CS50P - Lecture 3 - Exceptions
Summary
TLDRIn this CS50 lecture, David Malan introduces the concept of exceptions in Python, explaining how they're used to handle errors in code. He demonstrates syntax errors with a simple 'hello world' example and runtime errors with user input, showing how to use try, except, and else blocks for error handling. Malan also covers best practices for defensive programming, including the use of loops for repeated user input and the importance of minimizing the code executed within a try block to only what might fail. He concludes by abstracting the error handling into a reusable 'get_int' function, illustrating the Pythonic approach to exceptions.
Takeaways
- 📝 David Malan introduces the concept of exceptions in Python, explaining that they refer to problems in code that need to be resolved by the programmer.
- 🔍 Exceptions are categorized into syntax errors, which are mistakes in the code's structure that must be fixed before the program can run, and runtime errors, which occur during program execution and may require defensive programming to handle.
- 💬 The script demonstrates a syntax error through an example of an unterminated string literal, highlighting the importance of correct syntax for program execution.
- 🔑 The 'try' and 'except' keywords in Python are introduced as a means to handle exceptions, allowing programmers to write code that can gracefully manage errors.
- 🔄 The 'else' block in a try-except statement is explained as a way to execute code when the try block does not raise an exception, ensuring that certain code runs only if no errors occur.
- 🔧 The 'pass' keyword is shown as a way to explicitly do nothing when an exception is caught, allowing the program to continue running without handling the caught error.
- 🔀 The use of loops, specifically 'while True', is introduced to repeatedly prompt the user for input until a valid response is given, demonstrating the use of control flow in error handling.
- 🔑 The importance of proper variable scope and the potential for name errors due to variables not being defined when expected is discussed, with examples provided.
- 🛠️ The script showcases the process of refactoring code to improve its design and functionality, such as creating a 'get_int' function to abstract the process of getting an integer from the user.
- 📚 The concept of creating reusable and maintainable code is emphasized through the refinement of the 'get_int' function, which is improved to accept a prompt parameter, making it more versatile.
- 📉 The script concludes with a discussion on the inevitability of errors in programming and the importance of handling them effectively using Python's exception handling mechanisms.
Q & A
What are exceptions in programming?
-Exceptions in programming refer to problems or errors that occur in your code. They signify that something has gone wrong and ideally need to be addressed or solved by the programmer.
What is a syntax error?
-A syntax error is a type of error related to the structure of the code. It occurs when the code is not written correctly according to the programming language's rules, such as missing quotation marks or incorrect use of symbols.
How does the Python interpreter handle syntax errors?
-When a syntax error occurs, the Python interpreter stops executing the code and displays an error message indicating the nature of the error, such as 'unterminated string literal', and the location in the code where the error was found.
What is the purpose of the 'try' and 'except' keywords in Python?
-The 'try' and 'except' keywords in Python are used for error handling. The 'try' block allows you to test a block of code for errors, and the 'except' block lets you handle the error if one occurs, preventing the program from crashing and allowing for a graceful response to the error.
Why is it important to handle runtime errors in Python?
-Runtime errors occur during the execution of the program and can be unpredictable. Handling these errors is crucial because they can cause the program to crash or behave unexpectedly. Proper error handling allows the program to respond gracefully to unexpected situations, such as invalid user input.
What is a value error in Python?
-A value error in Python occurs when a function receives an argument that has the right type but an inappropriate value. For example, trying to convert a non-numeric string to an integer using the 'int' function would raise a value error.
How can you prompt a user for input and handle potential errors in Python?
-You can use the 'input' function to prompt a user for input. To handle potential errors, such as the user entering non-integer values when an integer is expected, you can use a 'try-except' block to catch and handle the error, such as by displaying a message and prompting the user again.
What is the purpose of the 'else' clause in a 'try-except' block?
-The 'else' clause in a 'try-except' block is executed if the code in the 'try' block does not raise an exception. It allows you to specify code that should run only if the 'try' block completes successfully without any errors.
What is a name error in Python?
-A name error in Python occurs when a variable is referenced before it has been assigned a value or is not defined in the current scope. This can happen if the variable is misspelled or if the code that defines the variable has not been reached during execution.
How can you create a reusable function in Python to handle user input and error checking?
-You can create a reusable function by defining a function that takes parameters, such as a prompt message, and uses these parameters to prompt the user and validate the input. The function can return the validated input or handle errors internally, making it versatile for different use cases.
What is the purpose of the 'pass' statement in Python?
