Java File Input/Output - It's Way Easier Than You Think
Summary
TLDRIn this tutorial, John teaches how to read and write text files in Java. He starts by demonstrating the use of BufferedWriter for file output, explaining how to create a file and write strings to it, including handling newlines and appending text from arrays. He then covers reading files with BufferedReader, showing how to read lines until the end of the file. John emphasizes proper exception handling and closing files to prevent resource leaks. The tutorial includes writing to both relative and absolute file paths.
Takeaways
- 📝 Learn how to read and write text files in Java from scratch.
- 👨🏫 Follow along with John's tutorial for a simple approach to file I/O in Java.
- 🔗 Subscribe to John's channel for weekly Java tutorial updates.
- 💻 Use BufferedWriter for writing data to a text file in Java.
- 📚 Import BufferedWriter using 'java.io.BufferedWriter'.
- 📄 Pass a FileWriter to the BufferedWriter constructor to specify the file for writing.
- 📑 Handle IOException by surrounding file operations with a try-catch block.
- ✍️ Use 'writer.write()' to write strings to the file.
- 🔄 Close the BufferedWriter with 'writer.close()' after writing to avoid resource leaks.
- 📁 The file is created in the same directory as your program by default.
- 🔄 Overwriting files is the default behavior when writing; be cautious if data needs to be preserved.
- 📑 Use BufferedReader to read data from a text file.
- 🔍 Import BufferedReader using 'java.io.BufferedReader'.
- 🔁 Use a while loop with 'reader.readLine()' to read through each line of the file until it returns null.
- 🗑️ Close the BufferedReader with 'reader.close()' after reading to free resources.
Q & A
What is the simplest way to read and write text files in Java according to the video?
-The video suggests using `BufferedWriter` for writing and `BufferedReader` for reading text files as the simplest way.
What class is recommended for file output in Java?
-The class recommended for file output in Java is `BufferedWriter`.
How do you create a BufferedWriter object in Java?
-You create a `BufferedWriter` object by passing a `FileWriter` object to its constructor, specifying the file you want to write to.
Why is it necessary to handle `IOException` when working with files in Java?
-It is necessary to handle `IOException` because file operations can throw an `IOException`, which needs to be caught and handled properly.
What should you do after you finish writing to a file?
-After finishing writing to a file, you should call the `close()` method on the `BufferedWriter` object to ensure that the data is written to the file.
What happens if you forget to close the BufferedWriter?
-If you forget to close the `BufferedWriter`, the file will be created but it will be blank, as the contents will not be written to it.
How can you write multiple lines to a file in Java?
-You can write multiple lines to a file by calling the `write()` method multiple times, each time including a newline character (`\n`) to start a new line.
How can you write the contents of an array to a file?
-You can write the contents of an array to a file by looping through the array and writing each element to the file on a new line.
What class is used for reading files in Java?
-The class used for reading files in Java is `BufferedReader`.
How do you read a line from a file using BufferedReader?
-You read a line from a file using `BufferedReader` by calling the `readLine()` method, which returns the next line of text from the file as a `String`.
How can you read all lines from a file until the end?
-You can read all lines from a file until the end by using a `while` loop that continues to read lines with `readLine()` as long as the returned line is not `null`.
