State Update Batching in Practice | Lecture 133 | React.JS 🔥
Summary
TLDRThis video script delves into the intricacies of state updates in React, demonstrating how React batches state updates asynchronously for improved performance. Through practical examples and code snippets, it explores the behavior of state updates within event handlers, setTimeout functions, and the changes introduced in React 18 for automatic batching across different scenarios. The script provides a comprehensive understanding of when and how to use callback functions for setting state based on the previous state, ensuring safe and predictable updates. With a hands-on approach, it effectively showcases the power of React's state management and batching mechanisms.
Takeaways
- 😄 React batches multiple state updates into a single re-render to improve performance.
- 🤔 Using console.log('render') can demonstrate when components re-render due to state changes.
- ⏱️ State updates in React are asynchronous, so accessing the updated state immediately after setState() will return the previous state value.
- ✅ When updating state based on the previous state, always use the callback function form of setState() to ensure the correct state is accessed.
- 🔄 React will not re-render a component if the new state is identical to the current state.
- ⚡ In React 18, state updates are automatically batched, even for asynchronous operations like setTimeout() and promises.
- 👴 In React 17 and earlier versions, state updates in asynchronous operations were not batched automatically.
- 🔑 Understanding batched updates and the asynchronous nature of setState() is crucial for writing efficient and bug-free React applications.
- 📝 The provided script demonstrates several examples and scenarios to illustrate these concepts in action.
- 🚀 Mastering state management and updates is a fundamental skill for becoming a proficient React developer.
Q & A
What is the purpose of the `handleUndo` function?
-The `handleUndo` function is implemented to reset the `showDetails` state to `true` and the `likes` state to `0`. It is used to undo the changes made to these two states.
How does React batch state updates, and how can this be proven?
-React batches multiple state updates together and only triggers a single re-render. This can be proven by logging a string to the console within the component's render method. If only one log appears after multiple state updates, it means the updates were batched and triggered a single re-render.
Why does the `console.log` statement with 'render' only appear once when clicking the 'undo' button?
-The 'render' log appears only once because the two state updates (`setShowDetails` and `setLikes`) were batched together, causing a single re-render of the component.
Why is the state updating asynchronous in React?
-State updating is asynchronous in React because the state is not updated immediately after calling the state setter function. Instead, the state is updated during the re-rendering process, which happens after the state setter function is called.
Why is it recommended to use a callback function when updating state based on the previous state?
-Using a callback function when updating state based on the previous state ensures that the state update is performed with the latest state value. This safeguards against potential issues caused by stale state values, especially when the component's logic changes or when the state update function is called in different contexts.
Why does the component not re-render when clicking the 'undo' button after the state has already been reset?
-The component does not re-render because both the `showDetails` and `likes` states were already at their default values (`true` and `0`, respectively). Since the new state values were equal to the current state, React did not trigger a re-render.
What is the purpose of the `handleTripleInc` function?
-The `handleTripleInc` function is intended to increase the `likes` state by 3 when clicking the 'Next' button with three plus signs.
Why does the `likes` state only increase by 1 when calling `setLikes` three times in the `handleTripleInc` function?
-The `likes` state only increases by 1 because the state updates are still asynchronous, and the subsequent state updates are based on the previous (stale) state value, not the updated value.
How does using a callback function in `setLikes` solve the issue of only increasing the `likes` state by 1?
-Using a callback function in `setLikes` allows access to the latest state value within the callback. This ensures that each subsequent state update is based on the most recent state value, enabling the `likes` state to increase by 3 as intended.
What is the difference between automatic batching in React 17 and React 18 when using `setTimeout`?
-In React 17, automatic batching did not occur inside a `setTimeout` function, resulting in multiple re-renders. However, in React 18, batching occurs automatically in `setTimeout` and other asynchronous situations, leading to a single re-render.
