Fabric Modding Tutorial - Minecraft 1.20: Advanced Item | #6
Summary
TLDRThis Minecraft modding tutorial introduces how to create a custom advanced item, specifically a metal detector. The item is coded with its own class, allowing unique functionalities such as checking for valuable blocks below the clicked block and notifying the player with coordinates. The tutorial covers item class creation, method overriding, and integrating the item into the game, including durability and damage handling. The metal detector item showcases the potential of modding to enhance gameplay with interactive, functional items.
Takeaways
- 🛠️ The tutorial focuses on adding a custom advanced item to Minecraft, specifically a 'metal detector' item that can detect valuable blocks like iron or diamond ore.
- 📦 The item is created within its own package for organizational purposes, following the convention of naming custom items with 'item' at the end.
- 🔧 The 'metal detector' item extends the base item class from the Minecraft modding API, allowing for the addition of custom functionality.
- 📝 The 'useOnBlock' method is overridden to implement the item's special behavior, which involves checking for valuable blocks below the clicked position.
- 🗄️ The script checks if the server is running the code (not the client) using 'context.getWorld().isClient' with a negation to ensure server-side execution.
- 🔍 A for loop is utilized to iterate through blocks below the clicked position, checking each block's state to determine if it's a valuable block.
- 💎 The 'isValuableBlock' method is a custom method that determines whether a block is considered valuable, such as iron or diamond ore.
- 📢 When a valuable block is found, the item outputs the block's coordinates to the player, and the item is damaged to simulate use.
- 🔨 The item has a durability limit set through 'fabric item settings', which determines how many times it can be used before breaking.
- 📝 The tutorial concludes with the item being registered within the mod and tested in-game, demonstrating its functionality and durability.
Q & A
What is the main focus of the tutorial described in the transcript?
-The main focus of the tutorial is to add a custom advanced item, specifically a metal detector, to Minecraft using modding.
What does the term 'advanced item' refer to in the context of Minecraft modding?
-In the context of Minecraft modding, an 'advanced item' refers to an item that has its own item class, allowing for the implementation of custom functionality beyond the standard capabilities of basic items.
Why is it recommended to end the name of custom items with 'Item' in Minecraft modding?
-It is recommended to end the name of custom items with 'Item' to clearly indicate that the class is an item class, which helps with organization and understanding within the codebase.
What method is used to define the behavior when the custom item is used on a block?
-The 'useOnBlock' method is used to define the behavior when the custom item is used on a block in Minecraft modding.
How does the metal detector item determine if a block is valuable?
-The metal detector item determines if a block is valuable by checking if the block state is of iron ore or diamond ore using a custom method called 'isValuableBlock'.
What is the purpose of the 'foundBlock' variable in the metal detector item's functionality?
-The 'foundBlock' variable is used to track whether a valuable block has been found during the search process. It is set to true when a valuable block is detected.
Why is it important to check if the code is running on the server and not the client in the metal detector item's functionality?
-It is important to check if the code is running on the server and not the client to ensure that the block checking and item damaging logic only occurs on the server, preventing potential client-side exploits or inconsistencies.
How does the tutorial handle the durability of the metal detector item in Minecraft?
-The tutorial handles the durability of the metal detector item by damaging it by one each time it is used to find a valuable block, with a maximum durability set to 64, after which the item will break.
What is the role of the 'use' method in the context of the custom metal detector item?
-The 'use' method in the context of the custom metal detector item is called when the player uses the item without targeting a block, but in this tutorial, the focus is on the 'useOnBlock' method for the item's functionality.
How is the metal detector item registered within the modding framework as described in the transcript?
-The metal detector item is registered within the modding framework by creating a new instance of the item class in the 'ModItems' class and setting its properties, such as max damage, and then adding it to the item group.
