Excel VBA Programming - Variables and Data Types | 2 - Variable Declarations and Assignments
Summary
TLDRThis lesson delves into the fundamentals of VBA programming with a focus on variables. It explains variables as placeholders for data, which can range from numbers to complex objects. The script covers variable declaration using the 'Dim' keyword, assigning data types, and the importance of variable names. It highlights the 'Option Explicit' setting for mandatory variable declaration and discusses variable scope, emphasizing the simplification variables bring to referencing and manipulating data within VBA code.
Takeaways
- π Variables in VBA are placeholders for data, which can be a number, text, or an entire VBA object like a range, workbook, or worksheet.
- π The process of using a variable involves two main steps: declaration, where you inform VBA about the variable's name and data type, and assignment, where you give the variable a specific value.
- π‘ The 'Dim' keyword in VBA is short for 'Dimension' and is used to declare a variable, indicating the start of the variable declaration.
- π Variable names in VBA should be descriptive, not exceed 255 characters, and cannot contain spaces, special characters, or be the same as VBA reserved keywords.
- π« Variable names cannot start with a number or an underscore, and must be unique within the procedure they are declared in due to the concept of scope.
- β οΈ 'Option Explicit' at the top of each module enforces that all variables must be declared before they are used, preventing potential errors.
- π’ Data types in VBA, such as 'Integer', define what kind of data a variable will store, which helps VBA allocate the appropriate amount of memory.
- π VBA is not case sensitive, meaning 'Age' and 'age' are considered the same variable, but it's best to maintain consistent casing for clarity.
- π Variables can simplify the process of referencing complex objects in VBA by using a simple name instead of a fully qualified reference.
- π οΈ Variables can be used in operations just like plain numbers, making them versatile tools for manipulating data within a VBA program.
- π The importance of variables will become more evident as programming progresses, especially when accessing and manipulating data on spreadsheets.
Q & A
What is a variable in the context of VBA programming?
-A variable in VBA programming is a placeholder for a piece of data, which can be a number, text, string, or a complete VBA object such as a range, workbook, or worksheet.
Why are variables important in VBA programming?
-Variables are important because they simplify the process of referencing complex objects and allow developers to avoid repeatedly writing out the same object references.
What are the two parts of a variable in VBA?
-The two parts of a variable in VBA are the declaration and the assignment of a specific value.
What does the keyword 'Dim' stand for in VBA?
-The keyword 'Dim' in VBA stands for Dimension, which is used to declare a variable and allocate a piece of computer memory for it.
What is the purpose of the 'Option Explicit' setting in the VBA editor?
-The 'Option Explicit' setting mandates that every variable must be declared before it is assigned a value, ensuring that the program explicitly states the intention to have a variable of a certain type.
What are some restrictions on variable names in VBA?
-Variable names in VBA cannot be greater than 255 characters, cannot contain spaces, mathematical symbols, punctuation characters, special characters, or be reserved VBA keywords. They also cannot start with a number or an underscore.
What is the difference between variable declaration and variable assignment in VBA?
-Variable declaration in VBA is the process of telling the program that a certain variable of a specific type will be used, while variable assignment is the process of giving that variable an actual value.
What is the scope of a variable in VBA?
-The scope of a variable in VBA refers to the boundary within which a variable exists. A variable only exists as long as the procedure in which it is declared is running.
Is VBA case sensitive when it comes to variable names?
-No, VBA is not case sensitive. A variable with a capital letter and the same variable with a lowercase letter are considered the same in VBA.
How can you use a variable in VBA after it has been declared and assigned a value?
-After a variable has been declared and assigned a value, you can reference it anywhere in the procedure's code, and it will represent the assigned value. You can also perform operations with it as you would with a plain number.
Why should variable names be descriptive in VBA?
-Variable names should be descriptive to clearly indicate what the variable represents or holds, making the code more readable and easier to understand.
