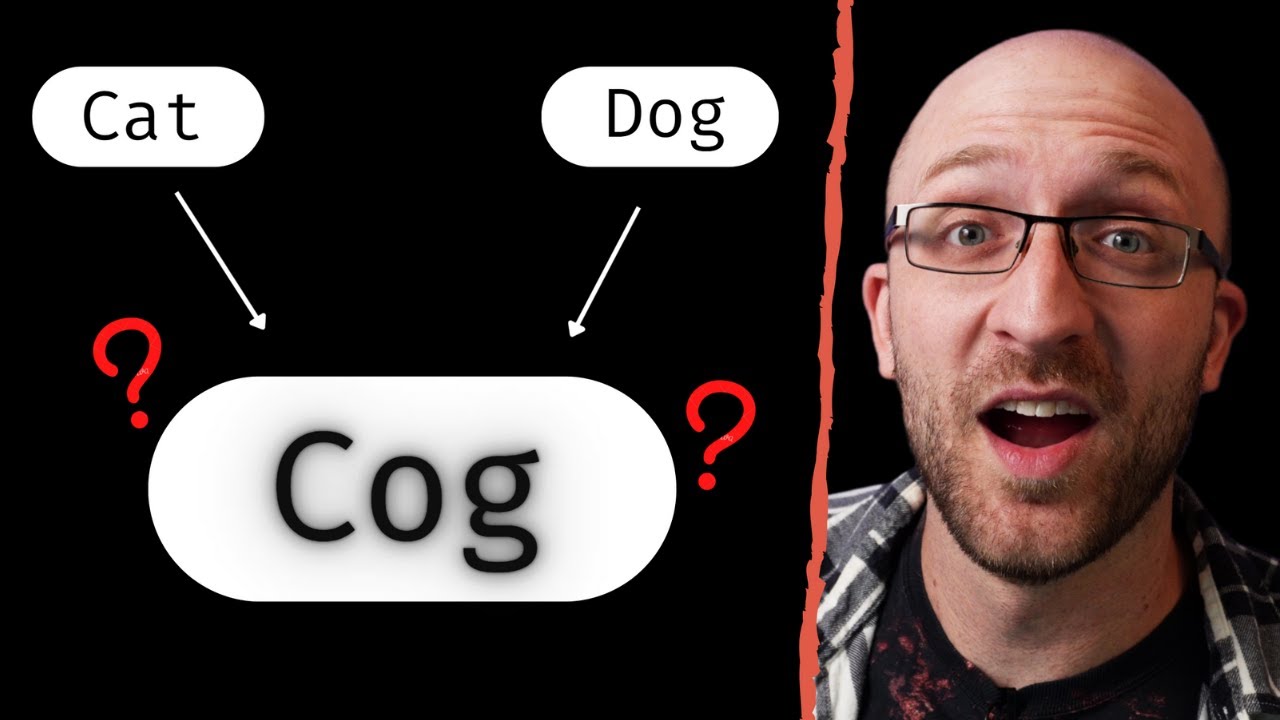
Java's Creators Rejected Multiple Inheritance - Here's Why
5.0 / 5 (0 votes)