Learn JavaScript Event Listeners In 18 Minutes
Summary
TLDRThis video from Web Dev Simplified with Kyle offers an in-depth exploration of JavaScript event listeners, suitable for both beginners and experienced developers. It begins with the fundamentals of creating event listeners, explaining how they work, and progresses to more advanced topics like event capturing and bubbling. The tutorial also covers delegation, stopping event propagation, and techniques for running events a specific number of times. Kyle simplifies complex concepts and provides practical examples, ensuring viewers gain a comprehensive understanding of event listeners to enhance their web development skills.
Takeaways
- 😀 Event listeners are essential for interactive web development, suitable for beginners and experienced developers alike.
- 📚 The video covers the basics of creating event listeners, explaining how they work and their importance in web development.
- 🔍 Capturing, bubbling, and delegation are key concepts in understanding how events propagate through the DOM.
- 👆 The 'target' property within the event object is crucial as it identifies the element that triggered the event.
- 🔄 Multiple event listeners can be added to the same element, and they execute in the order they were defined.
- 💡 Event bubbling is the process where an event works its way from the deepest nested element to the outermost element.
- 🔍 Event capturing is the opposite of bubbling, where the event starts at the outermost element and moves towards the target element.
- 🛑 The 'stopPropagation' method can be used to halt the event from continuing through the capture or bubble phase.
- 🔄 The 'once' option in event listeners allows an event to trigger just once before automatically removing itself.
- ♻️ The 'removeEventListener' method provides the functionality to manually remove an event listener when needed.
- 🎯 Event delegation is a powerful technique for handling events on dynamically added elements without the need to attach individual listeners to each one.
Q & A
What is the main topic of the video?
-The main topic of the video is event listeners in JavaScript, covering their basics, how they work, capturing and bubbling, delegation, and additional tips.
What are the two parameters required to create an event listener according to the video?
-The two parameters required to create an event listener are the type of event to listen for and a callback function that runs every time the event occurs.
What is the purpose of the 'target' property in an event object?
-The 'target' property in an event object refers to the element that was clicked on or the element on which the event occurred. It is important for identifying the specific element that triggered the event.
How can multiple event listeners be added for the same element?
-Multiple event listeners can be added by calling the 'addEventListener' method multiple times with different callback functions for the same event type on the same element.
What is event bubbling?
-Event bubbling is the process where an event works its way from the closest element to the furthest away element in the DOM tree, triggering event listeners for all elements along the path from the target to the document.
What is event capturing?
-Event capturing is the opposite of bubbling. It starts at the outermost element (document) and moves towards the target element, triggering event listeners in a top-down approach.
How can you stop the propagation of an event?
-You can stop the propagation of an event by calling the 'stopPropagation' method on the event object (e) within an event listener.
What is the purpose of the 'once' option in the 'addEventListener' method?
-The 'once' option, when set to true, ensures that the event listener will run only one time and then remove itself, preventing it from triggering again on subsequent events.
Why is it necessary to use a separate function for 'addEventListener' and 'removeEventListener'?
-Using a separate function ensures that the same function reference is used for both adding and removing the event listener. Anonymous functions created inline may not be the same, preventing 'removeEventListener' from correctly removing the listener.
What is event delegation and how does it solve the problem of dynamically added elements not having event listeners?
-Event delegation is the technique of adding an event listener to a parent element that will handle events triggered by its children, even those added dynamically after the listener was set. It leverages the bubbling phase of event propagation to manage events for all matching child elements.
Can you provide an example of how to implement event delegation?
-To implement event delegation, you can add an event listener to a common parent element (like 'document') and check if the 'target' of the event matches a specific selector (e.g., 'div'). If it matches, the callback function is executed, thus handling the event for all elements that match the selector, including those added later.
