Create a List of Todo items in SwiftUI | Todo List #1
Summary
TLDR在这段视频中,Nick介绍了如何使用MVVM架构来构建一个待办事项列表应用程序。首先,他解释了MVVM代表的含义,即模型(Model)、视图(View)和视图模型(ViewModel),并强调了视图(View)是用户界面逻辑的核心部分。接着,Nick展示了如何在Swift UI中构建两个主要的视图:一个是显示待办事项列表的视图,另一个是允许用户输入并添加新事项的视图。他通过创建一个新的Xcode项目,详细介绍了如何设置项目并开始编码。在构建过程中,Nick创建了一个名为“listView”的新视图,并在其中添加了一个列表,用于展示待办事项。此外,他还创建了一个“listRowView”组件,用于显示每个待办事项的复选框和标题。最后,Nick展示了如何添加导航栏按钮,包括一个编辑按钮和一个用于添加新事项的按钮,并简要介绍了如何构建一个简单的添加视图。视频以一个完整的待办事项列表和添加新事项的界面作为结束,为接下来的视频内容——深入探讨模型和待办事项数据——奠定了基础。
Takeaways
- 📝 视频中介绍了使用MVVM架构构建待办事项应用程序,MVVM代表模型(Model)、视图(View)、视图模型(ViewModel)。
- 🔍 第一个'V'代表视图(Views),视频中将构建应用程序中的两个主要视图:一个用于显示待办事项列表,另一个用于输入和添加新事项。
- 🚀 通过SwiftUI组件直接在屏幕上添加内容,使得这个视频成为课程中相对简单的一个部分。
- 💻 推荐使用Xcode 12.3或更高版本进行开发,但不是必须使用最新版本。
- 📱 创建一个新的Xcode项目,选择常规iOS应用,专门用于iPhone和iPad。
- 📍 在SwiftUI中,使用NavigationView将整个应用包裹起来,以便于导航。
- 📋 构建了一个ListView,它是一个列表,用于显示待办事项,并且可以添加导航标题和图标。
- 🔑 通过创建一个名为ListRowView的自定义组件来显示每个待办事项,包括复选框和事项标题。
- 🔄 为了代码的清晰和分离,将ListRowView组件分离到单独的文件中。
- 🗂 使用状态变量(State)来管理待办事项列表中的数据,而不是直接在视图中硬编码。
- ➕ 添加了一个Add View,用于输入新的待办事项,包括一个文本框和一个保存按钮。
- ✅ 通过使用NavigationViewLink实现了从ListView到Add View的导航,以及返回按钮的自动生成。
Q & A
什么是 MVVM 架构?
-MVVM 架构包含三个部分:Model、View 和 ViewModel。Model 是数据点,View 是应用程序的 UI 组件,ViewModel 则负责管理 View 所需的数据,将 Model 数据绑定到 View 上。
在 MVVM 架构中,ViewModel 的作用是什么?
-ViewModel 的作用是管理数据,为 View 提供数据,并处理与用户界面交互相关的逻辑。它负责将 Model 数据转化为适合在 View 上显示的格式,并与 View 进行双向数据绑定。
在视频中,为什么要将整个应用程序都包裹在 NavigationView 中?
-将整个应用程序包裹在 NavigationView 中是为了确保应用的每个视图都处于导航视图的环境中,这样可以轻松实现视图之间的导航和页面跳转。
为什么在 List 视图中使用了 Spacer?
-在 List 视图中使用 Spacer 是为了将列表项内容推到屏幕的左侧,使其左对齐。这样可以使列表项看起来更整齐、更规范。
为什么在 List 视图中创建了一个名为 listRowView 的组件?
-在 List 视图中创建 listRowView 组件是为了使列表项的样式和结构可重用,这样可以使代码更清晰、更模块化,也方便后续的维护和扩展。
为什么在 AddView 中使用了 ScrollView?
