02 - Expressions A - Python for Everybody Course
Summary
TLDRThis video script delves into the fundamental components of Python programming, including constants, reserved words, and variables. It explains how constants are unchanging values used for specific calculations, while variables are memory allocations with names that can store and change values. The script emphasizes the importance of understanding assignment statements and choosing meaningful variable names to enhance code readability. It also clarifies that Python does not interpret the meaning behind variable names, highlighting the need for clear and descriptive naming conventions to aid programmers in understanding the code's purpose.
Takeaways
- ๐ Constants in Python are unchanging values like numbers or strings that are used as starting points for calculations or conditions.
- ๐ Reserved words in Python have special meanings and are used for specific programming constructs, such as 'if' for conditional execution.
- ๐พ Variables are used to allocate memory and store data. They are named and can hold different values over time, hence the term 'variable'.
- ๐ The assignment statement in Python is like an arrow, indicating where to store the value, and it evaluates the right-hand side before assigning it to the left-hand side.
- ๐ Variable names in Python can start with a letter or underscore, and can contain letters, numbers, and underscores (except as the first character). They are case-sensitive.
- ๐ซ Avoid using underscores as the first character of a variable name, as they are often reserved for Python's own variables.
- ๐ Choosing descriptive variable names can improve code readability, but remember that Python does not understand the meaning behind the names.
- ๐ Variables can be reassigned new values, which overwrites the old values, demonstrating the 'variable' nature of variables.
- ๐ข Constants, reserved words, and variables are fundamental building blocks of Python programming.
- ๐ The script emphasizes the importance of understanding assignment statements and the role of variables in Python programming.
- ๐ค The speaker warns against attributing too much intelligence to Python based on mnemonic variable names, as Python is indifferent to the meaning of the names.
Q & A
What are constants in Python and why are they important?
-Constants in Python are values that do not change, such as numbers or strings. They are important because they provide a way to start calculations and can be used to set conditions, like checking if the number of hours worked is greater than 40.
What is the role of reserved words in Python programming?
-Reserved words in Python have special meanings and are used by Python to implement conditional execution and other language features. They are essential for the language to function properly.
How does Python allocate memory for variables?
-Python allocates memory for variables through the assignment statement. It assigns a value to a variable by finding a piece of memory, labeling it with the variable name, and then storing the value in that memory.
What is the significance of the assignment statement in Python?
-The assignment statement is significant because it assigns a value to a variable. It is important to think of it as having an arrow, indicating the direction of the assignment from the value to the variable.
Can variables in Python hold more than one value?
-Yes, in Python, variables can hold more than one value, especially when using collections like lists or dictionaries, which will be covered in later chapters.
What are the rules for naming variables in Python?
-Variable names in Python can start with a letter or an underscore, but not with a number or special character. They can contain letters, numbers, and underscores after the first character. Variable names are case-sensitive, and using meaningful names is recommended for better readability.
Why is it not recommended to start variable names with an underscore?
-Starting variable names with an underscore is not recommended because it is often reserved for variables that communicate with Python itself. Using underscores for normal variables can lead to confusion and is not a common practice.
What is the difference between using short, meaningless variable names and using meaningful or mnemonic variable names?
-Short, meaningless variable names might be convenient and easy to type but can make the code less readable and harder to understand. Meaningful or mnemonic variable names improve code readability and help others understand the purpose of the variable.
How does Python handle the evaluation of expressions in an assignment statement?
-Python evaluates the entire right-hand side of an assignment statement before changing the left-hand side. This means it calculates the expression first and then assigns the result to the variable on the left.
Why is it important to understand the 'arrow nature' of the assignment statement in Python?
-Understanding the 'arrow nature' of the assignment statement is important because it clarifies the direction of value assignment and helps prevent confusion when the same variable appears on both sides of the statement.
What is the purpose of the print function in Python?
-The print function in Python is used to output data to the console. It is a built-in function that takes the data to be printed as an argument within parentheses.
