Create a custom data model for Todo items in SwiftUI | Todo List #2
Summary
TLDR在这段视频脚本中,Nick介绍了在MVVM架构中创建和实现模型(Model)的过程。他们以待办事项列表应用为例,展示了如何构建一个自定义数据类型来表示待办事项。这个模型不仅包含事项的标题,还包含一个唯一标识符(ID)和一个布尔值(isCompleted),用以标记事项的完成状态。Nick还解释了如何让模型符合SwiftUI中的Identifiable协议,以便于在ForEach循环中使用,并演示了如何更新ListView和ListRowView来显示带有完成状态的待办事项。视频最后,Nick预告了下一集将介绍ViewModel,用于添加、删除和更新待办事项的逻辑。
Takeaways
- 📝 **模型定义**:在MVVM架构中,'Model'代表数据模型,用于定义应用程序的数据结构和状态。
- 📚 **自定义数据类型**:创建自定义数据类型(Model)来表示待办事项列表中的项目,包含标题、唯一标识符和完成状态。
- 🔍 **属性细节**:待办事项除了标题外,还应包含一个唯一ID和布尔值来标识是否完成。
- 🏗️ **简化生产应用**:虽然示例中的模型相对简单,但足以学习如何构建和有效实现模型。
- 📑 **Xcode项目**:在Xcode项目中创建一个新的模型组,并添加一个Swift文件来定义模型。
- 🔑 **唯一标识符**:为每个待办事项添加一个唯一ID,使用UUID生成随机字符串。
- 🔄 **符合协议**:使模型符合`Identifiable`协议,以便于在SwiftUI的`ForEach`循环中使用。
