Save and persist data with UserDefaults | Todo List #4
Summary
TLDR在这个视频中,Nick 介绍了如何在他们正在开发的应用程序中实现数据持久化。他们指出,尽管应用程序可以正常工作,但之前并未保存任何数据,导致关闭并重新打开应用程序后,所有新增的待办事项都会丢失。为了解决这个问题,Nick 决定使用 UserDefaults 来保存待办事项列表。他解释说,UserDefaults 适用于存储较小的数据片段,例如用户 ID 或当前用户的名称。在本例中,由于待办事项模型只包含标题和布尔值,因此使用 UserDefaults 是合适的。Nick 展示了如何将待办事项模型转换为 JSON 数据,然后存储到 UserDefaults 中,并在需要时再将其转换回模型。他还展示了如何通过在待办事项数组上使用计算属性和 didSet 来确保每次更改数组时都会保存到 UserDefaults。最后,他演示了如何从 UserDefaults 中检索数据,并更新应用程序中的待办事项列表。这个视频不仅提供了关于如何使用 UserDefaults 的实用指导,还练习了使用 guard 语句和 if let 语句进行可选绑定。
Takeaways
- 📝 课程中构建的应用程序可以正常工作,但之前没有实现数据保存功能,关闭再打开应用后,新添加的待办事项会丢失。
- 🔄 为了解决数据保存问题,视频介绍了如何使用用户默认设置(UserDefaults)来保存待办事项列表,使其在会话之间持久化。
- 📚 用户默认设置(UserDefaults)主要用于存储较小的数据片段,例如用户ID或用户名,而不适用于大型数据库。
- 🛠️ 通过将待办事项列表(Item Models)转换为JSON数据,可以存储在UserDefaults中,之后可以再次转换回待办事项列表。
- 🔑 为了能够将待办事项列表编码为数据,Item Model需要符合Codable协议,这样可以实现数据的编码和解码。
- 🔩 在ListView Model中创建了一个新的函数`saveItems`,用于将待办事项列表编码并存储到UserDefaults中。
- 🔄 通过计算属性和`didSet`属性观察器,确保每次待办事项列表(items array)发生变化时,都会调用`saveItems`函数进行保存。
- 🔑 引入了一个常量`itemsKey`作为UserDefaults中的键,避免直接在代码中硬编码键名,提高代码的可维护性。
- 📈 为了从UserDefaults中恢复待办事项列表,需要使用JSON Decoder将存储的数据解码回Item Models数组。
- 🛡️ 使用`guard`语句来安全地解包可选类型,确保数据存在且可以成功转换为待办事项列表。
- 🔄 通过在应用启动时从UserDefaults获取数据,并更新待办事项列表,实现了数据的持久化。
- 🎉 关闭应用后再打开,可以看到之前添加和修改的待办事项都被保存了下来,演示了数据持久化的成功实现。
Q & A
为什么在应用程序中添加了新的任务后,关闭再打开应用,任务会消失?
-这是因为应用尚未实现数据持久化功能,关闭应用后,之前添加的任务没有被保存,所以重新打开应用时会恢复到初始状态。
为了使应用中的数据在关闭后重新打开依然存在,应该使用什么技术?
-在视频中,Nick提到如果是为了上架App Store的应用,可能会使用Core Data。但在这个例子中,为了简单,选择使用了UserDefaults。
为什么在Swift UI Bootcamp课程中没有覆盖Core Data的内容?
-Nick没有在Swift UI Bootcamp中覆盖Core Data的内容,可能是因为课程的重点在于其他方面,或者是时间限制,或者是为了让课程更加集中和易于消化。
UserDefaults适合存储哪些类型的数据?
-UserDefaults主要适合存储较小的数据,例如用户ID或当前用户的姓名。对于较大的数据集,应该使用其他存储解决方案,如Core Data。
为什么在ListView Model中使用UserDefaults而不是App Storage?
-因为App Storage更适合在视图中直接使用,而ListView Model是一个类,所以在这个上下文中使用UserDefaults更为合适。
如何将Item Model转换为可以存储在UserDefaults中的数据?
