Studi Kasus Stack - Histori Browser
Summary
TLDRThis video tutorial covers the implementation of a stack data structure to simulate a browser's history feature. The tutorial demonstrates how to store and navigate URLs using stack operations like push and pop. It also explains parsing and extracting metadata from URLs, including titles and descriptions, using Python libraries. Additionally, the tutorial introduces web scraping techniques with the requests and lxml libraries, showcasing how to create a simple browser-like program that manages back and forward navigation. The video provides a hands-on example for understanding stack usage in real-world applications.
Takeaways
- 📚 The tutorial is about implementing a browser history feature using a stack data structure.
- 💻 The program simulates browser behavior, focusing on history management, including back and forward navigation.
- 🔄 The stack is used to store URLs as the user navigates through them, allowing for back and forward operations.
- 🔍 The tutorial covers the creation of a stack class with methods like push, pop, and checking the last item without removal.
- 🔒 Emphasis is placed on encapsulation, ensuring that data stored in the stack is private and only accessible through specific methods.
- 🌐 The tutorial explains how to parse a URL to extract components like scheme, net location, and port, using a Python library.
- ⚙️ The script demonstrates how to add URLs to history, navigate back and forward, and display the entire history.
- 🛠️ A bonus feature is added where the program retrieves and displays metadata like the title and description from a webpage.
- 📦 The tutorial makes use of Python libraries such as requests for fetching web content and lxml for parsing HTML.
- 📝 The final part of the tutorial highlights the potential of the program for web scraping and further browser automation.
Q & A
What is the main objective of the tutorial described in the script?
-The main objective of the tutorial is to teach how to implement a stack data structure to simulate browser history navigation, specifically handling back and forward operations.
What data structure is used to simulate browser history in the tutorial?
-The stack (or 'stek' in the script) data structure is used to simulate browser history, allowing the storage and retrieval of URLs in a Last-In-First-Out (LIFO) manner.
How does the program handle the 'back' and 'forward' browser actions?
-The 'back' action pops the last URL from the history stack and moves it to the forward stack. Conversely, the 'forward' action pops the last URL from the forward stack and moves it back to the history stack.
Why is encapsulation important in the context of this tutorial?
-Encapsulation is important because it restricts access to the stack's internal data, ensuring that the data can only be modified using defined methods like push and pop, preventing unauthorized or unintended modifications.
What are the two key metadata elements retrieved from a web page in the tutorial?
-The two key metadata elements retrieved from a web page are the 'title' and the 'description' of the page, which are commonly used to understand the content and purpose of the page.
How does the program extract and display the URL metadata?
-The program uses the `lxml` and `requests` libraries to parse the HTML content of a web page and extract metadata like the title and description, which are then displayed to the user.
What is the significance of the `__data` attribute in the stack implementation?
-The `__data` attribute is a private attribute that stores the stack's elements. Its private status ensures that it can only be accessed and modified through the stack's methods, following the principle of encapsulation.
Why does the program not implement a real browser but only simulates the browser history?
-The program focuses on teaching the stack data structure and its applications rather than building a full-fledged browser, so it only simulates the browser history without rendering actual web pages.
What libraries are recommended for more advanced web scraping in Python?
-For more advanced web scraping, the tutorial suggests using libraries like `BeautifulSoup` for parsing HTML, along with `requests` for fetching web content. For even more complex tasks, tools like `Scrapy` are recommended.
What is the purpose of the `Len` function in the stack implementation?
-The `Len` function in the stack implementation is used to return the number of elements currently stored in the stack, helping to manage and assess the stack's size.