- 🎨 **动态UI**:根据待办事项的完成状态动态显示不同的UI元素,如红色圆圈表示未完成,绿色勾选表示已完成。
- 📝 **初始化器**:创建一个初始化器,要求提供标题和完成状态,但最终移除了ID的初始化,因为每个项已经有ID。
- 📱 **预览自定义**:在SwiftUI的预览中使用静态变量来展示不同的待办事项状态。
- 🌟 **字体与颜色**:调整字体大小和颜色,使已完成的事项显示绿色,未完成的显示红色。
- 🔧 **ListView更新**:更新ListView,使用ListRowView展示每个待办事项,并根据其完成状态显示不同的图标和颜色。
Q & A
在Swift UI中,如何改变ListView中项的字体大小?
-可以通过在HStack中使用.font(.title2)来改变ListView中项的字体大小。
在接下来的视频中,将会介绍MVVM架构中的哪个部分?
-在接下来的视频中,将会介绍MVVM架构中的ViewModel部分,这涉及到添加、删除和更新待办事项的逻辑。
Outlines
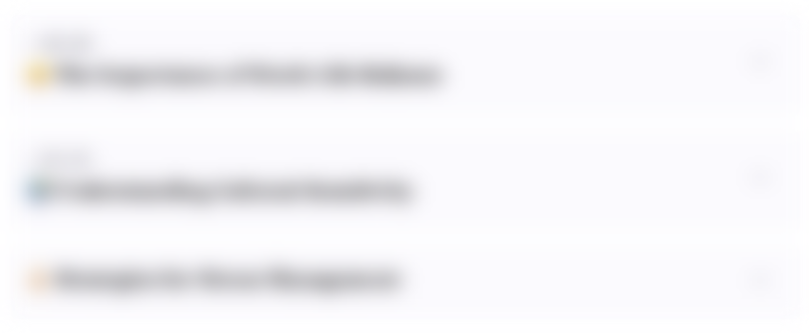
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
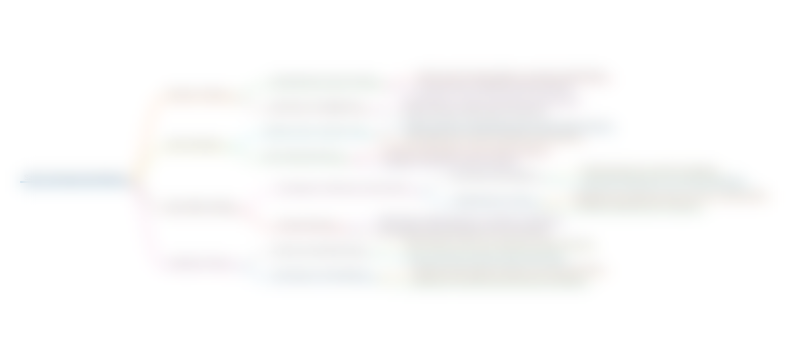
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
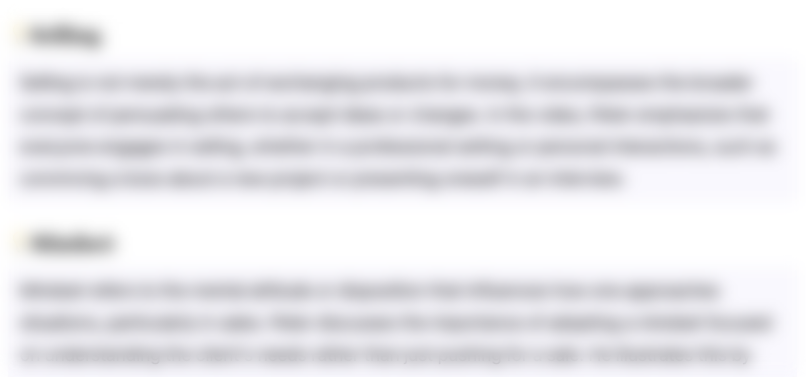
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
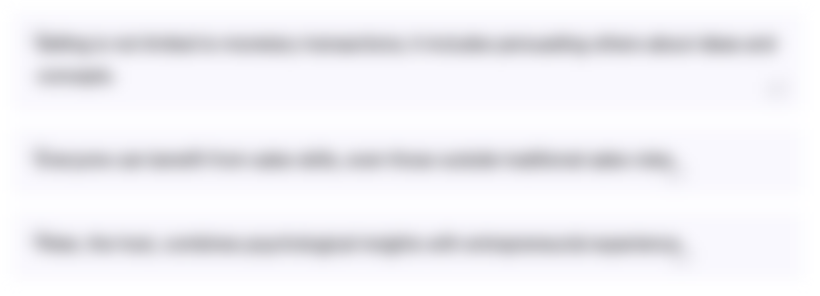