-通过将Item Model转换为JSON数据。首先,需要让Item Model符合Codable协议,然后使用JSON编码器将模型数组编码为数据。
为什么需要将Item Model符合Codable协议?
-Codable协议允许数据模型被编码和解码,这意味着可以将模型转换为数据(如JSON格式),并从数据重建模型。
在ListView Model中,如何确保每次更新items数组时都保存数据?
-通过在items属性上使用计算属性,并在didSet属性观察器中调用saveItems函数来实现。
如何从UserDefaults中检索并恢复保存的任务列表?
-首先从UserDefaults中获取保存的数据,然后使用JSON解码器将数据解码回Item Model的数组,并用这个数组更新items属性。
为什么在获取UserDefaults中的数据时要使用guard语句?
-使用guard语句是为了安全地处理可选值。如果从UserDefaults中获取的数据不存在,或者无法将其解码为Item Model数组,guard语句可以防止程序崩溃,并提供一个明确的退出点。
在视频中提到的“saveItems”函数的作用是什么?
-“saveItems”函数的作用是将当前的items数组(包含待办事项)编码为JSON数据,并将其存储到UserDefaults中,以实现数据的持久化。
为什么在视频中不直接在每个修改items数组的函数末尾添加saveItems调用?
-为了代码的效率和清晰,通过在items属性的didSet属性观察器中调用saveItems,可以确保无论何时items数组被修改,都会自动保存,而不是在每个修改函数中重复添加保存逻辑。
Outlines
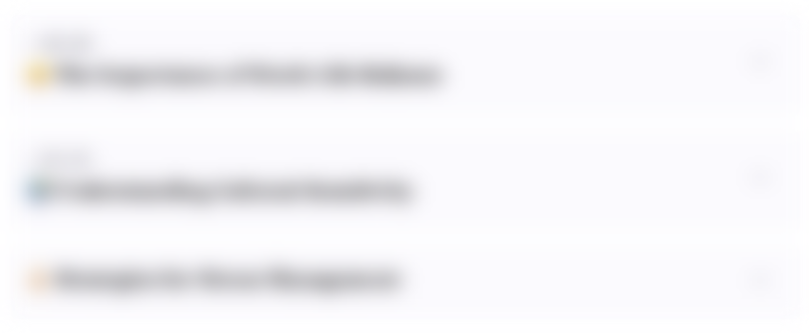
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
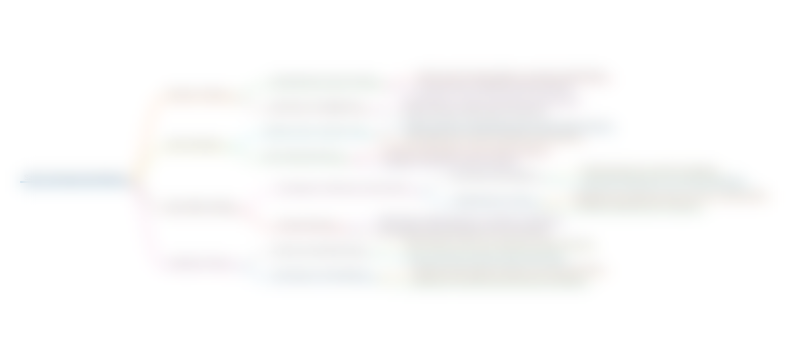
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