Outlines
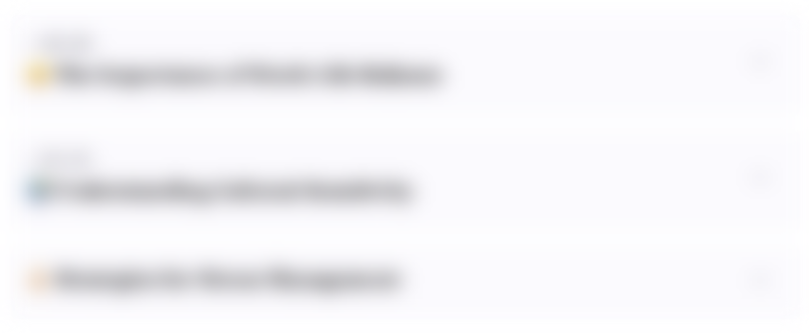
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
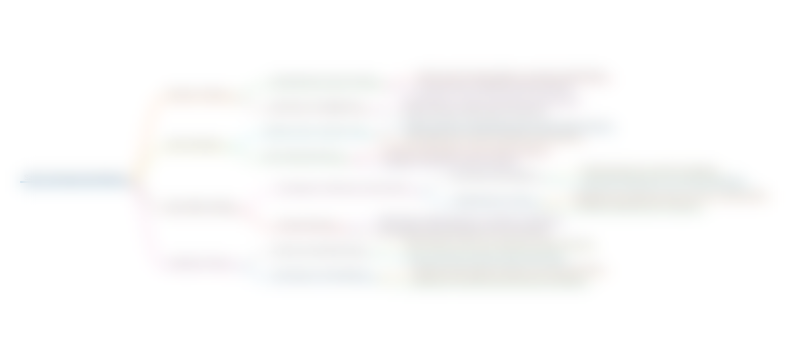
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
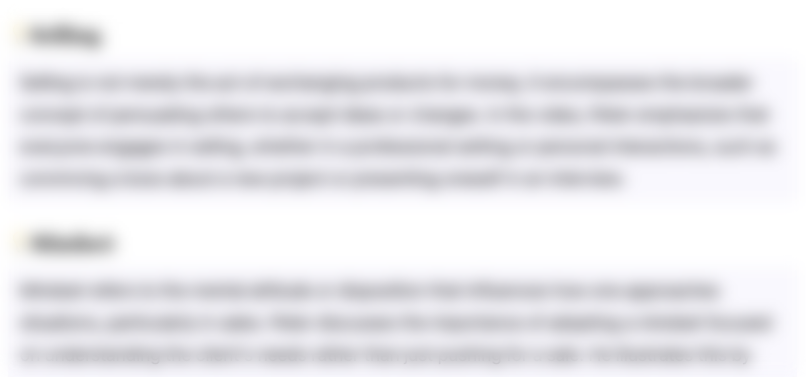
