Operators & Operands | Godot GDScript Tutorial | Ep 02
Summary
TLDRThis script delves into the fundamentals of programming, focusing on operands, operators, and their roles in manipulating values within a program. It explains assignment operators, including various shortcuts, and discusses the significance of comparison operators for evaluating expressions. The script further explores logical operators, illustrating how 'and', 'or', and 'not' evaluate truth values, emphasizing their importance in debugging and decision-making in code.
Takeaways
- π Operands in programming are numerical, text, boolean values, or object values that can be manipulated.
- π Operators are symbols used to manipulate and check operand values, including addition, subtraction, multiplication, division, and remainder.
- π Assignment operators assign values to variables, with the simple assignment operator being the equal sign.
- π Shortcut assignment operators combine an operation and assignment, such as 'x += y' which is equivalent to 'x = x + y'.
- β The remainder assignment operator provides the remainder of division, which can be useful for specific applications.
- π Comparison operators compare operands and return a boolean value based on the comparison, such as '==' for equality and '!=' for inequality.
- βοΈ Logical operators in GDScript include AND (&&), OR (||), and NOT (!), used to evaluate boolean expressions.
- π The AND operator returns false if any operand can be converted to false, stopping evaluation from left to right.
- π The OR operator returns true if any operand can be converted to true, also stopping evaluation from left to right.
- π The NOT operator inverts the truth value of a single operand, returning true if the operand is false and vice versa.
- π§ Understanding the conversion of values to boolean and the order of evaluation by logical operators is crucial for effective debugging and code logic.
Q & A
What are operands in the context of programming?
-Operands are numerical values, text, and boolean values that a program can manipulate.
What is the role of operators in programming?
-Operators are symbols used to manipulate and check operand values, such as addition, subtraction, multiplication, and division.
What is the purpose of assignment operators in programming?
-Assignment operators assign the value of the right operand to the left operand, with the simple assignment operator being the equal sign.
Can you provide an example of an assignment operator in GDScript?
-An example is 'x += y', which is a shorthand for 'x = x + y', and assigns the sum of x and y to x.
What is the function of the remainder assignment operator?
-The remainder assignment operator gives the remainder of the division of the right operand by the left operand.
How does a comparison operator work in programming?
-A comparison operator compares its operands and returns a boolean value based on whether the comparison is true.
What is the difference between the equal and not equal operators?
-The equal operator checks if two operands are the same, while the not equal operator checks if they are different.
What does the greater than operator symbolize in a comparison?
-The greater than operator symbolizes that the left operand is larger than the right operand.
How does the logical AND operator determine its result?
-The logical AND operator returns true only if all operands can be converted to true; if any can be converted to false, it returns false.
How does the logical OR operator differ from the AND operator?
-The logical OR operator returns true if at least one operand can be converted to true, whereas the AND operator requires all operands to be true.
What is the purpose of the NOT operator in logical expressions?
-The NOT operator is used to invert the truth value of a single operand, returning true if the operand can be converted to false, and vice versa.
Can you explain the concept of truthy and falsy values in programming?
-Truthy values are values that are considered true in a boolean context, like non-zero numbers or non-empty strings. Falsy values are those considered false, such as 0, false, or empty strings.