Outlines
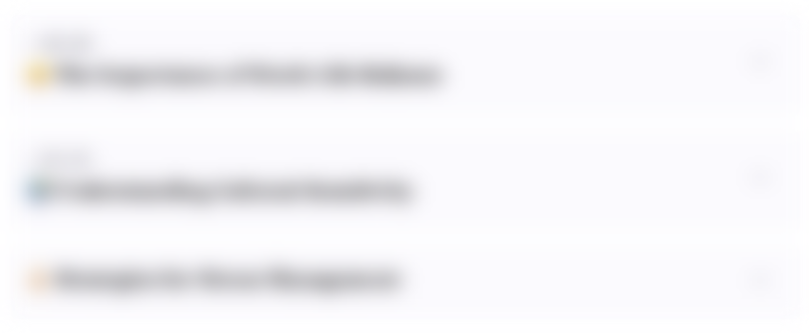
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
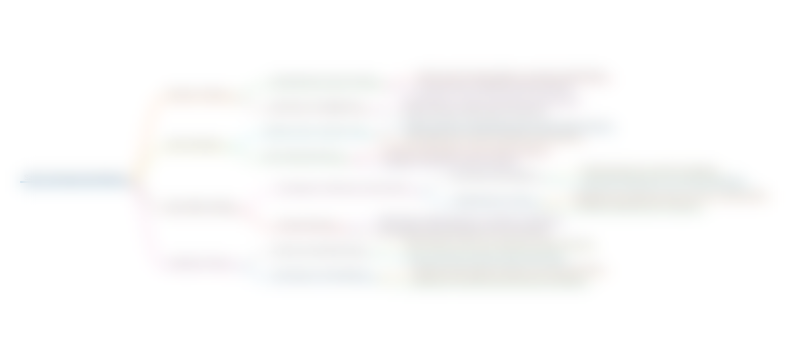
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
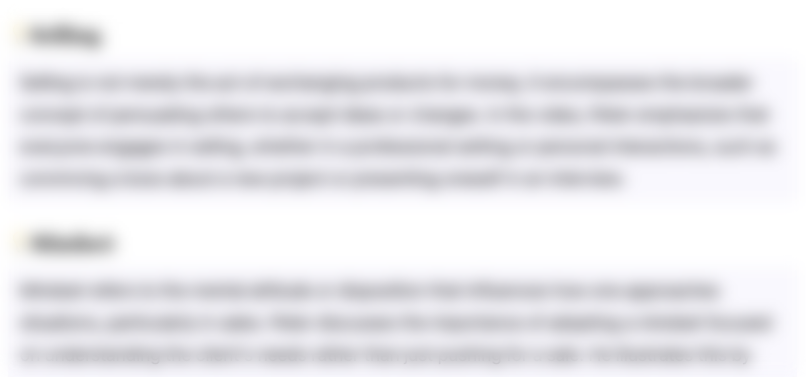
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
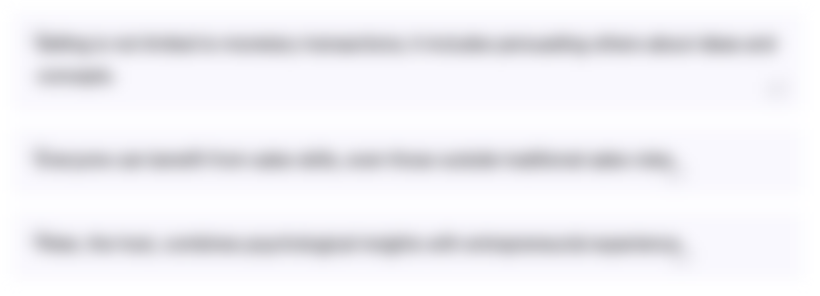
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
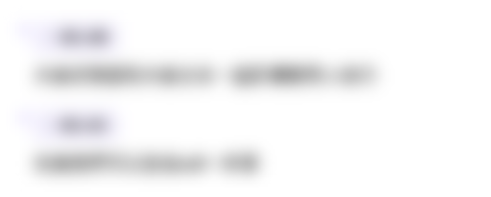