Outlines
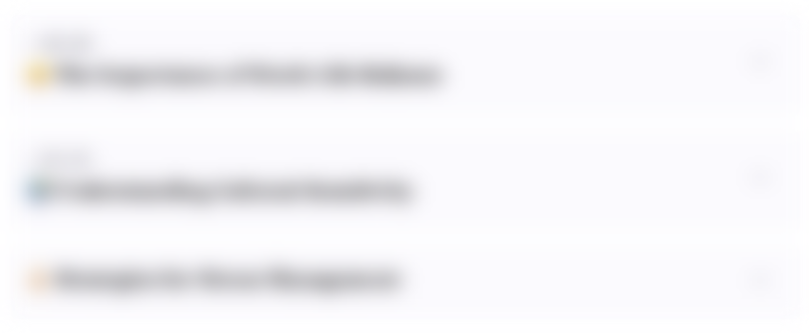
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
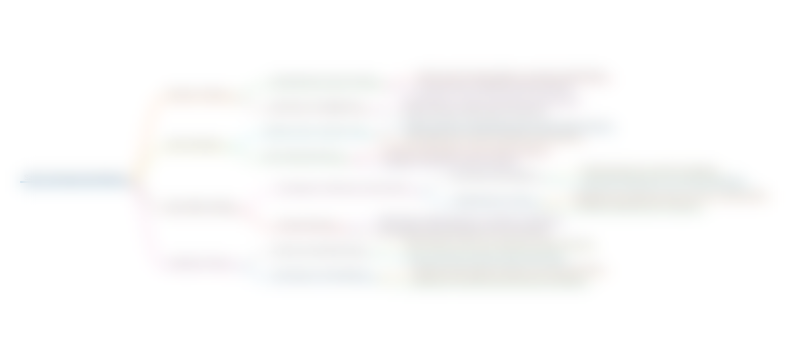
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
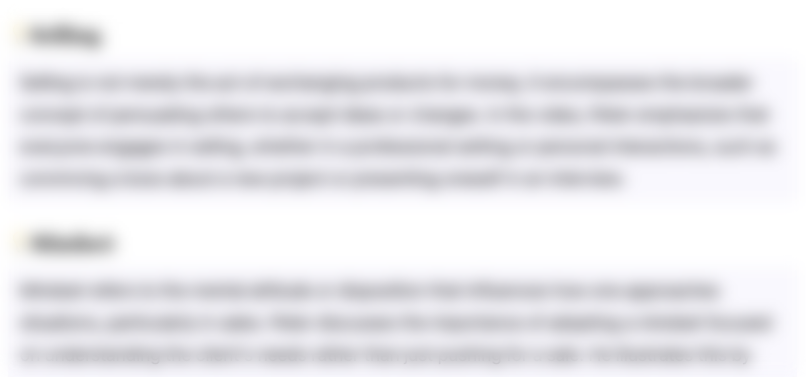
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
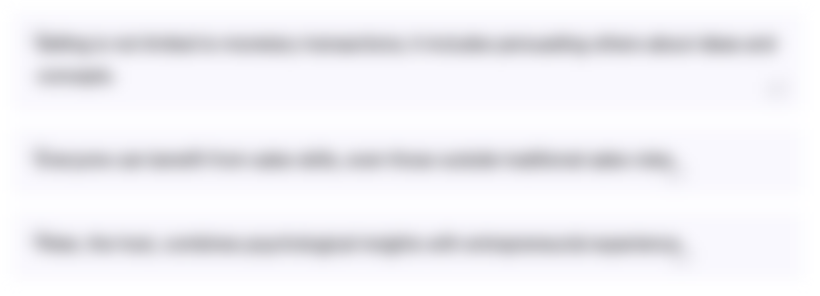
