02 - Expressions B - Python for Everybody Course
Summary
TLDRThis video script delves into the concept of expressions in programming, highlighting the use of operators and their precedence. It explains the importance of understanding data types and how they affect operations, showcasing Python's type system and the use of functions like `type`, `int`, and `float` for type conversion. The script also covers input and output functions, introduces the concept of comments, and presents a simple program for converting elevator floor numbers between US and European conventions, emphasizing the building blocks of programming such as variables, expressions, and user interaction.
Takeaways
- 📚 Expressions in programming are complex calculations done on the right-hand side of an assignment statement, utilizing operators similar to mathematical ones.
- 🔢 Basic operators in programming include addition (+), subtraction (-), multiplication (*), division (/), exponentiation (**), and modulo (%), chosen based on the limited characters available on keyboards from the 1960s and 1970s.
- 🎯 Operator precedence dictates the order of operations in expressions, with parentheses being the highest, followed by exponentiation, then multiplication and division (from left to right), and finally addition.
- 🤖 Python is intelligent in handling different data types, performing operations based on the type of operands involved, such as adding integers or concatenating strings.
- 🚫 Type errors can occur when attempting to perform operations on incompatible types, such as adding a string and an integer, which Python does not implicitly convert.
- 🔍 The `type` function in Python can be used to determine the type of a variable or constant, helping to understand and manage data types in a program.
- 🔄 Conversion functions like `int` and `float` allow for changing data types in Python, enabling operations between different types of numbers or between numbers and strings.
- 📉 Python 3 introduced more predictable behavior in division, always resulting in a floating-point number, unlike Python 2 which could truncate results in certain cases.
- 💬 The `input` function in Python is used to prompt the user for input, which is always returned as a string, requiring conversion if numerical operations are needed.
- 🏗️ A simple program example is provided, demonstrating how to convert floor numbers between European and U.S. numbering systems, illustrating the use of input, processing, and output in a program.
- 📝 Comments in Python, starting with a pound sign (#), allow developers to add explanatory text within the code that is ignored by the interpreter, aiding in readability and maintenance.
Q & A
What are expressions in programming?
-Expressions in programming are more complex calculations that can be performed on the right-hand side of an assignment statement, often using operators similar to mathematical operators.
Why do programming operators differ from mathematical operators?
-Programming operators differ because they must use characters available on the keyboard, especially from the 1960s and 1970s, when many programming languages were developed.
What symbol is used for multiplication in programming?
-The asterisk (*) is commonly used to represent multiplication in programming due to the absence of a times sign or a dot on the keyboard.
How is division represented in programming?
-Division is represented by a slash (/) in programming, as it cannot have two things overlaid like in mathematical notation.
What does the term 'modulo operator' refer to?
-The modulo operator refers to the remainder when one number is divided by another, also known as the modulo operation.
Why is the modulo operator useful in games?
-The modulo operator is useful in games for tasks such as picking a random number, like selecting a card at random, by using the modulo of a specific number.
What is operator precedence and why is it important?
-Operator precedence is the hierarchical order in which operators are evaluated in an expression. It's important for determining the correct evaluation of expressions without parentheses.
How does Python handle different data types in expressions?
-Python is smart about handling different data types, performing the appropriate operation based on the types of the variables or constants involved in the expression, such as concatenating strings or adding numbers.
What is the purpose of the 'type' function in Python?
-The 'type' function in Python is used to determine and return the data type of a variable or constant, helping programmers understand and manage data types in their code.
How does Python's handling of division differ between Python 2 and Python 3?
-In Python 3, division between two integers always produces a floating-point result, making it more predictable. In contrast, Python 2 had different behaviors for integer division, which could lead to unexpected results.
What is the role of comments in programming?
-Comments in programming are pieces of text that are ignored by the interpreter or compiler. They are used to explain the code, making it easier for others (or the original programmer at a later time) to understand.
What is the significance of the input function in Python?
-The input function in Python is used to pause the program and prompt the user to provide input. It's a fundamental part of handling user interaction within a program.
Can you provide an example of a simple Python program mentioned in the script?
-An example of a simple Python program mentioned is an 'Elevator Floor Conversion App' that takes a floor number from a European-style numbering system (starting from 0), adds one to convert it to a U.S.-style system (starting from 1), and then prints the result.