Outlines
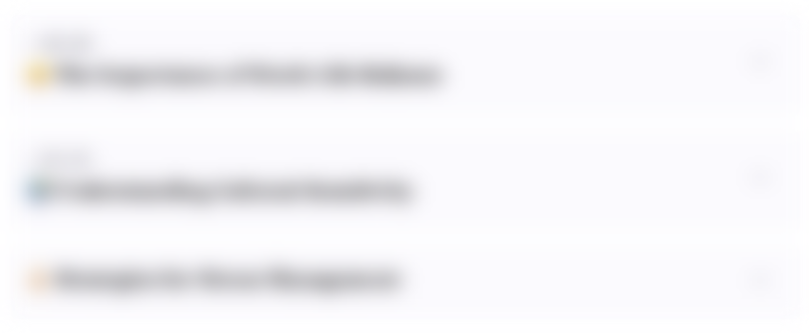
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
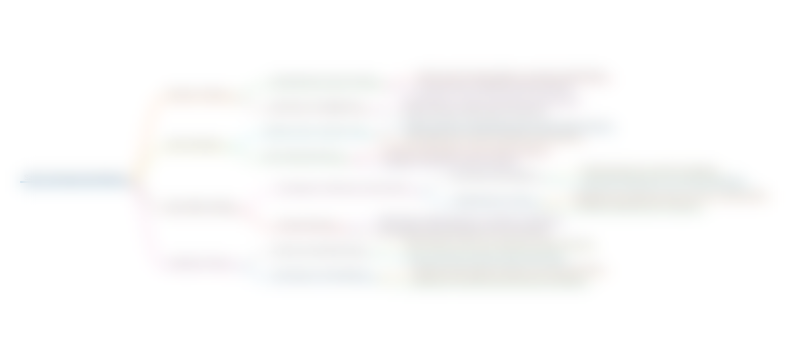
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
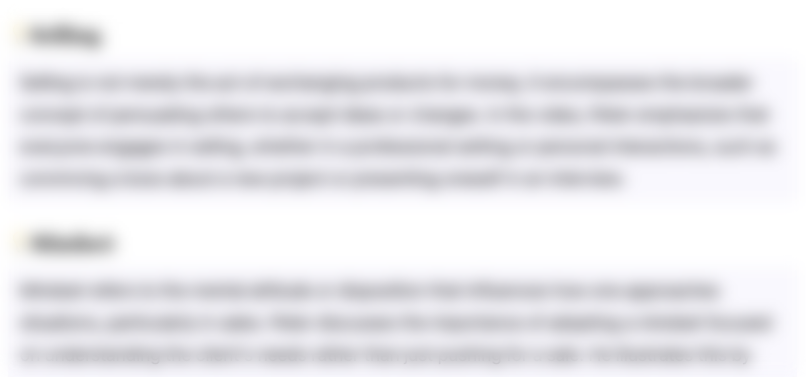
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
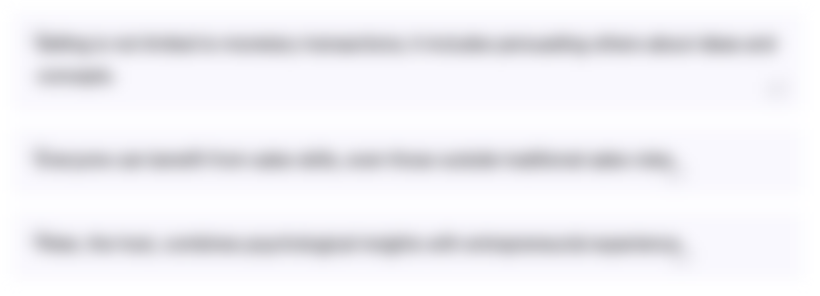
