Introduction To Testing In JavaScript With Jest
Summary
TLDRIn this informative tutorial, Kyle from Web Dev Simplified introduces viewers to the fundamentals of JavaScript testing using the Jest library. He demonstrates how to set up a testing environment, write unit tests for simple functions like sum, subtract, and array cloning, and utilize Jest's matchers to ensure code correctness. The video also highlights the importance of testing in development, its role in identifying bugs during code changes, and the confidence it provides when making large-scale updates to complex applications.
Takeaways
- 😀 Testing in JavaScript is a crucial skill for developers that can set them apart in the job market.
- 🔍 The video introduces 'jest', a popular JavaScript testing library, as an essential tool for testing.
- 📚 The tutorial starts with the basics of setting up 'jest' by initializing 'package.json' and installing it as a development dependency.
- 🛠️ It demonstrates how to write tests for simple JavaScript functions like adding, subtracting numbers, and duplicating arrays.
- 📝 The script explains the process of creating test files with the naming convention of appending '.test' to the original file name.
- 🧐 The importance of using 'expect' and 'toBe' in 'jest' for asserting that function outputs match expected results is highlighted.
- 🔄 The video clarifies the difference between 'toBe' (for strict equality) and 'toEqual' (for deep equality) in testing arrays.
- 📊 'jest' offers code coverage analysis, which is shown to ensure that all parts of the code are being tested.
- 🔍 The script emphasizes the value of unit testing, which focuses on testing individual components or functions in isolation.
- 🛡️ Tests provide confidence when making changes to the code, as they help identify any breaks or issues that arise from modifications.
- 🌟 The video concludes by advocating for the practice of testing as a means to ensure code quality and simplify the development process.
Q & A
What is the main topic of the video?
-The main topic of the video is testing in JavaScript, focusing on how to get started with testing using the Jest library.
Why is testing an important skill for developers?
-Testing is an important skill for developers because it helps set them apart from others who don't know testing, and it gives them an advantage when applying for jobs.
What does the instructor suggest for simplifying web development?
-The instructor suggests using the Jest library for testing in JavaScript as a way to simplify web development and ensure code quality.
What are the JavaScript files created for the purpose of this video?
-The JavaScript files created for this video are simple functions for adding two numbers, subtracting two numbers, and duplicating an array.
What is the spread operator in JavaScript and what does it do?
-The spread operator in JavaScript is a syntax that allows the expansion of elements from an iterable (like an array) into individual elements, and it is used for duplicating an array in the video.
How do you install the Jest library for testing in JavaScript?
-You install the Jest library by running 'npm install --save-dev jest', which adds Jest as a development dependency.
What is the purpose of the 'test' script in the package.json file?
-The 'test' script in the package.json file is used to run the Jest testing framework with the command 'npm test'.
How do you create a test file for a JavaScript function?
-To create a test file, you name it the same as the file you want to test with '.test' appended to the filename and '.js' as the extension.
What is the 'expect' function used for in Jest tests?
-The 'expect' function in Jest is used to specify the expected outcome of a test, allowing for assertions such as equality or matching certain conditions.
What does the 'toBe' matcher in Jest do?
-The 'toBe' matcher in Jest checks for strict equality, ensuring that the expected value and the actual value are identical in both value and reference.
Why is it important to test individual functions in unit testing?
-Testing individual functions in unit testing is important because it isolates each part of the code, making it easier to identify and fix issues when a test fails.
How can running tests help with making changes to the code?
-Running tests helps with making changes to the code by verifying that the changes did not break any existing functionality, providing immediate feedback if something is wrong.
What is the purpose of code coverage in testing?
-The purpose of code coverage in testing is to measure how much of the codebase is executed during the tests, ensuring that all parts of the code are tested and reducing the risk of undetected issues.
How does testing provide confidence when making large changes to an application?
-Testing provides confidence when making large changes to an application by quickly identifying any breaks or issues, allowing developers to ensure that the application remains stable and functional after changes.
What is the benefit of having a test suite for a large application?
-A test suite for a large application allows for quick and automated testing of the entire codebase, reducing the need for time-consuming manual testing and providing assurance that changes do not introduce new issues.