Outlines
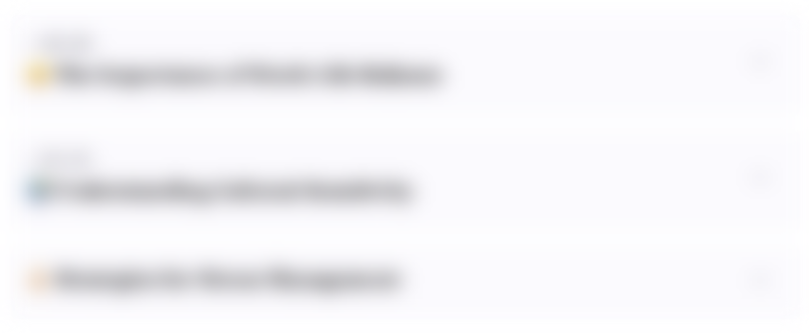
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
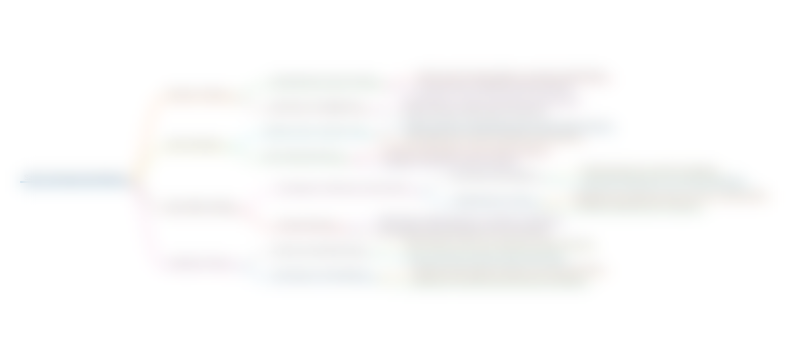
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
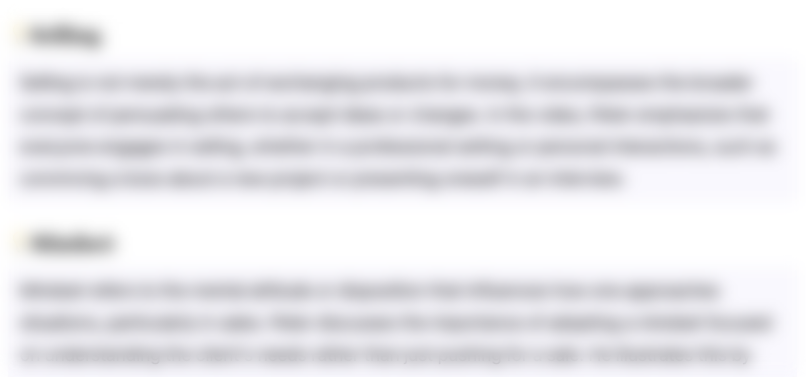
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
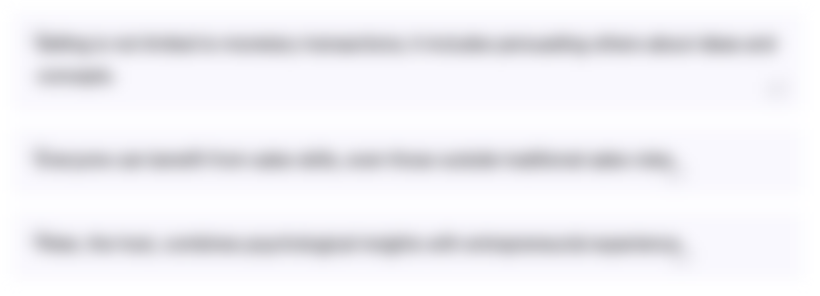