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
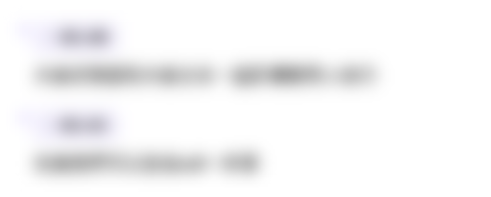
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
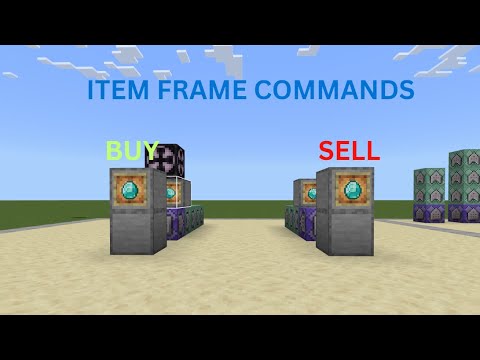
Minecraft Bedrock Item Frame Shop Command Block Tutorial
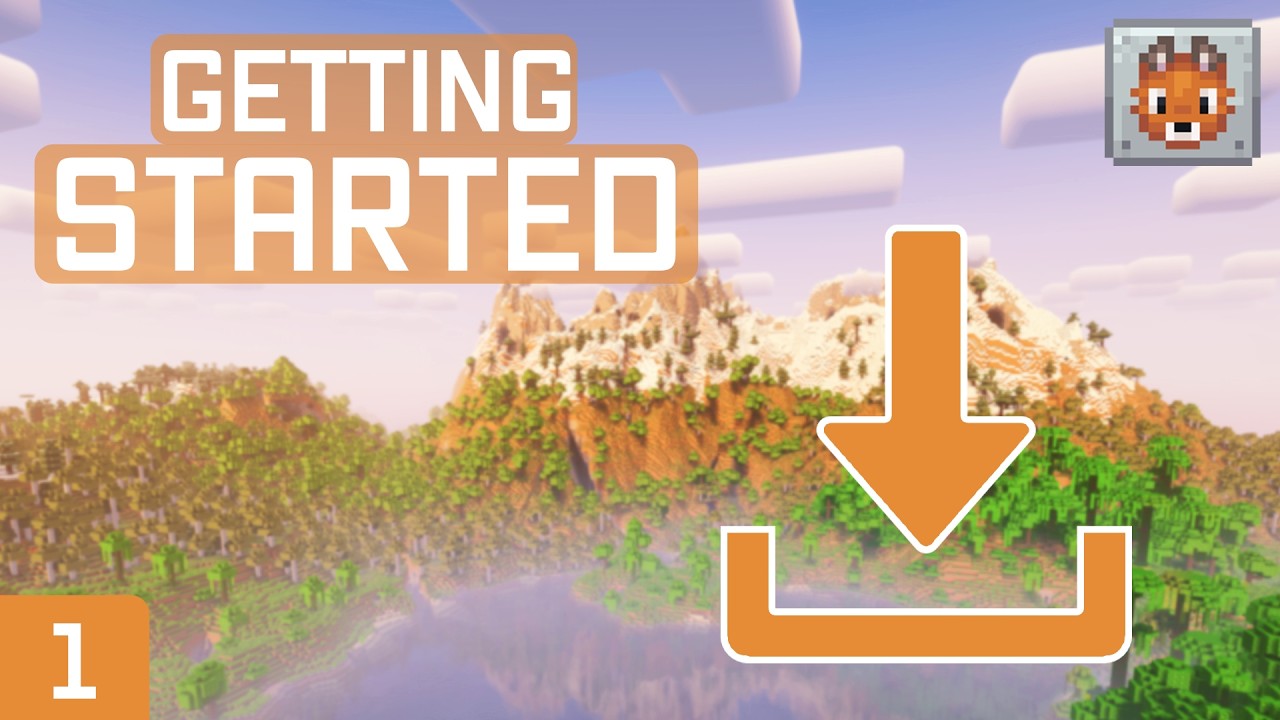
NeoForge Modding Tutorial - Minecraft 1.21: Getting Started | #1