-在 AddView 中使用 ScrollView 是为了确保当用户输入内容超出屏幕可视范围时,能够滚动查看所有内容。这样可以保证用户在输入较长内容时能够方便地进行操作。
为什么在 AddView 的按钮上使用了 NavigationLink?
-在 AddView 的按钮上使用 NavigationLink 是为了实现按钮点击后的页面跳转功能,将用户导航回列表视图,从而实现添加新项目后的导航流程。
为什么在 List 视图中使用了 @State 属性包裹 items 数组?
-在 List 视图中使用 @State 属性包裹 items 数组是为了在视图中引入数据,并使其能够随着用户交互而动态更新。这样可以确保视图与数据的同步性。
在 List 视图中,为什么要使用 @State 属性包裹 textFieldText 字符串?
-在 List 视图中使用 @State 属性包裹 textFieldText 字符串是为了创建一个与文本字段绑定的可变状态,以便在用户输入时更新文本字段的内容。这样可以确保文本字段的动态更新。
为什么在 AddView 的按钮上使用了 .frame(maxWidth: .infinity)?
-在 AddView 的按钮上使用 .frame(maxWidth: .infinity) 是为了让按钮的宽度自适应父视图的宽度,使其填充整个可用空间。这样可以确保按钮在不同屏幕尺寸下的一致性表现。