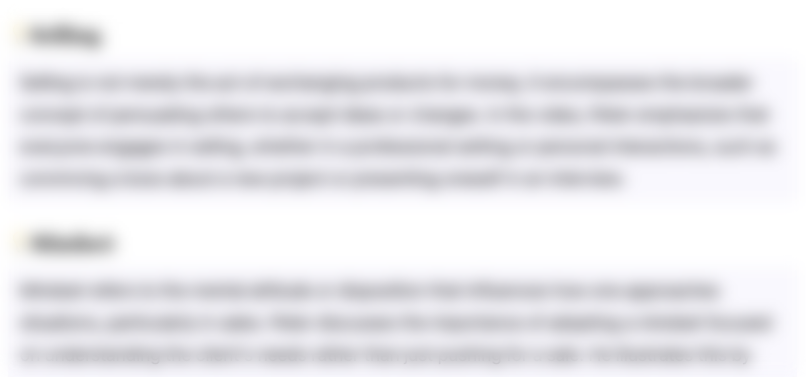
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
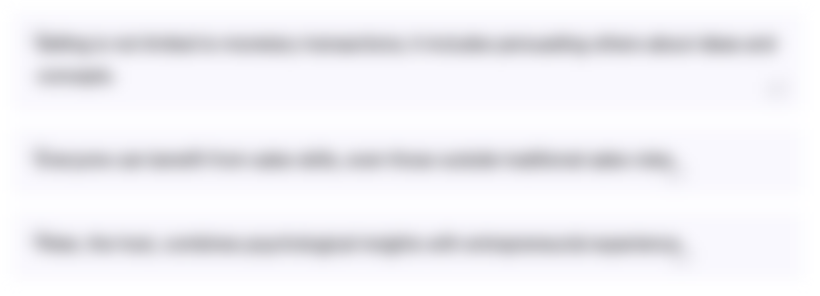
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
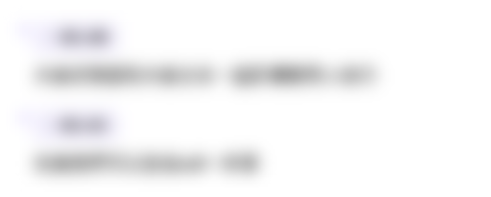
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
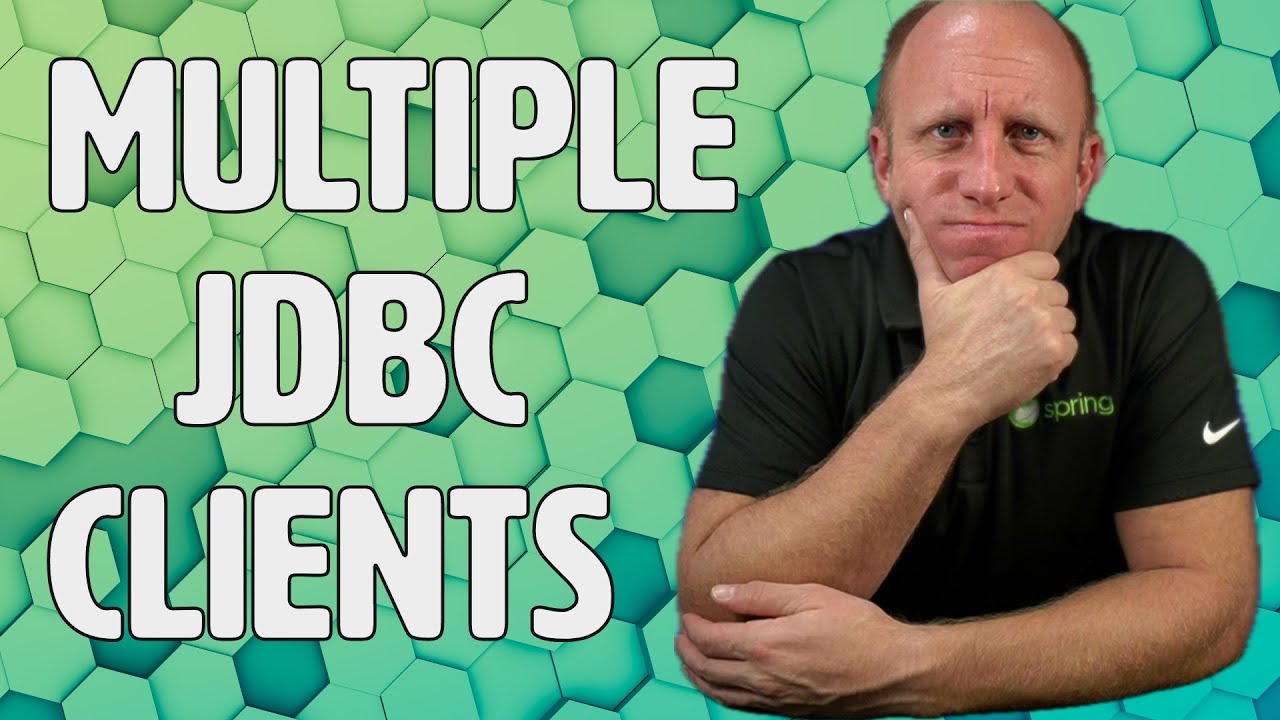
