COS 333: Chapter 7, Part 2
Summary
TLDRThis lecture delves into expressions and assignment statements, covering relational and boolean expressions, short-circuit evaluation, and various assignment statements. It explains operator coercion in languages like JavaScript and PHP, the importance of distinguishing between equality and assignment, and the use of compound and unary assignment operators. The lecture also touches on the nuances of assignment expressions in different programming languages, the potential pitfalls of short-circuit evaluation, and the concept of mixed mode assignments, highlighting the need for understanding type coercion in programming.
Takeaways
- π The lecture focuses on expressions and assignment statements, building upon the concepts introduced in the previous lecture.
- π It discusses relational and boolean expressions, explaining how they define relationships or test for true/false conditions between operands.
- π The script highlights the difference in behavior between languages like JavaScript, PHP, and C++/Java in terms of operator coercion during equality and inequality checks.
- π It emphasizes the use of '===' and '!==' in JavaScript and PHP for strict equality and inequality checks without type coercion.
- π The concept of short-circuit evaluation is introduced, explaining how it can prevent unnecessary evaluation of operands in expressions.
- π‘ Potential issues arising from the lack of short-circuit evaluation are presented, such as accessing invalid array indices.
- π The lecture covers various programming languages' approaches to short-circuit evaluation, with some languages providing options for programmers.
- π Assignment statements are detailed, including the different operators and syntax used across languages, and the potential for ambiguity with the '=' operator.
- ππ Compound and unary assignment operators are explained, showing shorthand methods for incrementing or decrementing values.
- π The script touches on the precedence and associativity of unary assignment operators, which is important for understanding expression evaluation.
- ππ The use of assignment statements as expressions in some languages, like C, is highlighted, noting the potential for errors and side effects.
Q & A
What topics are covered in the second lecture on chapter seven?
-The second lecture on chapter seven covers relational and boolean expressions, short circuit evaluation, assignment statements, and mixed mode assignment.
What are relational expressions and what types of operators are included in this category?
-Relational expressions define some sort of relationship between two operands. They include equality and inequality expressions, as well as operators such as less than (<), less than or equal to (<=), greater than (>), and greater than or equal to (>=).
How does operator coercion affect equality and inequality checks in JavaScript and PHP?
-In JavaScript and PHP, operator coercion allows for the comparison of operands of different types by converting one operand to the type of the other, enabling the comparison to take place.
What is the difference between using triple equals (===) and double equals (==) in JavaScript for equality checks?
-The triple equals (===) performs an equality check without type coercion, comparing both value and type, while the double equals (==) performs the check with type coercion, meaning the operands may be converted to comparable types before comparison.
What is short circuit evaluation and why is it used?
-Short circuit evaluation allows an expression to produce a result without necessarily evaluating all operands and operators within the expression. It is used to prevent unnecessary evaluations, especially when the outcome of an expression can be determined without evaluating all parts.
What potential problems can arise if short-circuit evaluation is not supported in a programming language?
-If short-circuit evaluation is not supported, it may lead to runtime errors, exceptions, or invalid operations when attempting to evaluate operands that should have been skipped, potentially causing program termination or unexpected behavior.
How do different programming languages handle short circuit evaluation?
-Languages like C, C++, and Java have standard boolean operators that are short-circuit evaluated, while bitwise boolean operators are not. Languages such as Ruby, Perl, ML, F#, and Python have all of their logical operators short-circuit evaluated.
What is a conditional target in the context of assignment statements?
-A conditional target is a feature supported by some programming languages like Perl, where an assignment can be made to one of multiple targets based on a condition, using a ternary-like operator syntax.
What is the general syntax for an assignment statement and what is the purpose of the assignment operator?
-The general syntax for an assignment statement involves an assignment operator, a left operand (target variable), and a right operand (expression). The assignment operator is used to store the value of the expression in the target variable.
What are the problems associated with using assignment as an expression?
-Using assignment as an expression can reduce error detection capabilities, as a mistaken assignment operator may be used instead of a comparison operator. Additionally, it can cause side effects if the assignment changes the value of a variable that should not be altered in that context.
How do mixed mode assignments differ between languages like Java and C# compared to Fortran, C, and Perl?
-In Java and C#, only widening coercions are allowed in assignments, which is a safer approach as it prevents potential data loss due to narrowing coercions. In contrast, Fortran, C, and Perl allow any coercion, including narrowing, which can lead to data loss or unexpected results.
