Streams
Summary
TLDRThe video script delves into the concept of iterators and streams in programming, particularly in Java. It explains how iterators sequentially access elements in a collection, while streams offer a more declarative approach, allowing for parallel processing and lazy evaluation. The script illustrates stream operations like filter, map, and reduce, highlighting their utility in transforming and summarizing data efficiently. It also introduces the idea of infinite streams and the importance of handling empty streams with optional types.
Takeaways
- π An iterator processes elements in a collection one by one in a sequence, allowing for operations like counting words longer than a certain number of letters.
- π A collection can be thought of as a stream, where elements are processed in a continuous flow, similar to watching a news ticker on TV.
- π Stream processing is an alternative to iterative processing, allowing for a different style of handling collections through functions that take and produce streams.
- π Stream processing can be more efficient as it supports parallel processing, making use of multiple computational resources for operations that are independent.
- π The concept of lazy evaluation in streams means that operations are performed only when necessary, potentially saving resources by not generating entire sequences.
- π§ Stream operations are typically non-destructive, meaning the original collection remains unchanged after the stream has been processed.
- π Streams can be created from various sources, including collections, arrays, or functions that generate values on demand.
- π Stream transformations include operations like filtering, mapping, and limiting, which can be chained together in a pipeline to process data.
- βΈ The use of 'limit' and 'skip' allows for control over the number of elements processed in a stream, enabling more granular data handling.
- π Operations like 'max', 'min', and 'findFirst' can be used as terminal steps in a stream pipeline to produce a single result from a stream.
- β οΈ Handling empty streams is important as certain operations may not be valid without elements to process, often returning an 'Optional' type to indicate the possibility of no result.
Q & A
What is an iterator and how does it work with collections?
-An iterator is a mechanism that allows you to traverse through a collection, such as a list or a set, and produce the elements one by one in a sequence. It is used to run through items in a collection and perform operations on them, such as counting words longer than 10 letters in a list of strings.
How does stream processing differ from iterative processing using an iterator?
-Stream processing allows you to think of the elements in a collection as a stream of values, similar to watching a TV stream. Instead of explicitly iterating through the collection one element at a time, you write functions that take these streams as input, process them, and produce a stream as output. This approach is more declarative and can potentially be parallelized, unlike iterative processing which is more imperative and sequential.
What is the advantage of using streams over iterators for processing collections?
-Streams offer a declarative programming style where you describe the transformations you want to achieve rather than how to achieve them. They also support lazy evaluation, allowing operations to be performed in parallel if computational resources are available, and can be terminated early if the desired result is achieved, making them more efficient in some scenarios.
How can you convert a collection into a stream in Java?
-In Java, you can convert a collection into a stream by using the `stream()` method provided by the Collections interface. This method takes a collection and returns a stream of its elements. For arrays, you can use the `Stream.of()` static method to achieve the same.
What is the concept of lazy evaluation in the context of streams?
-Lazy evaluation in streams means that the next step in processing is started as soon as the output of the previous step is available. This allows for efficient processing as you don't need to generate the entire stream before starting the next operation. It also enables operations like stopping the stream generation once a certain condition is met.
How can you create a stream from a function in Java?
-You can create a stream from a function using `Stream.generate()` which takes a function that generates values on demand. This is useful when you don't have a collection to start with but need to produce a sequence of values dynamically.
What is the purpose of the `filter` operation in streams?
-The `filter` operation in streams is used to select a subset of the stream's elements based on a predicate. It keeps only those elements for which the predicate returns true, effectively allowing you to extract specific items from the stream, such as words longer than 10 letters.
How does the `map` operation transform the elements of a stream?
-The `map` operation applies a function to each element in the stream, transforming them into a new form. For example, it can be used to extract the first letter of each word in a stream of strings or to convert each element in a way that suits theεη» processing needs.
What is the significance of `flatMap` in stream processing?
-The `flatMap` operation is a variant of `map` that flattens the output. If the `map` operation results in a stream of lists (or other nested structures), `flatMap` collapses these into a single stream, making it easier to handle the resulting elements as a simple sequence.
How can you handle empty streams in Java when performing operations like `max` or `first`?