Outlines
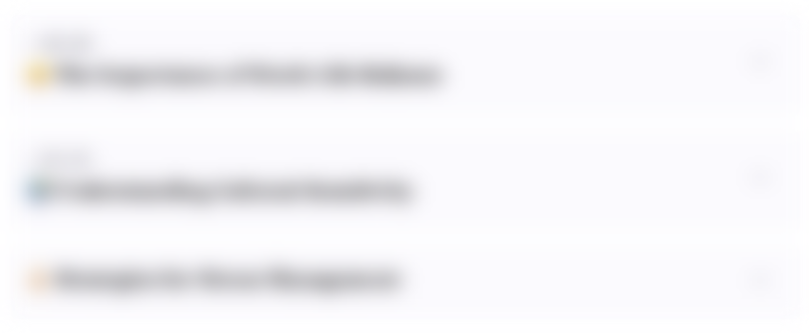
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
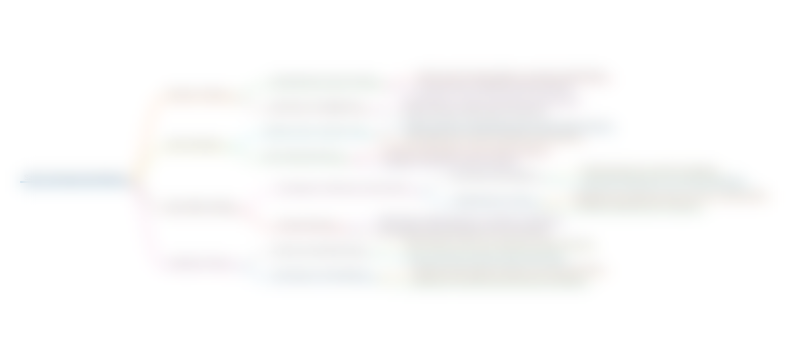
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
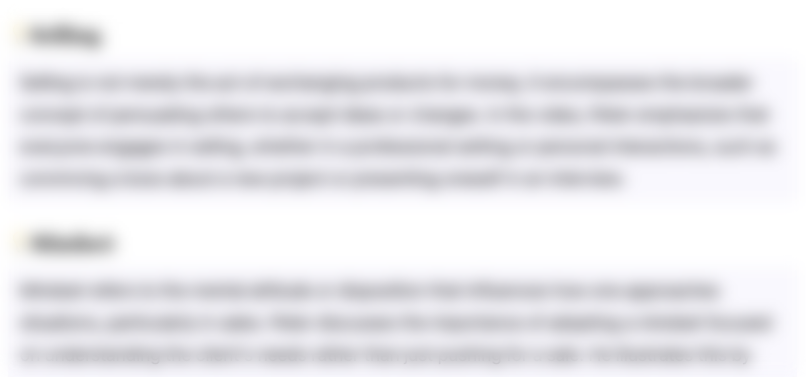
