undefined vs not defined in JS 🤔 | Namaste JavaScript Ep. 6
Summary
TLDRThis JavaScript tutorial delves into the concept of 'undefined', a unique keyword in JavaScript that signifies a variable has been declared but not yet assigned a value. The script explains how JavaScript's execution context pre-allocates memory for variables, even before code execution, setting them to 'undefined' as a placeholder. It contrasts 'undefined' with 'not defined', emphasizing the importance of not manually setting variables to 'undefined'. The video also touches on JavaScript's flexibility as a loosely typed language, allowing variables to hold different data types, and warns against assigning 'undefined' to variables, suggesting it should be reserved for its original purpose of indicating uninitialized variables.
Takeaways
- 🌐 JavaScript's 'undefined' is a unique keyword not found in other languages, and it plays a significant role in how JavaScript manages memory and execution.
- 💾 Before any code is executed, JavaScript allocates memory for variables and functions, assigning them a 'undefined' placeholder to indicate no value has been set yet.
- 🔍 Using the debugger, one can observe that variables hold 'undefined' before any values are assigned to them, showcasing JavaScript's pre-allocation of memory.
- 🚫 Attempting to access a variable that has not been declared results in 'not defined', which is different from 'undefined', indicating no memory has been allocated for it.
- 🔄 JavaScript is a loosely typed language, allowing variables to hold any data type, which contrasts with strictly typed languages that enforce data types for variables.
- 🛠️ JavaScript performs type coercion behind the scenes, allowing flexibility in assigning different data types to the same variable without explicit type declarations.
- ❌ It's considered bad practice to assign 'undefined' to a variable as it can lead to inconsistencies and confusion, as 'undefined' is meant to indicate a lack of assignment.
- 📚 Understanding the difference between 'undefined' and 'not defined' is crucial for debugging and writing clear JavaScript code.
- 🔑 The script emphasizes the importance of proper variable initialization and the avoidance of assigning 'undefined' to variables unnecessarily.
- 🎯 The video concludes with a teaser for the next topic, 'scope chain', which is a critical concept in JavaScript and a common interview question.
Q & A
What is the significance of the 'undefined' keyword in JavaScript?
-In JavaScript, 'undefined' is a special keyword that signifies a variable has been declared but not yet assigned a value. It is a placeholder value that the JavaScript engine assigns to variables during the memory allocation phase before any code is executed.
How does JavaScript's memory allocation for variables work?
-JavaScript allocates memory to variables during the creation of the global execution context, even before any code is executed. This allocation includes assigning the 'undefined' value as a placeholder until the variable is explicitly assigned a value.
What is the difference between 'undefined' and 'not defined' in JavaScript?
-In JavaScript, 'undefined' refers to a declared variable that has not been assigned a value yet, whereas 'not defined' means the variable has not been declared at all. 'Undefined' takes up memory space with a placeholder value, while 'not defined' variables do not exist in the scope.
Why is it considered a bad practice to assign 'undefined' to a variable?
-Assigning 'undefined' to a variable is considered bad practice because 'undefined' is a special value used by the JavaScript engine to indicate that a variable has not been assigned a value. Overriding this with an assignment can lead to confusion and inconsistencies in the code.
What does it mean for JavaScript to be a loosely typed language?
-Being a loosely typed language means that JavaScript does not require variables to be explicitly typed. A single variable can hold values of different types throughout its lifecycle without needing a type declaration.
Can you provide an example of how JavaScript handles type coercion?
-JavaScript handles type coercion automatically when different data types are used in expressions. For example, if a string and a number are used in an arithmetic operation, JavaScript will convert the string to a number to perform the calculation.
What is the purpose of using 'debugger' in JavaScript?
-The 'debugger' statement in JavaScript is used to initiate a debugging session at that point in the code. When the code execution reaches this statement, it pauses, allowing developers to inspect variables and step through the code to debug issues.
How can you check if a variable is 'undefined' in JavaScript?
-You can check if a variable is 'undefined' in JavaScript using the strict equality operator '==='. For example, 'if (a === undefined)' will evaluate to true if the variable 'a' has not been assigned a value.
What is the scope chain, and why is it important in JavaScript?
-The scope chain is a data structure in JavaScript that keeps track of all the variables that are currently in the scope of the currently executing code. It is important for understanding how variables are looked up and accessed in different scopes, which is crucial for writing maintainable and efficient code.
Why is the scope chain a hot interview topic for JavaScript developers?
-The scope chain is a hot interview topic because it tests a candidate's understanding of how JavaScript manages variable scope and execution contexts. Mastery of scope chain concepts is essential for writing efficient and bug-free JavaScript code.