-The 'pass' statement in Python is a null operation; nothing happens when it is executed. It can be used as a placeholder in a 'try-except' block to indicate that an exception has been caught but no action is to be taken, effectively ignoring the exception.
Outlines
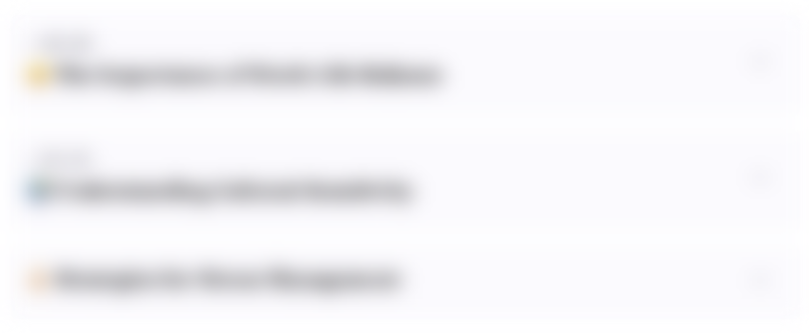
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
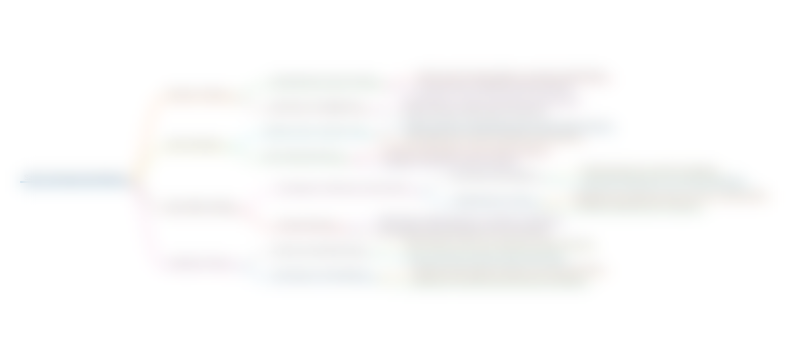