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
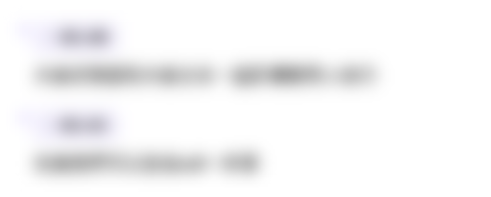
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
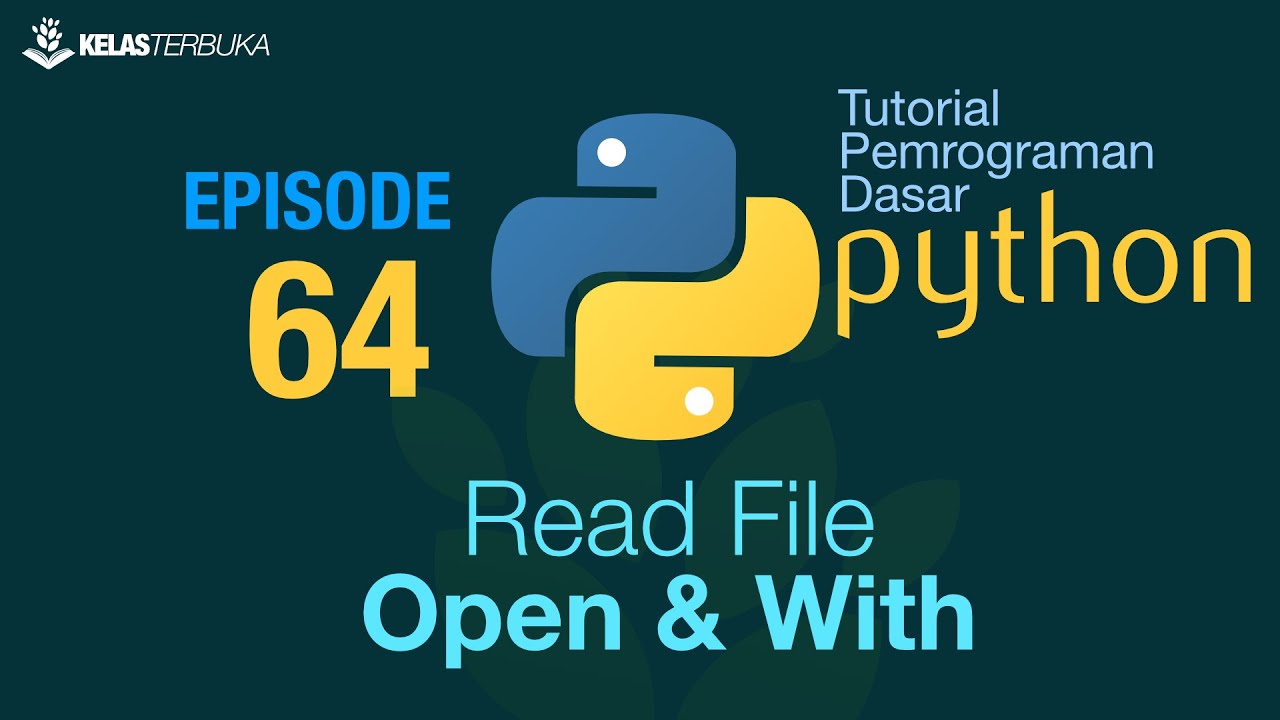
Belajar Python [Dasar] - 64 - Read external file - Open dan With
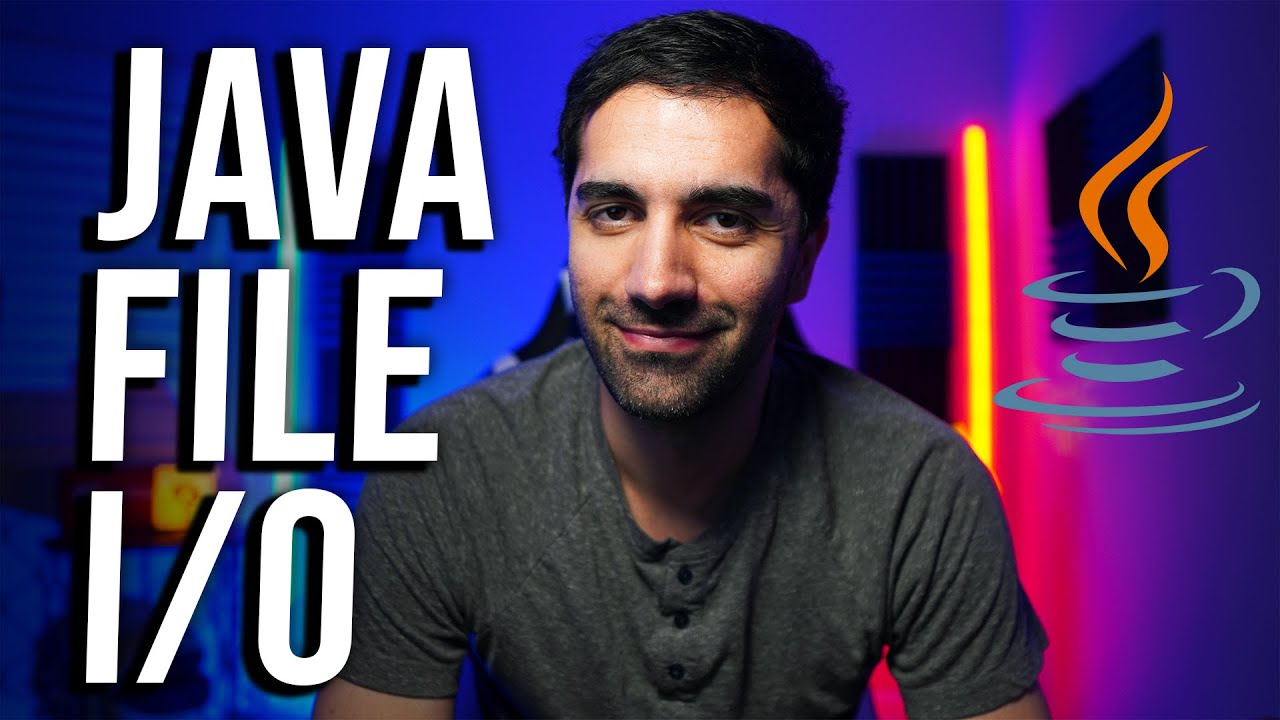