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
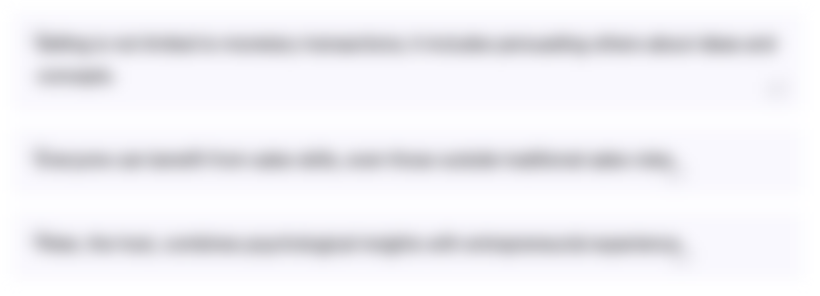
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
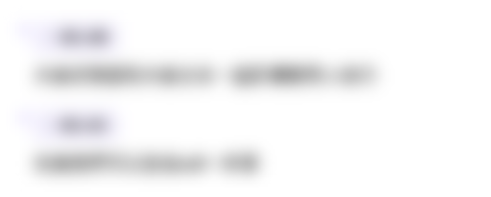
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
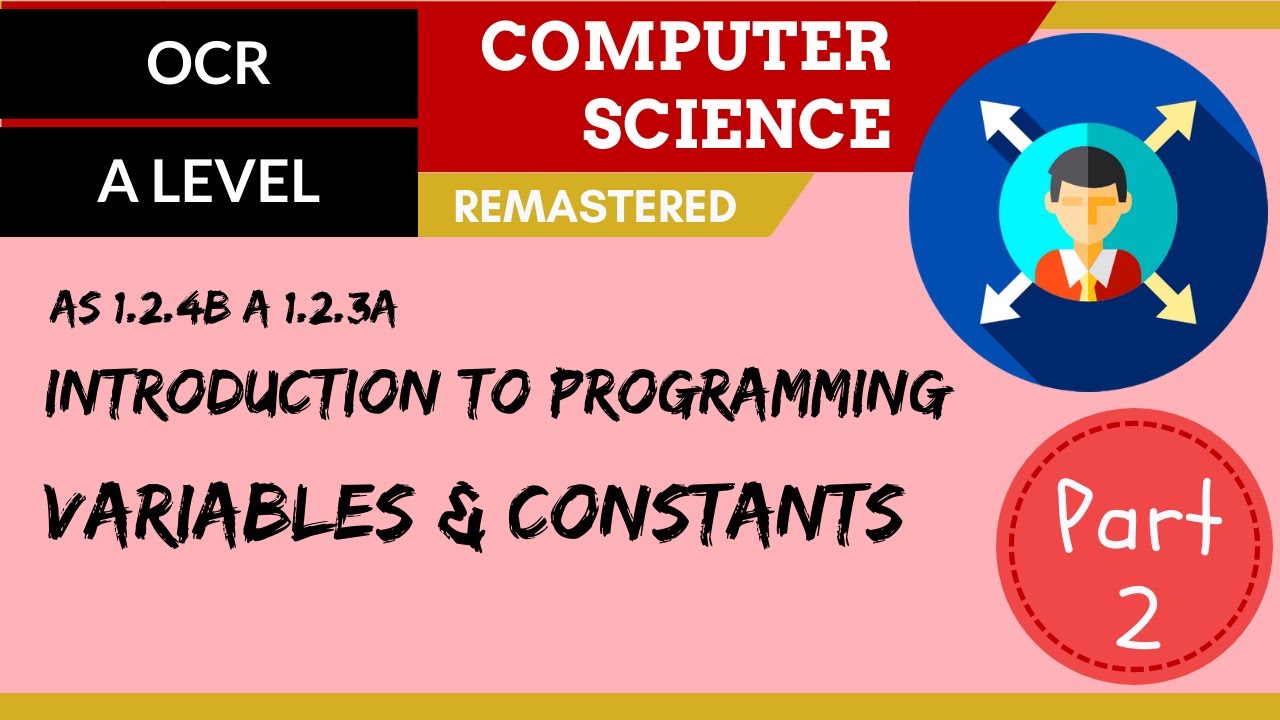
41. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 2 variables & constants
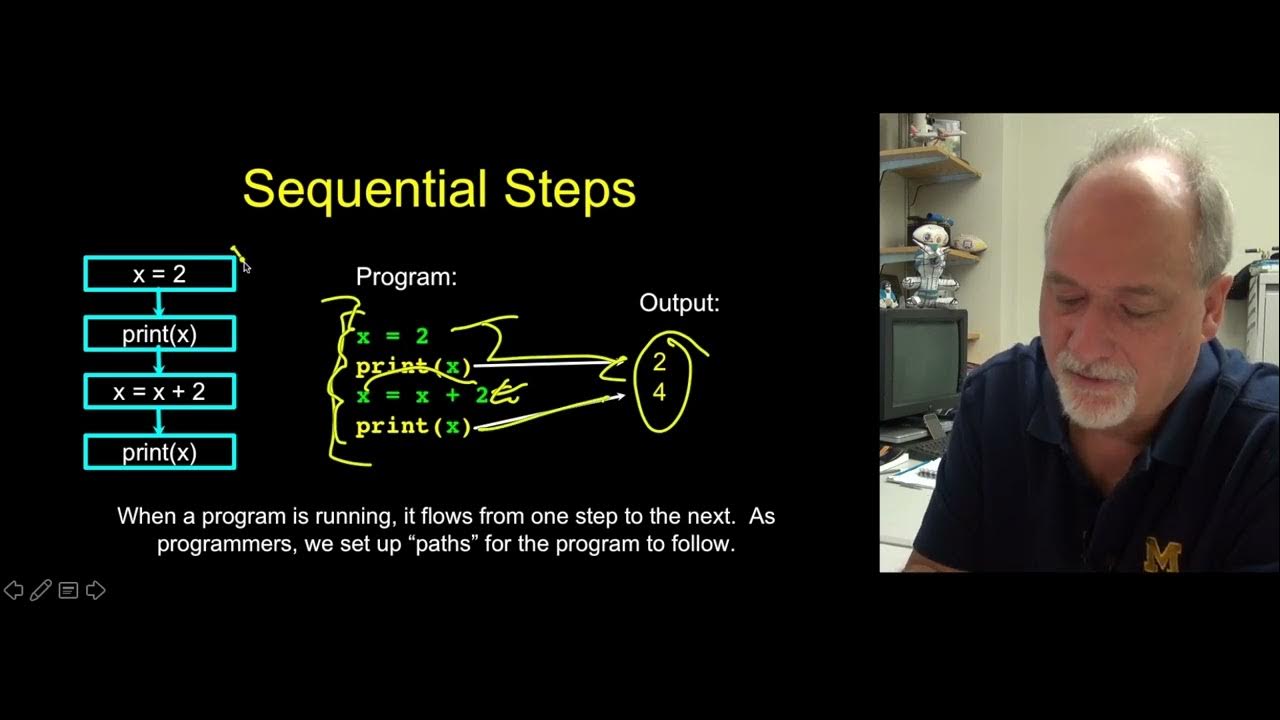
PY4E - Introduction (Chapter 1 Part 4)

#2 Tipe Data, Variabel, dan Operator
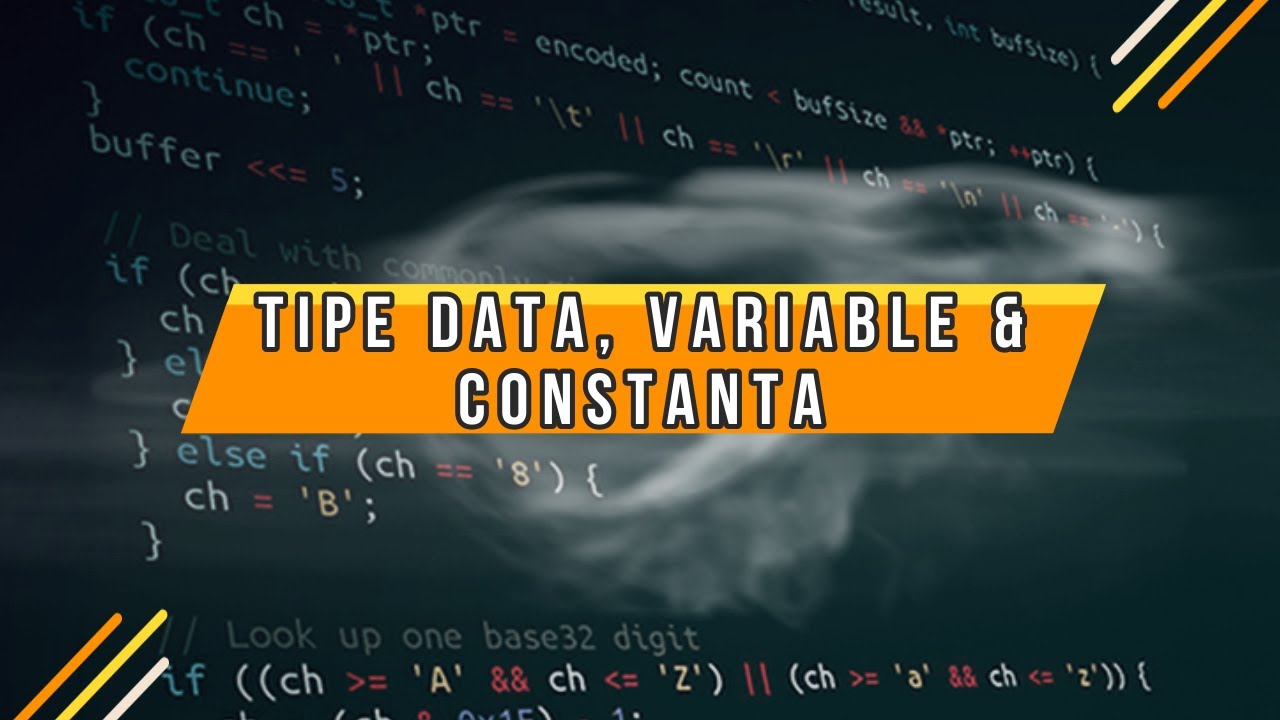