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
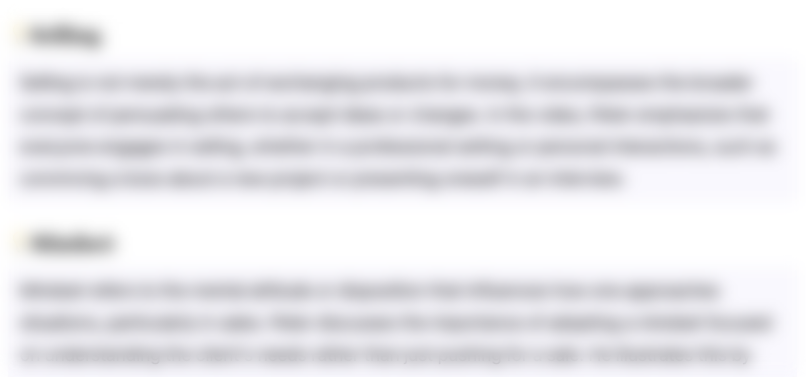
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
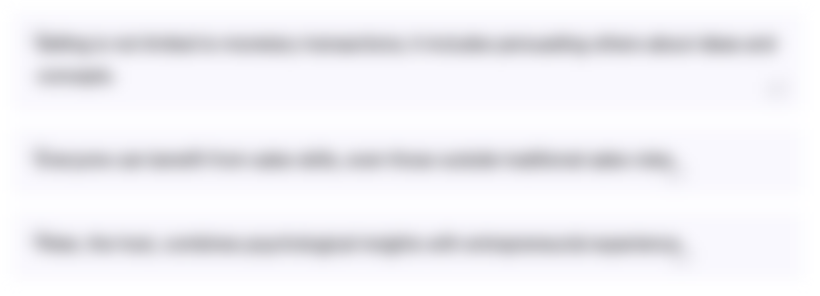
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
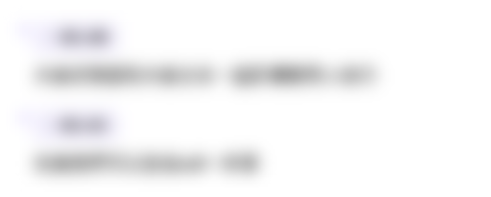
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
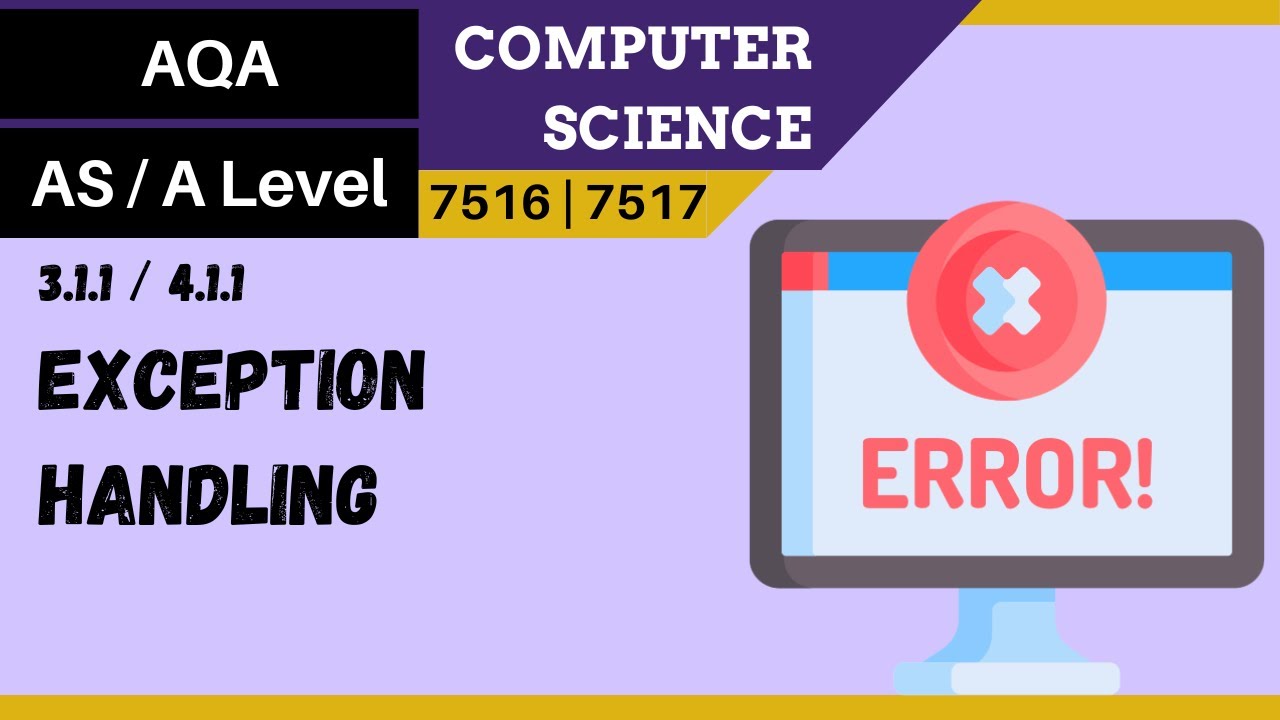