Outlines
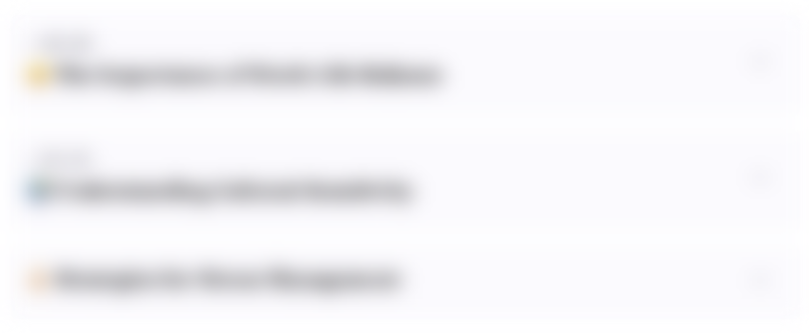
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
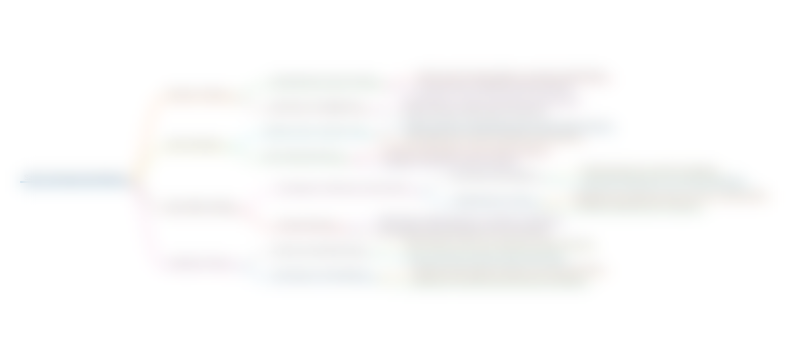
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
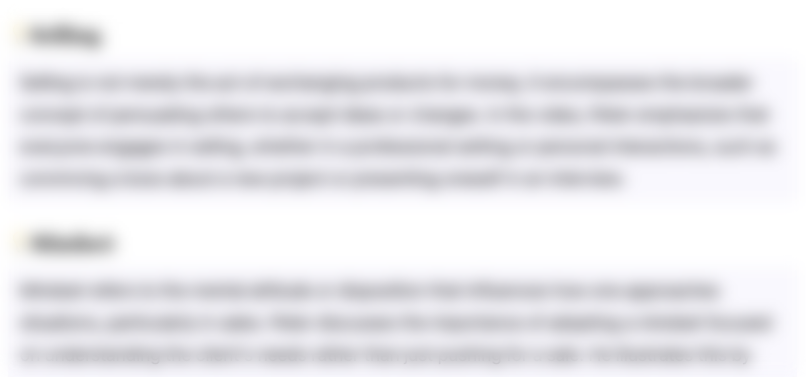