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
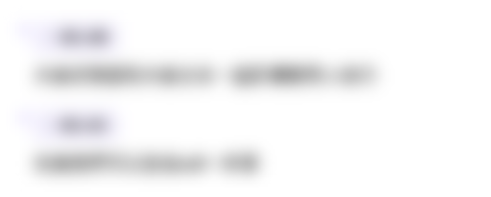
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

C_15 Operators in C - Part 3 | C Programming Tutorials

Kurikulum Merdeka Rangkuman Informatika Kelas 9 Bab 2
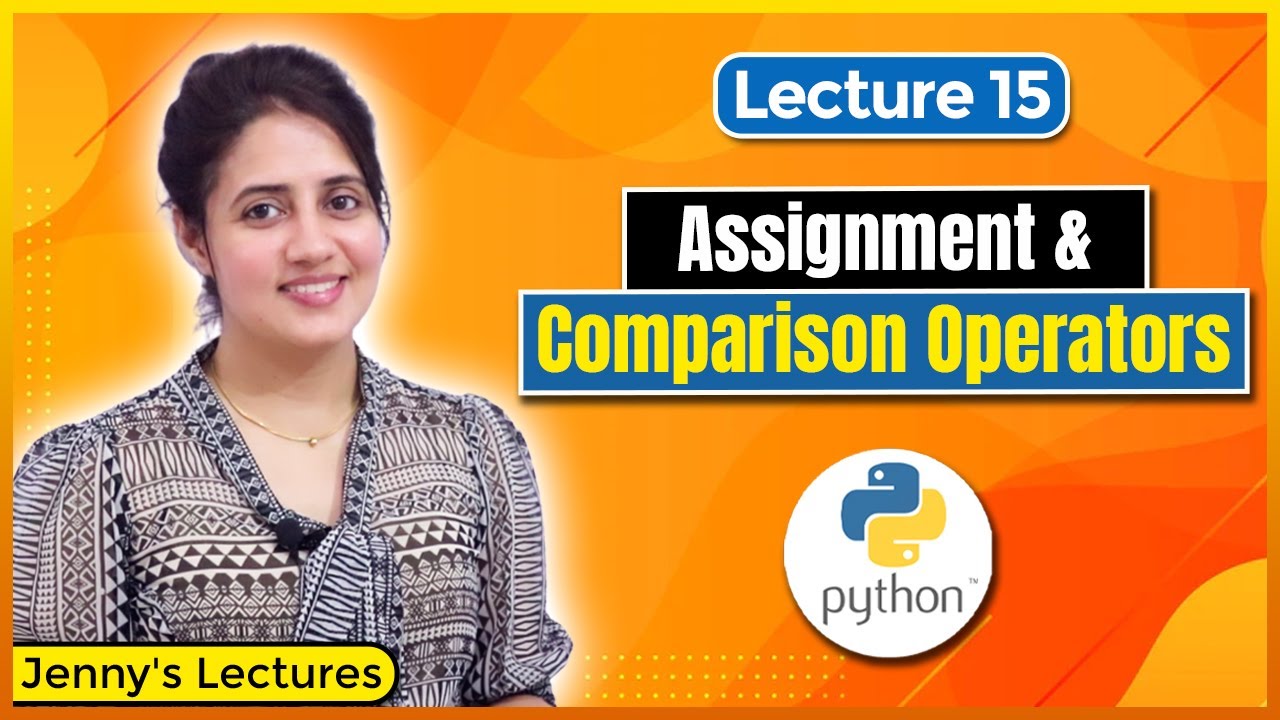
P_15 Operators in Python | Assignment and Comparison Operators | Python Tutorials for Beginners