Outlines
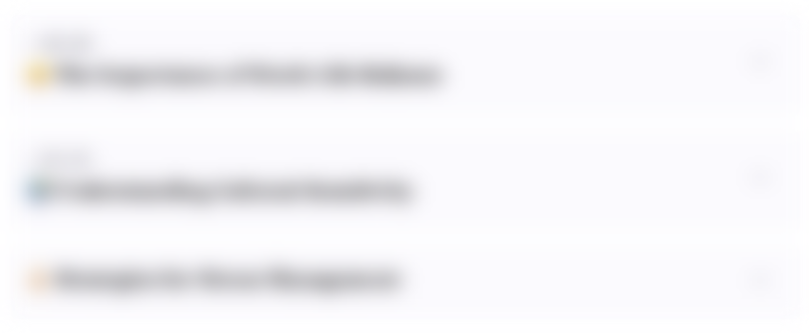
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
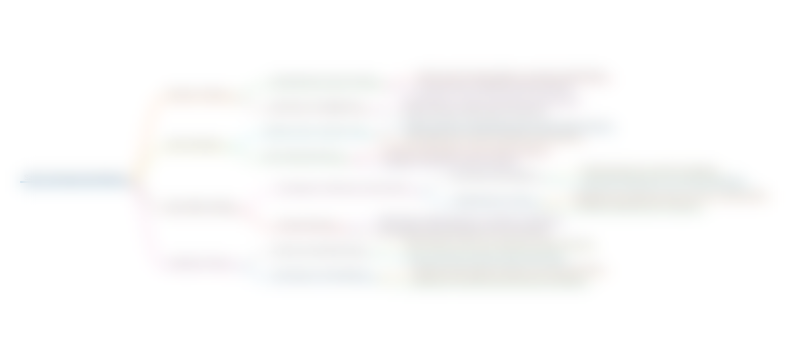
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
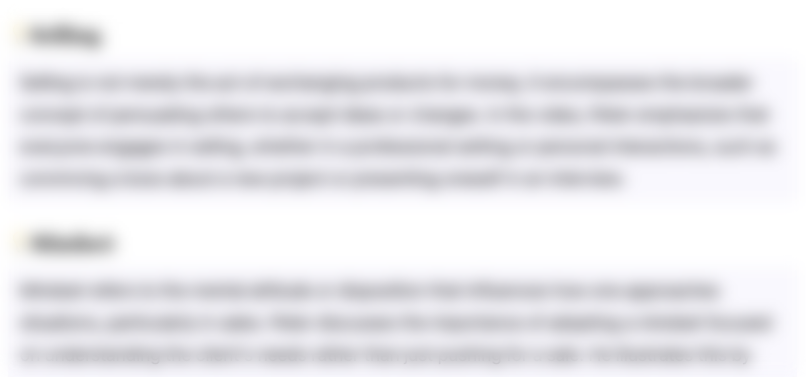
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
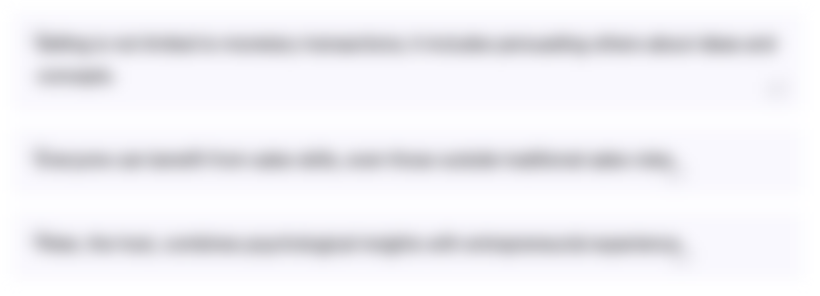