Outlines
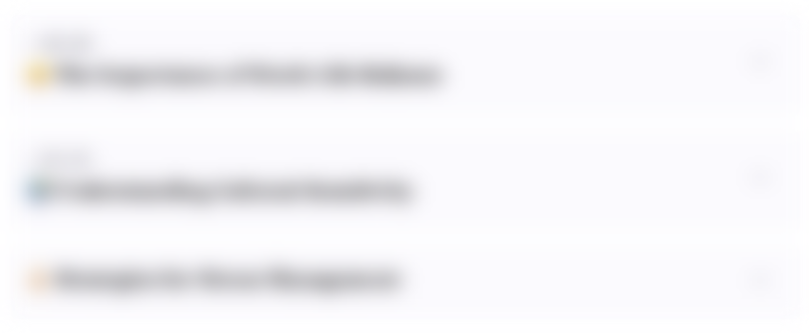
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
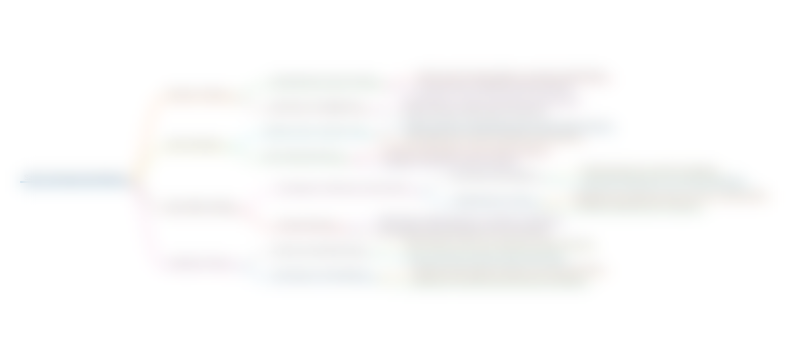
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
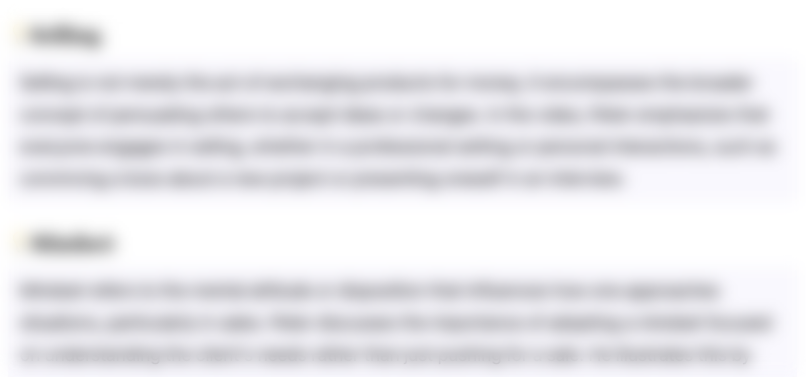
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
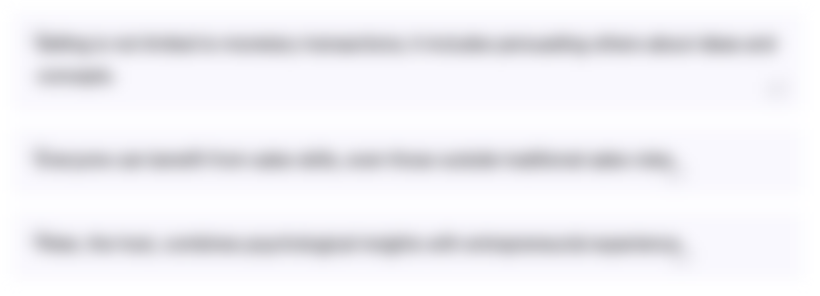
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
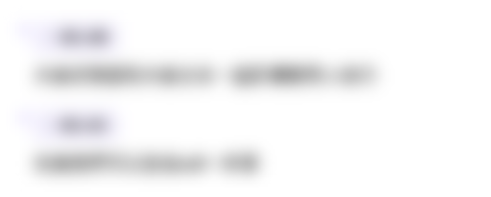
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)