Outlines
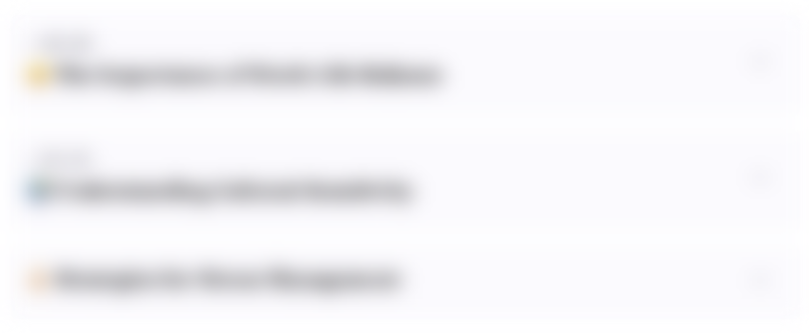
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
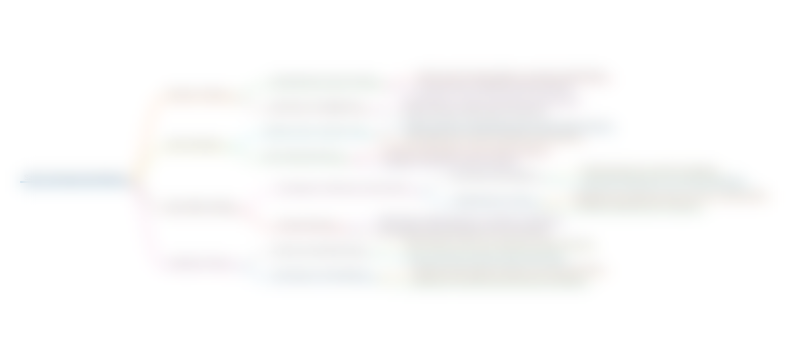
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
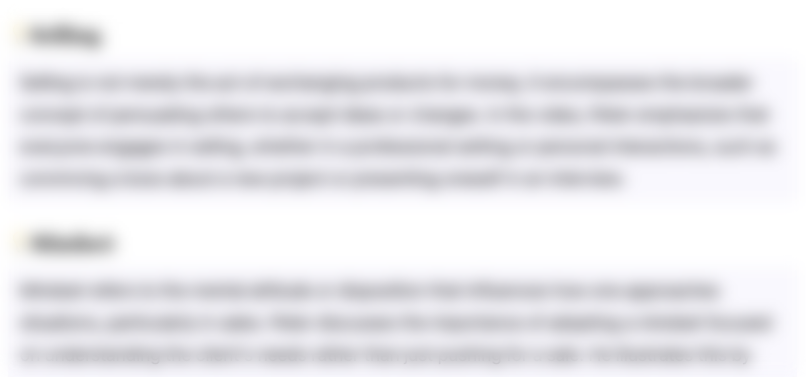
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
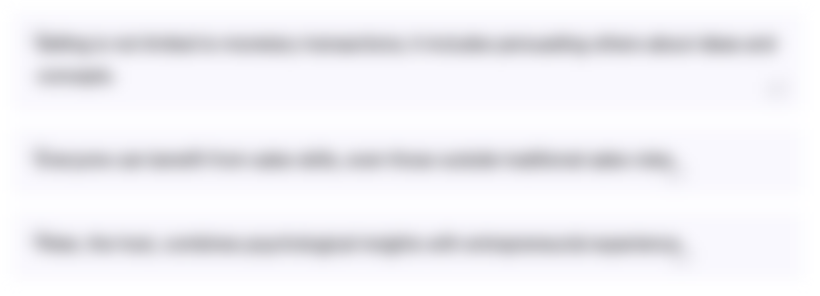
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
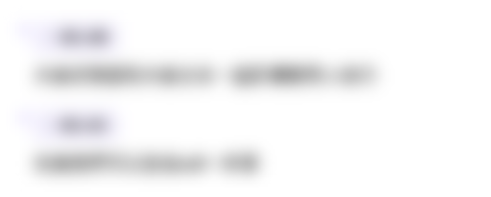
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)