Outlines
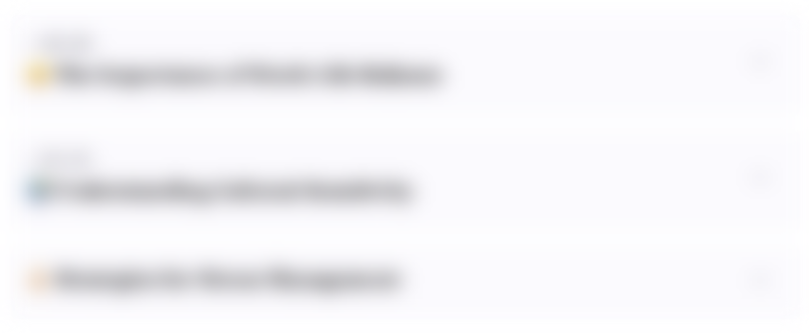
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
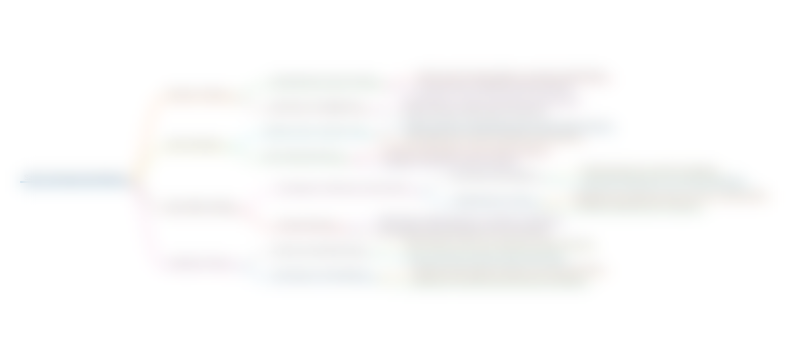
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
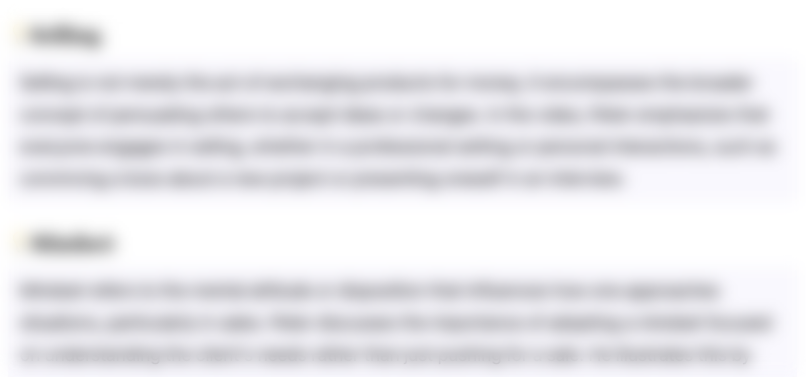
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
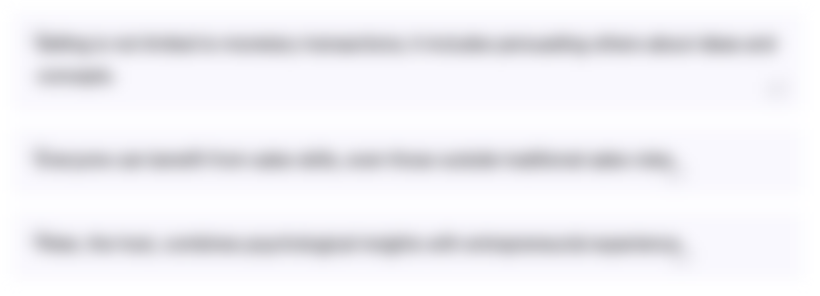
