Introduction To Lists In Python (Python Tutorial #4)
Summary
TLDRThis video from CS Dojo introduces Python lists, a versatile data structure similar to arrays in other languages. It demonstrates how to define, append, and manipulate lists, including adding mixed types and nested lists. The tutorial covers common list methods like 'append', 'pop', and indexing, and provides a practical exercise to swap elements within a list. The video also offers resources for further learning and support.
Takeaways
- 📝 Introduction to Lists: The script introduces lists in Python, a data type used to store a collection of items similar to arrays in other languages.
- 🔑 Defining a List: A list in Python can be defined using square brackets, with elements separated by commas.
- 📈 Appending Items: The 'append()' method is used to add items to the end of a list.
- 🔄 Type Flexibility: Python lists can contain elements of different data types, including numbers, strings, and even other lists.
- 📚 Nested Lists: A list can include another list as an element, creating a nested structure.
- ➖ Removing Items: The 'pop()' method removes the last item from a list, but can also be used to remove an item at a specific index.
- 🔑 Indexing: Items in a list can be accessed using zero-based indexing, with the first item at index 0.
- 🛠 Modifying Items: Elements in a list can be changed by selecting the item with its index and assigning a new value to it.
- 🔍 Retrieving Items: Specific items in a list can be retrieved using their index within square brackets.
- 🔄 Swapping Elements: The script demonstrates how to swap two elements in a list using temporary variables or a shortcut method.
- 📌 Additional Methods: The script mentions that there are many other predefined functions for lists in Python, with 'append()' and 'pop()' being among the most common.
- 📚 Learning Resources: The video provides a link to download sample files and suggests using an IDE like PyCharm for Python development.
- 🤝 Community Support: The script encourages viewers to support the channel through Patreon and provides a link for the same.
Q & A
What is a list in Python?
-A list in Python is a type of data structure similar to arrays in other languages, used to store a collection of items.
How do you define a list in Python?
-You can define a list in Python by using square brackets and separating elements with commas, e.g., a = [3, 10, -1].
Can you provide an example of how to assign a list to a variable in Python?
-Yes, you can assign a list to a variable using the syntax 'variable_name = [elements]'. For example, 'a = [3, 10, -1]' assigns a list with the elements 3, 10, and -1 to the variable 'a'.
How can you add an item to a list in Python?
-You can add an item to a list using the 'append()' method, like 'a.append(1)', which appends the number 1 to the list 'a'.
What is the dot notation used for in Python lists?
-The dot notation in Python is used to call methods on objects. For lists, it is used to call predefined functions like 'append()' and 'pop()'.
Can you mix different data types in a single list in Python?
-Yes, Python lists are dynamic and can contain elements of different data types, such as numbers and strings.
How do you append a string to a list in Python?
-You can append a string to a list using the 'append()' method followed by the string in quotes, like 'a.append("hello")'.
What is the purpose of the 'pop()' method in Python lists?
-The 'pop()' method is used to remove and return the last item from a list. It can also be used with an index to remove an item at a specific position.
How can you retrieve a specific item from a list in Python?
-You can retrieve a specific item from a list using indexing, e.g., 'a[0]' retrieves the first item in the list 'a'.
How do you change the content of a list item in Python?
-You can change the content of a list item by indexing the item with its position and assigning a new value to it, like 'a[0] = 100' changes the first item to 100.
What is the concept of a nested list in Python?
-A nested list in Python is a list that contains other lists as its elements. It allows for more complex data structures within a list.
Can you provide an example of how to swap two elements in a list in Python?
-Yes, you can use a temporary variable to hold one element and then swap the elements using their indices, e.g., 'temp = b[0]; b[0] = b[2]; b[2] = temp' swaps the first and last elements of the list 'b'.
Is there a shortcut for swapping two elements in a list in Python?
-Yes, you can swap two elements in a list using a single line of code: 'b[0], b[2] = b[2], b[0]', which directly swaps the elements without the need for a temporary variable.
Where can I find the sample file mentioned in the video script?
-You can download the sample file by visiting the website 'CSdojo.io/Python4'.
What is the recommended IDE for Python mentioned in the script?
-The script recommends using 'PyCharm' as a good IDE for Python.
