Handling Errors | Lecture 144 | React.JS π₯
Summary
TLDRThe video script focuses on error handling in web applications, particularly when dealing with asynchronous data fetching. It emphasizes the importance of being prepared for potential issues such as loss of internet connection or API errors. The tutorial demonstrates how to simulate and handle these scenarios by using try-catch blocks in JavaScript, updating application states to reflect errors, and displaying user-friendly error messages. It also introduces a state variable to manage error messages and conditional rendering to ensure a smooth user experience, even when data fetching fails.
Takeaways
- π Always assume errors can occur when dealing with asynchronous data in web applications.
- π» Simulate network issues like loss of internet connection to test error handling.
- πΆ Check the network tab to ensure the application behaves correctly under slow or offline conditions.
- π Use try-catch blocks to handle errors manually, as fetch does not inherently react to errors.
- π Check the response object's `ok` property to determine if the response is successful or not.
- π Throw new errors when the response is not okay to handle specific error cases.
- π Use a finally block to ensure certain code is executed regardless of whether an error occurred.
- π Maintain a separate state variable to track and display error messages.
- π Log error messages to the console for debugging purposes.
- π¨ Create a simple presentational component to display error messages with appropriate styling.
- π Ensure that the application's loading, error, and data display states are mutually exclusive.
Q & A
Why is it important to handle errors during data fetching in web applications?
-It is important to handle errors to provide a better user experience by informing users of issues, such as a lost internet connection, and to avoid leaving the application in a perpetual loading state.
How can you simulate a lost internet connection in a web application for testing purposes?
-You can simulate a lost internet connection by using the network tab in the browser's developer tools to set the connection to 'offline' while data is loading.
What does the 'response.ok' property signify in the context of fetch requests in JavaScript?
-The 'response.ok' property indicates whether the response was successful (status in the range 200-299) or not, helping to identify request failures.
What should you do in JavaScript code if the 'response.ok' property is false?
-If 'response.ok' is false, you should throw a new error to indicate that something went wrong with the fetch request.
What is the purpose of using a try-catch block in JavaScript?
-A try-catch block is used to catch errors in a section of code, allowing developers to handle exceptions and prevent the application from crashing.
Why is conditional rendering used in web applications?
-Conditional rendering is used to display different components or elements based on certain conditions, such as loading states or the presence of errors, enhancing user experience.
How can you manage the loading state in a React application to handle asynchronous data fetching?
-In a React application, you manage the loading state by using state variables to indicate when data is being fetched and then updating these variables appropriately to reflect when loading is complete or an error occurs.
What is the significance of the 'finally' block in error handling in JavaScript?
-The 'finally' block is executed after the try and catch blocks, regardless of the result, allowing for cleanup actions or resetting states like the loading state, ensuring code runs even after an error.
How can the absence of data from an API be handled in a web application?
-The absence of data can be handled by checking the received data and throwing an error or displaying a specific message if the expected data is undefined or does not meet required conditions.
Why is it important to handle different types of errors separately in web applications?
-Handling different types of errors separately allows for more tailored user feedback and specific troubleshooting steps, improving the overall user experience and application reliability.
Outlines
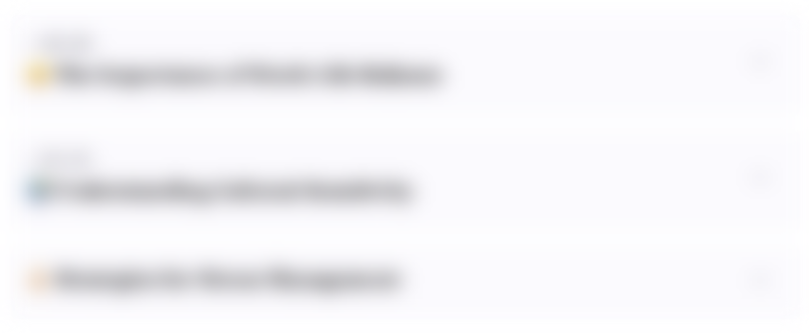
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
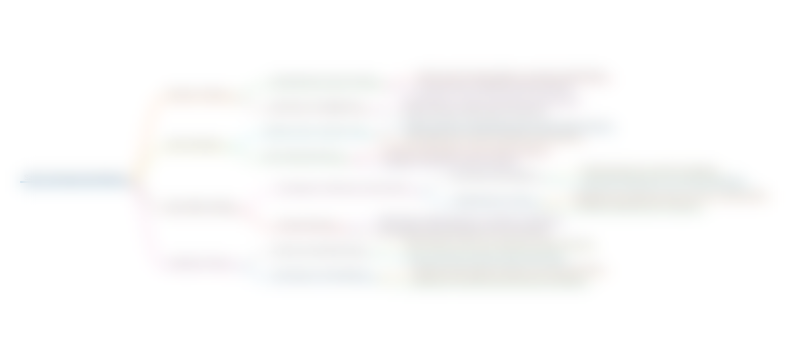
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
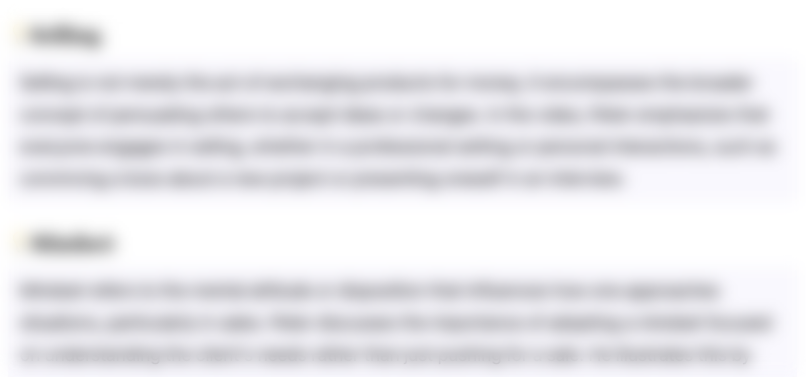
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
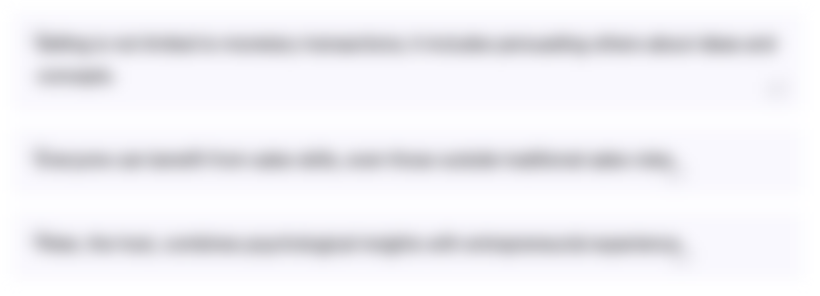
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
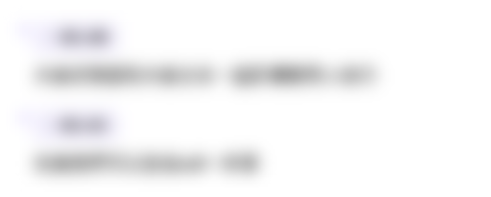
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)