Why does Ada perform fewer coercions than most other high-level programming languages in assignments?
-Ada performs fewer coercions to ensure type safety and prevent potential data loss or unexpected behavior. It does not perform any assignment coercions, requiring explicit conversions when necessary.
Outlines
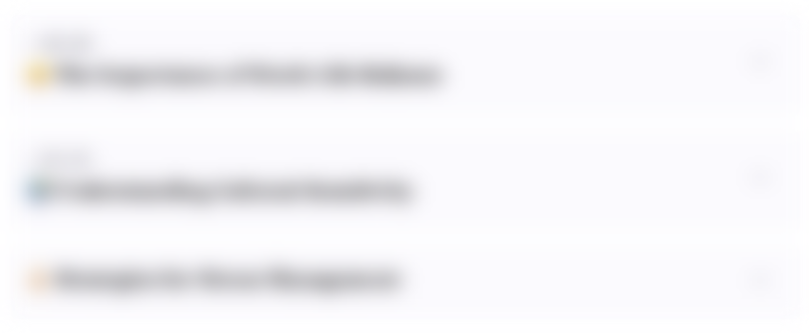
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
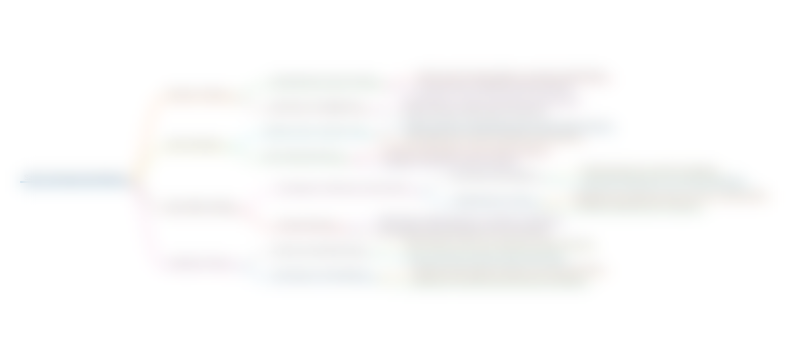
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
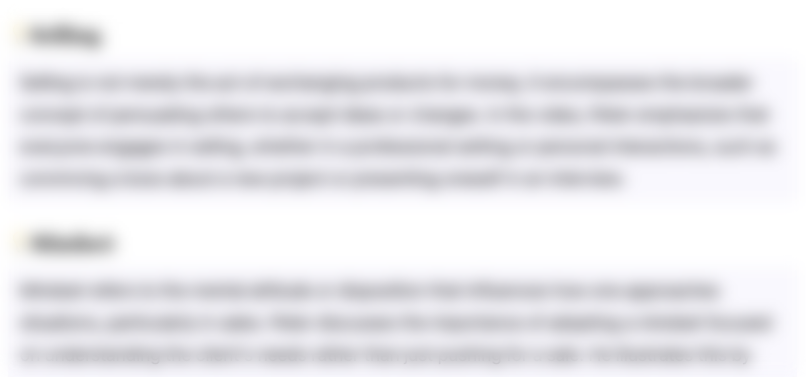
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
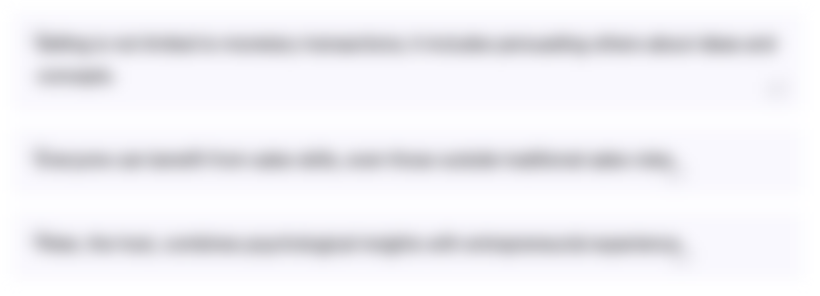
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
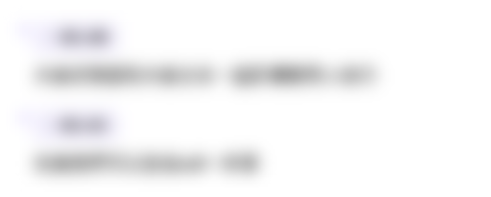
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)