Java File I/O (Reading & Writing)

Java Tutorial For Beginners | Input & Output Streams In Java | IO Streams In Java | SimpliCode
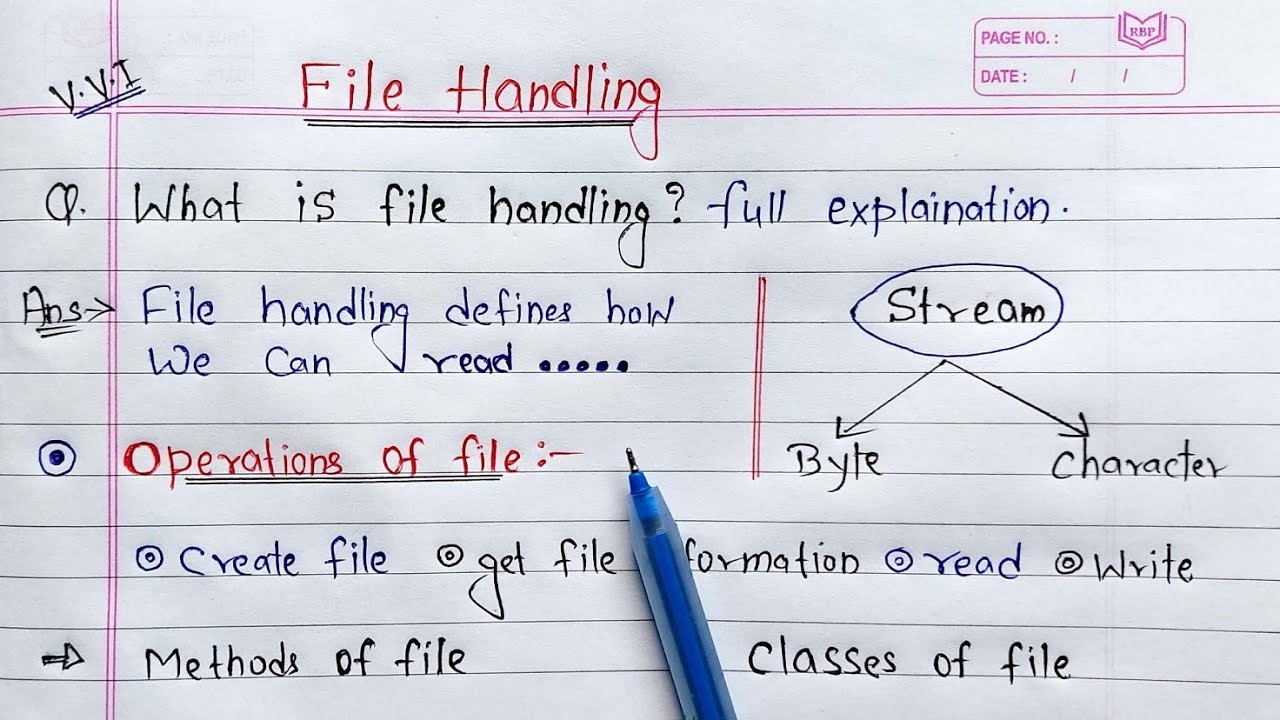
File Handling in Java | Java program to create a File

Tutorial-13: File Read and Write in SystemVue

C++ File Handling | Learn Coding
5.0 / 5 (0 votes)