Pemrograman Dasar - Tipe Data, Variable & Constanta
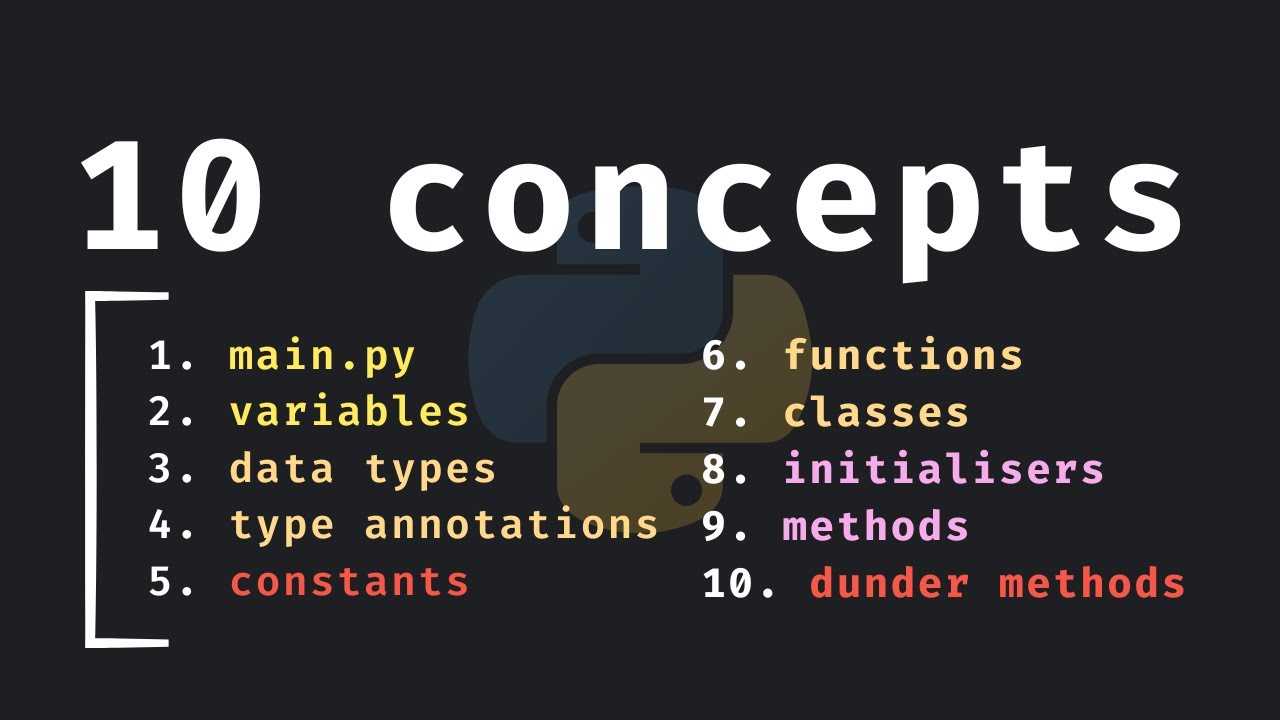
10 Important Python Concepts In 20 Minutes
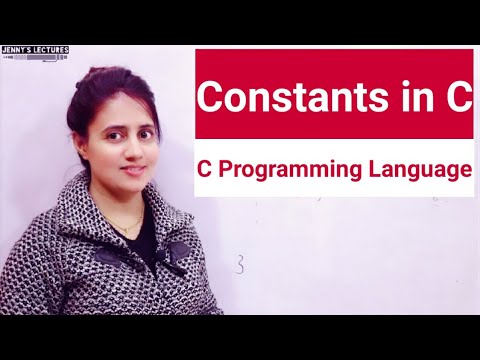
C_07 Constants in C | Types of Constants | Programming in C
5.0 / 5 (0 votes)