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
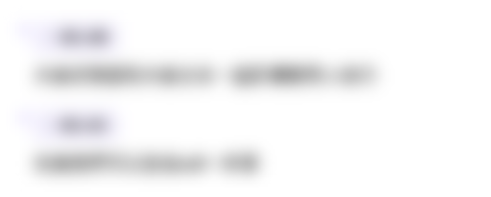
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
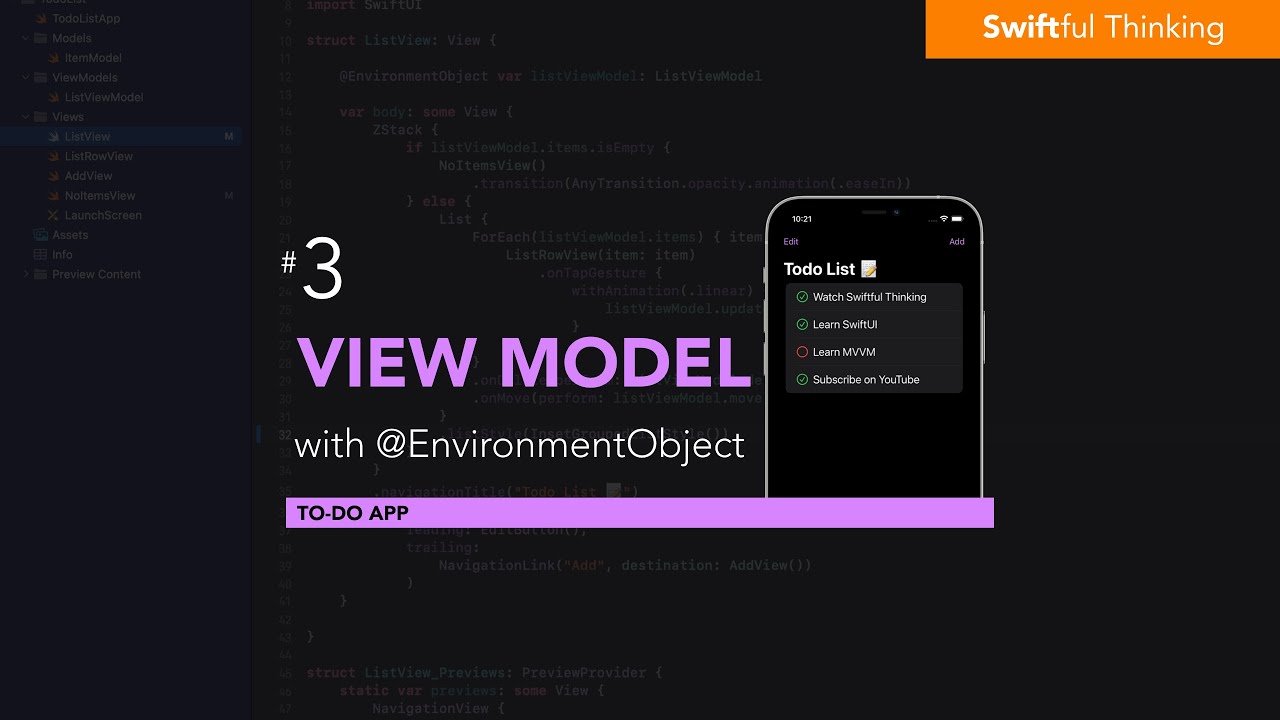
Add a ViewModel with @EnvironmentObject in SwiftUI | Todo List #3
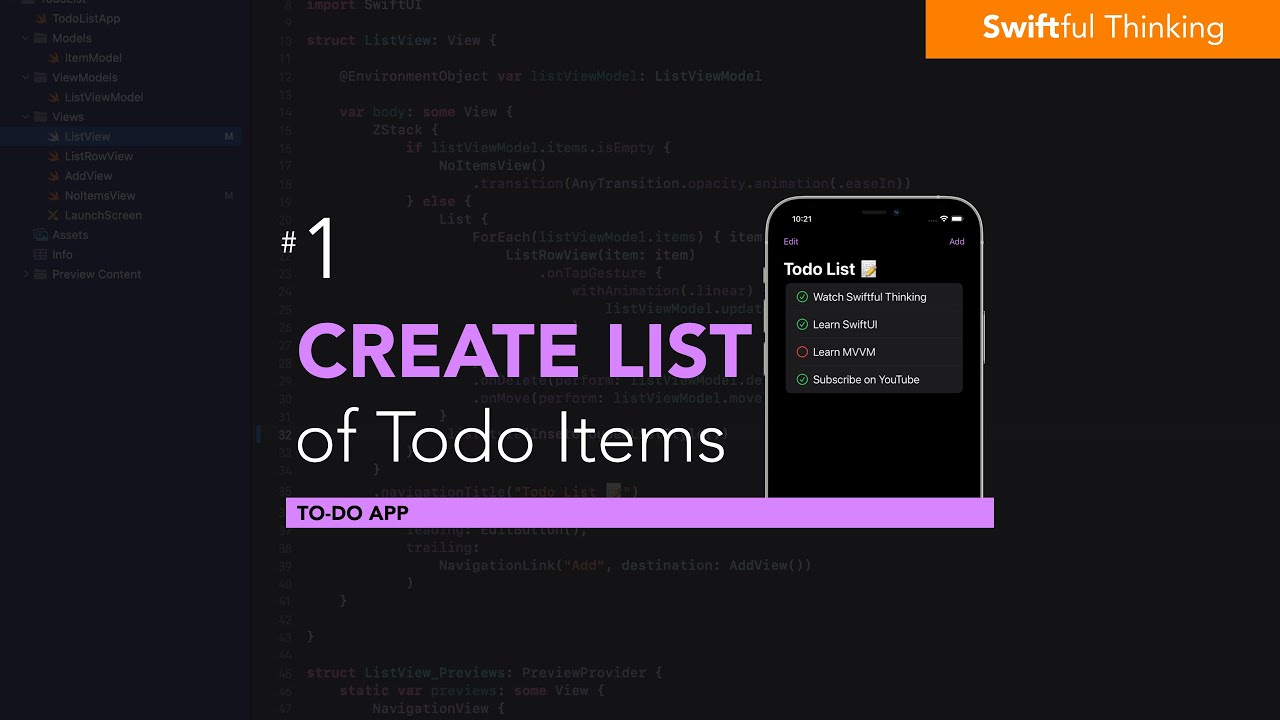
Create a List of Todo items in SwiftUI | Todo List #1
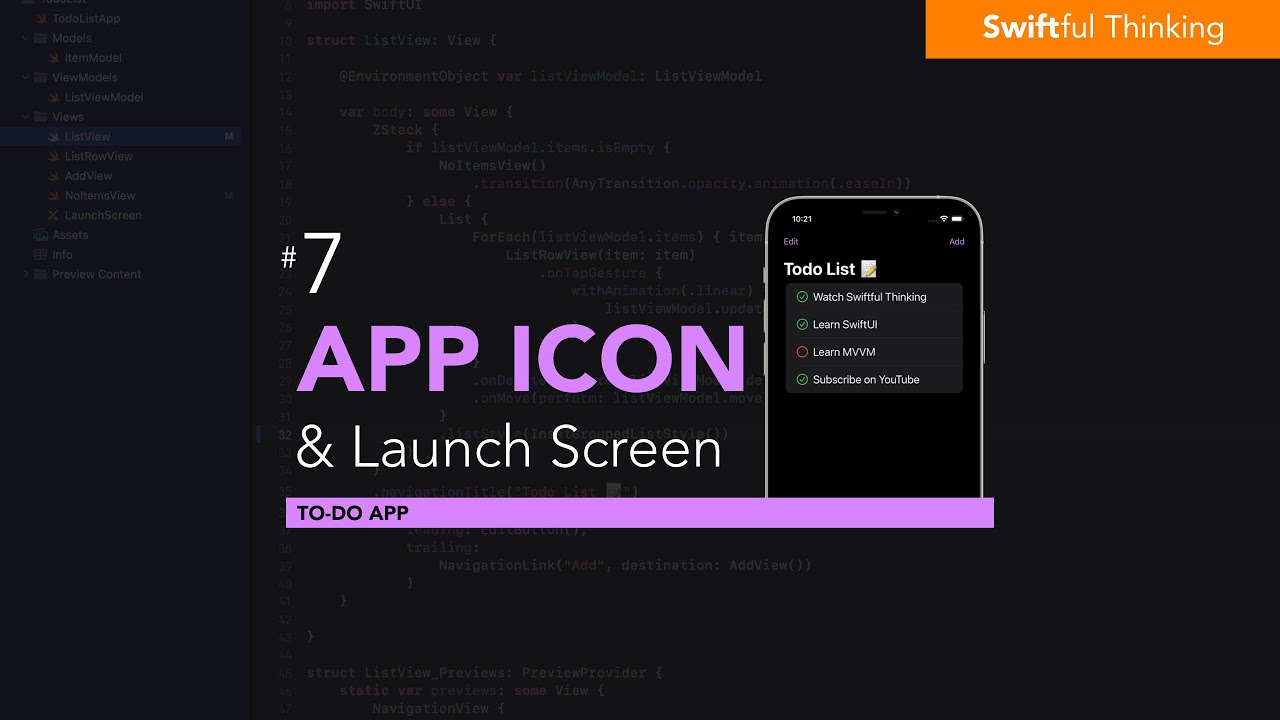