Multiple JDBC Clients - How to configure multiple DataSources in Spring
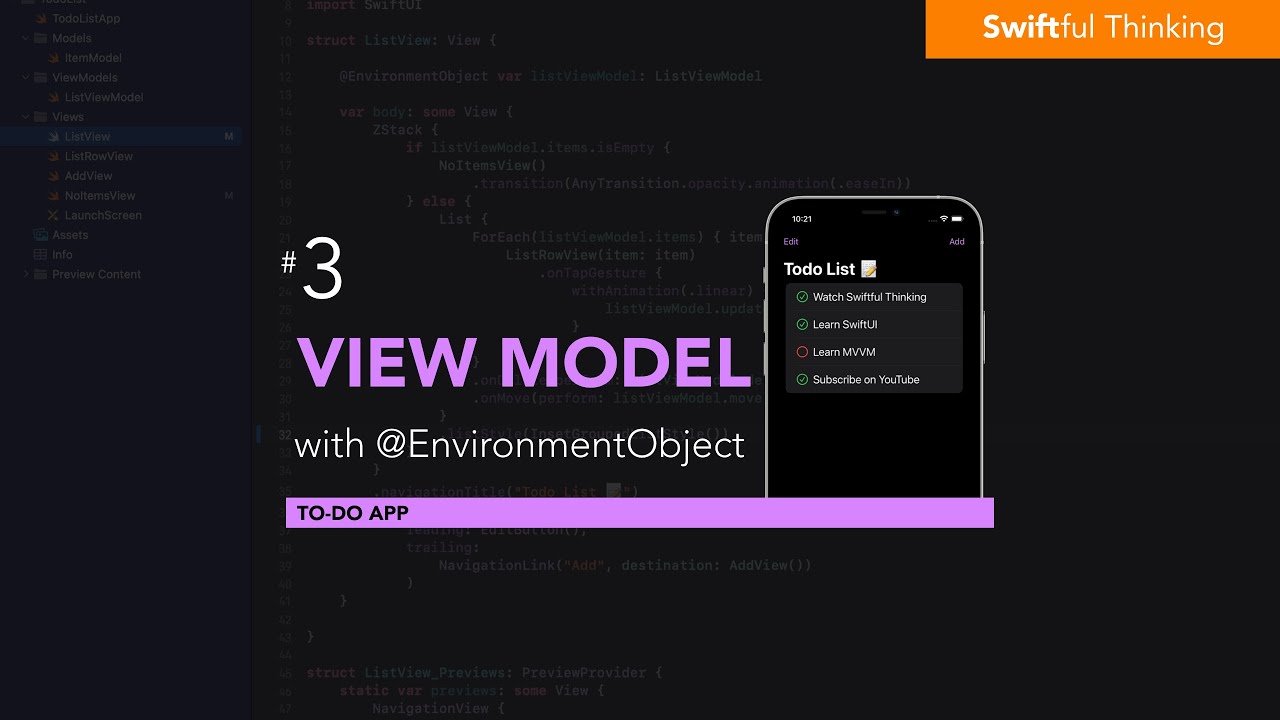
Add a ViewModel with @EnvironmentObject in SwiftUI | Todo List #3
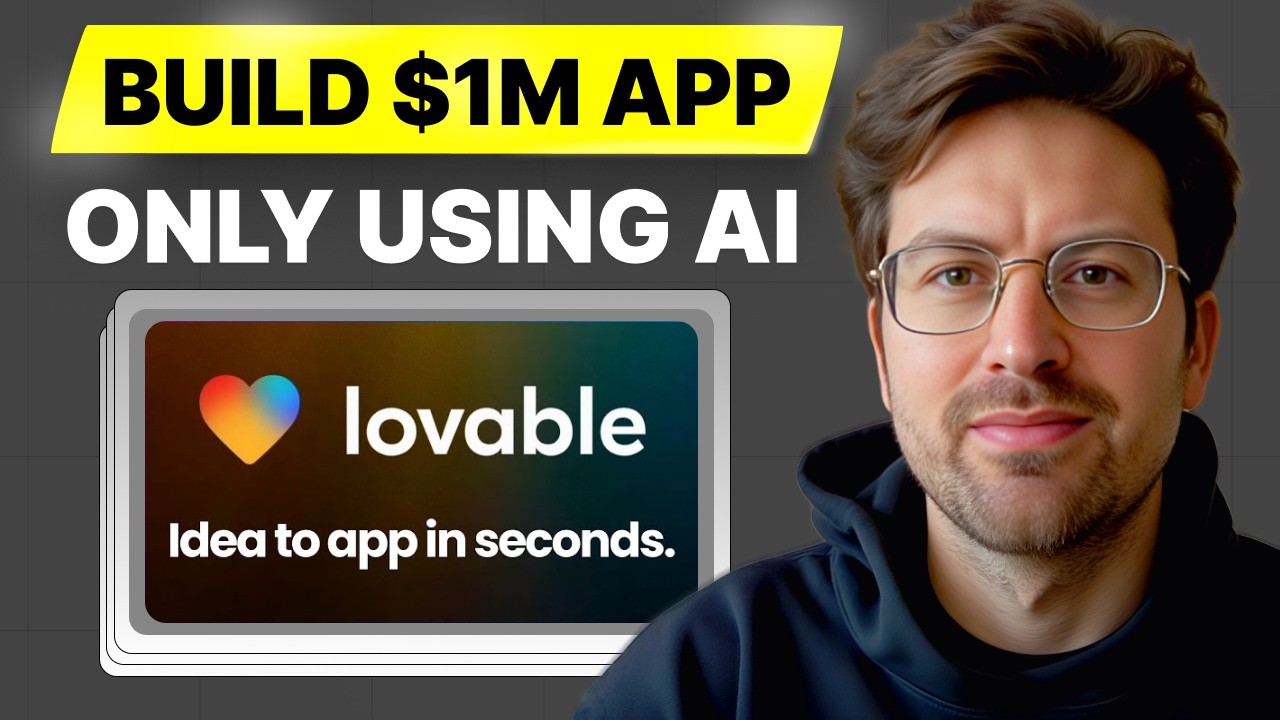
How to use AI to build your SaaS startup (Lovable, Supabase)
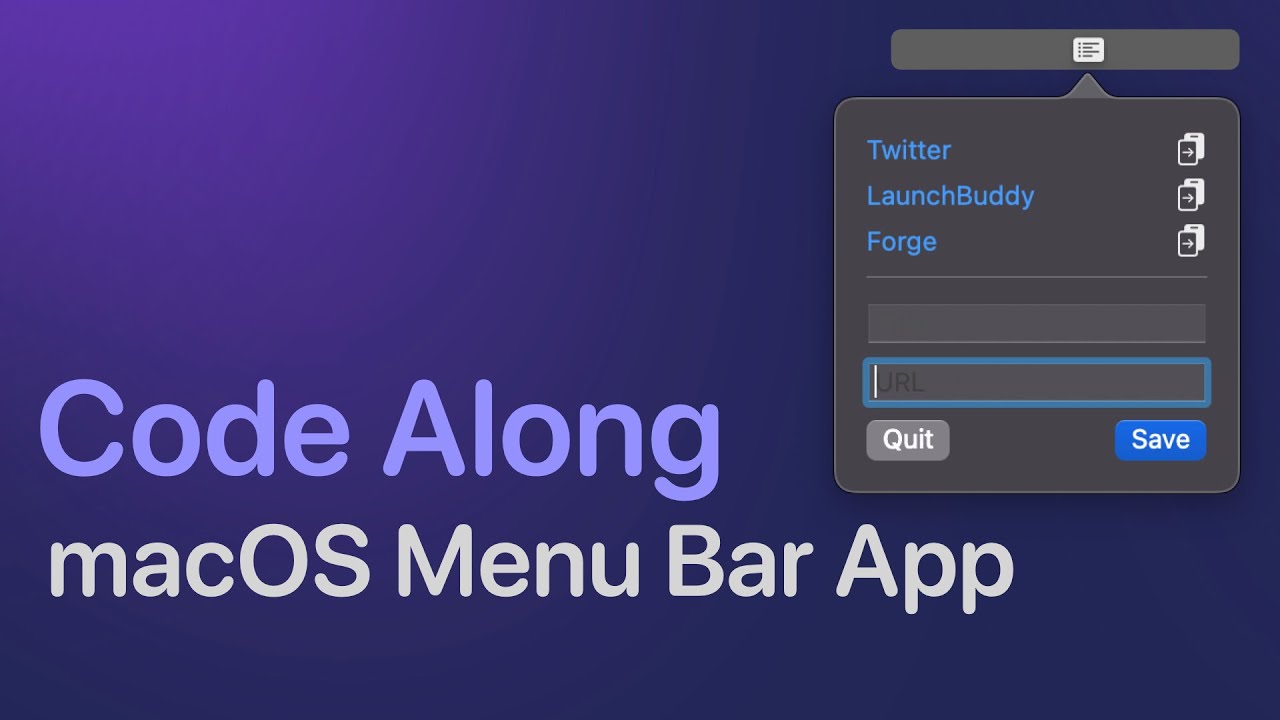
