Implementing Stack Using Array
Summary
TLDRIn this tutorial, the presenter demonstrates how to implement a stack class in Java. The video walks through the process of creating a stack using a class with key methods: `push` (to add elements), `display` (to show stack data), `pop` (to remove elements), and `top` (to view the top element). By pushing and popping values, the presenter explains how the stack operates in a Last In, First Out (LIFO) manner. The tutorial also includes examples to test the implementation, showing how data changes as operations are performed, ultimately helping viewers understand the stack structure and its use in programming.
Takeaways
- π The script demonstrates how to create a stack class in Java, including initializing variables and methods.
- π The stack class includes key variables: 'top' (index), 'maxSize' (the maximum size of the stack), and 'data' (the array to store stack elements).
- π The push method adds an integer to the stack and increases the 'top' index each time a new element is pushed.
- π The display method shows all the elements in the stack, starting from the topmost element.
- π The pop method removes the topmost element from the stack by decreasing the 'top' pointer.
- π The top method displays the top element of the stack without removing it.
- π The stack class is initialized with a default maximum size of 100, and it ensures no data is added if the stack is full.
- π The main method tests the functionality of the stack by pushing values onto the stack, displaying the data, performing pop operations, and displaying the data again.
- π The pop method prevents removal from an empty stack and includes checks to ensure safe operations.
- π The display method prints elements in the order they were inserted, with the most recent element at the top of the stack.
- π After performing pop operations, the top data is updated accordingly, and the stack's current state is displayed to reflect these changes.
Q & A
What is the purpose of the stack class in this tutorial?
-The purpose of the stack class is to demonstrate how to implement a basic stack data structure, where elements are stored and retrieved in a Last-In-First-Out (LIFO) order.
How does the push method work in the stack implementation?
-The push method adds an integer to the stack. It stores the value at the position indicated by the 'top' index and then increments the 'top' index to point to the next available position.
What variables are initialized in the stack class and what do they represent?
-The variables initialized in the stack class are 'top' (indicates the current position in the stack), 'maxSize' (the maximum capacity of the stack), and 'data' (an array that stores the stack elements).
Why is the top variable initialized to zero?
-The 'top' variable is initialized to zero because it represents the first available position in the stack, which starts at the first index of the data array.
How does the display method show the stack's data?
-The display method iterates from the top of the stack to the bottom (from the last added item to the first) and prints each element in the stack, displaying them in LIFO order.
What happens when the pop method is called?
-The pop method removes the most recently added item from the stack, decreases the 'top' index, and effectively makes the last element in the stack no longer accessible.
How does the stack handle the maximum size limit?
-The stack class sets a maximum size (e.g., 100). If the stack exceeds this size, no additional elements can be pushed onto the stack unless space becomes available by popping elements.
What does the 'top' method do in the stack class?
-The 'top' method returns the current element at the top of the stack, i.e., the most recently added element, without removing it from the stack.
What is the role of the 'main' method in the stack class?
-The 'main' method is used to create a stack instance and test the stack methods by pushing data into the stack, displaying the stack's contents, and performing pop and top operations.
What happens when a pop operation is performed twice consecutively?
-When two pop operations are performed consecutively, two elements are removed from the stack, and the stack shrinks accordingly. After these operations, the top of the stack points to the next available element.
Outlines
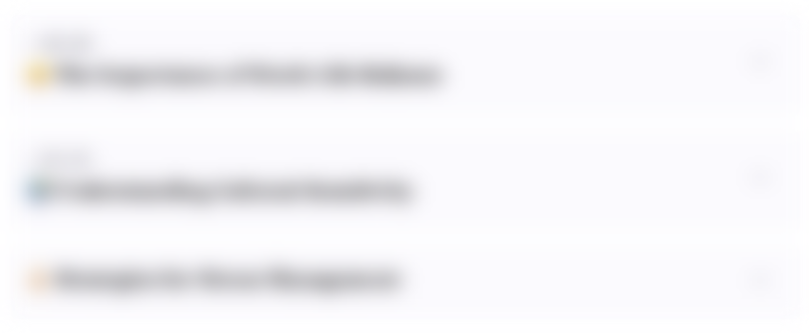
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
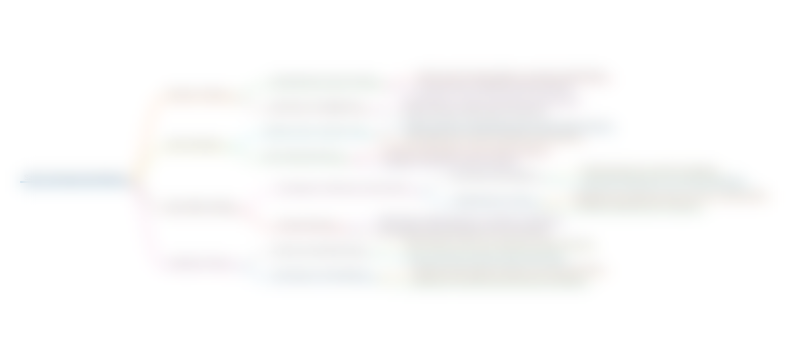
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
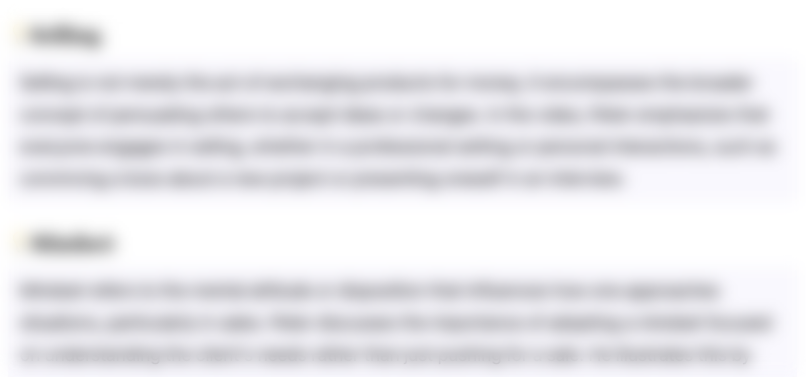
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
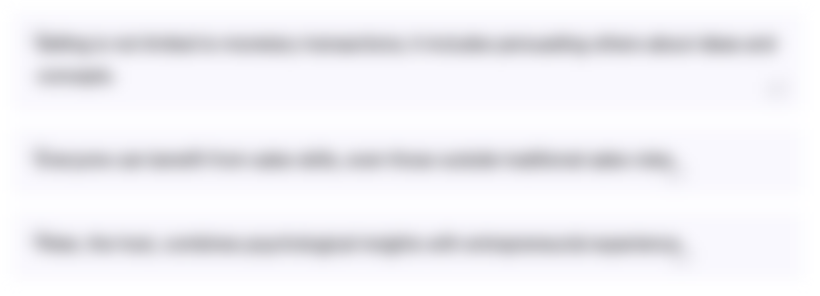
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
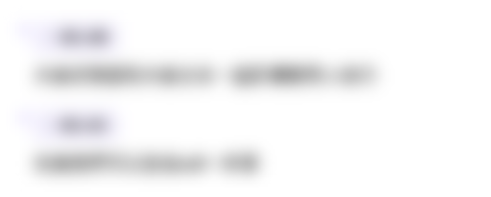
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)