How can I support the creator of the video?
-You can support the creator through Patreon by visiting 'CSdojo.io/PT'.
Outlines
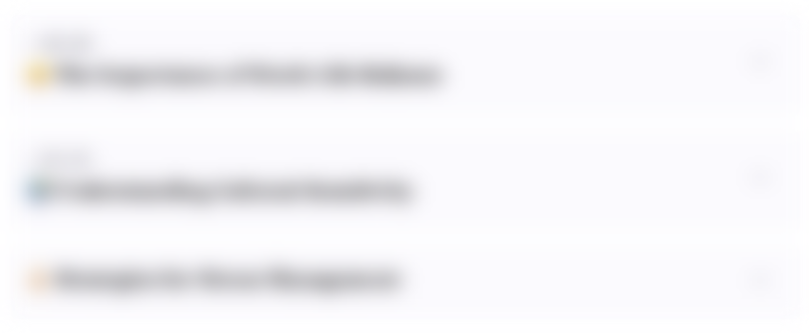
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
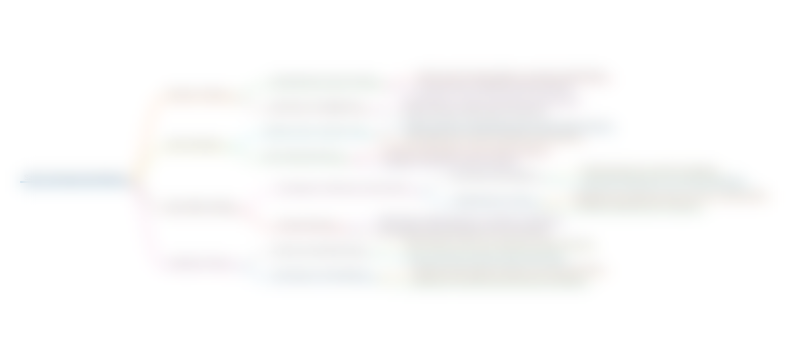
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
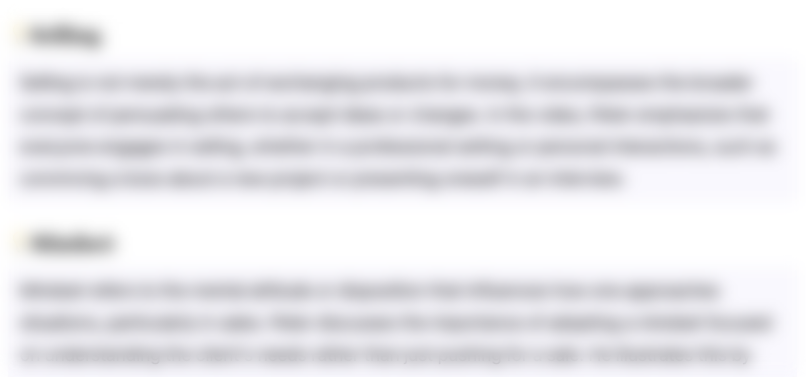
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
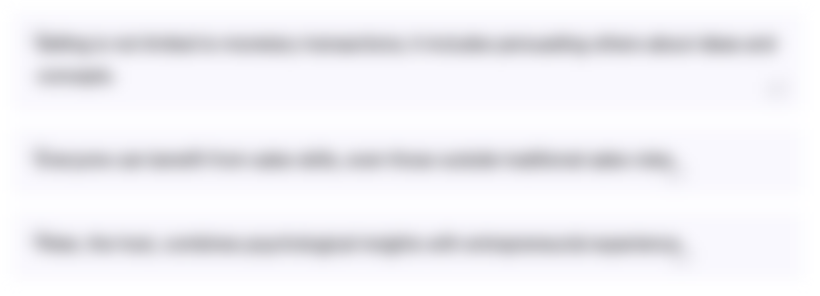
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
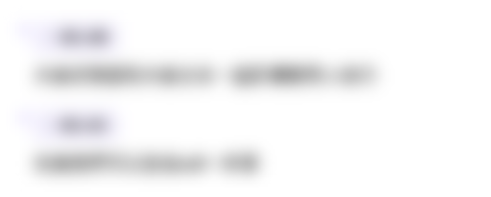
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)