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
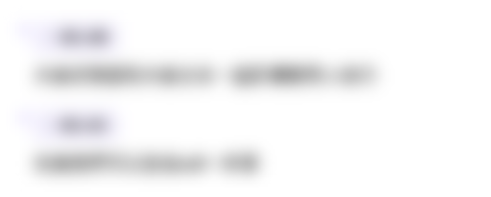
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Default Parameters | JavaScript 🔥 | Lecture 119

How JavaScript Code is executed? ❤️& Call Stack | Namaste JavaScript Ep. 2
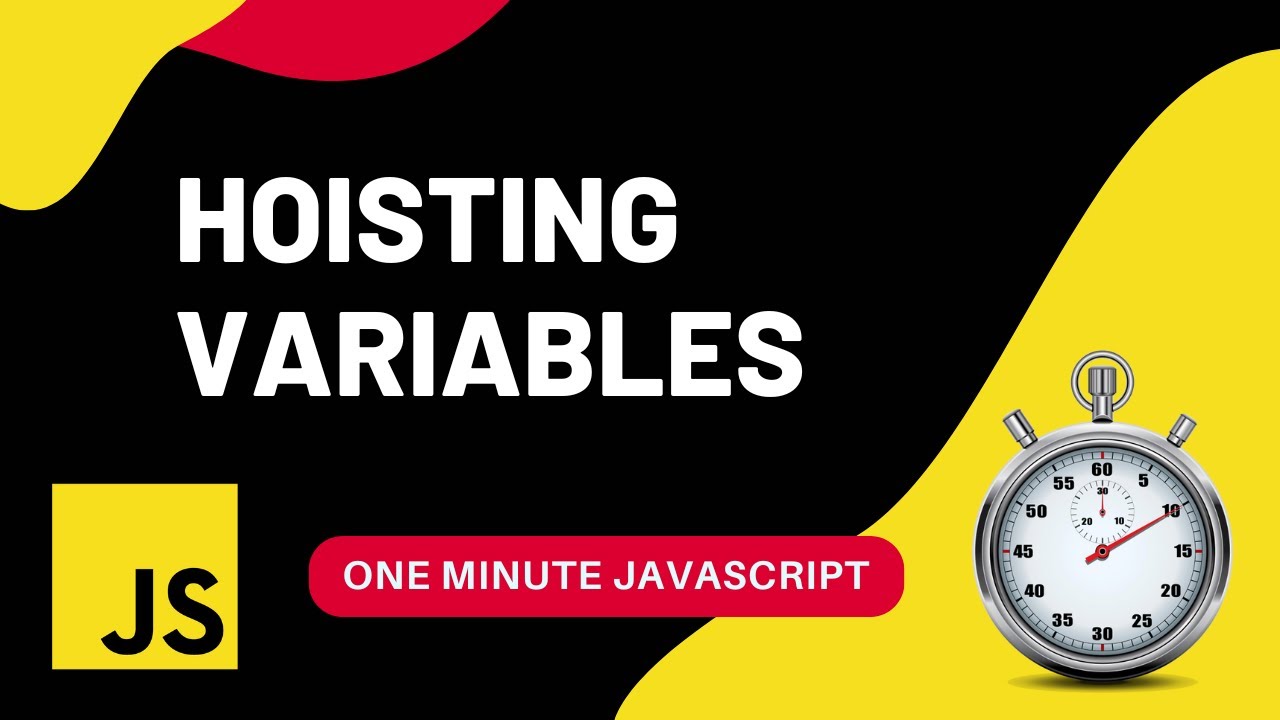