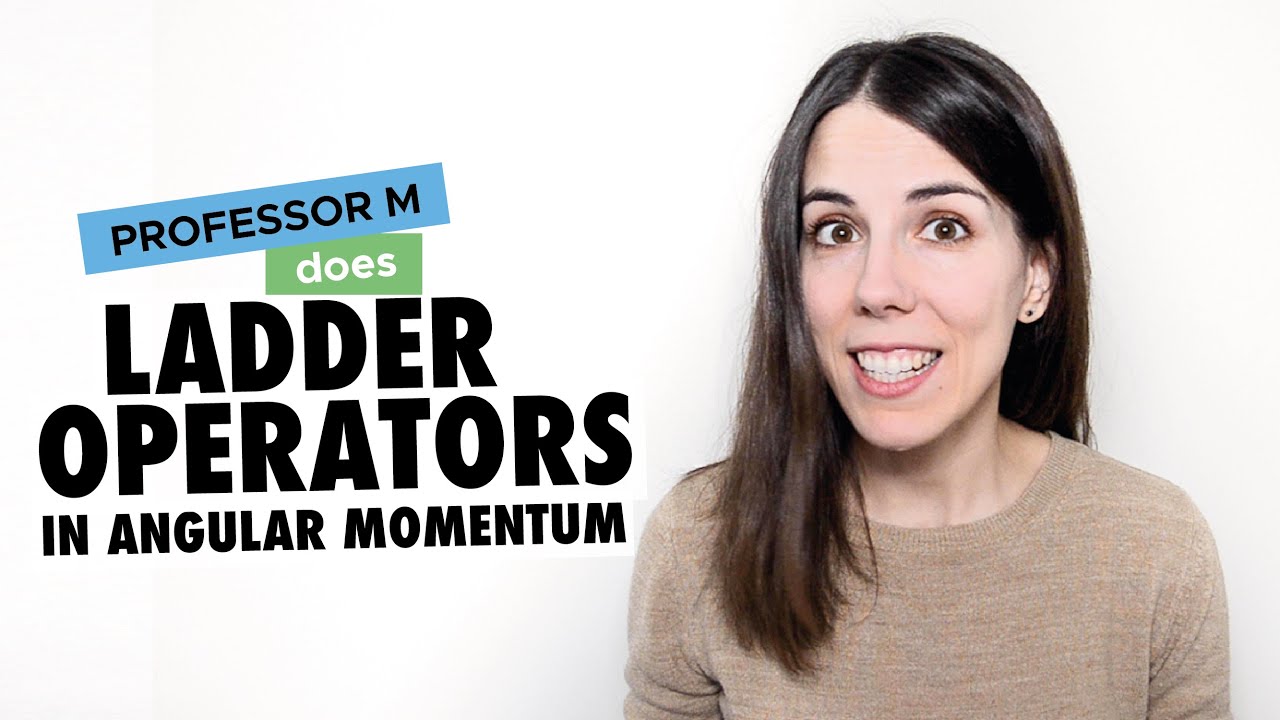
Ladder operators in angular momentum
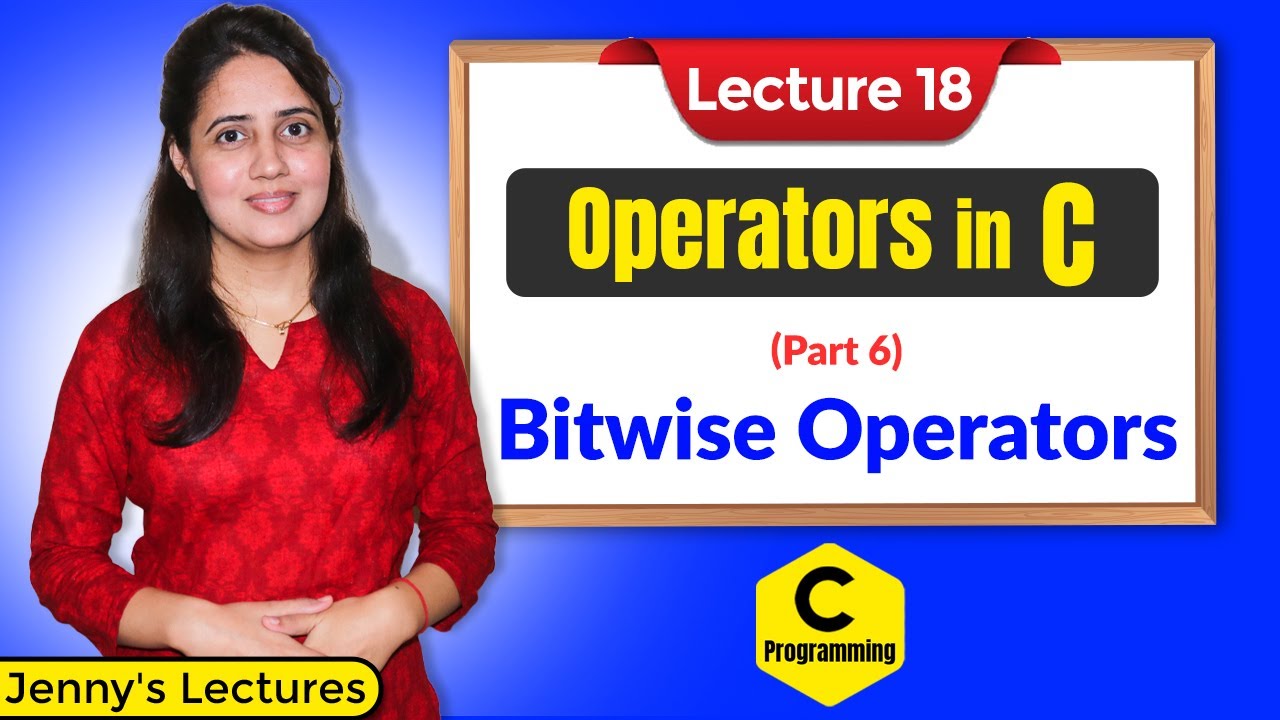
C_18 Operators in C - Part 6 | Bitwise Operators | C Programming Tutorials

Excel VBA Programming - Variables and Data Types | 2 - Variable Declarations and Assignments
5.0 / 5 (0 votes)