-Operations like `max`, `min`, and `first` on streams may result in an `Optional` type to handle cases where the stream is empty. This approach ensures that the result is well-defined even when no elements are present in the stream, preventing errors like `NoSuchElementException`.
Outlines
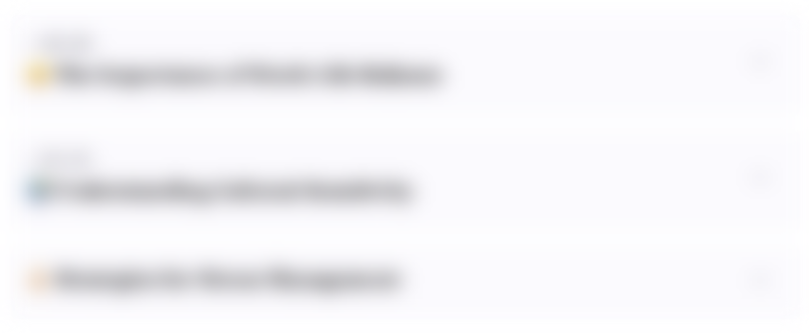
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
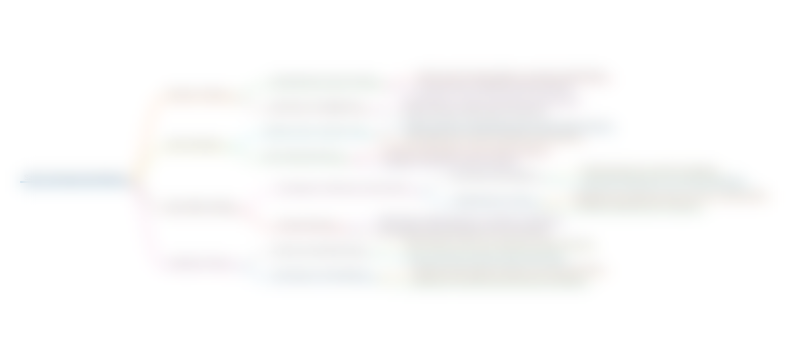
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
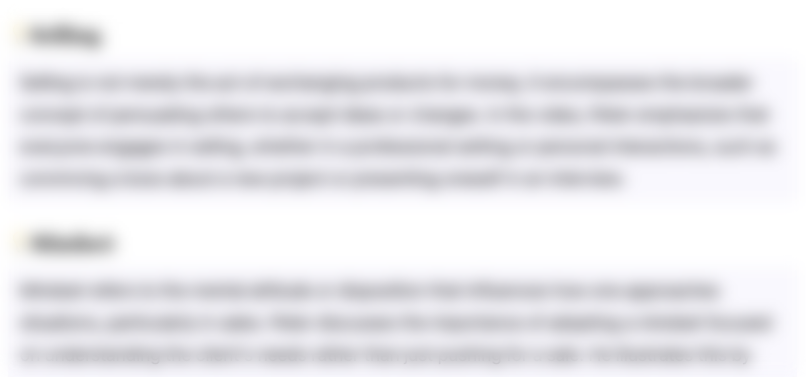
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
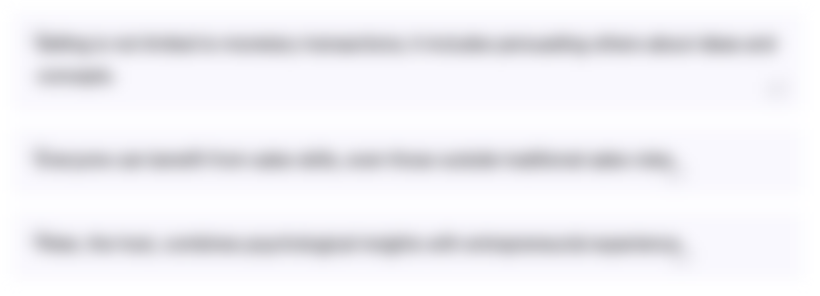
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
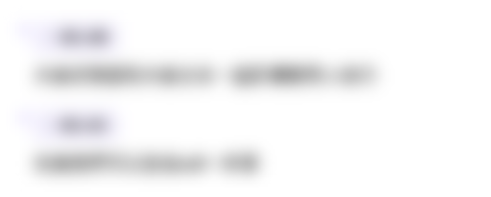
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)