Structures with Loot Chests (Mcreator 2022.1)
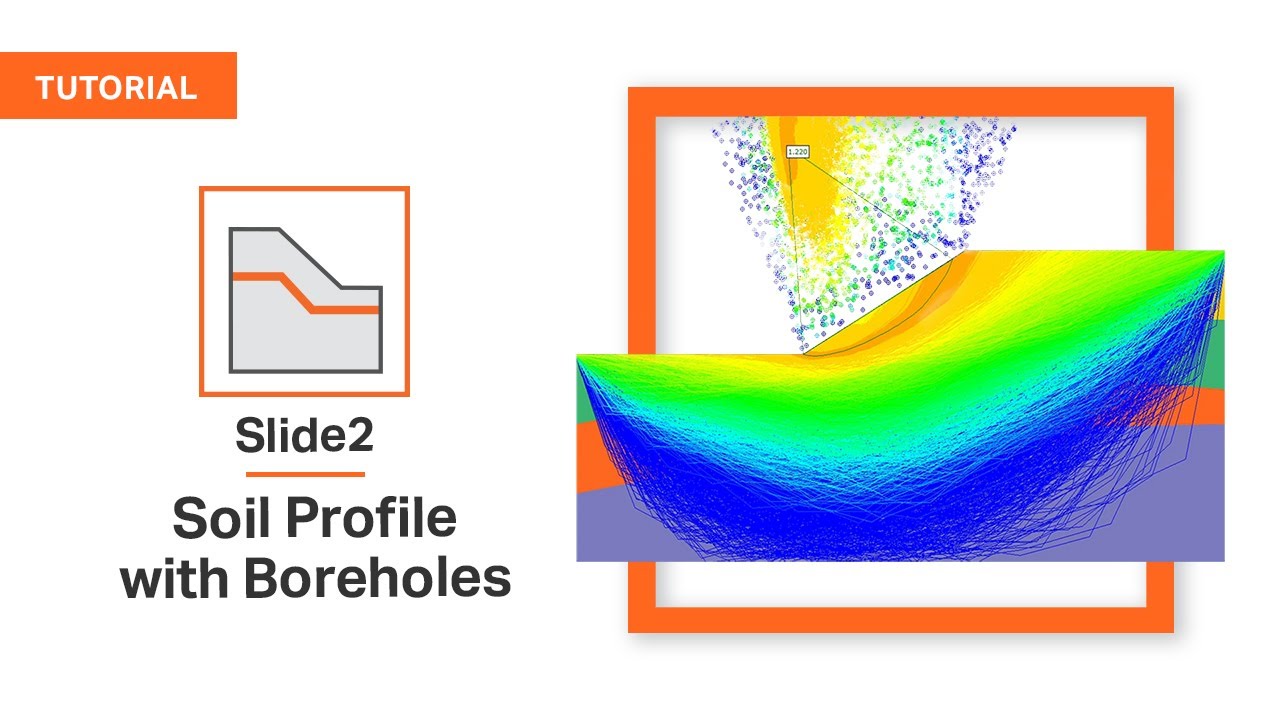
Slide2 Tutorial: Soil Profile Modelling with Boreholes

Part 1 - NGINX Web Server ( Installation & Configuration )
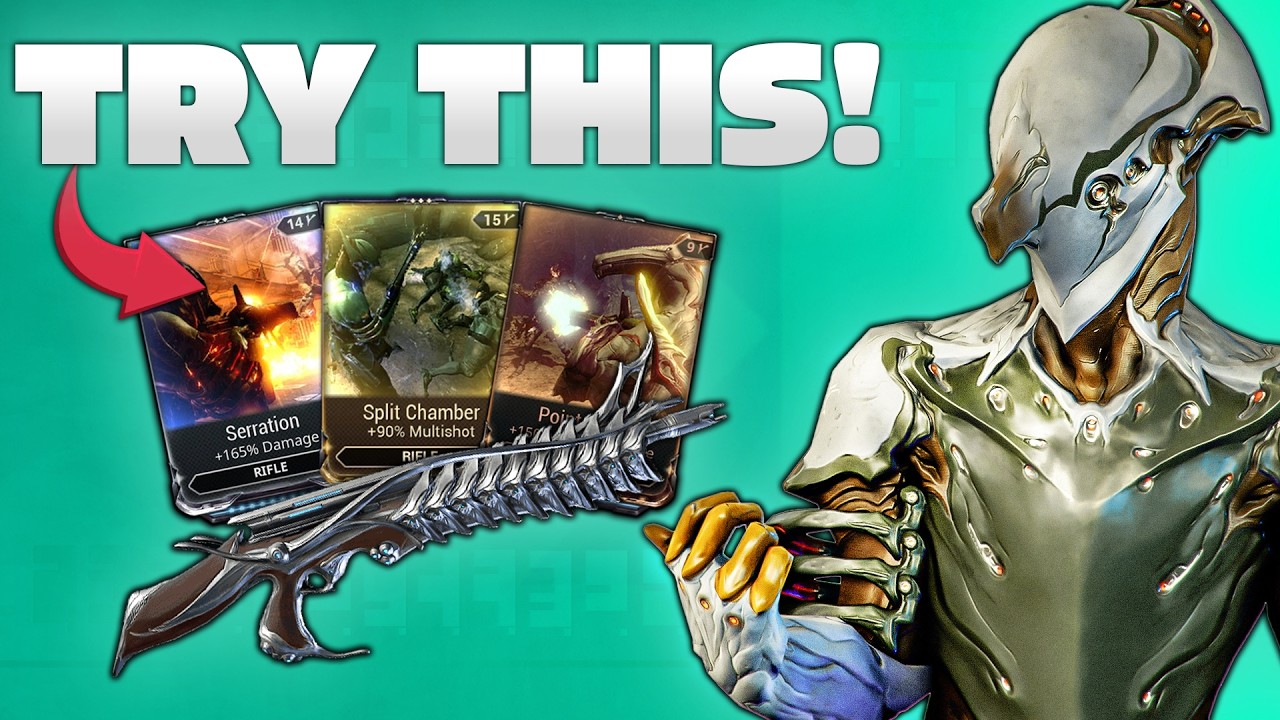
How to make ANY Weapon OP in Warframe!
5.0 / 5 (0 votes)