7. AQA A Level (7516-7517) SLR2 - 4.1.1 Exception handling

Eloquent Javascript: Error Propagation
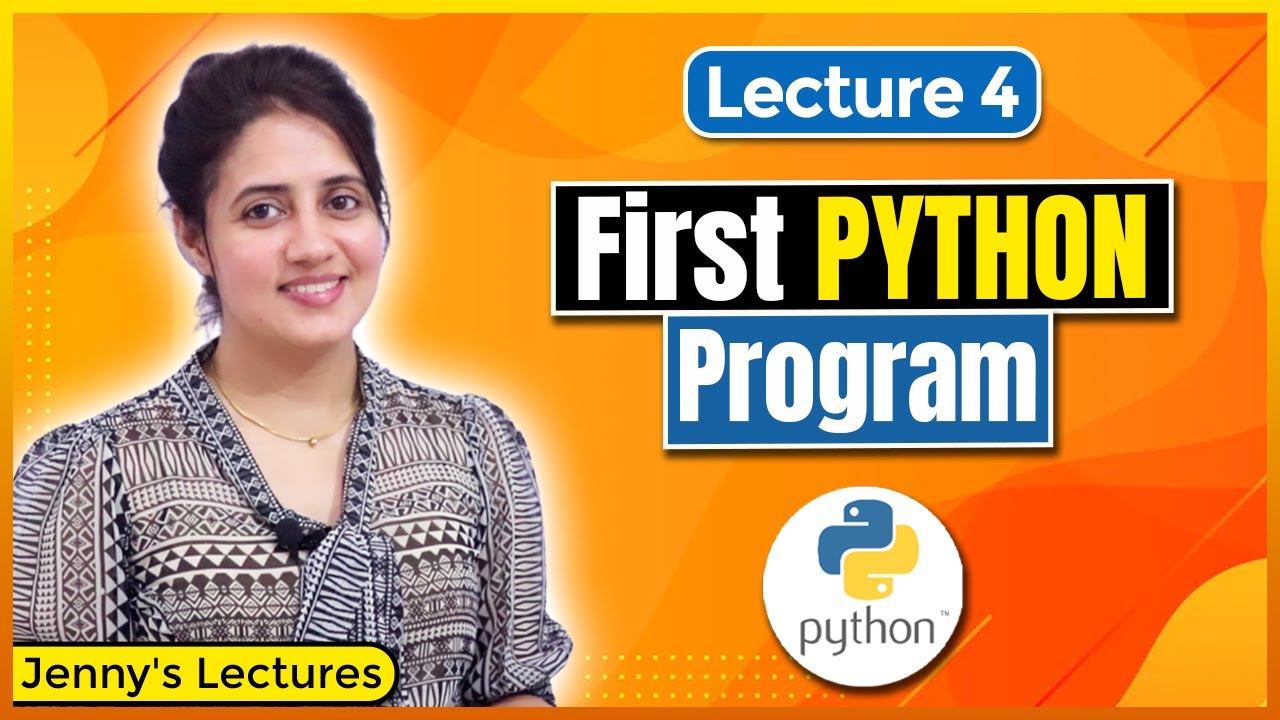
Writing First Python Program | Printing to Console in Python | Python Tutorials for Beginners #lec4

Handling Errors | Lecture 144 | React.JS 🔥
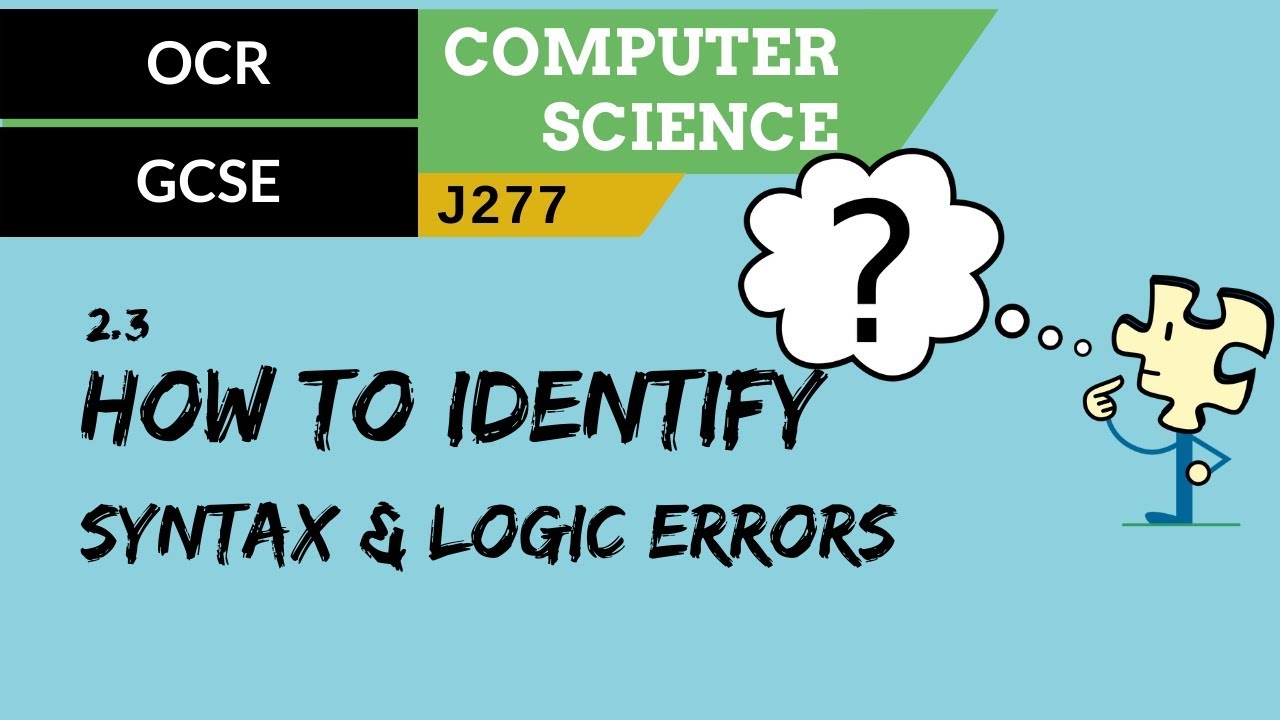
78. OCR GCSE (J277) 2.3 How to identify syntax and logic errors

CS50P - Introduction
5.0 / 5 (0 votes)