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Portugol M00A02 Como Funcionam as Linguagens

Excel VBA Programming - The Excel Object Model | 1 - Object Oriented Programming in Real Life
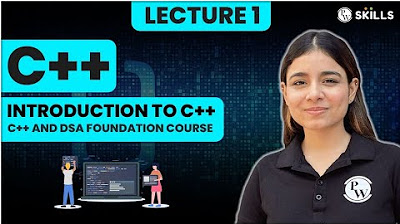
Introduction to C++ | Lecture 1 | C++ and DSA Foundation Course

Variables & Data Types In C: C Tutorial In Hindi #6
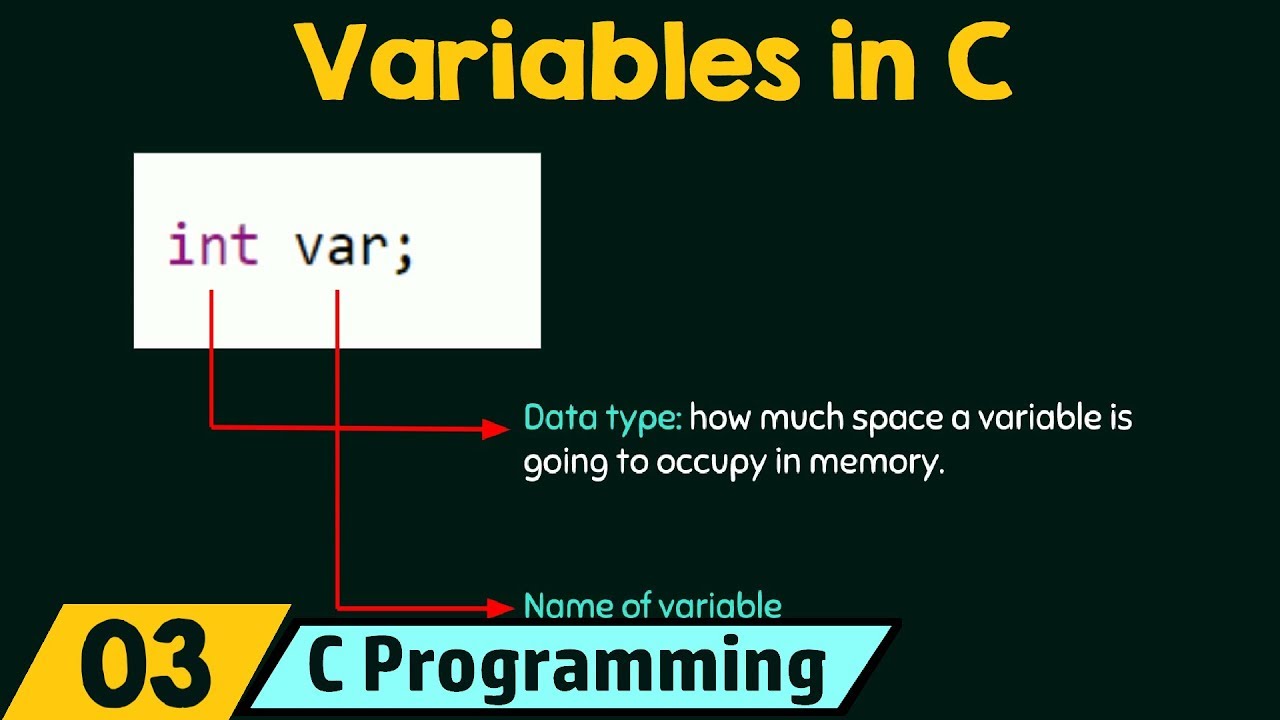
Introduction to Variables
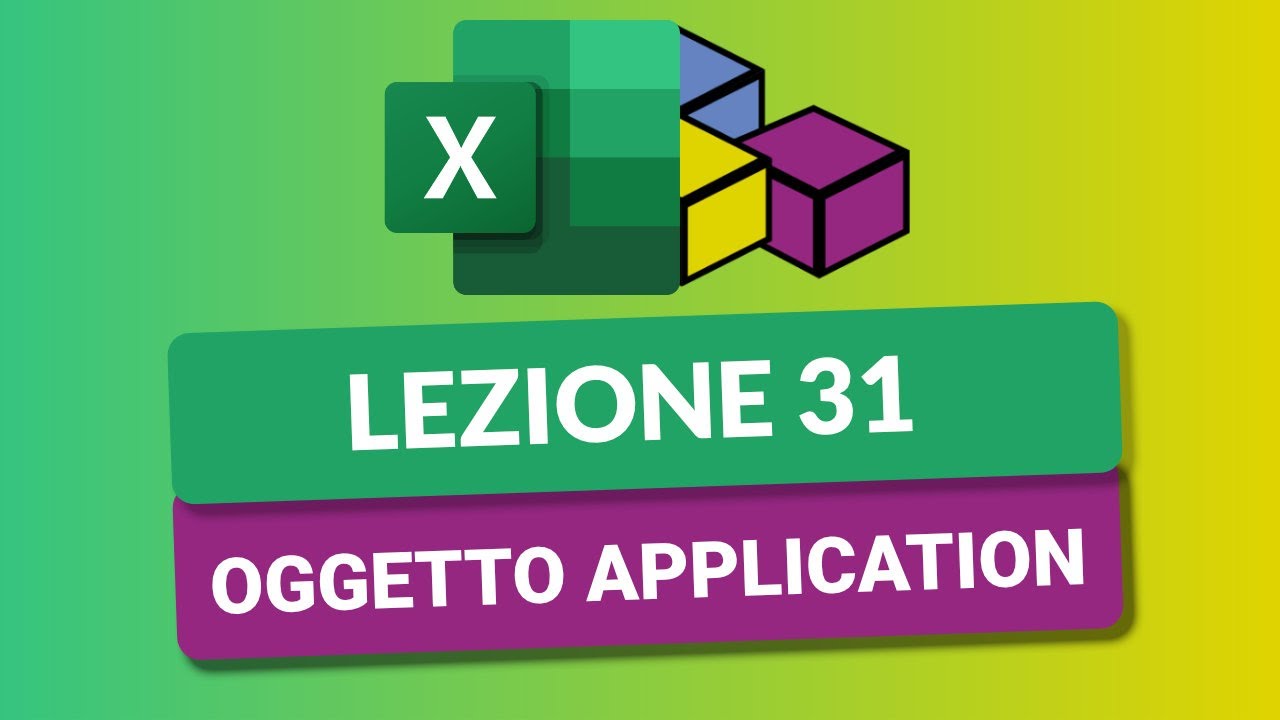
Oggetto Application - VBA Excel Tutorial Italiano 31
5.0 / 5 (0 votes)