macOS Menu Bar App (Code Along) | SwiftUI, Xcode
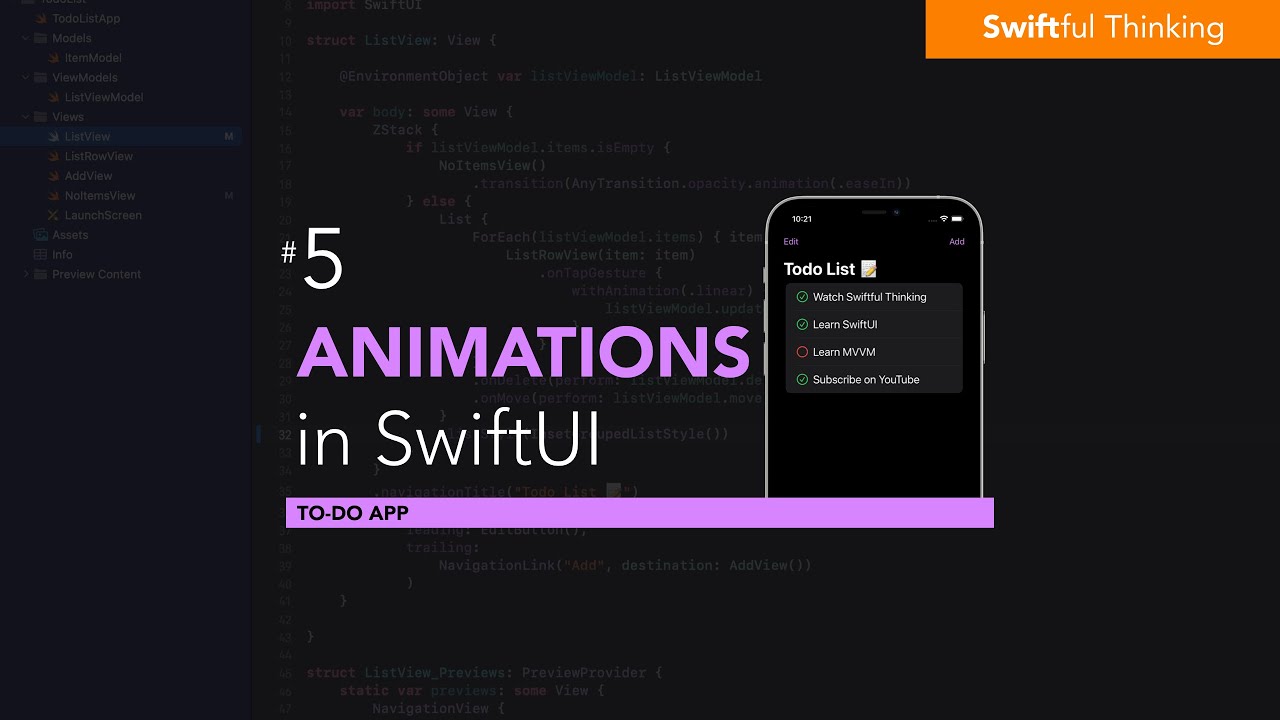
User Experience and Animations in SwiftUI app | Todo List #5
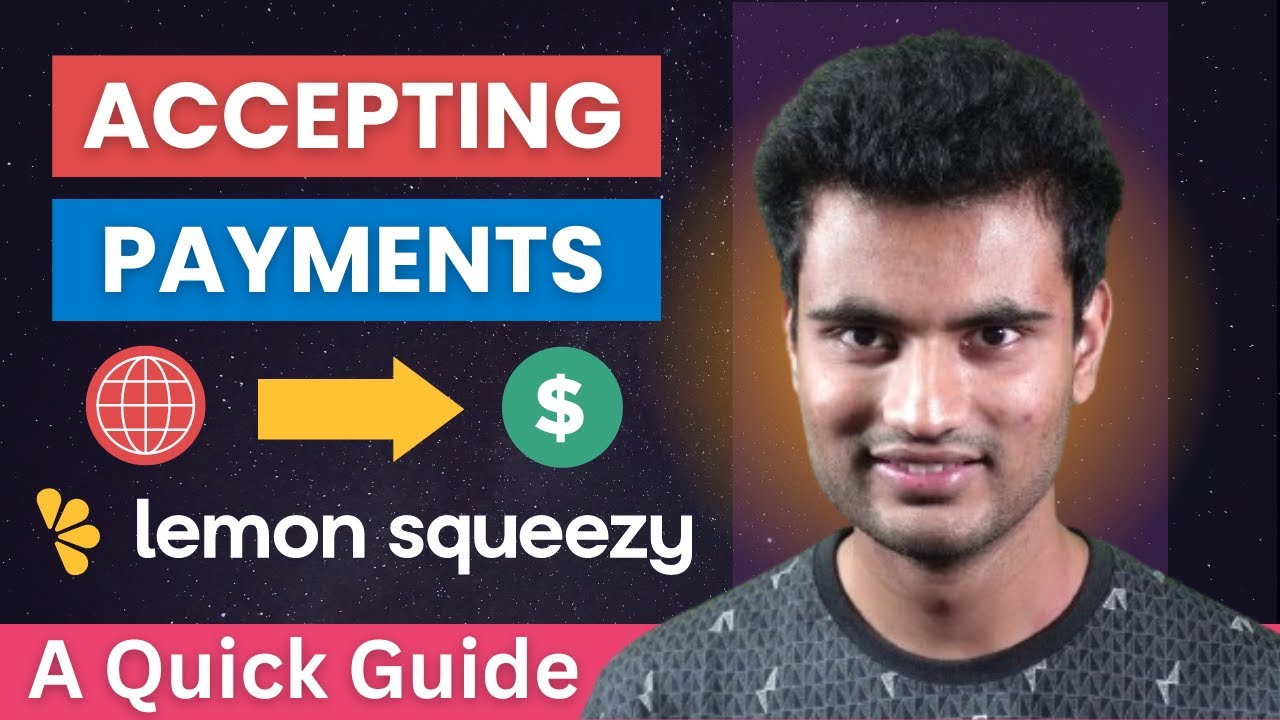
Accepting Payments using LemonSqueezy - Integrating LemonSqueezy into Next.js Tutorial
5.0 / 5 (0 votes)