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
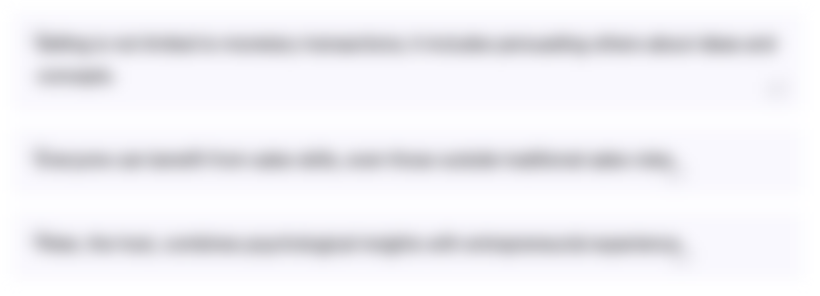
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
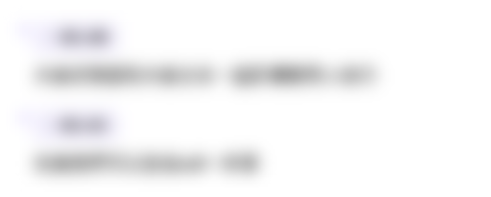
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
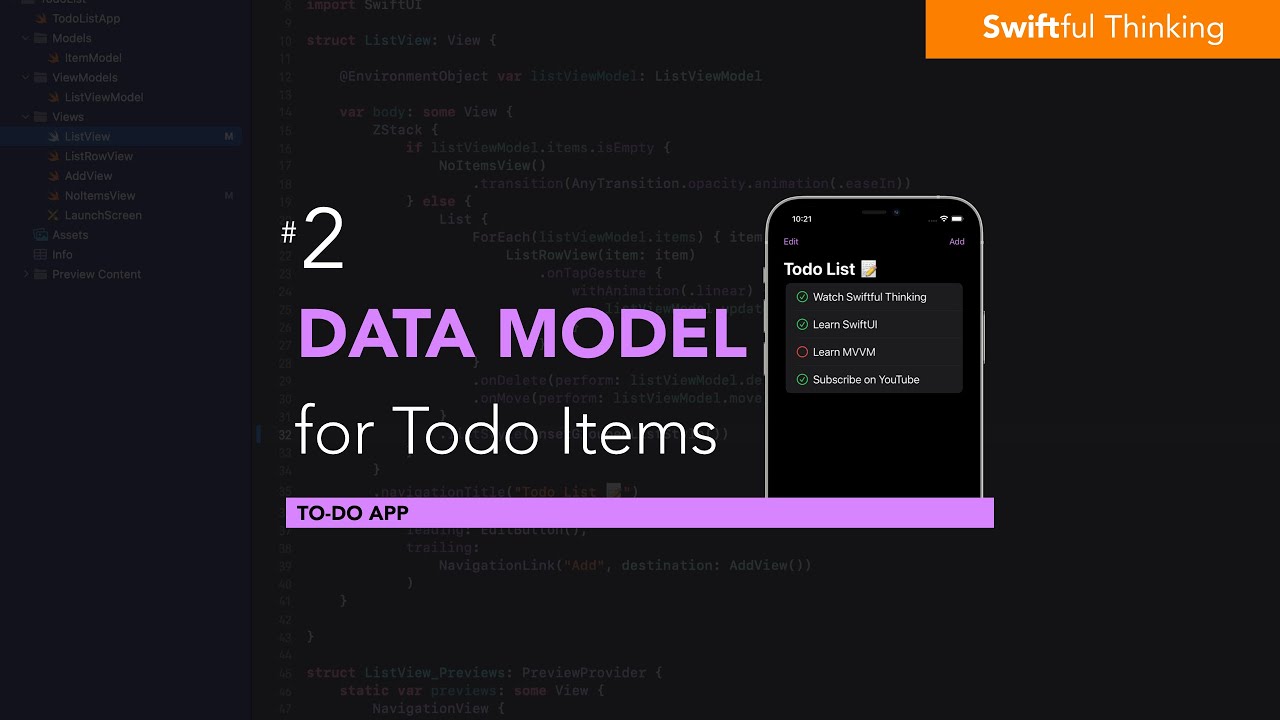