Outlines
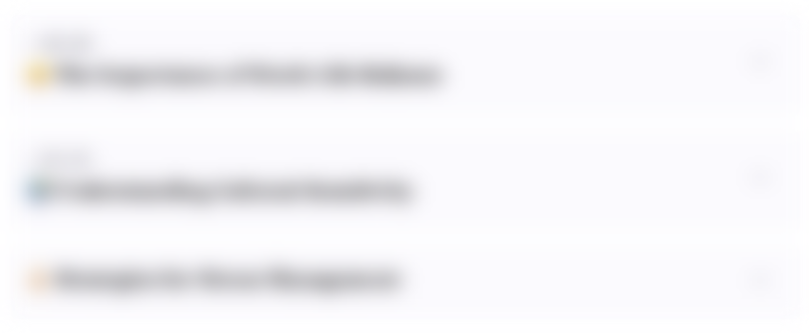
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
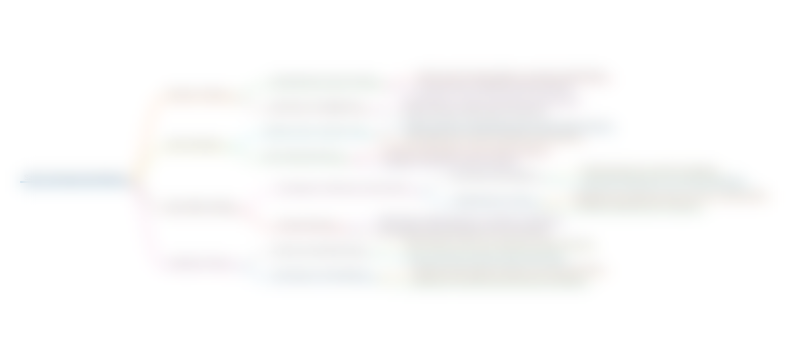
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
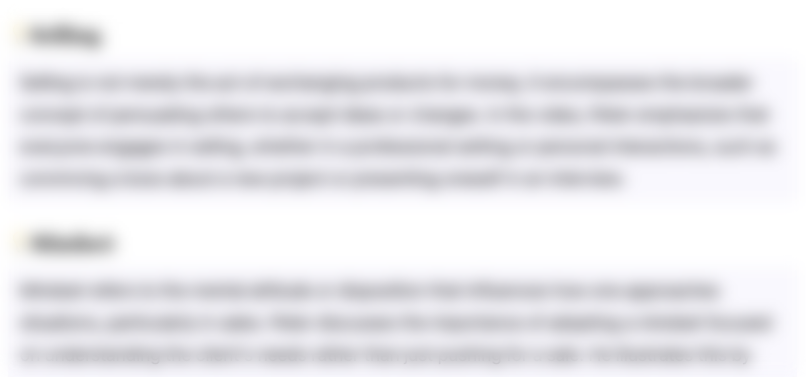
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
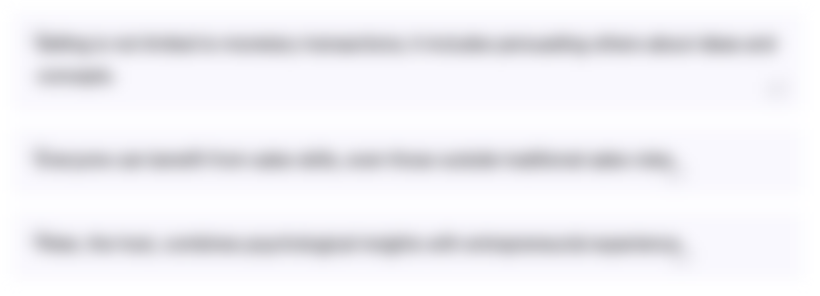
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
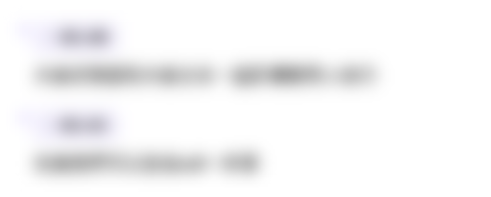
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)