Adding an App Icon and Launch Screen to SwiftUI | Todo List #7
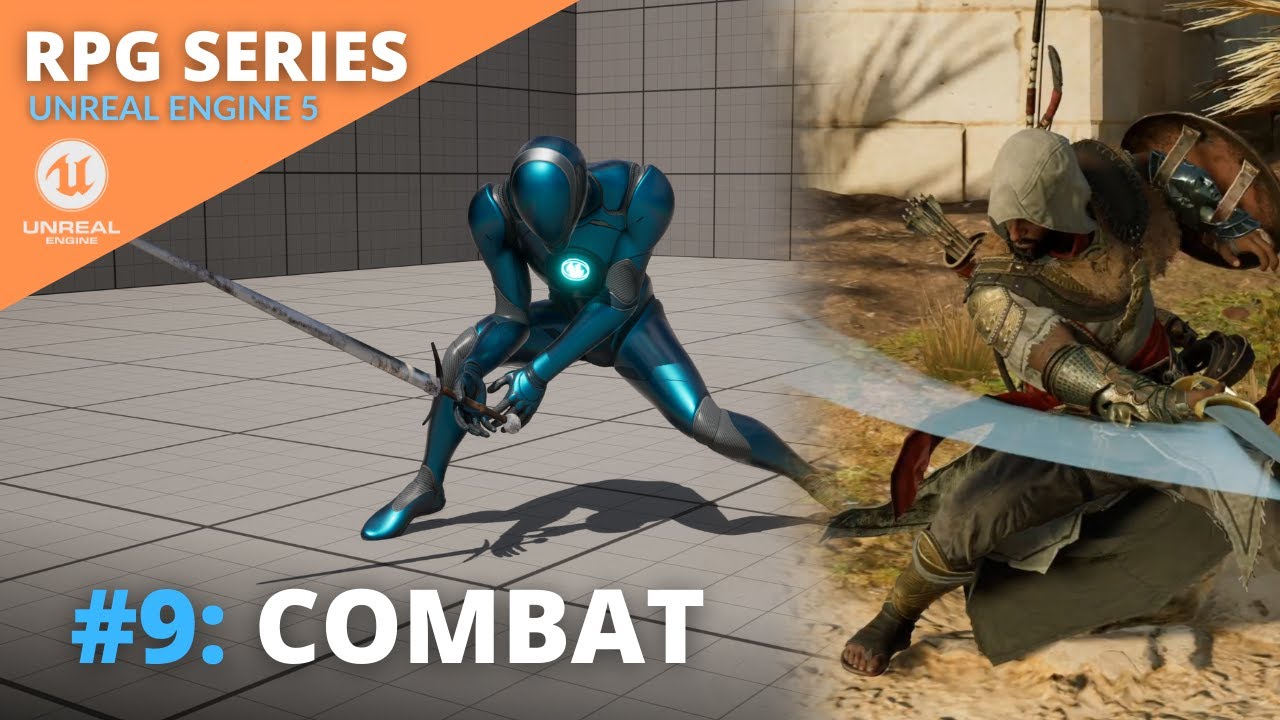
Unreal Engine 5 RPG Tutorial Series - #9: Combat
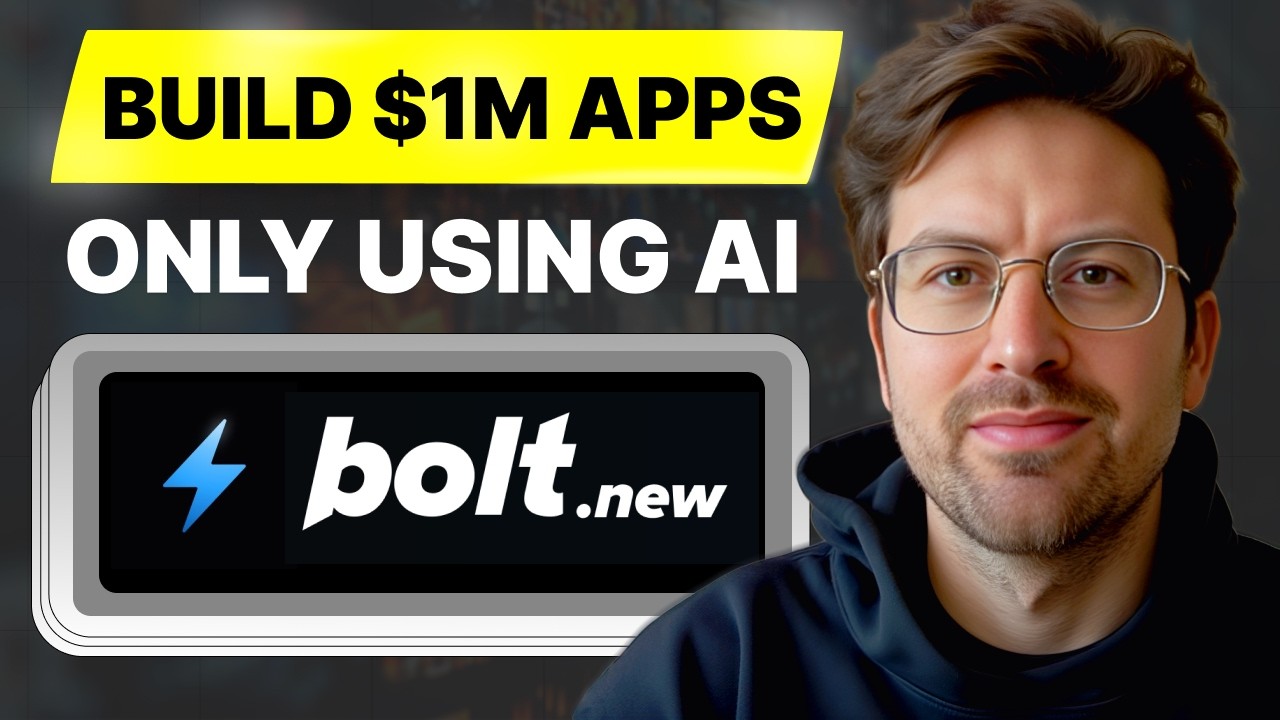
Bolt tutorial for beginners with the Bolt CEO Eric Simons
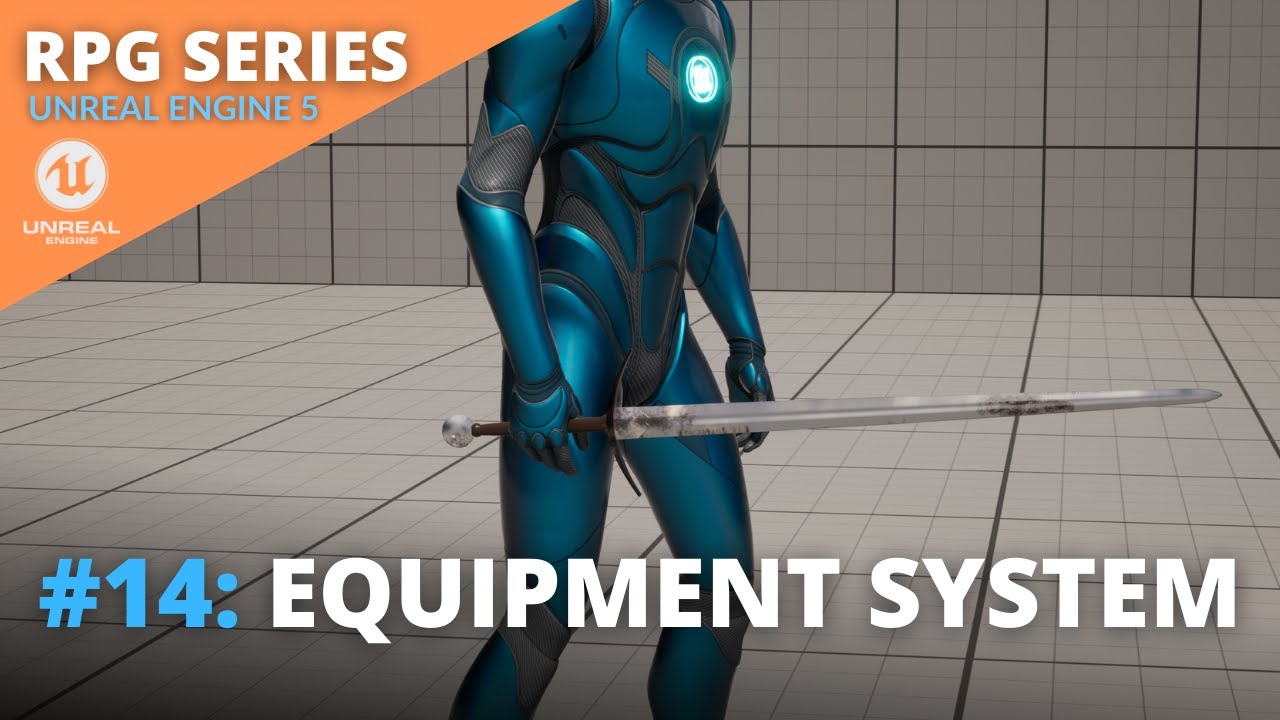
Unreal Engine 5 RPG Tutorial Series - #14: Equipment System
5.0 / 5 (0 votes)