Create a custom data model for Todo items in SwiftUI | Todo List #2
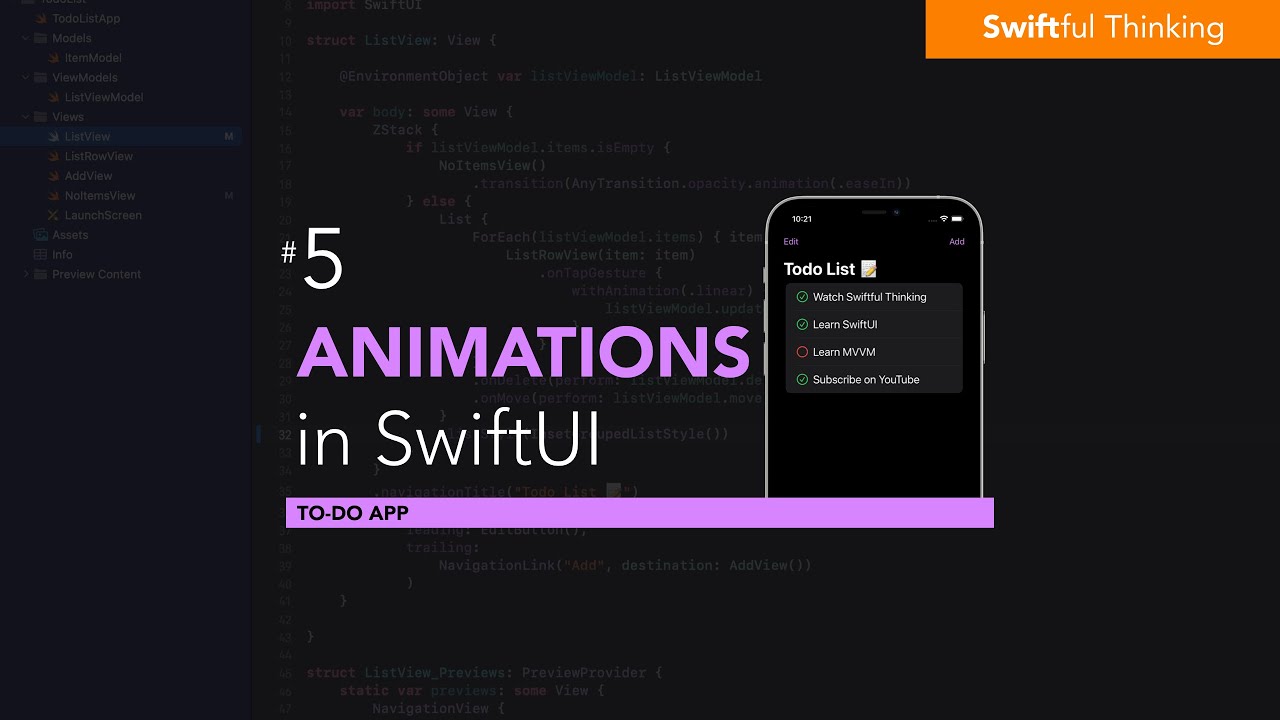
User Experience and Animations in SwiftUI app | Todo List #5
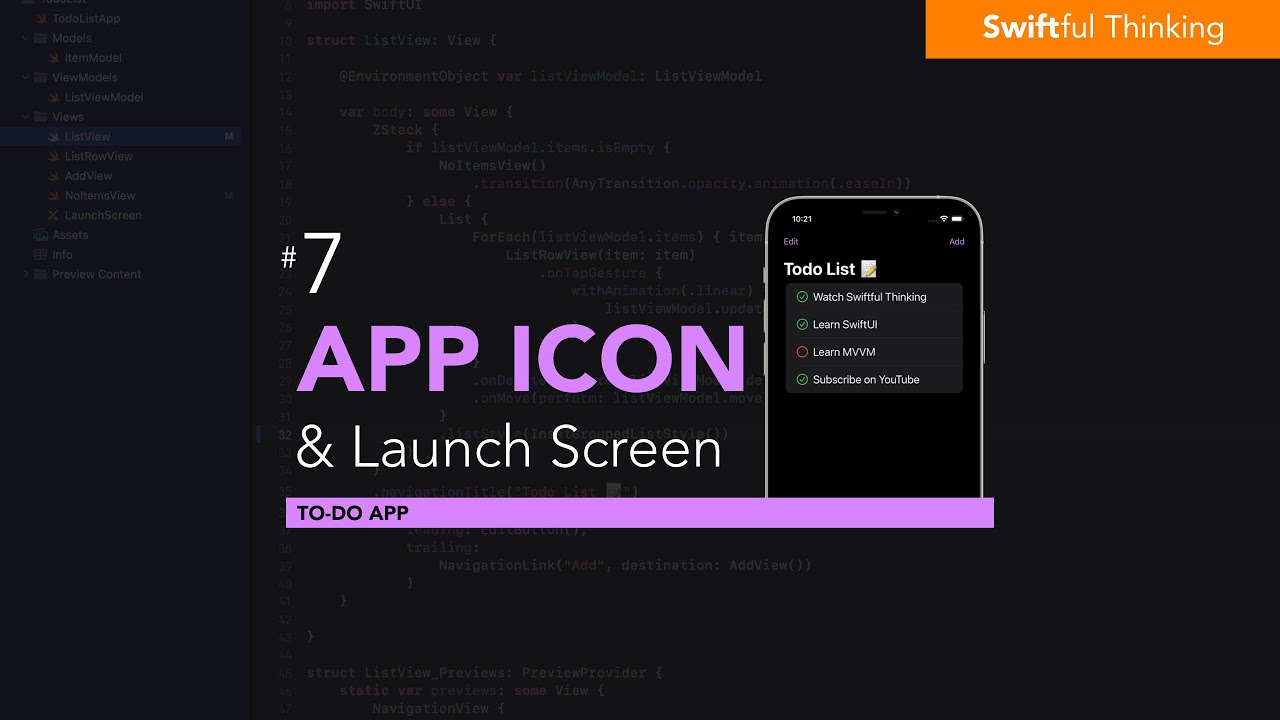
Adding an App Icon and Launch Screen to SwiftUI | Todo List #7
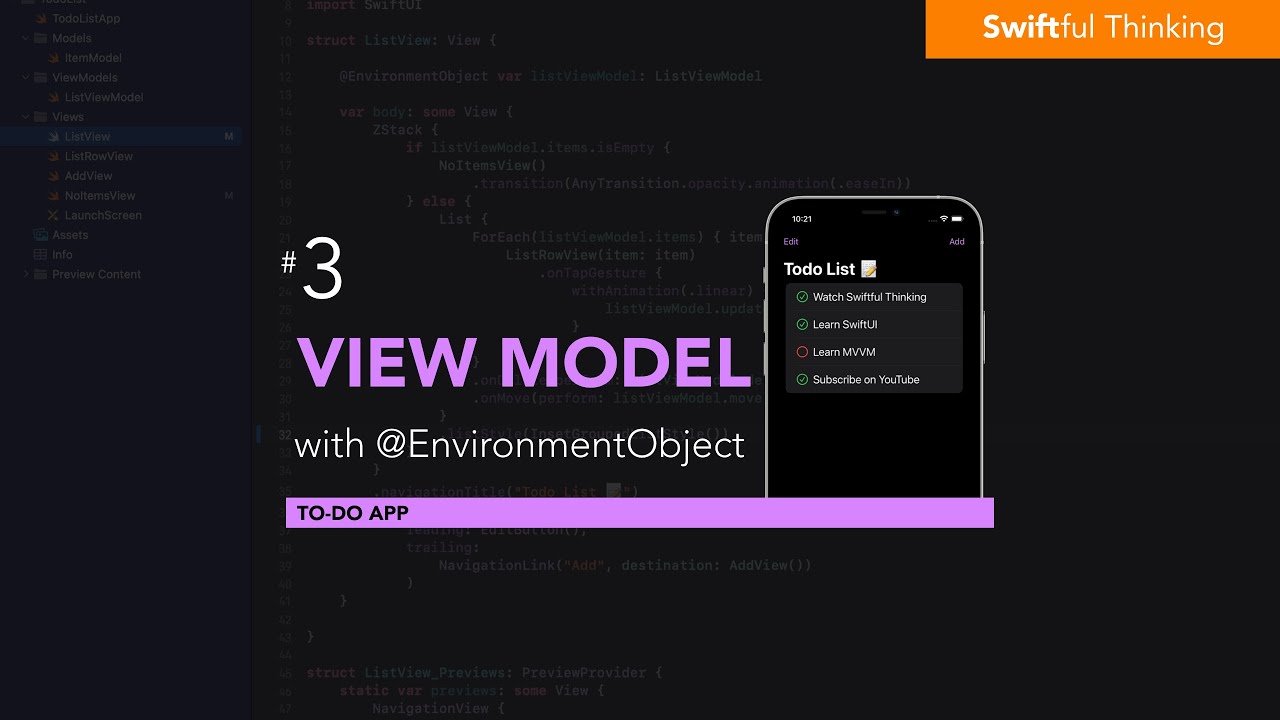