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
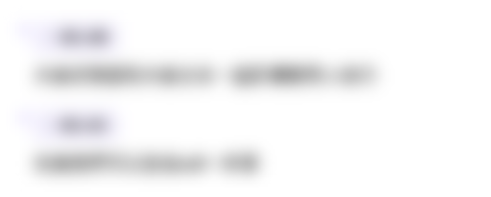
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

State Update Batching | Lecture 132 | React.JS 🔥

A react interview question on counter
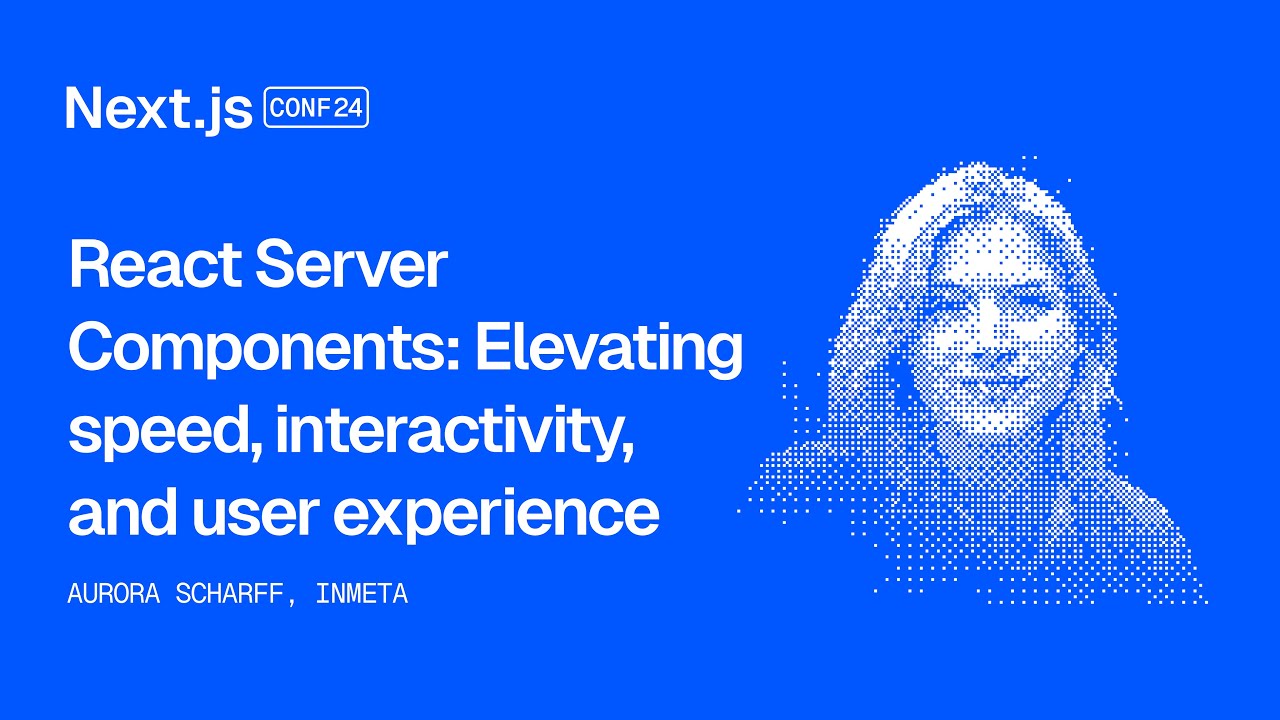