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
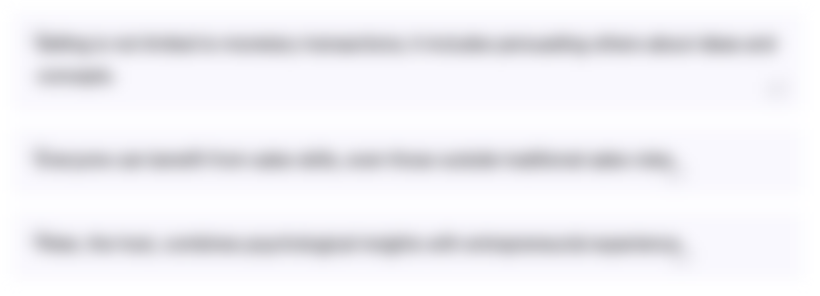
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
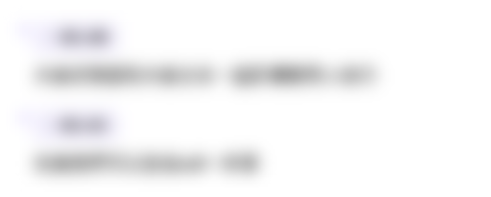
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
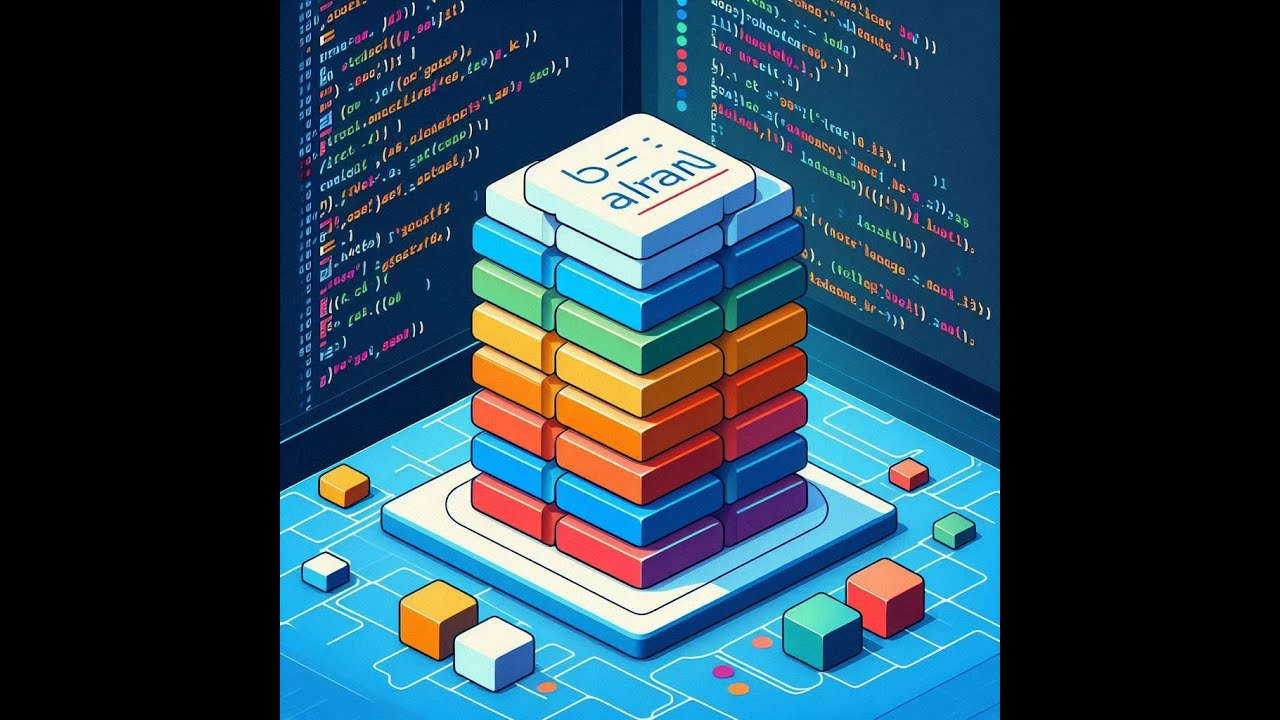
Implementing Stack Using Array
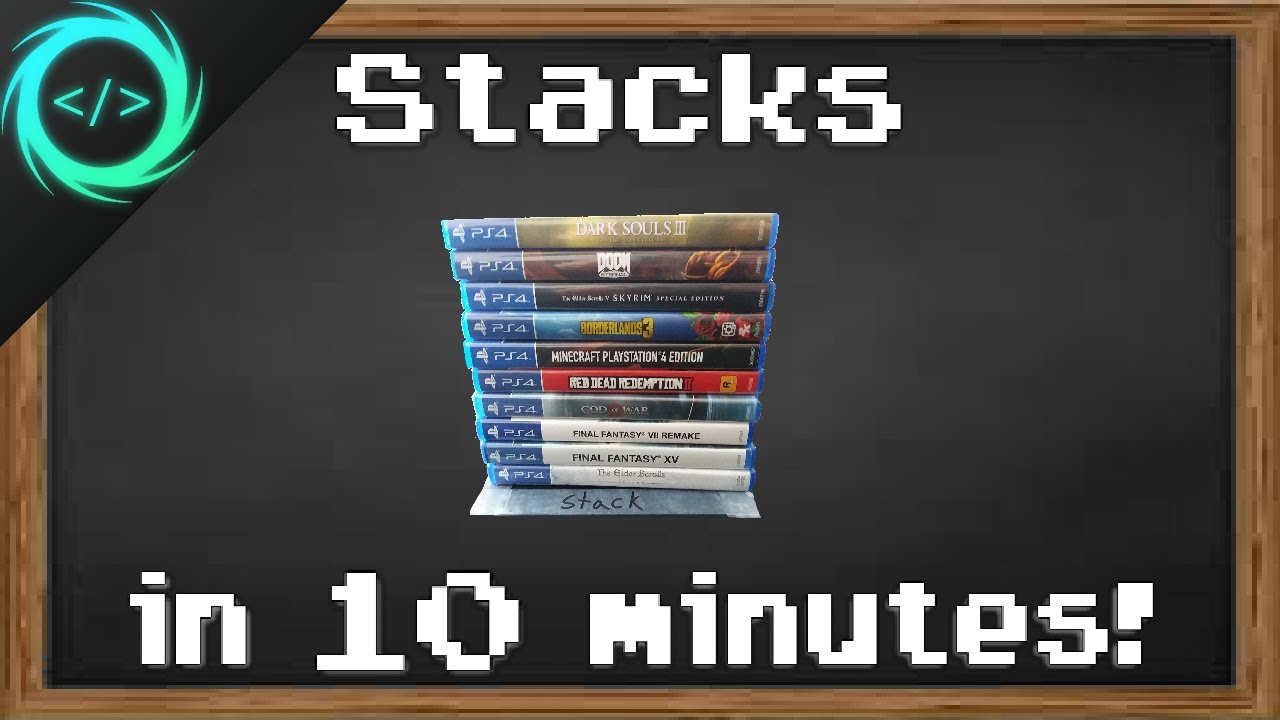
Learn Stack data structures in 10 minutes 📚
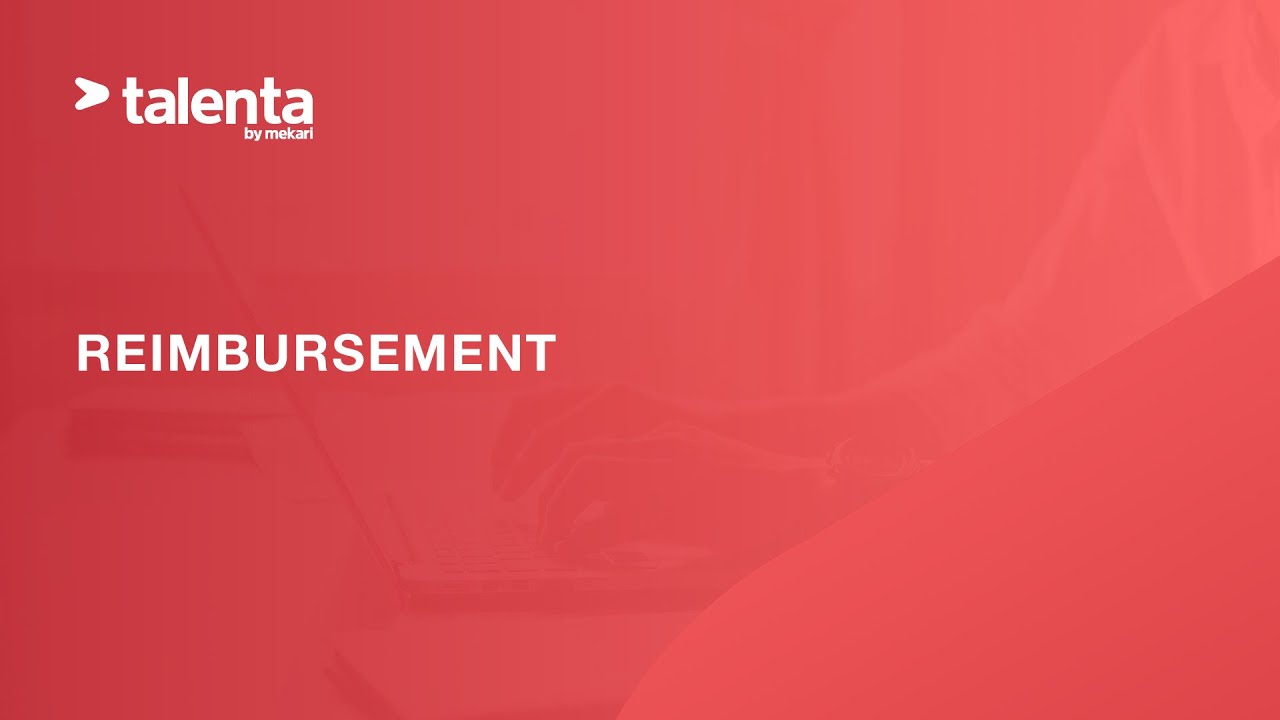
Tutorial - Reimbursement
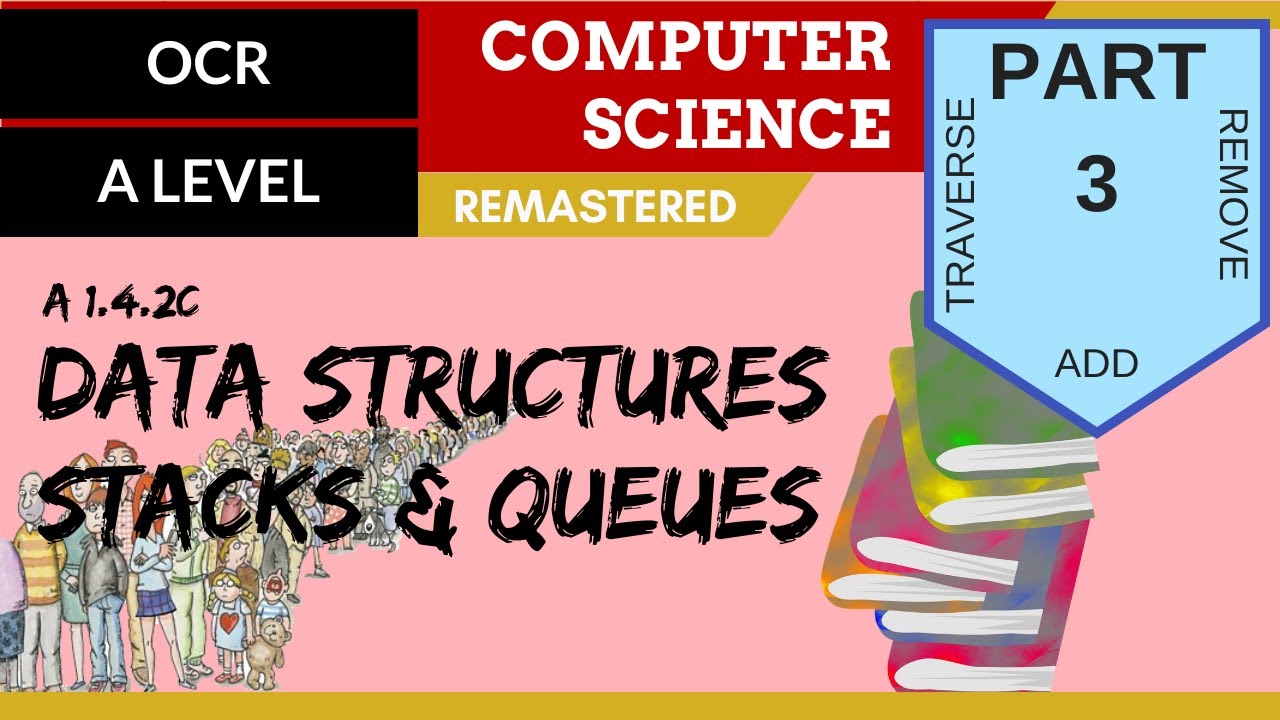
94. OCR A Level (H046-H446) SLR14 - 1.4 Data structures part 3 - Stacks & queues (operations)

PEMODELAN HEC-RAS 1D : JEMBATAN/BRIDGE

Lecture 5 Program structure, syntax and program to print single character in assembly language urdu
5.0 / 5 (0 votes)