Add a ViewModel with @EnvironmentObject in SwiftUI | Todo List #3
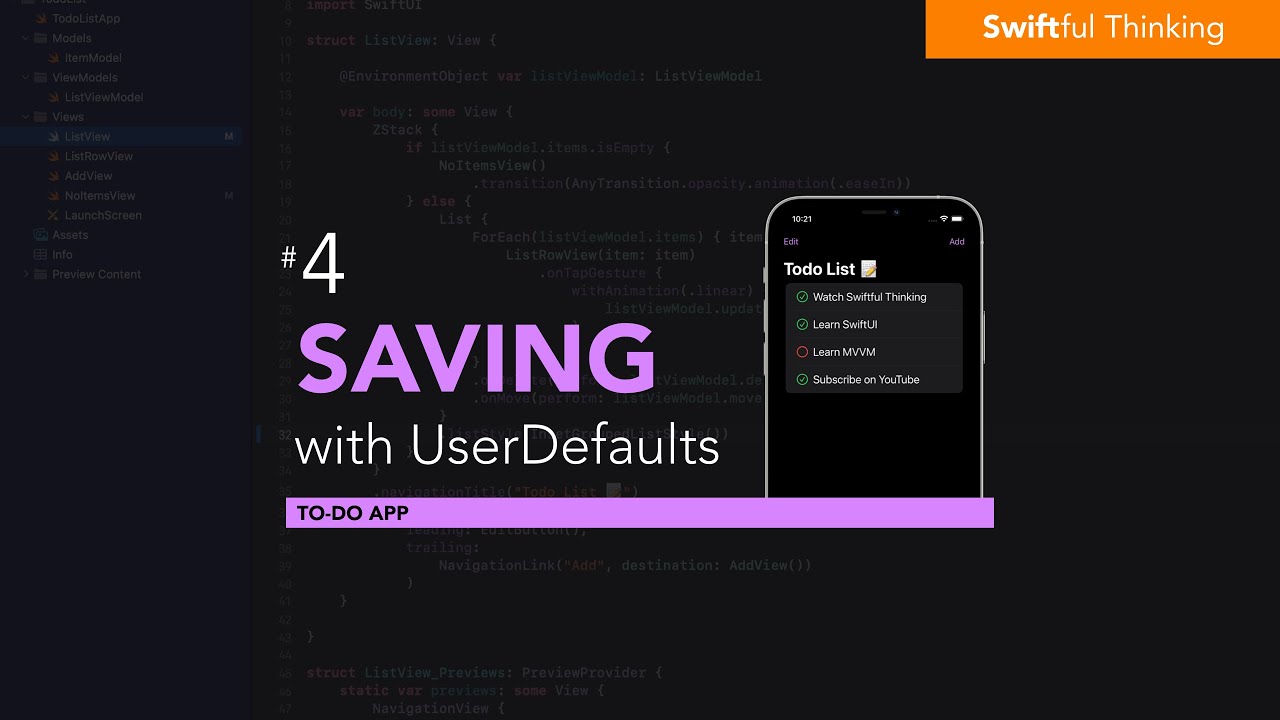
Save and persist data with UserDefaults | Todo List #4
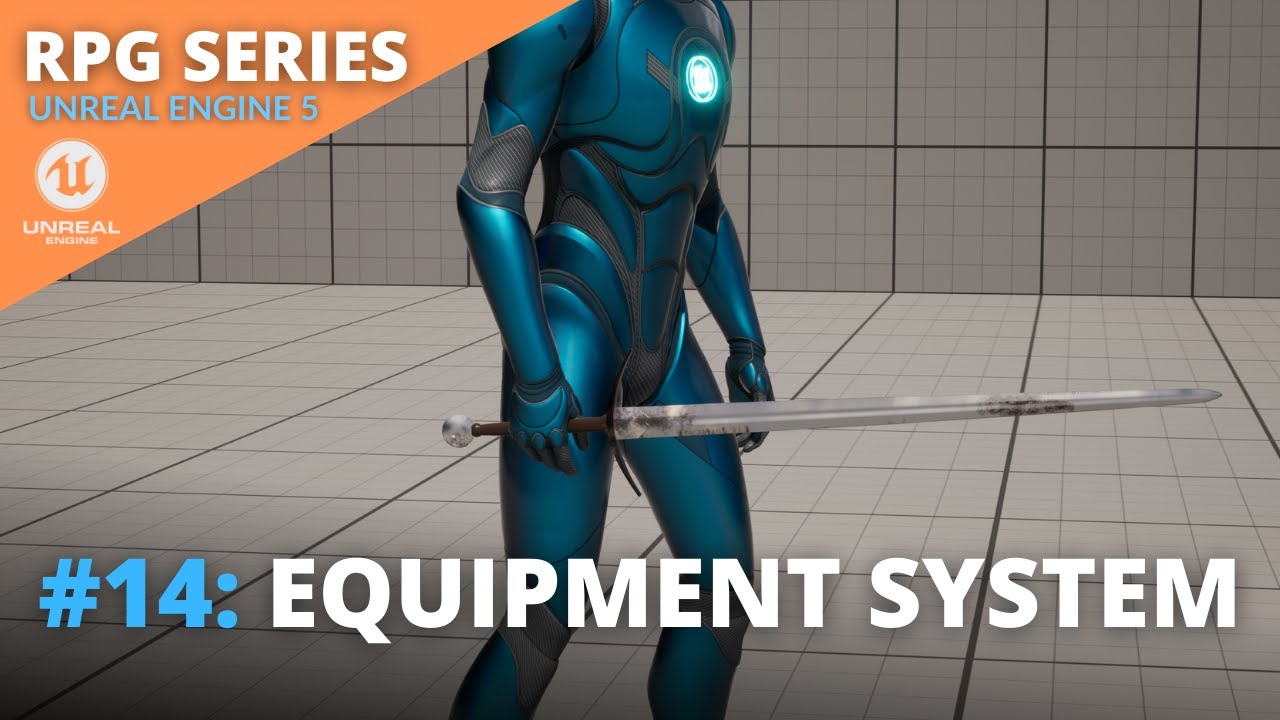
Unreal Engine 5 RPG Tutorial Series - #14: Equipment System
5.0 / 5 (0 votes)