React Server Components: Elevating speed, interactivity, and user experience (Aurora Scharff)
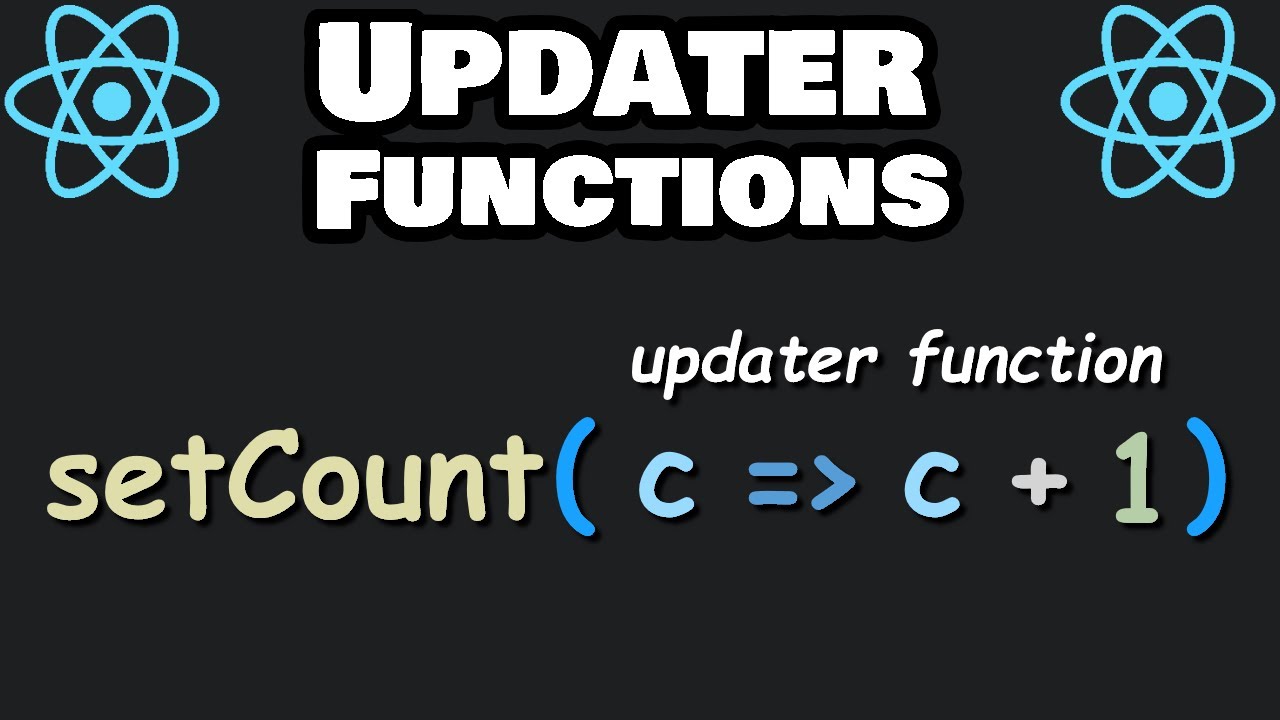
What are React updater functions? 🔄
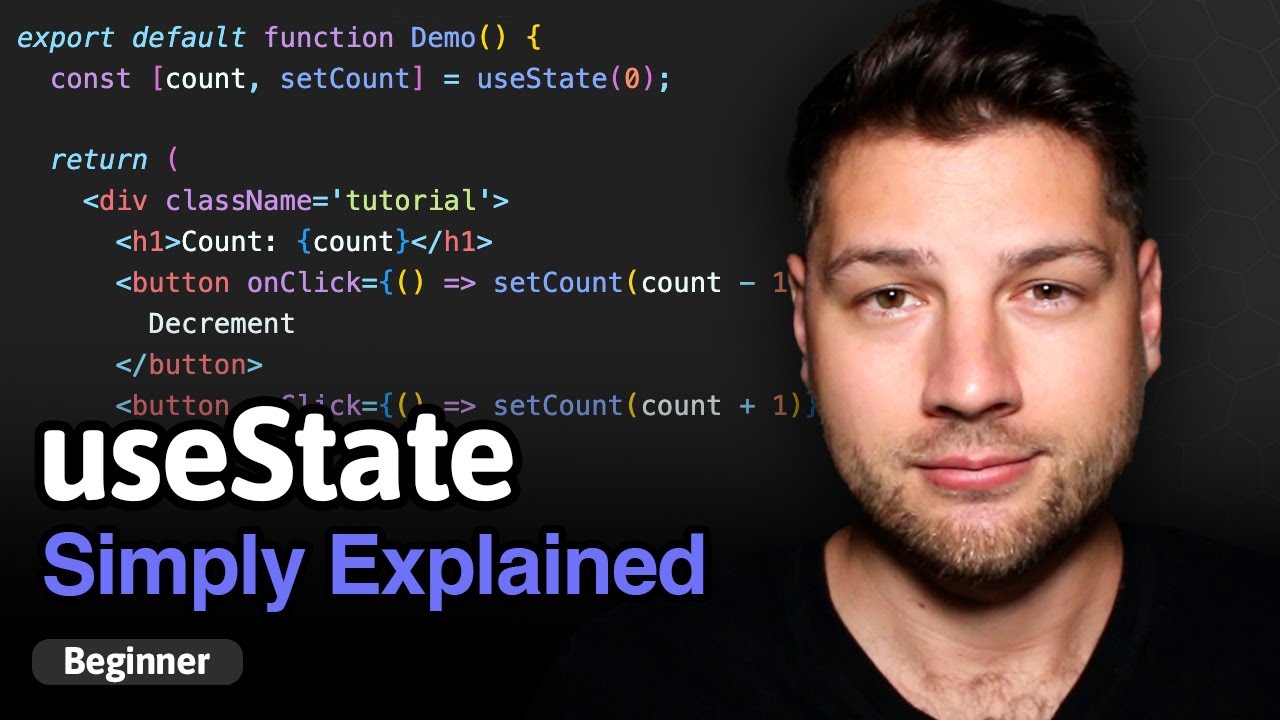
Learn React Hooks: useState - Simply Explained!

States | Mastering React: An In-Depth Zero to Hero Video Series
5.0 / 5 (0 votes)