Hoisting Variables | Javascript | One Minute Javascript | 1 Min JS | Quick JS

Hoisting in JavaScript 🔥(variables & functions) | Namaste JavaScript Ep. 3
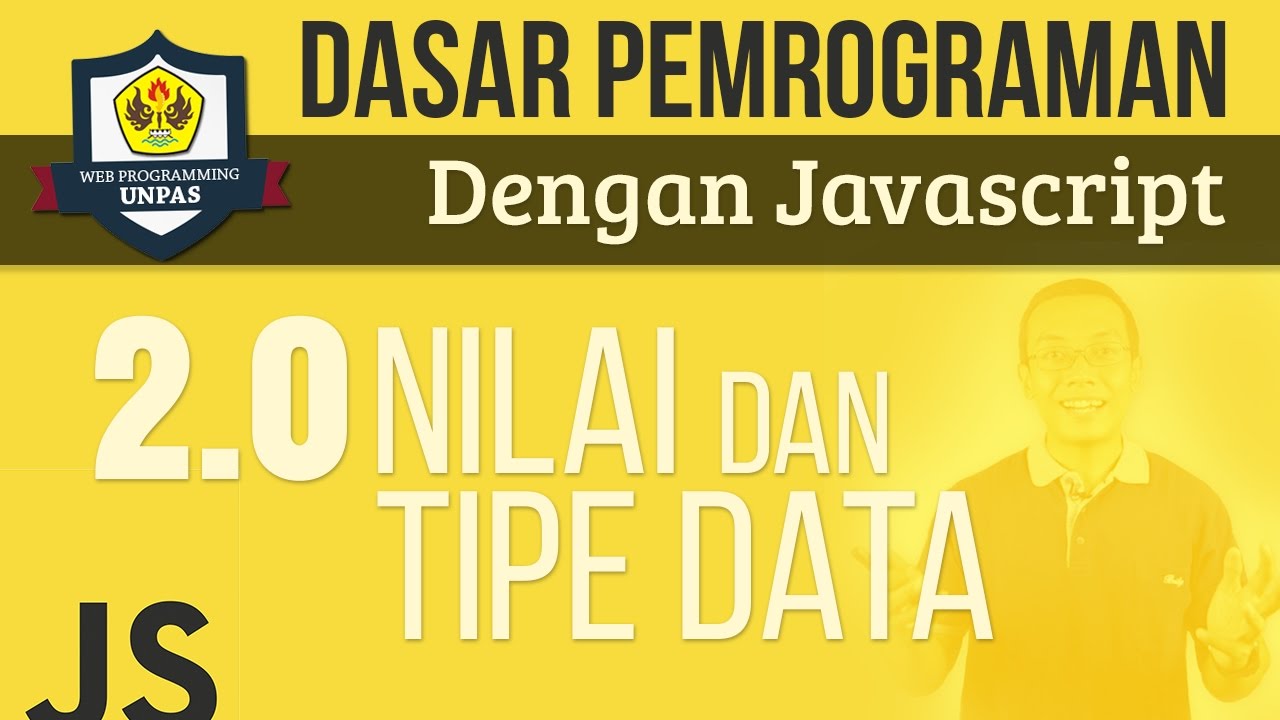
NILAI DAN TIPE DATA PADA JAVASCRIPT
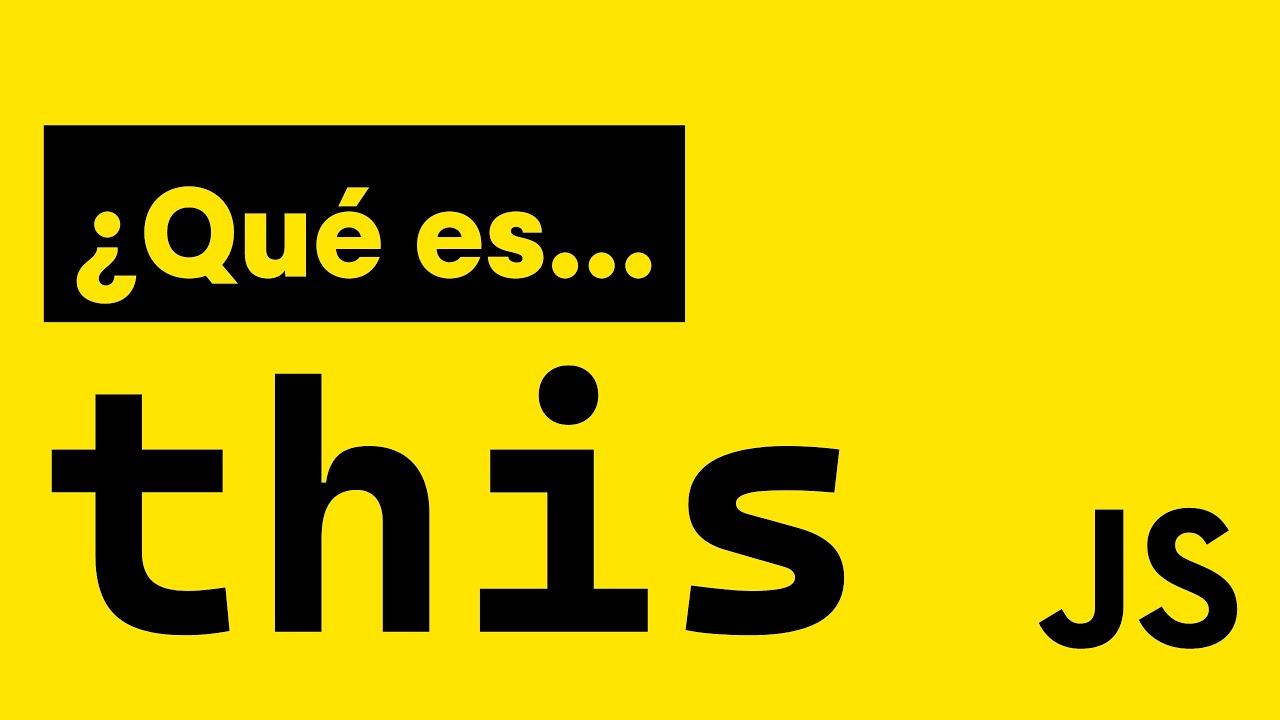
📚 ¿Qué es this en JavaScript? Explicación fácil, directa y sencilla con ejemplos
5.0 / 5 (0 votes)